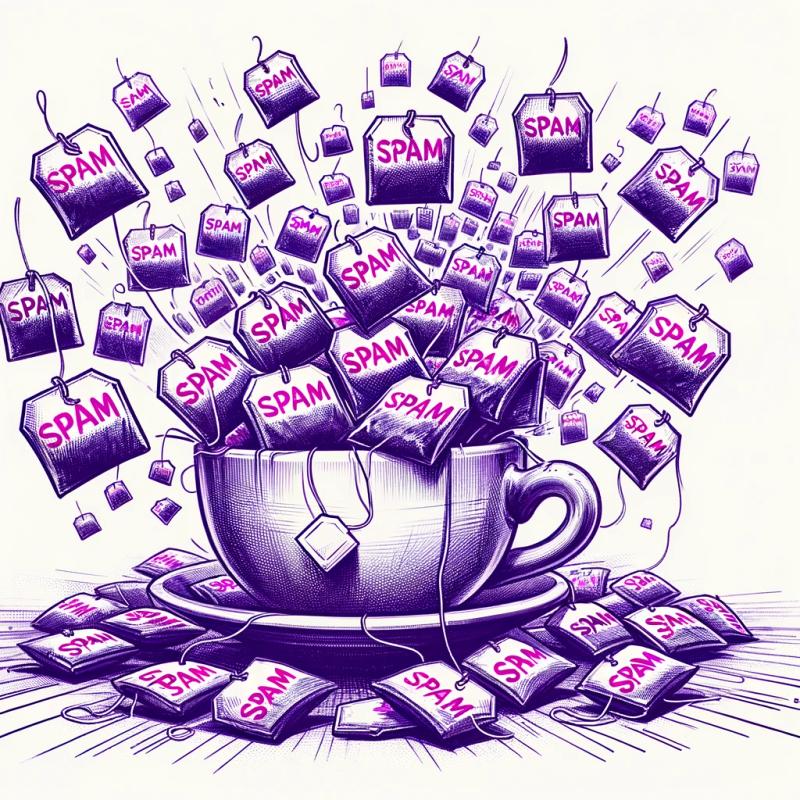
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
basic-influence-roles
Advanced tools
Readme
Detect and measure the basic role of influence each node plays within a directed network.
It supports a raw list of nodes, a Graphology DiGraph, as well as a method to be used in a distributed context for Big Data use cases.
This algorithm returns:
For in-depth theoretical details and more examples, please read the main repository intro.
All useful informations can be found in the following paragraphs:
npm install basic-influence-roles
Import BIRs package
Using import:
import BIRs from 'basic-influence-roles';
Using require:
const BIRs = require('basic-influence-roles');
Methods to detect BIRs.
BIRs.detectFromNodes(nodes=Array[Object]);
Field | Type | Required | Description |
---|---|---|---|
nodes | [{...}] | yes | An array of all nodes' data. |
nodes[i].id | any | yes | The name or id of the node. |
nodes[i].indegree | integer | yes | The number of incoming connections. |
nodes[i].outdegree | integer | yes | The number of outcoming connections. |
// The list of nodes with indegree and outdegree
nodes = [
{id: 1, indegree: 13, outdegree: 5},
{id: 2, indegree: 3, outdegree: 8},
{id: 3, indegree: 0, outdegree: 22},
{id: 4, indegree: 16, outdegree: 19},
{...}
];
// Measure the influence score and detect the basic influence roles
res = BIRs.detectFromNodes(nodes);
Detect roles of a Graphology Directed Graph
BIRs.detectGraphology(G);
Type | Required | Description |
---|---|---|
G | yes | A Graphology directed graph. |
const { DirectedGraph } = require('graphology');
// Create a directed graph
const G = new DirectedGraph({multi: false, allowSelfLoops: false});
// Add some nodes and edges
...
// Detect basic influence roles of nodes
res = BIRs.detectGraphology(G);
In case of Big Data or Huge Networks you can distribute the load in this way:
BIRs.detect(indegree, outdegree, nodeCount, data);
Field | Type | Required | Description |
---|---|---|---|
indegree | integer | yes | The number of incoming connections. |
outdegree | integer | yes | The number of outcoming connections. |
nodeCount | integer | yes | The total number of nodes. |
data | boolean | no | If true returns indegree and outdegree. |
// Get the total count of nodes
nodeCount = 8586987087;
// For every node in a huge network (use here a distributed loop instead)
nodes.map(([indegree, outdegree]) => {
// Get basic influence role of every node in network
return BIRs.detect(indegree, outdegree, nodeCount, true);
});
The output is a list of nodes reporting their id, role, role level, influence measure, influence ranking.
Field | Type | Description |
---|---|---|
id | any | The id of node. |
role | string | The basic influence role. |
roleInfluence | float | The influence magnitude related to the node's role. |
roleLevel | string | The level of role, a role subcategory. |
influence | float | A normalized influence score based on indegree and outdegree. |
indegree | integer | The number of incoming connections. |
outdegree | integer | The number of outcoming connections. |
normalizedIndegree | float | The normalized number of incoming connections. |
normalizedOutdegree | float | The normalized number of outcoming connections. |
rank | integer | The normalized influence ranking based on the value of influence field. |
[
{
id: 4,
role: 'hub',
roleInfluence: 0.9210526315789473,
roleLevel: 'strong',
influence: 0.9210526315789473,
indegree: 16,
outdegree: 19,
normalizedIndegree: 0.8421052631578947,
normalizedOutdegree: 1.0,
rank: 1
},
{
id: 3,
role: 'emitter',
roleInfluence: 0.9473684210526315,
roleLevel: 'strong',
influence: 0.47368421052631576,
indegree: 0,
outdegree: 18,
normalizedIndegree: 0.0,
normalizedOutdegree: 0.9473684210526315
rank: 2
},
...
]
Given a list of BIRs, can be calculated the distribution of BIRs in a network, as a normalized frequency between roles and also between their levels.
BIRs.distribution(data=[]);
Field | Type | Required | Description |
---|---|---|---|
data | [{...}] | yes | The list of roles, the output of BIRs' detection methods. |
// Detect basic influence roles of nodes
data = BIRs.detectFromNodes([...])
// Detect the distribution of BIRs
res = BIRs.distribution(data)
{
reducer: {
count: 12,
frequency: 0.12,
levels: {
none: {count: 0, frequency: 0.0},
branch: {count: 0, frequency: 0.0},
weak: {count: 7, frequency: 0.07},
strong: {count: 5, frequency: 0.05},
top: {count: 0, frequency: 0.0}
}
},
amplifier: {
count: 13,
frequency: 0.13,
levels: {
none: {count: 0, frequency: 0.0},
branch: {count: 0, frequency: 0.0},
weak: {count: 12, frequency: 0.12},
strong: {count: 1, frequency: 0.01},
top: {count: 0, frequency: 0.0}
}
},
emitter: {
count: 28,
frequency: 0.28,
levels: {
none: {count: 0, frequency: 0.0},
branch: {count: 18, frequency: 0.18},
weak: {count: 10, frequency: 0.1},
strong: {count: 0, frequency: 0.0},
top: {count: 0, frequency: 0.0}
}
},
...
}
The package is battle tested with a coverage of 98%. Unit tests are inside the folder /test
.
At first, install dev requirements:
npm install
To run all unit tests with coverage through nyc, type:
npm test
If you use this software in your work, please cite it as below:
Miceli, D. (2024). Basic Influence Roles (BIRs) [Computer software]. https://github.com/davidemiceli/basic-influence-roles
Or the BibTeX version:
@software{MiceliBasicInfluenceRoles2024,
author = {Miceli, Davide},
license = {MIT},
month = mar,
title = {{Basic Influence Roles (BIRs)}},
url = {https://github.com/davidemiceli/basic-influence-roles},
year = {2024}
}
Basic Influence Roles is an open source project available under the MIT license.
FAQs
Detect and measure the Basic Influence Role each node holds within a Directed Network.
The npm package basic-influence-roles receives a total of 1 weekly downloads. As such, basic-influence-roles popularity was classified as not popular.
We found that basic-influence-roles demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.