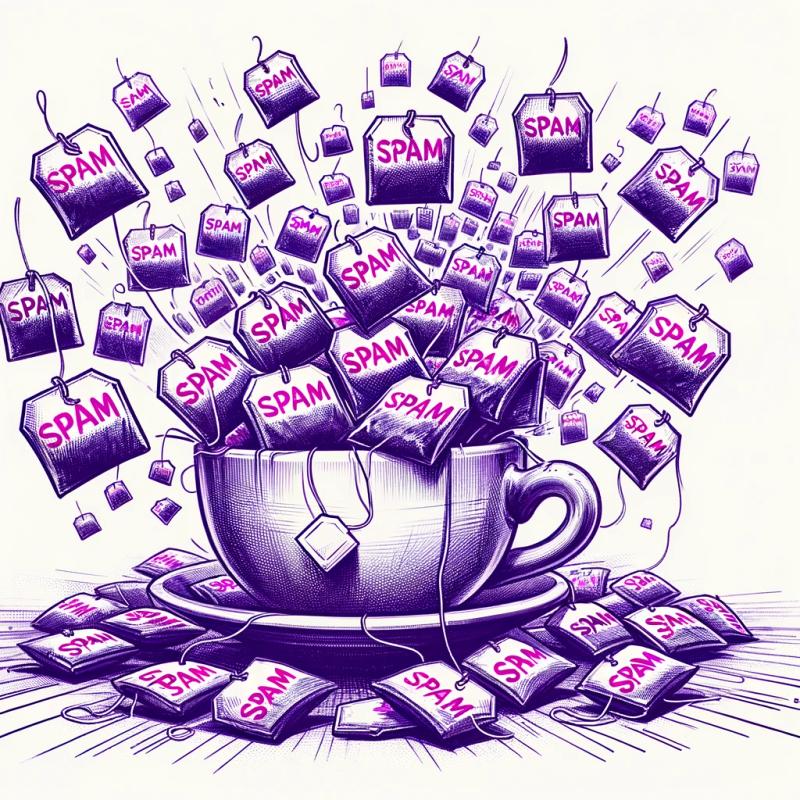
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
babel-preset-react-app
Advanced tools
Readme
This package includes the Babel preset used by Create React App.
Please refer to its documentation:
The easiest way to use this configuration is with Create React App, which includes it by default. You don’t need to install it separately in Create React App projects.
If you want to use this Babel preset in a project not built with Create React App, you can install it with the following steps.
First, install Babel.
Then install babel-preset-react-app.
npm install babel-preset-react-app --save-dev
Then create a file named .babelrc
with following contents in the root folder of your project:
{
"presets": ["react-app"]
}
This preset uses the useBuiltIns
option with transform-object-rest-spread and transform-react-jsx, which assumes that Object.assign
is available or polyfilled.
Make sure you have a .flowconfig
file at the root directory. You can also use the flow
option on .babelrc
:
{
"presets": [["react-app", { "flow": true, "typescript": false }]]
}
Make sure you have a tsconfig.json
file at the root directory. You can also use the typescript
option on .babelrc
:
{
"presets": [["react-app", { "flow": false, "typescript": true }]]
}
Absolute paths are enabled by default for imports. To use relative paths instead, set the absoluteRuntime
option in .babelrc
to false
:
{
"presets": [["react-app", { "absoluteRuntime": false }]]
}
FAQs
Babel preset used by Create React App
The npm package babel-preset-react-app receives a total of 3,120,605 weekly downloads. As such, babel-preset-react-app popularity was classified as popular.
We found that babel-preset-react-app demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.