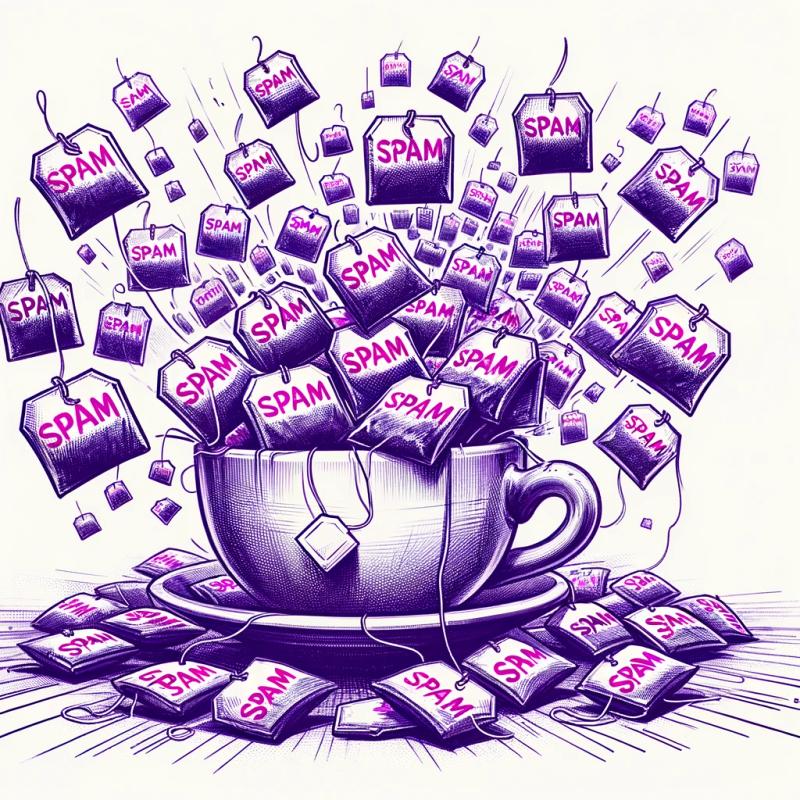
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
backpage
Advanced tools
Readme
Naive static HTML streaming based on React for Node.js CLI applications.
BackPage renders your React application to HTML and streams updates (static HTML snapshots) to your browser.
It is designed for really simple GUI as a complementary to text logs, so advanced user interaction is neither supported nor its goal.
/action/[action-name]
and /notify
endpoints.npm install react backpage
main.tsx
import {BackPage} from 'backpage';
import React from 'react';
import {App} from './app.js';
const page = new BackPage();
page.render(<App />);
// Print page information including URL.
page.guide();
app.tsx
import React, {useState, useEffect} from 'react';
export const App = () => {
const [count, setCount] = useState(0);
useEffect(() => {
const timer = setInterval(
setCount(count => count + 1),
1000,
);
return () => clearInterval(timer);
}, []);
return <div>Count: {count}</div>;
};
See Form and ActionButton for simple usage.
BackPage can proxy explicitly specified events that bubble to document
from the browser to your Node.js React application.
const page = new BackPage({
events: ['click'],
});
page.render(
<div
onClick={() => {
console.info('This will work.');
}}
>
Click me!
</div>,
);
Events are proxied asynchronously, and just for the purpose of triggering actions in your Node.js application.
Not all events bubble, please checkout relevant documents for more information.
Properties:
altKey
ctrlKey
metaKey
shiftKey
Effects:
event.target.value
to the value of the input element.A notification can be sent to the browser using either page.notify()
or /notify
endpoint.
To send notification to the browser using page.notify()
:
page.notify('Hello BackPage!');
page.notify({
title: 'Hello BackPage!',
body: 'This is a notification from BackPage.',
});
You can also setup a fallback for notifications not getting clicked within the timeout:
const page = new BackPage({
notify: {
// timeout: 30_000,
fallback: notification => {
// Handle the notification manually.
// Optionally return a webhook URL or request options to initiate an HTTP
// request.
return 'https://some.webhook/';
},
},
});
page.notify({
title: 'Hello BackPage!',
body: 'Click me or your webhook will get fired!',
});
To send notification to the browser using /notify
endpoint:
await fetch('http://localhost:12368/notify', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
title: 'Hello BackPage!',
body: 'This is a notification from BackPage.',
}),
});
A
timeout
field can also be specified (a number orfalse
) to override the default value.
A simple webhook can be setup with /action/[action-name]
endpoint.
page.registerAction('hello', data => {
console.info('Hello', data);
});
To trigger this action:
await fetch('http://localhost:12368/action/hello', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
name: 'BackPage',
}),
});
This uses the same mechanism as the Form
action, so you may want to avoid using the same action name (if you explicitly specify one for Form
).
By specifying a UUID as token, you can get a public URL from backpage.cloud:
import {BackPage, getPersistentToken} from 'backpage';
const page = new BackPage({
// You can also use any random UUID for temporary page.
token: getPersistentToken(),
// Different pages can be setup using the same token with different names.
// name: 'project-name',
});
page.guide();
You can also create a temporary front only page for web notifications using https://backpage.cloud/new.
Note: backpage.cloud may introduce value-added services for significant network traffic to cover the expense in the future.
Check out src/examples.
A Form
is based on HTML form
element and has similar usage, except that action
is proxied backed by POST
requests and accepts callback with the form data object as its parameter.
const action = data => console.info(data);
page.render(
<Form action={action}>
<input name="name" />
<button type="submit">Submit</button>
</Form>,
);
In many cases, only the button is relevant for an action. ActionButton
wraps a button within a Form
for those cases:
const action = () => console.info('Launch!');
page.render(<ActionButton action={action}>Launch</ActionButton>);
You can also put multiple ActionButton
s in an explicit Form
to share the form inputs:
const actionA = data => console.info('action-a', data);
const actionB = data => console.info('action-b', data);
page.render(
<Form>
<input name="name" />
<ActionButton action={actionA}>Action A</ActionButton>
<ActionButton action={actionB}>Action B</ActionButton>
</Form>,
);
Sets document.title
of the page.
page.render(
<>
<Title>Awesome Page</Title>
<div>Hello BackPage!</div>
</>,
);
You can also specify
title
inBackPage
options if it not dynamic.
Adds a style
element to the page with content loaded from src
(local path).
const App = () => (
<>
<Style src={STYLE_PATH} />
<div>Hello BackPage!</div>
</>
);
You can directly use
<link rel="stylesheet" href="..." />
for CSS links.
Intercepts console outputs using patch-console.
const App = () => (
<>
<h2>Logs</h2>
<Console />
</>
);
MIT License.
FAQs
Naive static HTML streaming based on React for Node.js CLI applications.
The npm package backpage receives a total of 1 weekly downloads. As such, backpage popularity was classified as not popular.
We found that backpage demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.