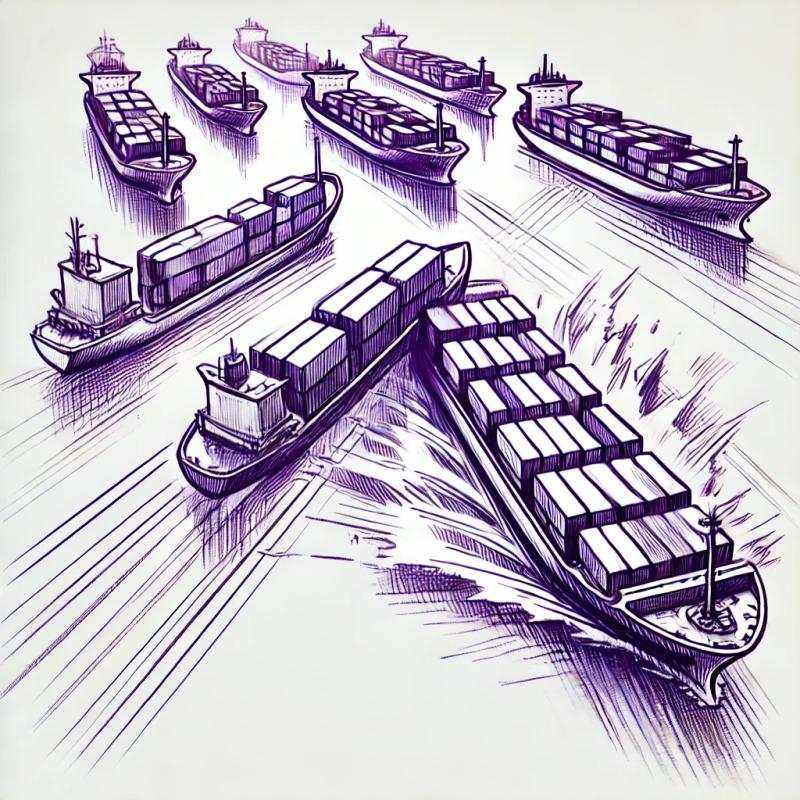
Security News
Namecheap Takes Down Polyfill.io Service Following Supply Chain Attack
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
backbone.nested-types
Advanced tools
Readme
In case you're precisely know what you're looking for, it's backbone.js extension adding type annotations to model attributes, easiest possible way of dealing with nested models and collections, and native properties for attributes. Providing you with a more or less complete, simple, and powerful object system for JavaScript.
In case if you don't, here is a brief outline of problems we're solving with this little thing. There are two major goals behind:
Simplify maping of complex server-side entities to client's models. It should:
parse
, toJSON
, and initialize
. It's not trivial thing to do it right.Simplify usage of models as contexts for template rendering. What we've done for that:
These issues are addressed in many different backbone plugins, but this one is defferent.
We solve these problems encouraging you to type less, than you used to. 'Type specs' in model's 'defaults' section do all the magic. So, if your attribute is a date, just write in defaults, that it's Date. That's it. No, you don't need a compiler, it's still old good vanilla JS you're get used to.
For any model attributes mentioned in 'defaults', use
model.first = model.second;
model.deep.nesting = some.thing.from.another.model;
instead of
model.set( 'first', model.get( 'second' ) );
model.deep.set( 'nesting', some.get( 'thing' ).get( 'from' ).get( 'another' ).get( 'model );
Great for accessing nested models from templates.
Also, you can define calculated native properties for models and collections like this:
var MyModel = Model.extend({
defaults : {
otherModel_id : 0,
yetAnotherModel_id : 2
},
__otherModelsCollection : null,
properties : {
otherModel : function(){
return this.__otherModelsCollection.get( this.otherModel_id );
},
yetAnotherModel : {
get : function(){
return this.__otherModelsCollection.get( this.yetAnotherModel_id );
},
set : function( model ){
this.yetAnotherModel_id = model.id;
}
}
}
});
// This could be done from some other place...
MyModel.prototype.__otherModelsCollection = ...
Great for implementing collection joins which looks like nested models. Also, since custom properties definition override default properties, it's well suitable for setting hooks on attribute's modification. In case you'll need such a weird stuff, of course.
You could use constructor functions as default value.
var User = Model.extend({
defaults : {
name : String,
created : Date,
loginCount: Number
}
});
New object will be created automatically for any typed attribute, no need to override initialize
.
When typed attribute assigned with value of different type, constructor will be invoked to
convert it to defined type.
var user = new User();
assert( user.created instanceof Date );
user.created = "2012-12-12 12:12"; // string will be converted to Date
assert( user.created instanceof Date );
user.loginCount = "jhkhjhjhj";
assert( user.loginCount === NaN );
It means, that you don't need to override Model.parse and Model.initialize when you receive time and date from the server. It will be parsed and serialized to JSON as ISO date automatically.
To have nested models and collections, annotate attribute with Model or Collection type.
var User = Model.extend({
defaults : {
name : String,
created : Date,
group : GroupModel,
permissions : PermissionCollection
}
});
No need to override initialize
, parse
, and toJSON
, everything will be done automatically.
There is a difference from regular types in 'set' behaviour. If attribute's current value is not null, and new value has different type, it will be delegated to 'set' method of nested model or collection. I.e. this code:
var user = new User();
user.group = {
name: "Admin"
};
user.permissions = [{ id: 5, type: 'full' }];
is equivalent of:
user.group.set({
name: "Admin"
};
user.permissions.set( [{ id: 5, type: 'full' }] );
This mechanics of 'set' allows you to work with JSON from in case of deeply nested models and collections without the need to override 'parse'. This code (considering that nested attributes defined as models):
user.group = {
nestedModel : {
deeplyNestedModel : {
attr : 'value'
},
attr : 5
}
};
is almost equivalent of:
user.group.nestedModel.deeplyNestedModel.set( 'attr', 'value' );
user.group.nestedModel.set( 'attr', 'value' );
but it will fire just single change
event.
Change events will be bubbled from nested models and collections.
change
and change:attribute
events for any changes in nested models and collections. Multiple change
events from submodels during bulk updates are carefully joined together, which make it suitable to subscribe View.render to the top model's change
.replace:attribute
event when model or collection is replaced with new object. You might need it to subscribe for events from submodels.model.nestedModel = other.nestedModel.deepClone(); // will create a copy of nested objects tree
var Base = Model.extend({
defaults: {
a : 1
}
});
var Derived = Base.extend({
defaults: {
b : 1
}
});
var instance = new Derived();
assert( instance.b === 1 );
assert( instance.a === 1 );
var myClass = Class.extend({
a : 1,
initialize : function( options ){
this.a = options.a
},
properties : {
b : function(){ return this.a + 1; }
}
});
You need a single file (nestedtypes.js) and backbone.js itself. It should work in browser with plain script tag, require.js or other AMD loader. Also, it's available as npm package for node.js (https://www.npmjs.org/package/backbone.nested-types).
Module exports three variables - Class, Model, Collection. You need to use them instead of backbone's.
FAQs
backbone.js extension adding type annotations to model attributes, easiest possible way of dealing with nested models and collections, and native properties for attributes. Providing you with a more or less complete, simple, and powerful object system for
The npm package backbone.nested-types receives a total of 1 weekly downloads. As such, backbone.nested-types popularity was classified as not popular.
We found that backbone.nested-types demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.
Security News
A JavaScript library maintainer is under fire after merging a controversial PR to support legacy versions of Node.js.