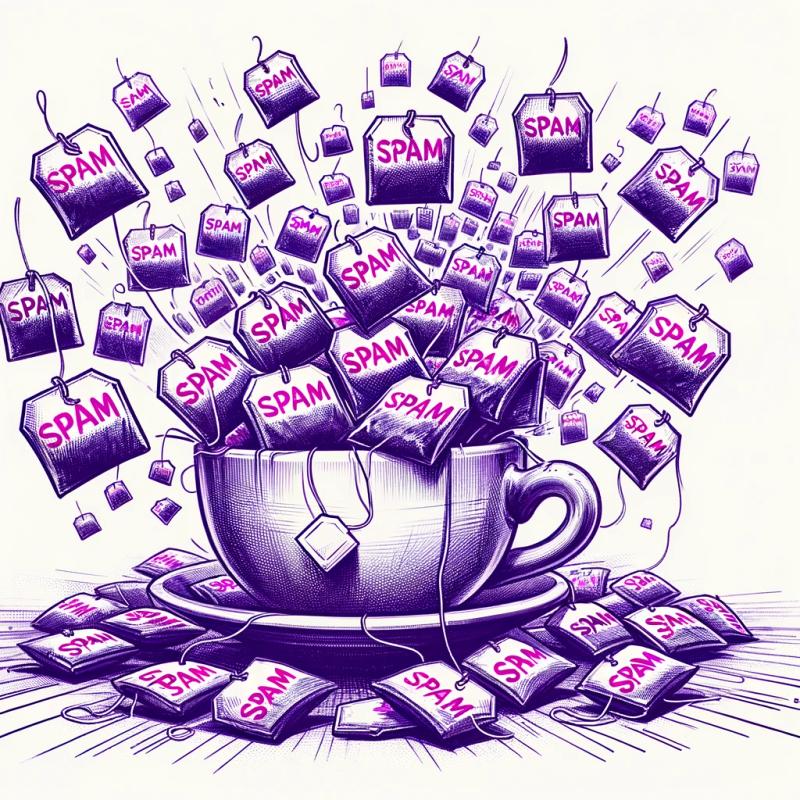
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Readme
A JavaScript utility library to manipulate Function.arguments
A nice library to deal with most of arguments annoying usecases. A first Error object, a second optional argument or a last function as a callback... Arget helps solve all these issues in one line of code. Ex:
function fn () {
var args = arget(arguments);
args.toArray(); // => [1, 'str', 2, true]
args.pick(Number); // => [1, 2]
args.match(null, Boolean, String); // => [1, true, 'str']
}
fn(1, 'str', 2, true);
Second example :
function fn () {
var [foo, bar, foobar] = arget(arguments).match(null, null, Function);
console.log(foo, bar, foobar);
}
fn({}, () => {}); // ==> {}, undefined, () => {}
fn({}, 'value', () => {}); // ==> {}, 'value', () => {}
npm install --save arget
When requiring the arget module, you'll get a function that instanciates the Arget wrapper using the arguments you are passing.
var arget = require('arget');
function fn () {
var wrapper = arget(arguments);
}
/!\ arget instanciates the wrapper whether you pass arguments as argument to arget or not, but you need to keep in mind that there is a huge performance issue if you don't.
Returns the first argument
function fn () {
return arget(arguments).first();
}
fn(1, 2, 3, 4); // ==> 1
fn(); // ==> undefined
Returns the last argument
function fn () {
return arget(arguments).last();
}
fn(1, 2, 3, 4); // ==> 4
fn(); // ==> undefined
.get(position, [constructor = undefined])
Returns element with the constructor and the position specified
function fn () {
return arget(arguments).get(1);
}
fn(true, 2, 3, 4); // ==> 2
fn(); // ==> undefined
function fn () {
return arget(arguments).get(1, Number);
}
fn(true, 2, 3, 4); // ==> 3
.getRight(position, [constructor = undefined])
Returns element with the constructor and the position from the right specified
function fn () {
return arget(arguments).get(1);
}
fn(true, 2, 3, 4); // ==> 3
fn(); // ==> undefined
function fn () {
return arget(arguments).get(0, Number);
}
fn(true, 2, 3, 4); // ==> 4
.all([constructor = undefined])
Returns elements with the constructor specified
function fn () {
return arget(arguments).all(Number);
}
fn(true, 2, 3, 4); // ==> [2, 3, 4]
fn(); // ==> []
Converts arguments object to array
function fn () {
return arget(arguments).toArray();
}
fn(1, 2, 3, 4); // ==> [1, 2, 3, 4]
fn(); // ==> []
.forEach(iteratee)
Iterates over the arguments
function fn () {
arget(arguments).forEach(e => console.log(e));
}
fn(1, 2, 3, 4); // ==> prints 1 2 3 4
The iteratee
takes 3 arguments :
iteratee(item, index, array)
item
: The elementindex
: The element index on the argumentsarray
: The arguments as array function fn () {
arget(arguments).forEach((item, index, array) => {
console.log(item, index, array)
});
}
fn(1, 2, 3);
// prints :
// 1 0 [1, 2, 3]
// 2 1 [1, 2, 3]
// 3 2 [1, 2, 3]
Alias of .forEach( )
.filter(predicate)
Returns an array filtred depending on the returned value of the predicate for each item. The result contains items that the predicate returned a truthy value for.
function fn () {
return arget(arguments).filter(e => e != 3);
}
fn(1, 2, 3, 4); // ==> 1 2 4
The predicate
takes 3 arguments :
predicate(item, index, array)
item
: The elementindex
: The element index on the argumentsarray
: The arguments as array.map(predicate)
Returns an array containing the result of the predicate for each element
function fn () {
return arget(arguments).map(e => e * 2);
}
fn(1, 2, 3, 4); // ==> 2 4 6 8
The iteratee
takes 3 arguments :
iteratee(item, index, array)
item
: The elementindex
: The element index on the argumentsarray
: The arguments as array.pick(contructor[, constructor[, ...]])
Returns an array of elements with the constructors specified
function fn () {
return arget(arguments).pick(Number)
}
fn(true, 1, 2, 'str'); // ==> [1, 2]
function fn () {
return arget(arguments).pick(Number, String)
}
fn(true, 1, {}, 'str'); // ==> [1, 'str']
.omit(contructor[, constructor[, ...]])
Returns an array of elements without those with the constructors specified
function fn () {
return arget(arguments).omit(Number)
}
fn(true, 1, 2, 'str'); // ==> [true, 'str']
function fn () {
return arget(arguments).omit(Number, String)
}
fn(true, 1, {}, 'str'); // ==> [true, {}]
.match(contructor[, constructor[, ...]])
Returns an array of elements depending in the pattern of constructors specified. When a falsy value is given instead of a constructor, the position is filled with an elements not matched yet.
function fn () {
return arget(arguments).match(null, null, Function);
}
fn(1, () => {}); // ==> [1, undefined, () => {}]
fn(1, 'value', () => {}); // ==> [1, 'value', () => {}]
function fn () {
return arget(arguments).match(Array, null, Number, null, Function);
}
fn(1, 2, 3, () => {}, []) // ==> [ [], 2, 1, 3, () => {} ]
/!\ : Note that the match method starts first by putting the items with the constructors specified on their position, then it fills the falsy positions with the rest.
.matchRight(contructor[, constructor[, ...]])
Similar to .match( ) but it loops from right to left.
Returns an array of elements depending in the pattern of constructors specified. When a falsy value is given instead of a constructor, the position is filled with an elements not matched yet.
function fn () {
return arget(arguments).matchRight(null, null, Function);
}
fn(1, () => {}); // ==> [undefined, 1, () => {}]
fn(1, 'value', () => {}); // ==> [1, 'value', () => {}]
function fn () {
return arget(arguments).matchRight(Array, null, Number, null, Function);
}
fn(1, 2, 3, () => {}, []) // ==> [ [], 1, 3, 2, () => {} ]
/!\ : Note that the matchRight method starts first by putting the items with the constructors specified on their position, then it fills the falsy positions with the rest.
Returns the number of elements
function fn () {
return arget(arguments).length
}
fn(); // ==> 0
fn(1, () => {}); // ==> 2
fn(1, 'value', () => {}); // ==> 3
MIT
FAQs
manipulate arguments properly
The npm package arget receives a total of 0 weekly downloads. As such, arget popularity was classified as not popular.
We found that arget demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.