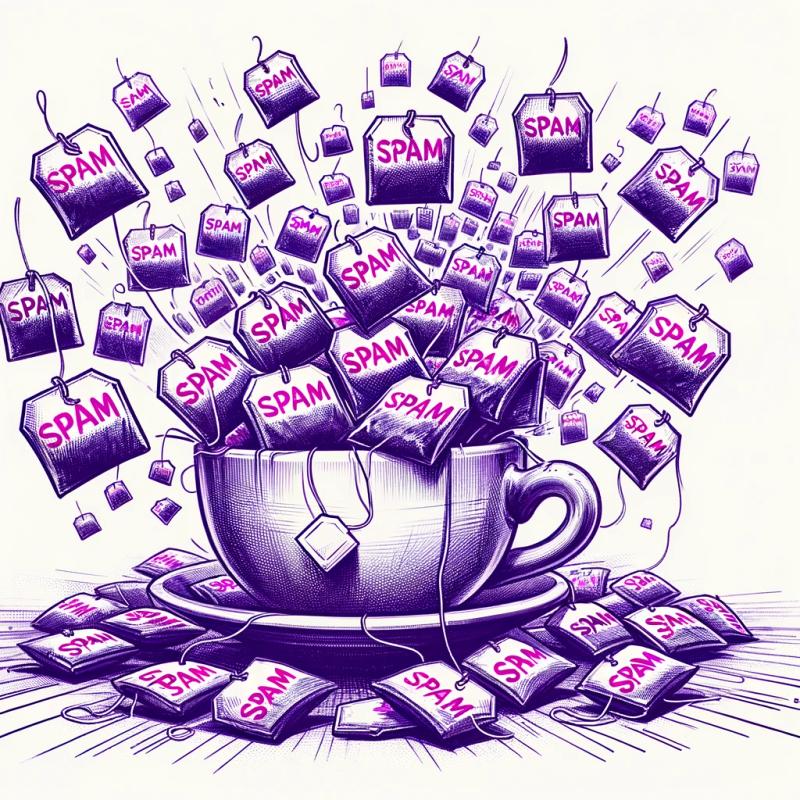
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
antd-form-has-error-hoc
Advanced tools
Readme
A ant-design form enhance high order component , auto collects form errors , you can use this.props.hasError
disabled your submit button, effectively reduce the amount of code
using yarn
:
yarn add antd-form-has-error-hoc
using npm
:
npm install antd-form-has-error-hoc --save
online example : https://lijinke666.github.io/antd-form-has-error-hoc/
import React from 'react';
import ReactDOM from 'react-dom';
import withAntdFormHasError, { IAntdFormHasErrorProps } from 'antd-form-has-error-hoc';
import { Form, Input, Button } from 'antd';
import { FormComponentProps } from 'antd/lib/form';
type Props = IAntdFormHasErrorProps & FormComponentProps;
@Form.create()
@withAntdFormHasError()
class App extends PureComponent<Props> {
render() {
const { getFieldDecorator } = this.props.form;
console.log(this.props.hasError) // true
return (
<Form>
<Form.Item>
{getFieldDecorator('username', {
// if set required true the hoc is get the field error
rules: [{ required: true, message: 'Please input your username!' }]
})(<Input placeholder="Username" />)}
</Form.Item>
<Form.Item>
{getFieldDecorator('password', {
// if set required false the hoc is ignore the field error
rules: [{ required: false, message: 'Please input your Password!' }]
})(<Input type="password" placeholder="Password" />)}
</Form.Item>
<Form.Item>
<Button
type="primary"
htmlType="submit"
// all required field not empty and validate success `this.props.hasError` is false
disabled={this.props.hasError}
>
Log in
</Button>
</Form.Item>
</Form>
);
}
}
// for edit
@Form.create()
@withAntdFormHasError()
class App extends PureComponent<Props> {
render() {
const { getFieldDecorator } = this.props.form;
console.log(this.props.hasError) // false
return (
<Form>
<Form.Item>
{getFieldDecorator('username', {
rules: [{ required: true, message: 'Please input your username!' }]
})(<Input placeholder="Username" />)}
</Form.Item>
<Form.Item>
{getFieldDecorator('password', {
rules: [{ required: true, message: 'Please input your Password!' }]
})(<Input type="password" placeholder="Password" />)}
</Form.Item>
</Form>
);
}
componentDidMount() {
// if edit , after set fields value complete , `this.props.hasError` is false
this.props.form.setFieldsValue({
username: "test user name",
password: 123456
})
}
}
// for ignore
@Form.create()
@withAntdFormHasError(['username','password'])
// or @withAntdFormHasError((fields)=> fields.slice(0,2))
class App extends PureComponent<Props> {
render() {
const { getFieldDecorator } = this.props.form;
// username and password field is required, but by hoc , you can ignore
console.log(this.props.hasError) // true
return (
<Form>
<Form.Item>
{getFieldDecorator('username', {
rules: [{ required: true, message: 'Please input your username!' }]
})(<Input placeholder="Username" />)}
</Form.Item>
<Form.Item>
{getFieldDecorator('password', {
rules: [{ required: true, message: 'Please input your Password!' }]
})(<Input type="password" placeholder="Password" />)}
</Form.Item>
</Form>
);
}
}
@Form.create()
@withAntdFormHasError()
class DynamicForm extends PureComponent {
state = {
visible: true,
fields: []
}
onToggleVisible = e => {
this.setState(
{
visible: e.target.checked
},
() => {
// form item dynamic increase or reduce you can call `this.props.updateFieldsStatus()`
// get new `this.props.hasError`
this.props.updateFieldsStatus()
}
)
}
addFields = () => {
this.setState({
fields: [...this.state.fields, 1]
}, () => {
this.props.updateFieldsStatus()
})
}
render() {
const { visible, fields } = this.state
const { getFieldDecorator } = this.props.form
return (
<Form style={formStyles}>
<h2>Dynamic Form</h2>
<Form.Item>
{getFieldDecorator('username', {
rules: [{ required: true, message: 'Please input your username!' }]
})(<Input placeholder="Username" />)}
</Form.Item>
<Form.Item>
{getFieldDecorator('password', {
rules: [{ required: true, message: 'Please input your Password!' }]
})(<Input type="password" placeholder="Password" />)}
</Form.Item>
{visible && (
<Form.Item>
{getFieldDecorator('phone', {
rules: [{ required: true, message: 'Please input your Phone!' }]
})(<Input type="text" placeholder="phone" />)}
</Form.Item>
)}
{fields.map((_, index) => {
return (
<Form.Item key={index}>
{getFieldDecorator(`field-${index}`, {
rules: [{ required: true, message: 'required' }]
})(<Input type="text" placeholder="phone" />)}
</Form.Item>
)
})}
<Form.Item>
<Button
type="primary"
htmlType="submit"
disabled={this.props.hasError}
>
Log in
</Button>
<Checkbox
style={{ marginLeft: 20 }}
checked={visible}
onChange={this.onToggleVisible}
>
toggle phone visible
</Checkbox>
<Button type="link" onClick={this.addFields}>
Add Fields
</Button>
</Form.Item>
</Form>
)
}
}
class ParentComponent extends PureComponent {
render() {
// if use `withAntdFormHasError` wrapper component in parent component
// use `defaultFieldsValue` fill form item fields
const defaultFieldsValue = {
username: 'test user name',
password: 123456
}
// get antd form ref
const getRef = (form) => {
console.log(form)
}
return (
<CreateForm defaultFieldsValue={defaultFieldsValue} wrappedComponentRef={getRef}/>
)
}
}
@withAntdFormHasError(needIgnoreFields?: string[] | (fields: string[]) => string[])
this.props.hasError
this.props.defaultFieldsValue
this.props.updateFieldsStatus()
git clone https://github.com/lijinke666/antd-form-has-error-hoc.git
npm install
npm start
FAQs
A ant-design form enhance high order component
The npm package antd-form-has-error-hoc receives a total of 7 weekly downloads. As such, antd-form-has-error-hoc popularity was classified as not popular.
We found that antd-form-has-error-hoc demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.