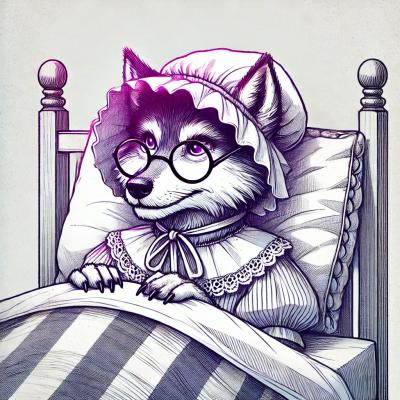
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
babel-plugin-transform-exponentiation-operator
Advanced tools
Compile exponentiation operator to ES5
The babel-plugin-transform-exponentiation-operator package is a Babel plugin that transforms the exponentiation operator (**) in JavaScript into a form that is compatible with older JavaScript environments that do not support this operator natively.
Transform Exponentiation Operator
This feature allows you to use the exponentiation operator (**) in your code, and the plugin will transform it into a call to Math.pow, which is compatible with older JavaScript environments.
const squared = 2 ** 2; // Transformed to Math.pow(2, 2);
This package transforms ES2015 modules to CommonJS. While it does not specifically handle the exponentiation operator, it is similar in that it transforms newer JavaScript syntax into a form that is compatible with older environments.
This package transforms async/await syntax into generator functions. Like babel-plugin-transform-exponentiation-operator, it helps in making modern JavaScript features compatible with older environments.
This package transforms the object rest/spread syntax into a form that is compatible with older JavaScript environments. It is similar in its goal of making modern JavaScript syntax usable in environments that do not support it natively.
Compile exponentiation operator to ES5
// x ** y
let squared = 2 ** 2;
// same as: 2 * 2
let cubed = 2 ** 3;
// same as: 2 * 2 * 2
// x **= y
let a = 2;
a **= 2;
// same as: a = a * a;
let b = 3;
b **= 3;
// same as: b = b * b * b;
npm install --save-dev babel-plugin-transform-exponentiation-operator
.babelrc
(Recommended).babelrc
{
"plugins": ["transform-exponentiation-operator"]
}
babel --plugins transform-exponentiation-operator script.js
require("babel-core").transform("code", {
plugins: ["transform-exponentiation-operator"]
});
FAQs
Compile exponentiation operator to ES5
The npm package babel-plugin-transform-exponentiation-operator receives a total of 0 weekly downloads. As such, babel-plugin-transform-exponentiation-operator popularity was classified as not popular.
We found that babel-plugin-transform-exponentiation-operator demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.