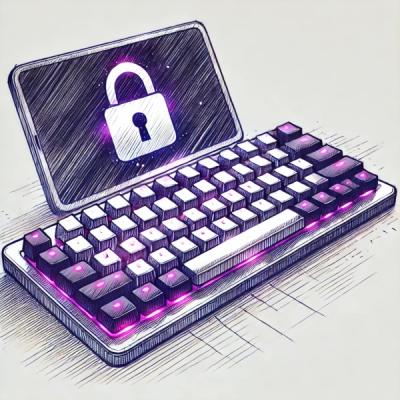
Research
Security News
Malicious npm Package Typosquats react-login-page to Deploy Keylogger
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
async-foreach
Advanced tools
Package description
The async-foreach package provides a way to asynchronously iterate over arrays in JavaScript, allowing for asynchronous operations within each iteration to complete before moving on to the next. It offers a simple API to work with, making it easier to handle asynchronous control flow without resorting to deeply nested callbacks.
Asynchronous forEach
This feature allows you to asynchronously process items in an array. The provided code sample demonstrates how to use async-foreach to log each item in an array with a delay, ensuring each asynchronous operation completes before moving to the next.
var forEach = require('async-foreach').forEach;
var items = [1, 2, 3, 4, 5];
forEach(items, function(item, index, arr) {
setTimeout(() => {
console.log('Item: ' + item);
}, item * 1000);
}, function() {
console.log('All items have been processed.');
});
The 'async' package offers a wide range of powerful utilities for working with asynchronous JavaScript, including methods for collections, control flow, and utilities. It's more comprehensive than async-foreach, providing more flexibility and options for handling asynchronous operations.
Bluebird is a fully featured promise library with focus on innovative features and performance. It includes utilities for converting callback-based APIs to promises, allowing for cleaner async code. While it doesn't provide a direct forEach equivalent, it offers `.map` and `.each` for iterating over collections asynchronously, which can serve similar purposes with promise-based control flow.
Readme
An optionally-asynchronous forEach with an interesting interface.
This code should work just fine in Node.js:
First, install the module with: npm install async-foreach
var forEach = require('async-foreach').forEach;
forEach(["a", "b", "c"], function(item, index, arr) {
console.log("each", item, index, arr);
});
// logs:
// each a 0 ["a", "b", "c"]
// each b 1 ["a", "b", "c"]
// each c 2 ["a", "b", "c"]
Or in the browser:
<script src="dist/ba-foreach.min.js"></script>
<script>
forEach(["a", "b", "c"], function(item, index, arr) {
console.log("each", item, index, arr);
});
// logs:
// each a 0 ["a", "b", "c"]
// each b 1 ["a", "b", "c"]
// each c 2 ["a", "b", "c"]
</script>
In the browser, you can attach the forEach method to any object.
<script>
this.exports = Bocoup.utils;
</script>
<script src="dist/ba-foreach.min.js"></script>
<script>
Bocoup.utils.forEach(["a", "b", "c"], function(item, index, arr) {
console.log("each", item, index, arr);
});
// logs:
// each a 0 ["a", "b", "c"]
// each b 1 ["a", "b", "c"]
// each c 2 ["a", "b", "c"]
</script>
The idea is to allow the callback to decide at runtime whether the loop will be synchronous or asynchronous. By using this
in a creative way (in situations where that value isn't already spoken for), an entire control API can be offered without over-complicating function signatures.
forEach(arr, function(item, index) {
// Synchronous.
});
forEach(arr, function(item, index) {
// Only when `this.async` is called does iteration becomes asynchronous. The
// loop won't be continued until the `done` function is executed.
var done = this.async();
// Continue in one second.
setTimeout(done, 1000);
});
forEach(arr, function(item, index) {
// Break out of synchronous iteration early by returning false.
return index !== 1;
});
forEach(arr, function(item, index) {
// Break out of asynchronous iteration early...
var done = this.async();
// ...by passing false to the done function.
setTimeout(function() {
done(index !== 1);
});
});
See the unit tests for more examples.
// Generic "done" callback.
function allDone(notAborted, arr) {
console.log("done", notAborted, arr);
}
// Synchronous.
forEach(["a", "b", "c"], function(item, index, arr) {
console.log("each", item, index, arr);
}, allDone);
// logs:
// each a 0 ["a", "b", "c"]
// each b 1 ["a", "b", "c"]
// each c 2 ["a", "b", "c"]
// done true ["a", "b", "c"]
// Synchronous with early abort.
forEach(["a", "b", "c"], function(item, index, arr) {
console.log("each", item, index, arr);
if (item === "b") { return false; }
}, allDone);
// logs:
// each a 0 ["a", "b", "c"]
// each b 1 ["a", "b", "c"]
// done false ["a", "b", "c"]
// Asynchronous.
forEach(["a", "b", "c"], function(item, index, arr) {
console.log("each", item, index, arr);
var done = this.async();
setTimeout(function() {
done();
}, 500);
}, allDone);
// logs:
// each a 0 ["a", "b", "c"]
// each b 1 ["a", "b", "c"]
// each c 2 ["a", "b", "c"]
// done true ["a", "b", "c"]
// Asynchronous with early abort.
forEach(["a", "b", "c"], function(item, index, arr) {
console.log("each", item, index, arr);
var done = this.async();
setTimeout(function() {
done(item !== "b");
}, 500);
}, allDone);
// logs:
// each a 0 ["a", "b", "c"]
// each b 1 ["a", "b", "c"]
// done false ["a", "b", "c"]
// Not actually asynchronous.
forEach(["a", "b", "c"], function(item, index, arr) {
console.log("each", item, index, arr);
var done = this.async()
done();
}, allDone);
// logs:
// each a 0 ["a", "b", "c"]
// each b 1 ["a", "b", "c"]
// each c 2 ["a", "b", "c"]
// done true ["a", "b", "c"]
// Not actually asynchronous with early abort.
forEach(["a", "b", "c"], function(item, index, arr) {
console.log("each", item, index, arr);
var done = this.async();
done(item !== "b");
}, allDone);
// logs:
// each a 0 ["a", "b", "c"]
// each b 1 ["a", "b", "c"]
// done false ["a", "b", "c"]
In lieu of a formal styleguide, take care to maintain the existing coding style. Add unit tests for any new or changed functionality. Lint and test your code using grunt.
Also, please don't edit files in the "dist" subdirectory as they are generated via grunt. You'll find source code in the "lib" subdirectory!
04/29/2013 v0.1.3 Removed hard Node.js version dependency.
11/17/2011 v0.1.2 Adding sparse array support. Invalid length properties are now sanitized. This closes issue #1 (like a boss).
11/11/2011 v0.1.1 Refactored code to be much simpler. Yay for unit tests!
11/11/2011 v0.1.0 Initial Release.
Copyright (c) 2012 "Cowboy" Ben Alman
Licensed under the MIT license.
http://benalman.com/about/license/
FAQs
Unknown package
The npm package async-foreach receives a total of 1,190,452 weekly downloads. As such, async-foreach popularity was classified as popular.
We found that async-foreach demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
Security News
The JavaScript community has launched the e18e initiative to improve ecosystem performance by cleaning up dependency trees, speeding up critical parts of the ecosystem, and documenting lighter alternatives to established tools.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.