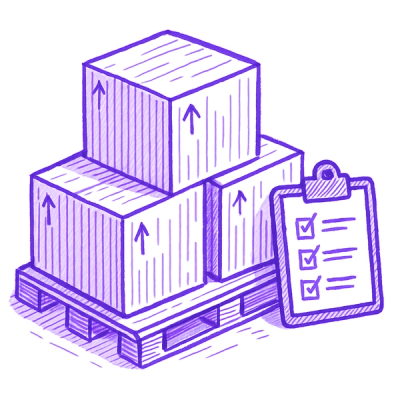
Security News
Open Source Maintainers Feeling the Weight of the EU’s Cyber Resilience Act
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
The 'argv' npm package is a simple and lightweight library for parsing command-line arguments in Node.js applications. It allows developers to easily access and manipulate command-line arguments passed to their scripts.
Basic Argument Parsing
This feature allows you to parse command-line arguments passed to your Node.js script. The 'argv.run()' method returns an object containing the parsed arguments.
const argv = require('argv');
const args = argv.run();
console.log(args);
Defining Options
This feature allows you to define options for your command-line arguments. You can specify the name, short name, type, description, and example usage for each option.
const argv = require('argv');
argv.option({
name: 'port',
short: 'p',
type: 'int',
description: 'The port to listen on',
example: "'script --port=8080' or 'script -p 8080'"
});
const args = argv.run();
console.log(args.options.port);
Handling Commands
This feature allows you to define and handle specific commands. Each command can have its own description and callback function that gets executed when the command is invoked.
const argv = require('argv');
argv.command('start', 'Start the server', function() {
console.log('Server started');
});
argv.command('stop', 'Stop the server', function() {
console.log('Server stopped');
});
argv.run();
Yargs is a powerful and feature-rich library for parsing command-line arguments in Node.js. It offers a wide range of functionalities, including support for commands, options, and argument validation. Compared to 'argv', 'yargs' provides more advanced features and better documentation.
Commander is a popular library for building command-line interfaces in Node.js. It allows you to define commands, options, and arguments with ease. 'Commander' is known for its simplicity and ease of use, making it a good alternative to 'argv' for basic CLI applications.
Minimist is a lightweight library for parsing command-line arguments in Node.js. It focuses on simplicity and minimalism, providing a straightforward way to access command-line arguments. 'Minimist' is a good choice if you need a small and fast library without the additional features offered by 'argv'.
argv
is a simple, zero dependency, command line argument parser for NodeJS. For a more feature-full CLI parser, checkout Commander.js or Yargs
$ npm install argv
var argv = require( 'argv' );
var args = argv.option( options ).run();
-> { targets: [], options: {} }
Runs the argument parser on the global arguments. Custom arguments array can be used by passing into this method
// Parses default arguments 'process.argv.slice( 2 )'
argv.run();
// Parses array instead
argv.run(["--option=123", "-o", "123"]);
argv
is a strict argument parser, which means all options must be defined before parsing starts.
argv.option({
name: "option",
short: "o",
type: "string",
description: "Defines an option for your script",
example: "'script --option=value' or 'script -o value'",
onset: function (args) {
args; // Object of current arguments parsed
},
});
Modules are nested commands for more complicated scripts. Each module has it's own set of options that have to be defined independently of the root options.
argv.mod({
mod: 'module',
description: 'Description of what the module is used for',
options: [ list of options ]
});
Types convert option values to useful JS objects. They are defined along with each option.
string
: Ensure values are stringspath
: Converts value into a fully resolved path.integer | int
: Converts value into an integerfloat
: Converts value into a float numberboolean | bool
: Converts value into a boolean object. 'true' and '1' are converted to true, everything else is false.csv
: Converts value into an array by splitting on comma's.list
: Allows for option to be defined multiple times, and each value added to an array[list|csv],[type]
: Combo type that allows you to create a list or csv and convert each individual value into a type.argv.option([
{
name: 'option',
type: 'csv,int'
},
{
name: 'path',
short: 'p',
type: 'list,path'
}
]);
// csv and int combo
$ script --option=123,456.001,789.01
-> option: [ 123, 456, 789 ]
// list and path combo
$ script -p /path/to/file1 -p /path/to/file2
-> option: [ '/path/to/file1', '/path/to/file2' ]
You can also create your own custom type for special conversions.
argv.type( 'squared', function( value ) {
value = parseFloat( value );
return value * value;
});
argv.option({
name: 'square',
short: 's',
type: 'squared'
});
$ script -s 2
-> 4
Defining the scripts version number will add the version option and print it out when asked.
argv.version( 'v1.0' );
$ script --version
v1.0
Custom information can be displayed at the top of the help printout using this method
argv.info( 'Special script info' );
$ script --help
Special script info
... Rest of Help Doc ...
If you have competing scripts accessing the argv
object, you can clear out any previous options that may have been set.
argv.clear().option([new options()]);
argv
injects a default help option initially and on clears. The help() method triggers the help printout.
argv.help();
FAQs
CLI Argument Parser
The npm package argv receives a total of 716,045 weekly downloads. As such, argv popularity was classified as popular.
We found that argv demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.