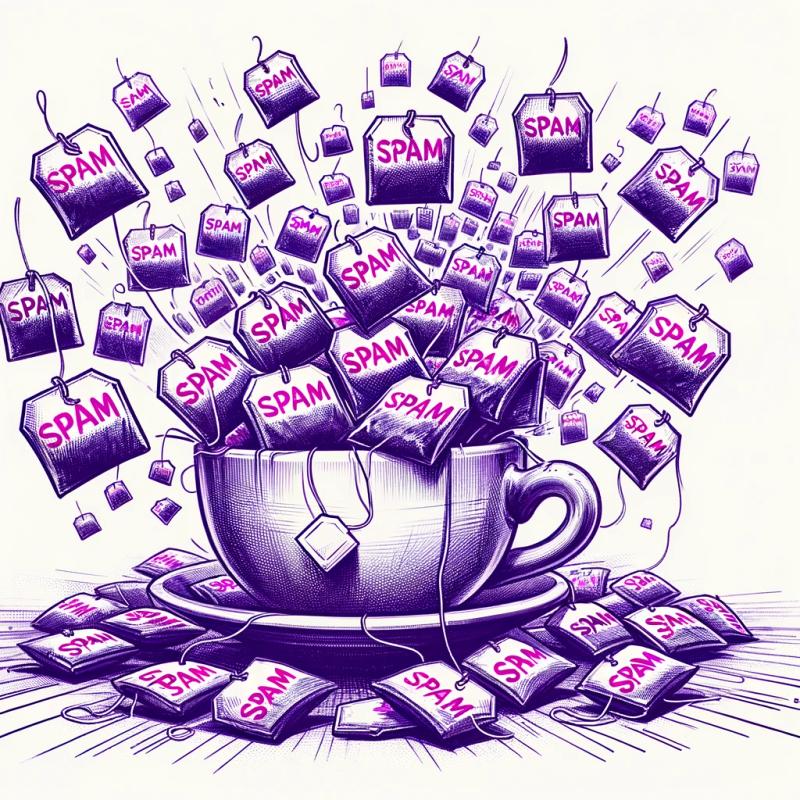
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
basic-auth
Advanced tools
Package description
The basic-auth npm package is a simple module to parse `Authorization` header for basic authentication in Node.js applications. It extracts the user credentials from the header and makes them available for authentication purposes.
Parse Basic Auth Credentials
This code demonstrates how to use basic-auth to parse the `Authorization` header in an Express.js application. It checks if the credentials match a predefined username and password, and either grants access or denies it with a 401 status code.
const auth = require('basic-auth');
const express = require('express');
const app = express();
app.use((req, res) => {
const credentials = auth(req);
if (!credentials || credentials.name !== 'user' || credentials.pass !== 'pass') {
res.statusCode = 401;
res.setHeader('WWW-Authenticate', 'Basic realm="example"');
res.end('Access denied');
} else {
res.end('Access granted');
}
});
app.listen(3000);
Passport-http is a strategy for Passport, an authentication middleware for Node.js. It implements Basic and Digest authentication. Compared to basic-auth, passport-http is more feature-rich, allowing for integration with the broader Passport ecosystem and providing more options for authentication.
Express-basic-auth is an authentication library designed specifically for Express.js applications. It provides a simple way to protect routes with basic authentication. Unlike basic-auth, which only parses the credentials, express-basic-auth handles the full authentication process, making it easier to use but less flexible for non-Express use cases.
Http-auth is a module that provides basic and digest authentication for HTTP and HTTPS protocols. It is similar to basic-auth but offers more features, such as integration with different types of data stores for credential checking and support for digest authentication.
Readme
Generic basic auth Authorization header field parser for whatever.
This is a Node.js module available through the
npm registry. Installation is done using the
npm install
command:
$ npm install basic-auth
var auth = require('basic-auth')
Get the basic auth credentials from the given request. The Authorization
header is parsed and if the header is invalid, undefined
is returned,
otherwise an object with name
and pass
properties.
Parse a basic auth authorization header string. This will return an object
with name
and pass
properties, or undefined
if the string is invalid.
Pass a Node.js request object to the module export. If parsing fails
undefined
is returned, otherwise an object with .name
and .pass
.
var auth = require('basic-auth')
var user = auth(req)
// => { name: 'something', pass: 'whatever' }
A header string from any other location can also be parsed with
auth.parse
, for example a Proxy-Authorization
header:
var auth = require('basic-auth')
var user = auth.parse(req.getHeader('Proxy-Authorization'))
var http = require('http')
var auth = require('basic-auth')
var compare = require('tsscmp')
// Create server
var server = http.createServer(function (req, res) {
var credentials = auth(req)
// Check credentials
// The "check" function will typically be against your user store
if (!credentials || !check(credentials.name, credentials.pass)) {
res.statusCode = 401
res.setHeader('WWW-Authenticate', 'Basic realm="example"')
res.end('Access denied')
} else {
res.end('Access granted')
}
})
// Basic function to validate credentials for example
function check (name, pass) {
var valid = true
// Simple method to prevent short-circut and use timing-safe compare
valid = compare(name, 'john') && valid
valid = compare(pass, 'secret') && valid
return valid
}
// Listen
server.listen(3000)
FAQs
node.js basic auth parser
The npm package basic-auth receives a total of 5,962,186 weekly downloads. As such, basic-auth popularity was classified as popular.
We found that basic-auth demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.