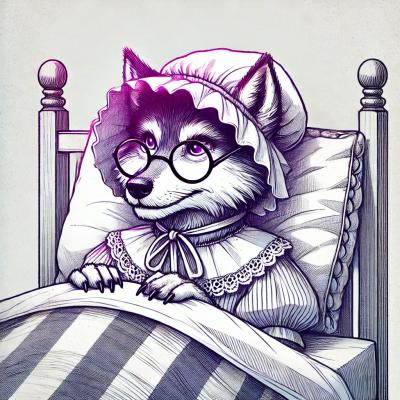
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
A benchmarking library that works on nearly all JavaScript platforms, supports high-resolution timers, and returns statistically significant results.
The 'benchmark' npm package is a library for benchmarking JavaScript code. It provides a simple and flexible API to measure the performance of code snippets, allowing developers to compare the speed of different implementations and optimize their code.
Basic Benchmarking
This feature allows you to create a suite of benchmarks to compare the performance of different code snippets. The example demonstrates how to add tests, attach listeners for cycle and completion events, and run the suite asynchronously.
const Benchmark = require('benchmark');
const suite = new Benchmark.Suite;
// add tests
suite.add('RegExp#test', function() {
/o/.test('Hello World!');
})
.add('String#indexOf', function() {
'Hello World!'.indexOf('o') > -1;
})
// add listeners
.on('cycle', function(event) {
console.log(String(event.target));
})
.on('complete', function() {
console.log('Fastest is ' + this.filter('fastest').map('name'));
})
// run async
.run({ 'async': true });
Event Handling
This feature allows you to handle various events during the benchmarking process, such as 'start', 'cycle', 'complete', etc. The example shows how to attach event listeners to a benchmark suite.
const Benchmark = require('benchmark');
const suite = new Benchmark.Suite;
suite.add('Example Test', function() {
// code to benchmark
})
.on('start', function() {
console.log('Benchmark started');
})
.on('complete', function() {
console.log('Benchmark completed');
})
.run();
Customizing Benchmark Options
This feature allows you to customize various options for your benchmarks, such as the minimum number of samples. The example demonstrates how to set the 'minSamples' option for a benchmark test.
const Benchmark = require('benchmark');
const suite = new Benchmark.Suite;
suite.add('Example Test', function() {
// code to benchmark
}, {
'minSamples': 100
})
.run();
The 'fastest' package is another benchmarking tool for JavaScript. It focuses on providing a simple API for running benchmarks and comparing the performance of different code snippets. Compared to 'benchmark', 'fastest' is more lightweight and may be easier to use for simple benchmarking tasks.
The 'matcha' package is a powerful benchmarking library for Node.js. It provides a command-line interface and a flexible API for writing and running benchmarks. 'matcha' offers more advanced features and a more comprehensive reporting system compared to 'benchmark'.
The 'benchmarkjs' package is a fork of the 'benchmark' library with additional features and improvements. It aims to provide a more modern and feature-rich benchmarking tool while maintaining compatibility with the original 'benchmark' API.
A robust benchmarking library that works on nearly all JavaScript platforms1, supports high-resolution timers, and returns statistically significant results. As seen on jsPerf.
Benchmark.js is part of the BestieJS "Best in Class" module collection. This means we promote solid browser/environment support, ES5 precedents, unit testing, and plenty of documentation.
The documentation for Benchmark.js can be viewed here: http://benchmarkjs.com/docs
For a list of upcoming features, check out our roadmap.
Benchmark.js has been tested in at least Adobe AIR 3.1, Chrome 5-21, Firefox 1.5-13, IE 6-9, Opera 9.25-12.01, Safari 3-6, Node.js 0.8.6, Narwhal 0.3.2, RingoJS 0.8, and Rhino 1.7RC5.
In a browser or Adobe AIR:
<script src="benchmark.js"></script>
Optionally, expose Java’s nanosecond timer by adding the nano
applet to the <body>
:
<applet code="nano" archive="nano.jar"></applet>
Or enable Chrome’s microsecond timer by using the command line switch:
--enable-benchmarking
Via npm:
npm install benchmark
In Node.js and RingoJS v0.8.0+:
var Benchmark = require('benchmark');
Optionally, use the microtime module by Wade Simmons:
npm install microtime
In RingoJS v0.7.0-:
var Benchmark = require('benchmark').Benchmark;
In Rhino:
load('benchmark.js');
In an AMD loader like RequireJS:
require({
'paths': {
'benchmark': 'path/to/benchmark'
}
},
['benchmark'], function(Benchmark) {
console.log(Benchmark.version);
});
// or with platform.js
// https://github.com/bestiejs/platform.js
require({
'paths': {
'benchmark': 'path/to/benchmark',
'platform': 'path/to/platform'
}
},
['benchmark', 'platform'], function(Benchmark, platform) {
Benchmark.platform = platform;
console.log(Benchmark.platform.name);
});
Usage example:
var suite = new Benchmark.Suite;
// add tests
suite.add('RegExp#test', function() {
/o/.test('Hello World!');
})
.add('String#indexOf', function() {
'Hello World!'.indexOf('o') > -1;
})
// add listeners
.on('cycle', function(event) {
console.log(String(event.target));
})
.on('complete', function() {
console.log('Fastest is ' + this.filter('fastest').pluck('name'));
})
// run async
.run({ 'async': true });
// logs:
// > RegExp#test x 4,161,532 +-0.99% (59 cycles)
// > String#indexOf x 6,139,623 +-1.00% (131 cycles)
// > Fastest is String#indexOf
FAQs
A benchmarking library that supports high-resolution timers & returns statistically significant results.
The npm package benchmark receives a total of 277,110 weekly downloads. As such, benchmark popularity was classified as popular.
We found that benchmark demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.