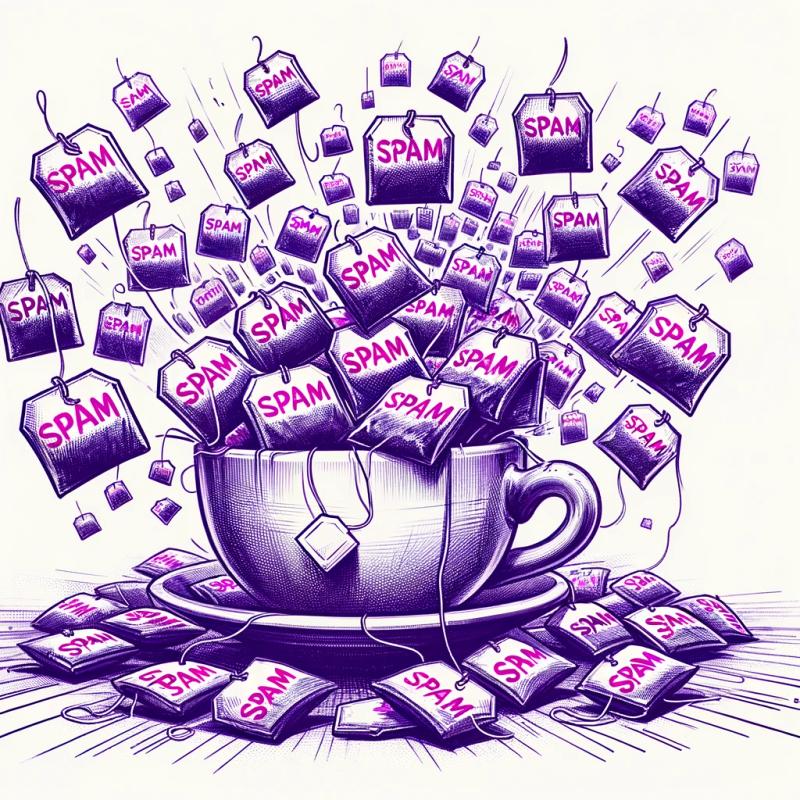
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
array-async-utils
Advanced tools
Changelog
1.0.1 - 2024-02-02
Readme
Small set of utility functions to perform async array mapping, filtering and reducing.
Simply install the array-async-utils
from npm.
You can use one of the following commands, or the equivalent command of the package manager of your choice.
npm install array-async-utils
yarn add array-async-utils
These funcitons accepts the input array as first paramenter, while the arguments, types and returned value resemble as much as possible the ones of the equivalent Array method.
Same behaviour as Array.map, but supports an async callback: all async callbacks are executed in parallel.
If one callback throws an error, the whole map function throws as well.
import { asyncMap } from 'array-async-utils';
import { getUser } from 'apis/user';
const yourFunction = async () => {
const userIds = ['92f9e7a1', '59ef0d84', '6b6cafba'];
const users = await asyncMap(userIds, async (userId) => {
const { user } = await getUser(userId);
return user;
});
};
// ...
const array = [1, 2, 3];
const mappedArray = await asyncMap<typeof array, MappedElement>(
array,
async (element) => {
// ...
}
);
// ^^^ callback will expect Promise<MappedElement> return type, and mappedArray will be of type MappedElement[]
Same as asyncMap
, but async callbacks are executed in series: each one is executed when the previous is finished.
Even if not common, it can be useful when some rece condition might happen in the async callback.
If one callback throws an error, the whole map function throws as well.
import { asyncSerialMap } from 'array-async-utils';
import { getUser } from 'apis/user';
import { userCache } from 'cache/user';
const yourFunction = async () => {
const userIds = ['92f9e7a1', '59ef0d84', '6b6cafba'];
const users = await asyncSerialMap(userIds, async (userId) => {
const cachedUser = userCache.get(userId);
if (cachedUser) {
return cachedUser;
}
const { user } = await getUser(userId);
cachedUser.set(userId, user);
return user;
});
};
// ...
const array = [1, 2, 3];
const mappedArray = await asyncMap<typeof array, MappedElement>(
array,
async (element) => {
// ...
}
);
// ^^^ callback will expect Promise<MappedElement> return type, and mappedArray will be of type MappedElement[]
Same behaviour as Array.flatMap, but supports an async callback: all async callbacks are executed in parallel.
If one callback throws an error, the whole map function throws as well.
import { asyncFlatMap } from 'array-async-utils';
import { getUsersByGroup } from 'apis/user';
const yourFunction = async () => {
const userGroups = ['groupA', 'groupB', 'groupC'];
const users = await asyncFlatMap(userIds, async (userId) => {
const { groupUsers } = await getUsersByGroup(userId);
return groupUsers;
});
};
// ...
const array = [1, 2, 3];
const mappedArray = await asyncFlatMap<typeof array, MappedElement>(
array,
async (element) => {
// ...
}
);
// ^^^ callback will expect Promise<MappedElement | MappedElement[]> return type, and mappedArray will be of type MappedElement[]
Same behaviour as Array.filter, but supports an async predicate: all async predicates are executed in parallel.
If one callback throws an error, the whole filter function throws as well.
IMPORTANT: unfortunately typescript doesn't support type guards with async function, so if you need to narrow down the type of the filtered array, please use the generics as described below
import { asyncFilter } from 'array-async-utils';
import { getUserStatus } from 'apis/user';
const yourFunction = async () => {
const userIds = ['asyncFilter', '59ef0d84', '6b6cafba'];
const users = await asyncFilter(userIds, async (userId) => {
const { isOnline } = await getUserStatus(userId);
return isOnline;
});
};
// ...
const array = [1, 2, 3, undefined];
const filteredArray = await asyncFilter<typeof array, number>(
array,
async (element) => {
// ...
}
);
// ^^^ filteredArray will be of type number[]
Same behaviour as Array.reduce, but supports an async callback. Async callbacks are executed in series: each one is executed when the previous is finished.
If one callback throws an error, the whole map function throws as well.
import { asyncReduce } from 'array-async-utils';
import { getArticleStats } from 'apis/user';
const yourFunction = async () => {
const articleIds = ['92f9e7a1', '59ef0d84', '6b6cafba'];
const totalInteractions = await asyncReduce(
articleIds,
async (accumulator, articleId) => {
const { articleInteractions } = getArticleStats(articleId);
return accumulator + articleInteractions;
},
0
);
};
// ...
const array = [1, 2, 3];
const reducedValue = await asyncReduce<typeof array, Record<string, number>>(
array,
async (accumulator, element) => {
// ...
},
{}
);
// ^^^ callback will expect Promise<Record<string, number>> return type;
// initialValue, accumulator and reducedValue will be of type Record<string, number>.
FAQs
Array map, flatMap, filter, reduce, etc. with async callback and predicate
The npm package array-async-utils receives a total of 1 weekly downloads. As such, array-async-utils popularity was classified as not popular.
We found that array-async-utils demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.