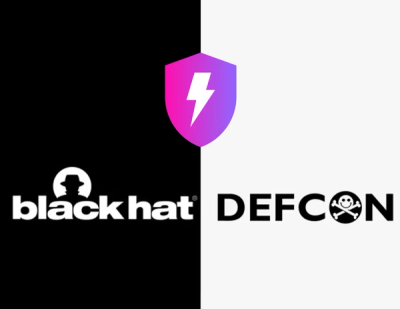
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
async-arrays
Advanced tools
This used to be an array-oriented flow control library. While it still is, async does it more completely.
Now it's just something to use when I want something more lightweight. YMMV
I find, most of the time, my asynchronous logic emerges from an array and I really just want to be able to control the completion of some job, and have a signal for all jobs. In many instances, this winds up being more versatile than a promise which limits you to a binary state and only groups returns according to it's state.
you can either retain an instance and use it that way:
import * as arrays from 'async-arrays';
// OR: const arrays = require('async-arrays');
arrays.forEach(array, iterator, calback);
arrays.forEach
: execute serially
arrays.forEach(array, function(item, index, done){
somethingAsynchronous(function(){
done();
});
}, function(){
//we're all done!
});
arrays.forAll
: execute all jobs in parallel
arrays.forAll(array, function(item, index, done){
somethingAsynchronous(function(){
done();
});
}, function(){
//we're all done!
});
arrays.forEachBatch
: execute all jobs in parallel up to a maximum #, then queue
arrays.forEachBatch(array, batchSize, function(item, index, done){
somethingAsynchronous(function(){
done();
});
}, function(){
//we're all done!
});
arrays.map
: map all elements of the array, but allow for asynchronous interaction. Alternatives are: arrays.map.each
(sequential) arrays.map.all
(parallel)
arrays.map(array, function(item, index, done){
somethingAsynchronous(function(newItem){
done(newItem);
});
}, function(mappedData){
//we're all done!
});
Attach to the prototype (using names which don't collide with the browser implementations):
require('async-arrays').proto();
forEachEmission
: execute serially
[].forEachEmission(function(item, index, done){
somethingAsynchronous(function(){
done();
});
}, function(){
//we're all done!
});
forAllEmissions
: execute all jobs in parallel
[].forAllEmissions(function(item, index, done){
somethingAsynchronous(function(){
done();
});
}, function(){
//we're all done!
});
forAllEmissionsInPool
: execute all jobs in parallel up to a maximum #, then queue for later
[].forAllEmissionsInPool(poolSize, function(item, index, done){
somethingAsynchronous(function(){
done();
});
}, function(){
//we're all done!
});
mapEmissions
: map all elements of the array, but allow for asynchronous interaction
[].mapEmissions(function(item, index, done){
somethingAsynchronous(function(newItem){
done(newItem);
});
}, function(mappedData){
//we're all done!
});
###Utility functions non mutating
['dog', 'cat', 'mouse'].contains('cat') //returns true;
['dog', 'cat'].combine(['mouse']) //returns ['dog', 'cat', 'mouse'];
mutators
['dog', 'cat', 'mouse'].erase('cat') //mutates the array to ['dog', 'mouse'];
['dog', 'cat', 'mouse'].empty('cat') //mutates the array to [];
That's just about it, and even better you can open up the source and check it out yourself. Super simple.
just run
mocha
Enjoy,
-Abbey Hawk Sparrow
FAQs
Async control for arrays
The npm package async-arrays receives a total of 11,595 weekly downloads. As such, async-arrays popularity was classified as popular.
We found that async-arrays demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.