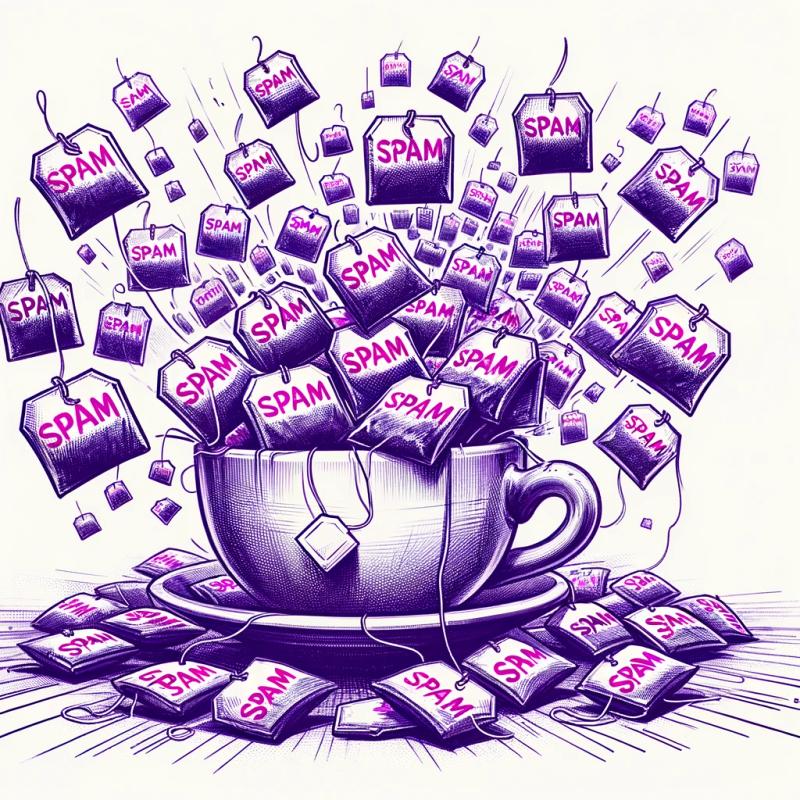
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
archer-svgs
Advanced tools
Readme
实际生产项目中基本都会用到svg
,随着项目迭代svg bundle
的体积会越来越大,在后续迭代中如果每新增或删除一个svg
就会导致svg
bundle的hash发生变化,用户就必须重新下载这个文件,为此会付出额外的流量开支。例如,现在有一个svg
bundle为100kb
,此时新增了一个1kb
的svg
,项目上线后,用户就需要为这1kb
的更新重新加载整个svg bundle
,即101kb
,毫无疑问,这是一种非常愚蠢的行为。这种方式还存在另一个问题,当两个不同的项目拥有相同的svg bundle
时,由于svg bundle
资源在不同域下,用户需要下载2份资源。archer-svgs
就是为了解决这个问题,通过它你可以更灵活、轻便地加载svg。
如果你觉得这个项目还不错,可以给我一个
star
和follow
来支持我 😘
npm install archer-svgs
yarn add archer-svgs
<script src="//unpkg.com/archer-svgs/lib/Archer.min.js"></script>
svg
资源的加载顺序为内存->硬盘缓存->远程服务器资源
,大大提高资源加载效率。
如果想进一步提高加载速度,可以将svg
资源放在cdn
上。
同时由于svg
资源是通过url
进行加载的,因此可以跨域共享diskCache
。
TypeScript
进行, 提供d.ts
文件提高开发效率。XMLHttpRequest
异步加载 svg
svg
archer-svgs
基于XMLHttpRequest
, 只要你的浏览器支持 xhr
,你就可以使用它!兼容性如下图所示:
如果需要在低版本浏览器使用,需要引入promises poly-fill
,
推荐使用taylorhakes/promise-polyfill,体积小并且兼容性很好。
你也可以使用<script>
去加载它。
<script src="https://cdn.jsdelivr.net/npm/promise-polyfill@8/dist/polyfill.min.js"></script>
必须先调用 set()
初始化配置,然后才能使用其它的Archer
方法!
import Archer from 'archer-svgs';
const archer = new Archer();
archer.set({
'ios-airplane': 'https://unpkg.com/ionicons@4.4.2/dist/ionicons/svg/ios-airplane.svg',
'md-airplane': 'https://unpkg.com/ionicons@4.4.2/dist/ionicons/svg/md-airplane.svg',
})
config - paramas
export interface IConfig {
[index: string]: string;
}
添加配置
archer.set({
'ios-airplane': 'https://unpkg.com/ionicons@4.4.2/dist/ionicons/svg/ios-airplane.svg',
})
archer.add({
'md-airplane': 'https://unpkg.com/ionicons@4.4.2/dist/ionicons/svg/md-airplane.svg',
})
/**
* config = {
* 'ios-airplane': 'https://unpkg.com/ionicons@4.4.2/dist/ionicons/svg/ios-airplane.svg',
* 'md-airplane': 'https://unpkg.com/ionicons@4.4.2/dist/ionicons/svg/md-airplane.svg',
* }
* /
startPrefetch
会对config
中的svg
进行预加载!当你调用svg
的时候将大大提高使用速度。
diskCache
为空时,从远程服务资源拉取资源,同时将资源缓存到memory
和diskCache
中。diskCache
不为空时,将本地资源加载到memory
中。archer.startPrefetch();
params
是config.svgs
的key
, 这个方法将返回svg
的内容。
console.log(archer.downloadSvg('ios-airplane'));
result:
<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 512 512"><path d="M407.7 224c-3.4 0-14.8.1-18 .3l-64.9 1.7c-.7 0-1.4-.3-1.7-.9L225.8 79.4c-2.9-4.6-8.1-7.4-13.5-7.4h-23.7c-5.6 0-7.5 5.6-5.5 10.8l50.1 142.8c.5 1.3-.4 2.7-1.8 2.7L109 230.1c-2.6.1-5-1.1-6.6-3.1l-37-45c-3-3.9-7.7-6.1-12.6-6.1H36c-2.8 0-4.7 2.7-3.8 5.3l19.9 68.7c1.5 3.8 1.5 8.1 0 11.9l-19.9 68.7c-.9 2.6 1 5.3 3.8 5.3h16.7c4.9 0 9.6-2.3 12.6-6.1L103 284c1.6-2 4.1-3.2 6.6-3.1l121.7 2.7c1.4.1 2.3 1.4 1.8 2.7L183 429.2c-2 5.2-.1 10.8 5.5 10.8h23.7c5.5 0 10.6-2.8 13.5-7.4L323.1 287c.4-.6 1-.9 1.7-.9l64.9 1.7c3.3.2 14.6.3 18 .3 44.3 0 72.3-14.3 72.3-32S452.1 224 407.7 224z"/></svg>
通过url
加载svg
。
const svg = archer.fetchSvg('https://unpkg.com/ionicons@4.4.2/dist/ionicons/svg/ios-airplane.svg')
console.log(svg);
result:
<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 512 512"><path d="M407.7 224c-3.4 0-14.8.1-18 .3l-64.9 1.7c-.7 0-1.4-.3-1.7-.9L225.8 79.4c-2.9-4.6-8.1-7.4-13.5-7.4h-23.7c-5.6 0-7.5 5.6-5.5 10.8l50.1 142.8c.5 1.3-.4 2.7-1.8 2.7L109 230.1c-2.6.1-5-1.1-6.6-3.1l-37-45c-3-3.9-7.7-6.1-12.6-6.1H36c-2.8 0-4.7 2.7-3.8 5.3l19.9 68.7c1.5 3.8 1.5 8.1 0 11.9l-19.9 68.7c-.9 2.6 1 5.3 3.8 5.3h16.7c4.9 0 9.6-2.3 12.6-6.1L103 284c1.6-2 4.1-3.2 6.6-3.1l121.7 2.7c1.4.1 2.3 1.4 1.8 2.7L183 429.2c-2 5.2-.1 10.8 5.5 10.8h23.7c5.5 0 10.6-2.8 13.5-7.4L323.1 287c.4-.6 1-.9 1.7-.9l64.9 1.7c3.3.2 14.6.3 18 .3 44.3 0 72.3-14.3 72.3-32S452.1 224 407.7 224z"/></svg>
设置预加载svg
的最大并发下载数,默认值是2
。
例如修改最大并发量为5
。
archer.setThreadNum(5);
跨域加载svg静态资源的时候需要服务端配置Access-Control-Allow-Origin
。
import Icon from 'archer-svgs/lib/react';
// 初始化配置
Icon.archer.set({
'ios-airplane': 'https://unpkg.com/ionicons@4.4.2/dist/ionicons/svg/ios-airplane.svg',
});
// 预加载 - 根据实际需求,也可以不进行预加载
Icon.archer.startPrefetch();
<Icon type="ios-airplane"/>
FAQs
load svg async
The npm package archer-svgs receives a total of 13 weekly downloads. As such, archer-svgs popularity was classified as not popular.
We found that archer-svgs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.