async-task
Advanced tools
Comparing version
{ | ||
"name": "async-task", | ||
"version": "0.3.22", | ||
"description": "Run a AsyncTask using a web worker.", | ||
"version": "0.3.42", | ||
"description": "Run a AsyncTask using a web worker or child_process.", | ||
"main": "index.js", | ||
@@ -10,3 +10,3 @@ "directories": { | ||
"scripts": { | ||
"test": "./node_modules/karma/bin/karma start karma.conf.js --single-run true" | ||
"test": "echo '--- RUNNING BROWSER TESTS----: \n' && ./node_modules/karma/bin/karma start karma.conf.js --single-run true && echo '\n-----RUNNING NODE TESTS----:\n' && node_modules/mocha/bin/mocha test/specs/AsyncTask.js" | ||
}, | ||
@@ -18,3 +18,3 @@ "repository": { | ||
"author": "", | ||
"license": "NONE", | ||
"license": "MIT", | ||
"bugs": { | ||
@@ -25,7 +25,9 @@ "url": "https://github.com/gorillatron/async-task/issues" | ||
"dependencies": { | ||
"background-worker": "0.0.2", | ||
"background-worker": "^0.1.0", | ||
"bluebird": "^2.3.11", | ||
"underscore": "^1.7.0" | ||
"uuid": "^2.0.1" | ||
}, | ||
"devDependencies": { | ||
"detect-node": "^2.0.3", | ||
"expect.js": "^0.3.1", | ||
"karma": "^0.12.25", | ||
@@ -32,0 +34,0 @@ "karma-browserify": "^1.0.0", |
AsyncTask | ||
========= | ||
Execute tasks on web Workers without seperate files. | ||
Execute tasks asynchronous tasks without seperate files. In browsers without ```Worker``` support it fallbacks to ```iframe```. | ||
Inn Nodejs it spawns a process using ```child_process```. | ||
### Install | ||
``` | ||
npm install async-task | ||
``` | ||
### Usage | ||
```javascript | ||
var AsyncTask = require( 'async-task' ).AsyncTask | ||
var AsyncTask = require( 'async-task' ) | ||
var task = new AsyncTask({ | ||
doInBackground: function( a, b ) { | ||
return a + b | ||
} | ||
doInBackground: (a, b) -> a + b | ||
}) | ||
task.execute(1, 2, function( result ){ | ||
result === 3 | ||
}) | ||
task.execute(1, 2) | ||
.then(function( result ) { | ||
result === 3 | ||
}) | ||
.catch( handleException ) | ||
``` | ||
Or using promises | ||
### API | ||
#### AsyncTask( options ) | ||
Creates a new AsyncTask | ||
##### options | ||
* ```options.doInBackground``` The work(function) to be done in the worker. | ||
* ```options.keepAlive``` Keep worker alive so ```.execute``` can be called multiple times. | ||
* ```options.worker``` Supply worker if you want to share worker between tasks. **NB!: termination of worker is left to the user** | ||
#### asyncTask.execute( args... ):bluebird/Promise | ||
Execute the ```doInBackground``` function with supplied args. | ||
###### Sharing worker example | ||
```javascript | ||
var AsyncTask = require( 'async-task' ).AsyncTask, | ||
PromiseInterface = require( 'async-task' ).PromiseInterface | ||
var AsyncTask = require( 'async-task' ) | ||
var BackgroundWorker = require( 'background-worker' ) | ||
AsyncTask.defaults.asyncInterface = PromiseInterface | ||
var worker = new BackgroundWorker({}) | ||
var task = new AsyncTask({ | ||
doInBackground: function( a, b ) { | ||
return a + b | ||
} | ||
var taskA = new AsyncTask({ | ||
worker: worker, | ||
doInBackground: () -> 'a' | ||
}) | ||
task | ||
.execute(1, 2) | ||
.then(function( result ) { | ||
result === 3 | ||
}) | ||
.error( handleError ) | ||
.catch( handleException) | ||
var taskB = new AsyncTask({ | ||
worker: worker, | ||
doInBackground: () -> 'b' | ||
}) | ||
Promise.all([ | ||
taskA.execute(), | ||
taskB.execute() | ||
]).then(function(result) { | ||
result == [ 'a', 'b' ] | ||
worker.terminate() | ||
}) | ||
``` | ||
#### Test | ||
```npm run-script test``` | ||
### Roadmap | ||
* ```doInBackground``` can return a promise or maybe even a ```generator*``` so you can iterate over ```asyncTask.execute``` | ||
*Partially made, with <3 at:* | ||
[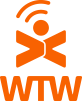](https://github.com/wtw-software/) |
@@ -1,7 +0,4 @@ | ||
var _ = require( 'underscore' ), | ||
inherits = require( 'util' ) .inherits, | ||
EventEmitter = require( 'events' ).EventEmitter, | ||
BackgroundWorker = require( 'background-worker' ) | ||
var BackgroundWorker = require( 'background-worker' ) | ||
var uuid = require( 'uuid' ) | ||
module.exports = AsyncTask | ||
@@ -11,12 +8,12 @@ | ||
* @class AsyncTask | ||
* @extends EventEmitter | ||
* @author Jørn Andre Tangen @gorillatron | ||
*/ | ||
function AsyncTask( options ) { | ||
EventEmitter.apply( this, arguments ) | ||
options = typeof options != 'undefined' ? options : {} | ||
options = typeof options != 'undefined' ? options : {} | ||
this.__uuid = uuid.v4() | ||
this.__hasExecuted = false | ||
this.__keepAlive = options.keepAlive | ||
this.__boundArguments = [] | ||
this.__sharingworker = false | ||
@@ -26,30 +23,27 @@ this.doInBackground = options.doInBackground ? options.doInBackground : null | ||
if( options.worker ) { | ||
this.__sharingworker = true | ||
this.setWorker( options.worker ) | ||
} | ||
} | ||
inherits( AsyncTask, EventEmitter ) | ||
/* | ||
* Default options for new AsyncTasks | ||
* @static | ||
* Setup a background-worker/BackgroundWorker | ||
* @public | ||
* @object | ||
* @property {object} defaults - The default values for new AsyncTask's. | ||
* @property {AsyncInterface} defaults.asyncInterfaceImplementation - The interface implementation to use for all new AsyncTasks | ||
* @function | ||
*/ | ||
AsyncTask.defaults = {} | ||
AsyncTask.prototype.setWorker = function( worker ) { | ||
this._worker = worker | ||
this._worker.importScripts = this.importScripts | ||
this._worker.define( this.__uuid + '::doInBackground', this.doInBackground.toString() ) | ||
return this._worker | ||
} | ||
/* | ||
* Identity object for unset values | ||
* @public | ||
* @function | ||
* Array slice | ||
* @private | ||
* @function | ||
* @returns Array | ||
*/ | ||
AsyncTask.prototype.setupWorker = function() { | ||
if( !this.worker ) { | ||
this.worker = new BackgroundWorker({}) | ||
this.worker.importScripts = this.importScripts | ||
this.worker.define( 'doInBackground', this.doInBackground.toString() ) | ||
this.worker.start() | ||
} | ||
return this.worker | ||
} | ||
var slice = Array.prototype.slice | ||
@@ -60,6 +54,6 @@ /* | ||
* @function | ||
* @returns {AsyncInterface} | ||
* @returns {bluebird/Promise} | ||
*/ | ||
AsyncTask.prototype.execute = function() { | ||
var args, taskPromise | ||
var worker, args, taskPromise | ||
@@ -70,16 +64,17 @@ if( this.__hasExecuted && !this.__keepAlive ) { | ||
if( !this._worker ) { | ||
this.setWorker( new BackgroundWorker({}) ) | ||
} | ||
this.__hasExecuted = true | ||
this.emit( 'execute' ) | ||
worker = this._worker | ||
args = slice.call( arguments ) | ||
taskPromise = worker.run( this.__uuid + '::doInBackground', args ) | ||
this.setupWorker() | ||
if( !this.__keepAlive && !this.__sharingworker ) { | ||
taskPromise.finally(function() { worker.terminate() }) | ||
} | ||
args = Array.prototype.slice.call( arguments ) | ||
taskPromise = this.worker.run( 'doInBackground', args ) | ||
if( !this.__keepAlive ) | ||
taskPromise.finally( _.bind(this.worker.terminate, this.worker) ) | ||
return taskPromise | ||
} |
var AsyncTask = require( '../../index' ) | ||
var BackgroundWorker = require( 'background-worker' ) | ||
var Promise = require( 'bluebird' ) | ||
var expect = require( 'expect.js' ) | ||
var isNode = require( 'detect-node' ) | ||
describe( 'AsyncTask', function() { | ||
@@ -21,2 +26,41 @@ | ||
it('should throw error if executed for the second time', function() { | ||
var asyncTask = new AsyncTask({ | ||
doInBackground: function( a, b ) { | ||
return a + b | ||
} | ||
}) | ||
var exception | ||
try { | ||
asyncTask.execute(3,3) | ||
asyncTask.execute(3,3) | ||
} | ||
catch( e ) { | ||
exception = e | ||
} | ||
expect( exception ).to.be.an( Error ) | ||
}) | ||
it('should be able to execute multipe times if option.keepAlive', function( done ) { | ||
var asyncTask = new AsyncTask({ | ||
keepAlive: true, | ||
doInBackground: function( a, b ) { | ||
return a + b | ||
} | ||
}) | ||
Promise.all([ | ||
asyncTask.execute(1,1), | ||
asyncTask.execute(2,2), | ||
asyncTask.execute(3,3) | ||
]).then(function(results) { | ||
expect(results).to.eql([2,4,6]) | ||
done() | ||
}) | ||
}) | ||
it('should give error to callback on errors', function( done ) { | ||
@@ -35,14 +79,50 @@ var asyncTask = new AsyncTask({ | ||
it('should import scripts', function( done ) { | ||
var asyncTask = new AsyncTask({ | ||
importScripts: [location.protocol + "//" + location.host + "/base/test/assets/import.js"], | ||
doInBackground: function() { | ||
return importedFunc() | ||
} | ||
if( !isNode ) { | ||
it('should import scripts', function( done ) { | ||
var asyncTask = new AsyncTask({ | ||
importScripts: [location.protocol + "//" + location.host + "/base/test/assets/import.js"], | ||
doInBackground: function() { | ||
return importedFunc() | ||
} | ||
}) | ||
asyncTask.execute(null).then(function( result ) { | ||
expect( result ).to.equal( 'imported' ) | ||
done() | ||
}) | ||
}) | ||
} | ||
asyncTask.execute(null).then(function( result ) { | ||
expect( result ).to.equal( 'imported' ) | ||
}) | ||
describe('Sharing background-worker', function() { | ||
it('should work gdmit', function( done ) { | ||
var worker = new BackgroundWorker({}) | ||
var taskA = new AsyncTask({ | ||
worker: worker, | ||
doInBackground: function() {return "a" } | ||
}) | ||
var taskB = new AsyncTask({ | ||
worker: worker, | ||
doInBackground: function() {return "b" } | ||
}) | ||
var taskC = new AsyncTask({ | ||
worker: worker, | ||
doInBackground: function() {return "c" } | ||
}) | ||
Promise.all([ | ||
taskA.execute(), | ||
taskB.execute(), | ||
taskC.execute() | ||
]).then(function(result) { | ||
expect( result ).to.eql([ 'a', 'b', 'c' ]) | ||
worker.terminate() | ||
done() | ||
}) | ||
}) | ||
@@ -52,3 +132,2 @@ | ||
}) |
Explicitly Unlicensed Item
License(Experimental) Something was found which is explicitly marked as unlicensed.
Found 1 instance in 1 package
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
Misc. License Issues
License(Experimental) A package's licensing information has fine-grained problems.
Found 1 instance in 1 package
9665
40.03%0
-100%0
-100%100
Infinity%221
38.99%85
102.38%0
-100%8
33.33%+ Added
+ Added
+ Added
+ Added
- Removed
- Removed
- Removed
Updated