Comparing version 0.0.6 to 0.0.8
557
asynk.js
@@ -1,2 +0,3 @@ | ||
var _ = require('underscore'); | ||
var utils = require('./lib/utils'); | ||
var Deferred = require('./lib/deferred'); | ||
@@ -17,5 +18,4 @@ /********************************************************************************/ | ||
var Task = function(parentStack, id, fct) { | ||
var self = this; | ||
this.parentStack = parentStack; | ||
var Task = function (context, id, fct) { | ||
this.context = context; | ||
this.id = id; | ||
@@ -29,7 +29,7 @@ this.alias = null; | ||
Task.prototype.setCallback = function(callback) { | ||
Task.prototype.setCallback = function (callback) { | ||
this.callback = callback; | ||
}; | ||
Task.prototype.execute = function() { | ||
Task.prototype.execute = function () { | ||
var self = this; | ||
@@ -42,13 +42,17 @@ if (!this.checkDependencies()) { | ||
this.args.forEach(function(arg, index) { | ||
var resolved = []; | ||
this.args.forEach(function (arg, index) { | ||
if (arg instanceof DefArg) { | ||
self.args[index] = arg.resolve(self); | ||
resolved[index] = arg.resolve(self); | ||
} | ||
else { | ||
resolved[index] = arg; | ||
} | ||
}); | ||
if (this.status === RUNNING) { | ||
this.fct.apply(null, this.args); | ||
this.fct.apply(null, resolved); | ||
} | ||
}; | ||
Task.prototype.fail = function(err) { | ||
Task.prototype.fail = function (err) { | ||
this.status = FAIL; | ||
@@ -58,11 +62,24 @@ this.fct.apply(null, [err, null]); | ||
Task.prototype.checkDependencies = function() { | ||
for (key in this.dependencies) { | ||
Task.prototype.checkDependencies = function () { | ||
for (var key in this.dependencies) { | ||
var id = this.dependencies[key]; | ||
if (_.isString(id)) { | ||
id = this.parentStack.aliasMap[id]; | ||
if (utils.isString(id)) { | ||
var alias = this.context.aliasMap[id]; | ||
if (utils.isUndefined(alias)) { | ||
throw new Error('Unknown dependancy alias: ' + id); | ||
} | ||
if (utils.isArray(alias)) { | ||
for (var i in alias) { | ||
var taskid = alias[i]; | ||
if (this.context.tasks[taskid].status !== DONE) { | ||
return false; | ||
} | ||
} | ||
} | ||
else if (this.context.tasks[alias].status !== DONE) { | ||
return false; | ||
} | ||
} | ||
if (_.isUndefined(this.parentStack.results[id])) { | ||
else if (this.context.tasks[id].status !== DONE) { | ||
return false; | ||
@@ -78,3 +95,3 @@ } | ||
var DefArg = function(type, val) { | ||
var DefArg = function (type, val) { | ||
this.type = type; | ||
@@ -84,7 +101,7 @@ this.value = val; | ||
DefArg.prototype.resolve = function(task) { | ||
DefArg.prototype.resolve = function (task) { | ||
switch (this.type) { | ||
case 'order': | ||
if (this.value >= 0) { | ||
if (_.isUndefined(task.parentStack.results[this.value])) { | ||
if (task.context.tasks[this.value].status !== DONE) { | ||
task.status = WAITING_FOR_DEPENDENCY; | ||
@@ -95,7 +112,7 @@ task.dependencies.push(this.value); | ||
else { | ||
return task.parentStack.results[this.value]; | ||
return task.context.results[this.value]; | ||
} | ||
} | ||
else if (this.value < 0) { | ||
if (_.isUndefined(task.parentStack.results[task.id + this.value])) { | ||
if (task.context.tasks[task.id + this.value].status !== DONE) { | ||
task.status = WAITING_FOR_DEPENDENCY; | ||
@@ -106,3 +123,3 @@ task.dependencies.push(task.id + this.value); | ||
else { | ||
return task.parentStack.results[task.id + this.value]; | ||
return task.context.results[task.id + this.value]; | ||
} | ||
@@ -114,6 +131,6 @@ } | ||
if (this.value === 'all') { | ||
return task.parentStack.results; | ||
return task.context.results; | ||
} | ||
else { | ||
return task.parentStack.results[task.parentStack.aliasMap[this.value]]; | ||
return task.context.results[task.context.aliasMap[this.value]]; | ||
} | ||
@@ -135,9 +152,9 @@ break; | ||
/********************************************************************************/ | ||
var Progressive = function(start, step) { | ||
var Progressive = function (start, step) { | ||
this.step = step || 1; | ||
this.last = (_.isUndefined(start) ? 1 : start) - this.step; | ||
this.last = (utils.isUndefined(start) ? 1 : start) - this.step; | ||
this.stack = []; | ||
}; | ||
Progressive.prototype.push = function(order, fct) { | ||
Progressive.prototype.push = function (order, fct) { | ||
var self = this; | ||
@@ -147,11 +164,11 @@ var task = {order: order, fct: fct}; | ||
return function(){ | ||
task.args = _.toArray(arguments); | ||
return function () { | ||
task.args = utils.toArray(arguments); | ||
var reCheck = true; | ||
while (reCheck) { | ||
reCheck = false; | ||
for(i in self.stack){ | ||
for (var i in self.stack) { | ||
var queued = self.stack[i]; | ||
if (queued.order === (self.last + self.step)) { | ||
if (!_.isUndefined(queued.args)) { | ||
if (!utils.isUndefined(queued.args)) { | ||
queued.fct.apply(null, queued.args); | ||
@@ -175,6 +192,6 @@ self.stack.slice(i, 1); | ||
/********************************************************************************/ | ||
var Fifo = function() { | ||
var Fifo = function () { | ||
this.stack = []; | ||
}; | ||
Fifo.prototype.push = function(fct) { | ||
Fifo.prototype.push = function (fct) { | ||
var self = this; | ||
@@ -184,6 +201,6 @@ var task = {}; | ||
this.stack.push(task); | ||
return function() { | ||
task.args = _.toArray(arguments); | ||
return function () { | ||
task.args = utils.toArray(arguments); | ||
if (self.stack[0] === task) { | ||
while (self.stack.length && !_.isUndefined(self.stack[0].args)) { | ||
while (self.stack.length && !utils.isUndefined(self.stack[0].args)) { | ||
var callback = self.stack.shift(); | ||
@@ -200,221 +217,307 @@ callback.fct.apply(null, callback.args); | ||
var Asynk = function() { | ||
var self = this; | ||
this.tasks = []; | ||
this.aliasMap = []; | ||
this.results = []; | ||
this.currentTasks = []; | ||
}; | ||
var Asynk = function () { | ||
Asynk.prototype.add = function(fct) { | ||
if ( _.isUndefined(fct) || !_.isFunction(fct) ) { | ||
throw new Error('Asynk add require a function as argument'); | ||
} | ||
var newId = this.tasks.length; | ||
this.tasks[newId] = new Task(this, newId, fct); | ||
this.currentTasks = [newId]; | ||
return this; | ||
}; | ||
var after = { | ||
loop: function (condition) { | ||
if (!utils.isFunction(condition)) { | ||
throw new Error('Asynk loop function require a function as first argument'); | ||
} | ||
_Context.loopDefer = _Context.loopDefer || new Deferred(); | ||
_Context.loopResults = _Context.loopResults || []; | ||
this.done(function () { | ||
var result = utils.toArray(arguments); | ||
if (result.length > 1) { | ||
Asynk.prototype.each = function(datas, fct) { | ||
if ( _.isUndefined(fct) || !_.isFunction(fct) ) { | ||
throw new Error('Asynk each require a function as second argument'); | ||
} | ||
var self = this; | ||
self.currentTasks = []; | ||
datas.forEach(function(data) { | ||
var newId = self.tasks.length; | ||
self.tasks[newId] = new Task(self, newId, fct); | ||
self.tasks[newId].item = data; | ||
self.currentTasks.push(newId); | ||
}); | ||
return this; | ||
}; | ||
_Context.loopResults.push(result); | ||
} | ||
else { | ||
_Context.loopResults.push(result[0]); | ||
} | ||
Asynk.prototype.args = function() { | ||
args = _.toArray(arguments); | ||
var self = this; | ||
this.currentTasks.forEach(function(currentTask) { | ||
self.tasks[currentTask].args = _.clone(args); | ||
}); | ||
return this; | ||
}; | ||
if (condition.apply(null, result)) { | ||
//reset values | ||
for (var i in _Context.tasks) { | ||
_Context.tasks[i].status = WAITING; | ||
} | ||
_Context.results = []; | ||
_Context.currentTasks = []; | ||
_Context.exec.fct.apply(null, _Context.exec.args).loop(condition); | ||
} | ||
else { | ||
_Context.loopDefer.resolve(_Context.loopResults); | ||
} | ||
}); | ||
this.fail(_Context.loopDefer.reject); | ||
return _Context.loopDefer.promise(); | ||
}, | ||
asyncLoop: function (condition) { | ||
if (!utils.isFunction(condition)) { | ||
throw new Error('Asynk loop function require a function as first argument'); | ||
} | ||
_Context.loopDefer = _Context.loopDefer || new Deferred(); | ||
_Context.loopResults = _Context.loopResults || []; | ||
this.done(function () { | ||
var result; | ||
if (arguments.length > 1) { | ||
result = utils.toArray(arguments); | ||
} | ||
else { | ||
result = arguments[0]; | ||
} | ||
_Context.loopResults.push(result); | ||
condition.apply(null, [result, function (err, loop) { | ||
if (err) { | ||
return _Context.loopDefer.reject(err); | ||
} | ||
if (loop) { | ||
//reset values | ||
for (var i in _Context.tasks) { | ||
_Context.tasks[i].status = WAITING; | ||
} | ||
_Context.results = []; | ||
_Context.currentTasks = []; | ||
_Context.exec.fct.apply(null, _Context.exec.args).asyncLoop(condition); | ||
} | ||
else { | ||
_Context.loopDefer.resolve(_Context.loopResults); | ||
} | ||
}]); | ||
}); | ||
this.fail(_Context.loopDefer.reject); | ||
return _Context.loopDefer.promise(); | ||
} | ||
}; | ||
Asynk.prototype.require = function(dependency) { | ||
var self = this; | ||
this.currentTasks.forEach(function(currentTask) { | ||
var current = self.tasks[currentTask]; | ||
current.dependencies.push(dependency); | ||
}); | ||
return this; | ||
}; | ||
var Asynk = {}; | ||
Asynk.prototype.alias = function(alias) { | ||
if (_.isString(alias)) { | ||
var self = this; | ||
this.currentTasks.forEach(function(currentTask, index) { | ||
if (self.currentTasks.length > 1) { | ||
alias += index; | ||
} | ||
self.tasks[currentTask].alias = alias; | ||
self.aliasMap[alias] = currentTask; | ||
}); | ||
} | ||
return this; | ||
}; | ||
var _Context = { | ||
tasks: [], | ||
aliasMap: {}, | ||
results: [], | ||
currentTasks: Asynk.currentTasks | ||
}; | ||
Asynk.prototype.serie = function(endcall, endcallArgs) { | ||
if ( _.isUndefined(endcall) || !_.isFunction(endcall) ) { | ||
throw new Error('Asynk serie require a function as first argument'); | ||
} | ||
var self = this; | ||
var endTask = new Task(this, 'end', endcall); | ||
endTask.args = endcallArgs || this.results; | ||
var cb = function(err, data) { | ||
if (!err) { | ||
self.results.push(data); | ||
self.next(); | ||
Asynk.add = function (fct) { | ||
if (utils.isUndefined(fct) || !utils.isFunction(fct)) { | ||
throw new Error('Asynk add require a function as argument'); | ||
} | ||
else { | ||
endTask.fail(err); | ||
var newId = _Context.tasks.length; | ||
_Context.tasks[newId] = new Task(_Context, newId, fct); | ||
_Context.currentTasks = [newId]; | ||
this.args(new DefArg('callback')); | ||
return this; | ||
}; | ||
Asynk.each = function (datas, fct) { | ||
if (utils.isUndefined(fct) || !utils.isFunction(fct)) { | ||
throw new Error('Asynk each require a function as second argument'); | ||
} | ||
_Context.currentTasks = []; | ||
datas.forEach(function (data) { | ||
var newId = _Context.tasks.length; | ||
_Context.tasks[newId] = new Task(_Context, newId, fct); | ||
_Context.tasks[newId].item = data; | ||
_Context.currentTasks.push(newId); | ||
}); | ||
this.args(new DefArg('item'), new DefArg('callback')); | ||
return this; | ||
}; | ||
this.next = function() { | ||
var current = self.tasks.shift(); | ||
if (current) { | ||
current.setCallback(cb); | ||
current.execute(); | ||
Asynk.args = function () { | ||
args = utils.toArray(arguments); | ||
_Context.currentTasks.forEach(function (currentTask) { | ||
_Context.tasks[currentTask].args = utils.toArray(args); | ||
}); | ||
return this; | ||
}; | ||
Asynk.require = function (dependency) { | ||
_Context.currentTasks.forEach(function (currentTask) { | ||
var current = _Context.tasks[currentTask]; | ||
current.dependencies.push(dependency); | ||
}); | ||
return this; | ||
}; | ||
Asynk.alias = function (alias) { | ||
if (utils.isString(alias)) { | ||
_Context.currentTasks.forEach(function (currentTask, index) { | ||
_Context.tasks[currentTask].alias = alias; | ||
}); | ||
if (_Context.currentTasks.length > 1) { | ||
_Context.aliasMap[alias] = _Context.currentTasks; | ||
} | ||
else { | ||
_Context.aliasMap[alias] = _Context.currentTasks[0]; | ||
} | ||
} | ||
else { | ||
endTask.execute(); | ||
} | ||
return this; | ||
}; | ||
this.next(); | ||
return this; | ||
}; | ||
Asynk.prototype.parallel = function(endcall, endcallArgs) { | ||
if ( _.isUndefined(endcall) || !_.isFunction(endcall) ) { | ||
throw new Error('Asynk parallel require a function as first argument'); | ||
} | ||
var self = this; | ||
var endTask = new Task(this, 'end', endcall); | ||
endTask.args = endcallArgs || this.results; | ||
Asynk.serie = function (endcallArgs) { | ||
_Context.exec = { | ||
fct: Asynk.serie, | ||
args: [endcallArgs] | ||
}; | ||
var defer = new Deferred(); | ||
var endTask = new Task(_Context, 'end', defer.resolve.bind(defer)); | ||
endTask.args = endcallArgs || [new DefArg('alias', 'all')]; | ||
var currentId = 0; | ||
var count = 0; | ||
var todo = self.tasks.length; | ||
var cb = function(task, err, data) { | ||
task.status = DONE; | ||
if (!err) { | ||
self.results[task.id] = data; | ||
count++; | ||
if (count >= todo) { | ||
var cb = function (err, data) { | ||
if (!err) { | ||
_Context.tasks[currentId].status = DONE; | ||
_Context.results.push(data); | ||
currentId++; | ||
next(); | ||
} | ||
else { | ||
_Context.tasks[currentId].status = FAIL; | ||
defer.fail(err); | ||
endTask.fail(err); | ||
} | ||
}; | ||
var next = function () { | ||
var current = _Context.tasks[currentId]; | ||
if (current) { | ||
current.setCallback(cb); | ||
current.execute(); | ||
if (current.status === WAITING_FOR_DEPENDENCY) { | ||
defer.reject(new Error('could not resolve this dependancies')); | ||
} | ||
} | ||
else { | ||
endTask.execute(); | ||
} | ||
}; | ||
next(); | ||
return defer.promise(after); | ||
}; | ||
Asynk.parallel = function (endcallArgs) { | ||
_Context.exec = { | ||
fct: Asynk.parallel, | ||
args: [endcallArgs] | ||
}; | ||
var defer = new Deferred(); | ||
var endTask = new Task(_Context, 'end', defer.resolve.bind(defer)); | ||
endTask.args = endcallArgs || [new DefArg('alias', 'all')]; | ||
var count = 0; | ||
var todo = _Context.tasks.length; | ||
var cb = function (task, err, data) { | ||
task.status = DONE; | ||
if (!err) { | ||
_Context.results[task.id] = data; | ||
count++; | ||
if (count >= todo) { | ||
endTask.execute(); | ||
} | ||
else { | ||
_Context.tasks.forEach(function (task) { | ||
if (task.status === WAITING_FOR_DEPENDENCY) { | ||
task.execute(); | ||
} | ||
}); | ||
} | ||
} | ||
else { | ||
self.tasks.forEach(function(task) { | ||
if (task.status === WAITING_FOR_DEPENDENCY) { | ||
task.execute(); | ||
} | ||
}); | ||
defer.fail(err); | ||
endTask.fail(err); | ||
} | ||
}; | ||
if (_Context.tasks.length === 0) { | ||
endTask.execute(); | ||
return defer.promise(); | ||
} | ||
else { | ||
endTask.fail(err); | ||
} | ||
}; | ||
if (this.tasks.length === 0){ | ||
endTask.execute(); | ||
return this; | ||
} | ||
this.tasks.forEach(function(task) { | ||
task.setCallback(function(err, data) { | ||
cb(task, err, data); | ||
_Context.tasks.forEach(function (task) { | ||
task.setCallback(function (err, data) { | ||
cb(task, err, data); | ||
}); | ||
}); | ||
}); | ||
this.tasks.forEach(function(task) { | ||
task.execute(); | ||
}); | ||
_Context.tasks.forEach(function (task) { | ||
task.execute(); | ||
}); | ||
return this; | ||
}; | ||
return defer.promise(after); | ||
}; | ||
Asynk.prototype.parallelLimited = function(limit, endcall, endcallArgs) { | ||
if ( _.isUndefined(endcall) || !_.isFunction(endcall) ) { | ||
throw new Error('Asynk parallelLimited require a function as second argument'); | ||
} | ||
var self = this; | ||
var endTask = new Task(this, 'end', endcall); | ||
endTask.args = endcallArgs || this.results; | ||
Asynk.parallelLimited = function (limit, endcallArgs) { | ||
_Context.exec = { | ||
fct: Asynk.parallelLimited, | ||
args: [limit, endcallArgs] | ||
}; | ||
var defer = new Deferred(); | ||
var endTask = new Task(_Context, 'end', defer.resolve.bind(defer)); | ||
endTask.args = endcallArgs || [new DefArg('alias', 'all')]; | ||
var count = 0; | ||
var todo = self.tasks.length; | ||
var cb = function(task, err, data) { | ||
task.status = DONE; | ||
if (!err) { | ||
self.results[task.id] = data; | ||
count++; | ||
if (count >= todo) { | ||
endTask.execute(); | ||
var count = 0; | ||
var todo = _Context.tasks.length; | ||
var cb = function (task, err, data) { | ||
task.status = DONE; | ||
if (!err) { | ||
_Context.results[task.id] = data; | ||
count++; | ||
if (count >= todo) { | ||
endTask.execute(); | ||
} | ||
else { | ||
_Context.tasks.forEach(function (task) { | ||
var stats = utils.countBy(_Context.tasks, function (task) { | ||
return task.status; | ||
}); | ||
var running = stats[RUNNING] || 0; | ||
if ((running < limit) && (task.status < RUNNING)) { | ||
task.execute(); | ||
} | ||
}); | ||
} | ||
} | ||
else { | ||
self.tasks.forEach(function(task) { | ||
var stats = _.countBy(self.tasks, function(task) { | ||
return task.status; | ||
}); | ||
var running = stats[RUNNING] || 0; | ||
if ((running < limit) && (task.status < RUNNING)) { | ||
task.execute(); | ||
} | ||
}); | ||
defer.fail(err); | ||
endTask.fail(err); | ||
} | ||
}; | ||
if (_Context.tasks.length === 0) { | ||
endTask.execute(); | ||
return defer.promise(); | ||
} | ||
else { | ||
endTask.fail(err); | ||
} | ||
}; | ||
if (this.tasks.length === 0){ | ||
endTask.execute(); | ||
return this; | ||
} | ||
this.tasks.forEach(function(task) { | ||
task.setCallback(function(err, data) { | ||
cb(task, err, data); | ||
_Context.tasks.forEach(function (task) { | ||
task.setCallback(function (err, data) { | ||
cb(task, err, data); | ||
}); | ||
}); | ||
}); | ||
this.tasks.forEach(function(task) { | ||
var stats = _.countBy(self.tasks, function(task) { | ||
return task.status; | ||
_Context.tasks.forEach(function (task) { | ||
var stats = utils.countBy(_Context.tasks, function (task) { | ||
return task.status; | ||
}); | ||
var running = stats[RUNNING] || 0; | ||
if ((running < limit) && (task.status < RUNNING)) { | ||
task.execute(); | ||
} | ||
}); | ||
var running = stats[RUNNING] || 0; | ||
if ((running < limit) && (task.status < RUNNING)) { | ||
task.execute(); | ||
} | ||
}); | ||
return this; | ||
return defer.promise(after); | ||
}; | ||
return Asynk; | ||
}; | ||
/* | ||
Asynk.prototype.getTask = function(id){ | ||
if (_.isString(id)) { | ||
if (_.) { | ||
id = this.aliasMap[id]; | ||
} | ||
} | ||
return this.tasks[id]; | ||
}*/ | ||
module.exports = { | ||
add: function(fct) { return new Asynk().add(fct); }, | ||
each: function(datas, fct) { return new Asynk().each(datas, fct); }, | ||
add: function (fct) { | ||
return Asynk().add(fct); | ||
}, | ||
each: function (datas, fct) { | ||
return Asynk().each(datas, fct); | ||
}, | ||
callback: new DefArg('callback'), | ||
data: function(val) { | ||
if (_.isString(val)) { | ||
data: function (val) { | ||
if (utils.isString(val)) { | ||
return new DefArg('alias', val); | ||
@@ -427,6 +530,12 @@ } | ||
item: new DefArg('item'), | ||
fifo: function(){ return new Fifo(); }, | ||
progressive: function(start,step){ return new Progressive(start,step); } | ||
}; | ||
fifo: function () { | ||
return new Fifo(); | ||
}, | ||
progressive: function (start, step) { | ||
return new Progressive(start, step); | ||
}, | ||
deferred: function () { | ||
return new Deferred(); | ||
}, | ||
when: new Deferred().when | ||
}; |
{ | ||
"name": "asynk", | ||
"version": "0.0.6", | ||
"description": "flow control library for javascript", | ||
"version": "0.0.8", | ||
"description": "Asynk is a feature-rich JavaScript library designed to simplify common asynchronous JavaScript programming tasks.", | ||
"main": "asynk.js", | ||
@@ -16,3 +16,6 @@ "scripts": { | ||
"asynk", | ||
"async" | ||
"async", | ||
"deferred", | ||
"callback", | ||
"promise" | ||
], | ||
@@ -22,6 +25,5 @@ "author": "Alexandre Tiertant", | ||
"dependencies": { | ||
"underscore": ">=1.7.0" | ||
}, | ||
"devDependencies": { | ||
"colors": ">=1.0.3" | ||
"mocha": ">=2.2.5" | ||
}, | ||
@@ -28,0 +30,0 @@ "bugs": { |
225
README.md
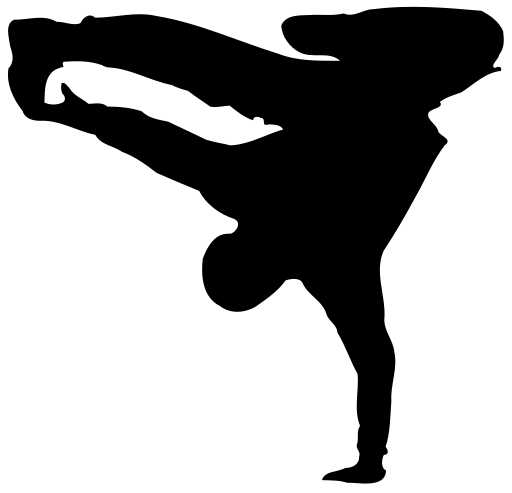Asynk.js | ||
-------- | ||
Asynk is a small tool for javascript asynchronous task management. | ||
Asynk is a feature-rich JavaScript library designed to simplify common asynchronous JavaScript programming tasks. | ||
@@ -14,7 +14,7 @@ | ||
.add(fs.readFile).args('./TEST.txt', "utf8",asynk.callback).alias('content') | ||
.serie(console.log,[asynk.data('content')]); | ||
.serie([asynk.data('content')]).done(console.log); | ||
//Log 'hello world' in console | ||
``` | ||
Asynk adapt to fit your functions syntax without writting a warper function and can dynimaquely get results from a task output back to an other input and more... | ||
Asynk adapt to fit your functions syntax without writting a warper function and can dynamically get results from a task output back to an other input and more... | ||
see full documentation below. | ||
@@ -30,2 +30,6 @@ | ||
## License | ||
MIT | ||
## Adding Task | ||
@@ -41,3 +45,3 @@ | ||
arguments: | ||
* fct: an asynchronous function | ||
* fct: an asynchronous function (default arguments value is [asynk.callback]) | ||
@@ -57,3 +61,3 @@ ```javascript | ||
* array_data: an array of data | ||
* fct: an asynchronous function | ||
* fct: an asynchronous function (default arguments value is [asynk.item,asynk.callback]) | ||
@@ -153,8 +157,9 @@ ```javascript | ||
### Serie | ||
*serie(fct,args)* | ||
*serie(args)* | ||
arguments: | ||
* fct: a function called once serie is finnished | ||
* args: an array of arguments to pass to fct | ||
* args: an array of arguments to pass to fct ( default is asynk.data('all') ) | ||
return: a promise | ||
in this mode,all task are execute one by one in the order they where inserted. | ||
@@ -164,3 +169,3 @@ | ||
asynk.each(['one','two'],my_function).args(asynk.item,asynk.callback) | ||
.serie(console.log,[asynk.data('all')]); | ||
.serie().done(console.log); | ||
``` | ||
@@ -172,8 +177,9 @@ | ||
### Parallel | ||
*parallel(fct,args)* | ||
*parallel(args)* | ||
arguments: | ||
* fct: a function called once parallel is finnished | ||
* args: an array of arguments to pass to fct | ||
* args: an array of arguments that are passed to doneCallbacks ( default is asynk.data('all') ) | ||
return: a promise | ||
in this mode,all task are started at the same time. | ||
@@ -183,3 +189,3 @@ | ||
asynk.each(['one','two'],my_function).args(asynk.item,asynk.callback) | ||
.parallel(console.log,[asynk.data('all')]); | ||
.parallel().done(console.log); | ||
``` | ||
@@ -191,9 +197,10 @@ | ||
### ParallelLimited | ||
*parallelLimited(limit,fct,args)* | ||
*parallelLimited(limit,args)* | ||
arguments: | ||
* limit: an integer number of maximum parallel task to execute | ||
* fct: a function called once parallelLimited is finnished | ||
* args: an array of arguments to pass to fct | ||
* args: an array of arguments to pass to fct ( default is asynk.data('all') ) | ||
return: a promise | ||
in this mode,a predefined number(limit) of task are running in the same time. | ||
@@ -203,3 +210,3 @@ | ||
asynk.each([0,0,0,0],my_function).args(asynk.item,asynk.callback) | ||
.parallelLimited(2,console.log,[asynk.data('all')]); | ||
.parallelLimited(2).done(console.log); | ||
``` | ||
@@ -210,2 +217,186 @@ | ||
## Deffered | ||
### Asynk.deffered | ||
*asynk.deffered()* | ||
A factory function that returns a chainable utility object with methods to register multiple callbacks into callback queues, invoke callback queues, and relay the success or failure state of any synchronous or asynchronous function. | ||
return: a deffered object | ||
```javascript | ||
var defered = asynk.deferred(); | ||
var promise = defered.promise(); | ||
promise.done(function(arg){ | ||
console.log('hello ' + arg); | ||
}); | ||
defered.resolve('world'); | ||
``` | ||
### Always | ||
*always(fct)* | ||
Add function to be called when the deferred object is either resolved or rejected. | ||
arguments: | ||
* fct: a function called when deferred object is resolved or rejected. | ||
return: the deffered/promise object | ||
### Done | ||
*done(fct)* | ||
Add function to be called when the deferred object is resolved. | ||
arguments: | ||
* fct: a function called when deferred object is resolved. | ||
return: the deffered/promise object | ||
### Fail | ||
*fail(fct)* | ||
Add function to be called when the deferred object is rejected. | ||
arguments: | ||
* fct: a function called when deferred object is rejected. | ||
return: the deffered/promise object | ||
### IsRejected | ||
*isRejected()* | ||
Determine whether a deferred object has been rejected. | ||
return: a boolean | ||
### IsResolved | ||
*isResolved()* | ||
Determine whether a deferred object has been resolved. | ||
return: a boolean | ||
### Notify | ||
*notify(args)* | ||
Call the progressCallbacks on a deferred object with the given args. | ||
arguments: | ||
* args: optional agruments passed to progessCallbacks. | ||
return: the deffered object | ||
### NotifyWith | ||
*notifyWith(context, args)* | ||
Call the progressCallbacks on a deferred object with the given context and args. | ||
arguments: | ||
* context: context passed to progressCallbacks as the `this` object. | ||
* args: optional array of agruments passed to progessCallbacks. | ||
return: the deffered object | ||
### Pipe | ||
*pipe(doneFilter)* | ||
Utility method to filter and/or chain deferreds. | ||
arguments: | ||
*doneFilter: A function called as doneCallback that return is passed to pipe returned promise doneCallbacks as arguments. | ||
return: a promise object | ||
### Progress | ||
*progress(fct)* | ||
Add function to be called when the deferred object generates progress notifications. | ||
arguments: | ||
* fct: a function called when deferred object notify function is called. | ||
return: the deffered/promise object | ||
### Promise | ||
*promise(obj)* | ||
Return a deferred’s Promise object. | ||
arguments: | ||
* obj: object that receive promise's functions. | ||
return: a promise object | ||
### Reject | ||
*reject(args)* | ||
Reject a deferred object and call any failCallbacks with the given args. | ||
arguments: | ||
* args: arguments that are passed to the failCallbacks. | ||
return: the deffered/promise object | ||
### RejectWith | ||
*rejectWith(context, args)* | ||
Reject a deferred object and call any failCallbacks with the given context and args. | ||
arguments: | ||
* context: context passed to failCallbacks as the `this` object. | ||
* args: optional array of agruments passed to failCallbacks. | ||
return: the deffered object | ||
### Resolve | ||
*resolve(args)* | ||
Resolve a deferred object and call any doneCallbacks with the given args. | ||
arguments: | ||
* args: arguments that are passed to the doneCallbacks. | ||
return: the deffered/promise object | ||
### ResolveWith | ||
*resolveWith(context, args)* | ||
Resolve a deferred object and call any doneCallbacks with the given context and args. | ||
arguments: | ||
* context: context passed to doneCallbacks as the `this` object. | ||
* args: optional array of agruments passed to doneCallbacks. | ||
return: the deffered object | ||
### State | ||
*state()* | ||
Determine the current state of a deferred object. | ||
return: a string('pending','resolved' or 'rejected') | ||
### Then | ||
*then(doneFilter, failFilter, progressFilter)* | ||
Add functions to be called when the deferred object is resolved, rejected, or still in progress. | ||
arguments: | ||
* doneFilter: A function called as doneCallback that return is passed to then returned promise doneCallbacks as arguments. | ||
* failFilter: A function called as failCallback that return is passed to then returned promise doneCallbacks as arguments. | ||
* progressFilter: A function called as progressCallback that return is passed to then returned promise progressCallbacks as arguments. | ||
return: a promise object | ||
### When | ||
*when(deferreds)* | ||
Provides a way to execute callbacks functions based on one or more deferred objects that represent asynchronous events. | ||
arguments: | ||
* deferreds: a list of deferred or plain javascript objects. | ||
return: a promise object | ||
## Stacks | ||
@@ -212,0 +403,0 @@ |
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
Filesystem access
Supply chain riskAccesses the file system, and could potentially read sensitive data.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
67098
0
15
1439
450
1
1
- Removedunderscore@>=1.7.0
- Removedunderscore@1.13.7(transitive)