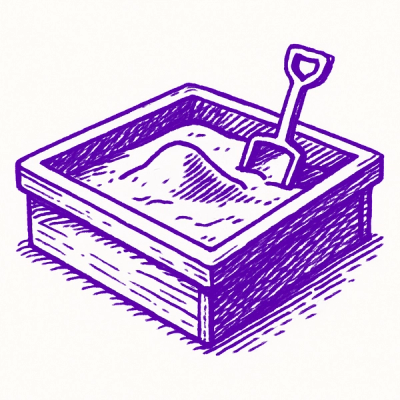
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Allow perform every, filter, find, findIndex, forEach, map, reduce, reduceRight and some on array using Async callback
Purpose of this package is to provide async/await
callbacks for every
, filter
, find
, findIndex
, forEach
, map
, reduce
, reduceRight
and some
methods in Array.
const { AsyncRay } = require('async-ray');
async function dummy(element, needle) {
return Promise.resolve(element > needle);
}
const inputArray = [10, 20, 30, 40];
// Call Every method
const output = await AsyncRay(inputArray).aEvery(
async (i, index, collection) => {
// Dummy async function
return await dummy(i, 5);
}
);
console.log(output);
// Output is true
async function dummy(element, needle) {
return Promise.resolve(element > needle);
}
const inputArray = [1, 2, 3, 4];
// Call Filter method
const filterArray = await AsyncRay(inputArray).aFilter(
async (i, index, collection) => {
// Dummy async function
return await dummy(i, 2);
}
);
console.log(filterArray);
// Output is [3, 4]
Find will return the found value or undefined
async function dummy(element, needle) {
return Promise.resolve(element === needle);
}
const inputArray = [1, 2, 3, 4];
// Call Find method
const outputElement = await AsyncRay(inputArray).aFind(
async (i, index, collection) => {
return await dummy(i, 2);
}
);
console.log('Output is ', outputElement);
// Output is 2
FindIndex will return the index of found value or -1
async function dummy(element, needle) {
return Promise.resolve(element === needle);
}
const inputArray = [1, 2, 3, 4];
// Call Find method
const outputIndex = await AsyncRay(inputArray).aFindIndex(
async (i, index, collection) => {
return await dummy(i, 2);
}
);
console.log('Output is ', outputIndex);
// Output is 1
async function dummy(element) {
return Promise.resolve([element, element * 2]);
}
const inputArray = [1, 2, 3, 4];
// Call Map method
const flatMappedArray = await AsyncRay(inputArray).aFlatMap(
async (i, index, collection) => {
// Dummy async function
return await dummy(i);
}
);
console.log(flatMappedArray);
// Output is [1, 2, 2, 4, 3, 6, 4, 8]
async function dummy(element) {
return Promise.resolve(element);
}
const inputArray = [1, 2, 3, 4];
const outputArray = [];
// Call ForEach method
await AsyncRay(inputArray).aForEach(async (i, index, collection) => {
outputArray.push(await dummy(i));
});
console.log('Output is ', outputArray);
// Output is [1, 2, 3, 4]
async function dummy(element) {
return Promise.resolve(element);
}
const inputArray = [1, 2, 3, 4];
// Call Map method
const mappedArray = await AsyncRay(inputArray).aMap(
async (i, index, collection) => {
// Dummy async function
return await dummy(i);
}
);
console.log(mappedArray);
// Output is [1, 2, 3, 4]
async function dummy(element) {
return Promise.resolve(element);
}
const inputArray = [10, 20, 30, 40];
// Call Reduce method
const output = await AsyncRay(inputArray).aReduce(
async (acc, i, index, collection) => {
return acc + (await dummy(i));
},
1
);
console.log('Output is ', output);
// Output is 101
async function dummy(element) {
return Promise.resolve(element);
}
const inputArray = [10, 20, 30, 40];
// Call Reduce method
const output = await AsyncRay(inputArray).aReduceRight(
async (acc, i, index, collection) => {
return acc + (await dummy(i));
},
1
);
console.log('Output is ', output);
// Output is 101
async function dummy(element, needle) {
return Promise.resolve(element > needle);
}
const inputArray = [10, 20, 30, 40];
// Call Some method
const output = await AsyncRay(inputArray).aSome(
async (i, index, collection) => {
// Dummy async function
return await dummy(i, 30);
}
);
console.log(output);
// Output is true
You can use each method without creating AsyncRay
object.
import {
aEvery, aFilter, aFind, aFindIndex,
aForEach, aMap, aReduce, aReduceRight, aSome
} from 'async-ray';
// aEvery
const everyResult = await aEvery(
[1, 2, 3],
async (e) => Promise.resolve(e > 0)
);
// aFilter
const filterResult = await aFilter(
[1, 2, 3],
async (e) => Promise.resolve(e > 1)
);
// aFind
const findResult = await aFind(
[1, 2, 3],
async (e) => Promise.resolve(e === 3)
);
// aFindIndex
const findIndexResult = await aFindIndex(
[1, 2, 3],
async (e) => Promise.resolve(e === 2)
);
// aForEach
const forEachResult: number[] = [];
await aForEach(
[1, 2, 3],
async (e) => {
const op = await Promise.resolve(e * 10);
forEachResult.push(op);
}
);
// aMap
const mapResult = await aMap(
[1, 2, 3],
async (e) => Promise.resolve(e * 10)
);
// aReduce
const reduceResult = await aReduce(
[1, 2, 3],
async (acc, e) => Promise.resolve(e + acc),
0
);
// aReduceRight
const reduceRightResult = await aReduceRight(
[1, 2, 3],
async (acc, e) => Promise.resolve(e + acc),
0
);
// aSome
const someResult = await aSome(
[1, 2, 3],
async (e) => Promise.resolve(e > 1)
);
Chain
functionalityconst { Chain } = require('async-ray');
Chaining will return an instance of Async-Ray if returned type is an array.
aMap()
and aFilter()
The process()
method must be called explicitly to process the chain because aMap()
and aFilter()
method returns an array.
const input = [1, 2, 3];
const op = await Chain(input)
.aMap(
async (e) => Promise.resolve(e * 10)
)
.aFilter(
async (e) => Promise.resolve(e > 10)
)
.aMap(
async (e) => Promise.resolve(e * 10)
)
// Call the process() method to execute the chain
.process();
console.log('Output is ', op);
// Output is [ 200, 300 ]
aMap()
, aFilter()
and aFind()
The process()
method should not be called because aFind()
does not return an array.
const input = [1, 2, 3];
const op = await Chain(input)
.aMap(
async (e) => Promise.resolve(e * 10)
)
.aFilter(
async (e) => Promise.resolve(e > 10)
)
.aMap(
async (e) => Promise.resolve(e * 10)
)
.aFind(
async (e) => Promise.resolve(e === 300)
);
// No need to call process() method
console.log('Output is ', op);
// Output is 300
Chain
with filter()
const input = [1, 2, 3];
const op = (
await Chain(input)
.aMap(
async (e) => Promise.resolve(e * 10)
)
.aFilter(
async (e) => Promise.resolve(e > 10)
)
.aMap(
async (e) => Promise.resolve(e * 10)
)
.process()
)
.filter(e => e > 200)
console.log('Output is ', op);
// Output is [ 300 ]
Chain
with find()
const input = [1, 2, 3];
const op = (
await Chain(input)
.aMap(
async (e) => Promise.resolve(e * 10)
)
.aFilter(
async (e) => Promise.resolve(e > 10)
)
.aMap(
async (e) => Promise.resolve(e * 10)
)
.process()
)
.find(e => e === 200)
console.log('Output is ', op);
// Output is 200
FAQs
Allow perform every, filter, find, findIndex, forEach, map, reduce, reduceRight and some on array using Async callback
The npm package async-ray receives a total of 790 weekly downloads. As such, async-ray popularity was classified as not popular.
We found that async-ray demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.