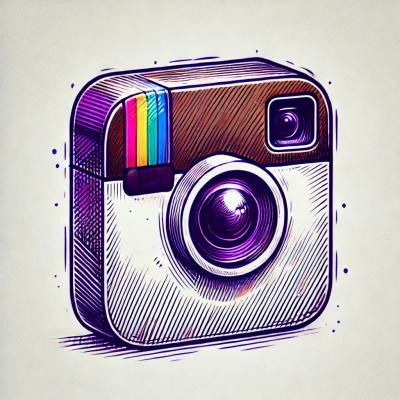
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
babel-code-frame
Advanced tools
Generate errors that contain a code frame that point to source locations.
The babel-code-frame package is a utility that allows developers to generate a string representing a code frame that highlights a specific portion of the code, typically used to point out syntax errors or other code issues. It can be used to enhance error messages with a visual context of where the issue is located in the source code.
Highlighting code
This feature allows you to highlight a specific part of the code. In the provided code sample, it highlights the word 'Foo' in the given rawLines string.
const { codeFrameColumns } = require('babel-code-frame');
const rawLines = `class Foo {\n constructor()\n}`;
const location = { start: { line: 1, column: 16 }, end: { line: 1, column: 19 } };
const result = codeFrameColumns(rawLines, location, { highlightCode: true });
console.log(result);
Chalk is a popular npm package used for styling terminal strings. While it doesn't generate code frames, it can be used in conjunction with other tools to highlight specific parts of the text in the terminal, similar to how babel-code-frame highlights code.
ansi-styles is a package that provides ANSI escape codes for styling strings in the terminal. Like chalk, it can be used to style and highlight text but does not provide code frame generation.
Cardinal is a syntax highlighter for JavaScript code that can be used in the terminal. It provides functionality similar to babel-code-frame's highlighting feature but is focused on syntax highlighting rather than pinpointing specific code locations.
Generate errors that contain a code frame that point to source locations.
npm install --save-dev babel-code-frame
import codeFrame from 'babel-code-frame';
const rawLines = `class Foo {
constructor()
}`;
const lineNumber = 2;
const colNumber = 16;
const result = codeFrame(rawLines, lineNumber, colNumber, { /* options */ });
console.log(result);
1 | class Foo {
> 2 | constructor()
| ^
3 | }
If the column number is not known, you may pass null
instead.
highlightCode
boolean
, defaults to false
.
Toggles syntax highlighting the code as JavaScript for terminals.
linesAbove
number
, defaults to 2
.
Adjust the number of lines to show above the error.
linesBelow
number
, defaults to 3
.
Adjust the number of lines to show below the error.
forceColor
boolean
, defaults to false
.
Enable this to forcibly syntax highlight the code as JavaScript (for non-terminals); overrides highlightCode
.
FAQs
Generate errors that contain a code frame that point to source locations.
The npm package babel-code-frame receives a total of 5,155,270 weekly downloads. As such, babel-code-frame popularity was classified as popular.
We found that babel-code-frame demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.