Comparing version 3.0.0 to 4.0.0
358
bl.js
'use strict' | ||
var DuplexStream = require('readable-stream').Duplex | ||
, util = require('util') | ||
function BufferList (callback) { | ||
if (!(this instanceof BufferList)) | ||
return new BufferList(callback) | ||
const DuplexStream = require('readable-stream').Duplex | ||
const util = require('util') | ||
const BufferList = require('./BufferList') | ||
this._bufs = [] | ||
this.length = 0 | ||
function BufferListStream (callback) { | ||
if (!(this instanceof BufferListStream)) { | ||
return new BufferListStream(callback) | ||
} | ||
if (typeof callback == 'function') { | ||
if (typeof callback === 'function') { | ||
this._callback = callback | ||
var piper = function piper (err) { | ||
const piper = function piper (err) { | ||
if (this._callback) { | ||
@@ -28,76 +28,29 @@ this._callback(err) | ||
}) | ||
} else { | ||
this.append(callback) | ||
callback = null | ||
} | ||
BufferList._init.call(this, callback) | ||
DuplexStream.call(this) | ||
} | ||
util.inherits(BufferListStream, DuplexStream) | ||
Object.assign(BufferListStream.prototype, BufferList.prototype) | ||
util.inherits(BufferList, DuplexStream) | ||
BufferList.prototype._offset = function _offset (offset) { | ||
var tot = 0, i = 0, _t | ||
if (offset === 0) return [ 0, 0 ] | ||
for (; i < this._bufs.length; i++) { | ||
_t = tot + this._bufs[i].length | ||
if (offset < _t || i == this._bufs.length - 1) { | ||
return [ i, offset - tot ] | ||
} | ||
tot = _t | ||
} | ||
BufferListStream.prototype._new = function _new (callback) { | ||
return new BufferListStream(callback) | ||
} | ||
BufferList.prototype._reverseOffset = function (blOffset) { | ||
var bufferId = blOffset[0] | ||
var offset = blOffset[1] | ||
for (var i = 0; i < bufferId; i++) { | ||
offset += this._bufs[i].length | ||
} | ||
return offset | ||
} | ||
BufferList.prototype.append = function append (buf) { | ||
var i = 0 | ||
if (Buffer.isBuffer(buf)) { | ||
this._appendBuffer(buf) | ||
} else if (Array.isArray(buf)) { | ||
for (; i < buf.length; i++) | ||
this.append(buf[i]) | ||
} else if (buf instanceof BufferList) { | ||
// unwrap argument into individual BufferLists | ||
for (; i < buf._bufs.length; i++) | ||
this.append(buf._bufs[i]) | ||
} else if (buf != null) { | ||
// coerce number arguments to strings, since Buffer(number) does | ||
// uninitialized memory allocation | ||
if (typeof buf == 'number') | ||
buf = buf.toString() | ||
this._appendBuffer(Buffer.from(buf)) | ||
} | ||
return this | ||
} | ||
BufferList.prototype._appendBuffer = function appendBuffer (buf) { | ||
this._bufs.push(buf) | ||
this.length += buf.length | ||
} | ||
BufferList.prototype._write = function _write (buf, encoding, callback) { | ||
BufferListStream.prototype._write = function _write (buf, encoding, callback) { | ||
this._appendBuffer(buf) | ||
if (typeof callback == 'function') | ||
if (typeof callback === 'function') { | ||
callback() | ||
} | ||
} | ||
BufferList.prototype._read = function _read (size) { | ||
if (!this.length) | ||
BufferListStream.prototype._read = function _read (size) { | ||
if (!this.length) { | ||
return this.push(null) | ||
} | ||
@@ -109,4 +62,3 @@ size = Math.min(size, this.length) | ||
BufferList.prototype.end = function end (chunk) { | ||
BufferListStream.prototype.end = function end (chunk) { | ||
DuplexStream.prototype.end.call(this, chunk) | ||
@@ -120,146 +72,3 @@ | ||
BufferList.prototype.get = function get (index) { | ||
if (index > this.length || index < 0) { | ||
return undefined | ||
} | ||
var offset = this._offset(index) | ||
return this._bufs[offset[0]][offset[1]] | ||
} | ||
BufferList.prototype.slice = function slice (start, end) { | ||
if (typeof start == 'number' && start < 0) | ||
start += this.length | ||
if (typeof end == 'number' && end < 0) | ||
end += this.length | ||
return this.copy(null, 0, start, end) | ||
} | ||
BufferList.prototype.copy = function copy (dst, dstStart, srcStart, srcEnd) { | ||
if (typeof srcStart != 'number' || srcStart < 0) | ||
srcStart = 0 | ||
if (typeof srcEnd != 'number' || srcEnd > this.length) | ||
srcEnd = this.length | ||
if (srcStart >= this.length) | ||
return dst || Buffer.alloc(0) | ||
if (srcEnd <= 0) | ||
return dst || Buffer.alloc(0) | ||
var copy = !!dst | ||
, off = this._offset(srcStart) | ||
, len = srcEnd - srcStart | ||
, bytes = len | ||
, bufoff = (copy && dstStart) || 0 | ||
, start = off[1] | ||
, l | ||
, i | ||
// copy/slice everything | ||
if (srcStart === 0 && srcEnd == this.length) { | ||
if (!copy) { // slice, but full concat if multiple buffers | ||
return this._bufs.length === 1 | ||
? this._bufs[0] | ||
: Buffer.concat(this._bufs, this.length) | ||
} | ||
// copy, need to copy individual buffers | ||
for (i = 0; i < this._bufs.length; i++) { | ||
this._bufs[i].copy(dst, bufoff) | ||
bufoff += this._bufs[i].length | ||
} | ||
return dst | ||
} | ||
// easy, cheap case where it's a subset of one of the buffers | ||
if (bytes <= this._bufs[off[0]].length - start) { | ||
return copy | ||
? this._bufs[off[0]].copy(dst, dstStart, start, start + bytes) | ||
: this._bufs[off[0]].slice(start, start + bytes) | ||
} | ||
if (!copy) // a slice, we need something to copy in to | ||
dst = Buffer.allocUnsafe(len) | ||
for (i = off[0]; i < this._bufs.length; i++) { | ||
l = this._bufs[i].length - start | ||
if (bytes > l) { | ||
this._bufs[i].copy(dst, bufoff, start) | ||
} else { | ||
this._bufs[i].copy(dst, bufoff, start, start + bytes) | ||
break | ||
} | ||
bufoff += l | ||
bytes -= l | ||
if (start) | ||
start = 0 | ||
} | ||
return dst | ||
} | ||
BufferList.prototype.shallowSlice = function shallowSlice (start, end) { | ||
start = start || 0 | ||
end = typeof end !== 'number' ? this.length : end | ||
if (start < 0) | ||
start += this.length | ||
if (end < 0) | ||
end += this.length | ||
if (start === end) { | ||
return new BufferList() | ||
} | ||
var startOffset = this._offset(start) | ||
, endOffset = this._offset(end) | ||
, buffers = this._bufs.slice(startOffset[0], endOffset[0] + 1) | ||
if (endOffset[1] == 0) | ||
buffers.pop() | ||
else | ||
buffers[buffers.length-1] = buffers[buffers.length-1].slice(0, endOffset[1]) | ||
if (startOffset[1] != 0) | ||
buffers[0] = buffers[0].slice(startOffset[1]) | ||
return new BufferList(buffers) | ||
} | ||
BufferList.prototype.toString = function toString (encoding, start, end) { | ||
return this.slice(start, end).toString(encoding) | ||
} | ||
BufferList.prototype.consume = function consume (bytes) { | ||
while (this._bufs.length) { | ||
if (bytes >= this._bufs[0].length) { | ||
bytes -= this._bufs[0].length | ||
this.length -= this._bufs[0].length | ||
this._bufs.shift() | ||
} else { | ||
this._bufs[0] = this._bufs[0].slice(bytes) | ||
this.length -= bytes | ||
break | ||
} | ||
} | ||
return this | ||
} | ||
BufferList.prototype.duplicate = function duplicate () { | ||
var i = 0 | ||
, copy = new BufferList() | ||
for (; i < this._bufs.length; i++) | ||
copy.append(this._bufs[i]) | ||
return copy | ||
} | ||
BufferList.prototype._destroy = function _destroy (err, cb) { | ||
BufferListStream.prototype._destroy = function _destroy (err, cb) { | ||
this._bufs.length = 0 | ||
@@ -270,117 +79,10 @@ this.length = 0 | ||
BufferList.prototype.indexOf = function (search, offset, encoding) { | ||
if (encoding === undefined && typeof offset === 'string') { | ||
encoding = offset | ||
offset = undefined | ||
} | ||
if (typeof search === 'function' || Array.isArray(search)) { | ||
throw new TypeError('The "value" argument must be one of type string, Buffer, BufferList, or Uint8Array.') | ||
} else if (typeof search === 'number') { | ||
search = Buffer.from([search]) | ||
} else if (typeof search === 'string') { | ||
search = Buffer.from(search, encoding) | ||
} else if (search instanceof BufferList) { | ||
search = search.slice() | ||
} else if (!Buffer.isBuffer(search)) { | ||
search = Buffer.from(search) | ||
} | ||
offset = Number(offset || 0) | ||
if (isNaN(offset)) { | ||
offset = 0 | ||
} | ||
if (offset < 0) { | ||
offset = this.length + offset | ||
} | ||
if (offset < 0) { | ||
offset = 0 | ||
} | ||
if (search.length === 0) { | ||
return offset > this.length ? this.length : offset | ||
} | ||
var blOffset = this._offset(offset) | ||
var blIndex = blOffset[0] // index of which internal buffer we're working on | ||
var buffOffset = blOffset[1] // offset of the internal buffer we're working on | ||
// scan over each buffer | ||
for (blIndex; blIndex < this._bufs.length; blIndex++) { | ||
var buff = this._bufs[blIndex] | ||
while(buffOffset < buff.length) { | ||
var availableWindow = buff.length - buffOffset | ||
if (availableWindow >= search.length) { | ||
var nativeSearchResult = buff.indexOf(search, buffOffset) | ||
if (nativeSearchResult !== -1) { | ||
return this._reverseOffset([blIndex, nativeSearchResult]) | ||
} | ||
buffOffset = buff.length - search.length + 1 // end of native search window | ||
} else { | ||
var revOffset = this._reverseOffset([blIndex, buffOffset]) | ||
if (this._match(revOffset, search)) { | ||
return revOffset | ||
} | ||
buffOffset++ | ||
} | ||
} | ||
buffOffset = 0 | ||
} | ||
return -1 | ||
BufferListStream.prototype._isBufferList = function _isBufferList (b) { | ||
return b instanceof BufferListStream || b instanceof BufferList || BufferListStream.isBufferList(b) | ||
} | ||
BufferList.prototype._match = function(offset, search) { | ||
if (this.length - offset < search.length) { | ||
return false | ||
} | ||
for (var searchOffset = 0; searchOffset < search.length ; searchOffset++) { | ||
if(this.get(offset + searchOffset) !== search[searchOffset]){ | ||
return false | ||
} | ||
} | ||
return true | ||
} | ||
BufferListStream.isBufferList = BufferList.isBufferList | ||
;(function () { | ||
var methods = { | ||
'readDoubleBE' : 8 | ||
, 'readDoubleLE' : 8 | ||
, 'readFloatBE' : 4 | ||
, 'readFloatLE' : 4 | ||
, 'readInt32BE' : 4 | ||
, 'readInt32LE' : 4 | ||
, 'readUInt32BE' : 4 | ||
, 'readUInt32LE' : 4 | ||
, 'readInt16BE' : 2 | ||
, 'readInt16LE' : 2 | ||
, 'readUInt16BE' : 2 | ||
, 'readUInt16LE' : 2 | ||
, 'readInt8' : 1 | ||
, 'readUInt8' : 1 | ||
, 'readIntBE' : null | ||
, 'readIntLE' : null | ||
, 'readUIntBE' : null | ||
, 'readUIntLE' : null | ||
} | ||
for (var m in methods) { | ||
(function (m) { | ||
if (methods[m] === null) { | ||
BufferList.prototype[m] = function (offset, byteLength) { | ||
return this.slice(offset, offset + byteLength)[m](0, byteLength) | ||
} | ||
} | ||
else { | ||
BufferList.prototype[m] = function (offset) { | ||
return this.slice(offset, offset + methods[m])[m](0) | ||
} | ||
} | ||
}(m)) | ||
} | ||
}()) | ||
module.exports = BufferList | ||
module.exports = BufferListStream | ||
module.exports.BufferListStream = BufferListStream | ||
module.exports.BufferList = BufferList |
The MIT License (MIT) | ||
===================== | ||
Copyright (c) 2013-2018 bl contributors | ||
Copyright (c) 2013-2019 bl contributors | ||
---------------------------------- | ||
@@ -6,0 +6,0 @@ |
{ | ||
"name": "bl", | ||
"version": "3.0.0", | ||
"version": "4.0.0", | ||
"description": "Buffer List: collect buffers and access with a standard readable Buffer interface, streamable too!", | ||
"main": "bl.js", | ||
"scripts": { | ||
"test": "node test/test.js | faucet" | ||
"lint": "standard *.js test/*.js", | ||
"test": "npm run lint && node test/test.js | faucet" | ||
}, | ||
@@ -27,9 +28,9 @@ "repository": { | ||
"dependencies": { | ||
"readable-stream": "^3.0.1" | ||
"readable-stream": "^3.4.0" | ||
}, | ||
"devDependencies": { | ||
"faucet": "0.0.1", | ||
"hash_file": "~0.1.1", | ||
"tape": "~4.9.1" | ||
"faucet": "~0.0.1", | ||
"standard": "^14.3.0", | ||
"tape": "^4.11.0" | ||
} | ||
} |
@@ -7,4 +7,3 @@ # bl *(BufferList)* | ||
[](https://nodei.co/npm/bl/) | ||
[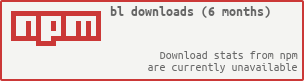](https://nodei.co/npm/bl/) | ||
[](https://nodei.co/npm/bl/) | ||
@@ -16,5 +15,5 @@ **bl** is a storage object for collections of Node Buffers, exposing them with the main Buffer readable API. Also works as a duplex stream so you can collect buffers from a stream that emits them and emit buffers to a stream that consumes them! | ||
```js | ||
const BufferList = require('bl') | ||
const { BufferList } = require('bl') | ||
var bl = new BufferList() | ||
const bl = new BufferList() | ||
bl.append(Buffer.from('abcd')) | ||
@@ -50,7 +49,7 @@ bl.append(Buffer.from('efg')) | ||
```js | ||
const bl = require('bl') | ||
, fs = require('fs') | ||
const { BufferListStream } = require('bl') | ||
const fs = require('fs') | ||
fs.createReadStream('README.md') | ||
.pipe(bl(function (err, data) { // note 'new' isn't strictly required | ||
.pipe(BufferListStream((err, data) => { // note 'new' isn't strictly required | ||
// `data` is a complete Buffer object containing the full data | ||
@@ -64,8 +63,10 @@ console.log(data.toString()) | ||
Or to fetch a URL using [hyperquest](https://github.com/substack/hyperquest) (should work with [request](http://github.com/mikeal/request) and even plain Node http too!): | ||
```js | ||
const hyperquest = require('hyperquest') | ||
, bl = require('bl') | ||
, url = 'https://raw.github.com/rvagg/bl/master/README.md' | ||
const { BufferListStream } = require('bl') | ||
hyperquest(url).pipe(bl(function (err, data) { | ||
const url = 'https://raw.github.com/rvagg/bl/master/README.md' | ||
hyperquest(url).pipe(BufferListStream((err, data) => { | ||
console.log(data.toString()) | ||
@@ -78,4 +79,4 @@ })) | ||
```js | ||
const BufferList = require('bl') | ||
, fs = require('fs') | ||
const { BufferList } = require('bl') | ||
const fs = require('fs') | ||
@@ -93,3 +94,4 @@ var bl = new BufferList() | ||
* <a href="#ctor"><code><b>new BufferList([ callback ])</b></code></a> | ||
* <a href="#ctor"><code><b>new BufferList([ buf ])</b></code></a> | ||
* <a href="#isBufferList"><code><b>BufferList.isBufferList(obj)</b></code></a> | ||
* <a href="#length"><code>bl.<b>length</b></code></a> | ||
@@ -106,24 +108,29 @@ * <a href="#append"><code>bl.<b>append(buffer)</b></code></a> | ||
* <a href="#readXX"><code>bl.<b>readDoubleBE()</b></code>, <code>bl.<b>readDoubleLE()</b></code>, <code>bl.<b>readFloatBE()</b></code>, <code>bl.<b>readFloatLE()</b></code>, <code>bl.<b>readInt32BE()</b></code>, <code>bl.<b>readInt32LE()</b></code>, <code>bl.<b>readUInt32BE()</b></code>, <code>bl.<b>readUInt32LE()</b></code>, <code>bl.<b>readInt16BE()</b></code>, <code>bl.<b>readInt16LE()</b></code>, <code>bl.<b>readUInt16BE()</b></code>, <code>bl.<b>readUInt16LE()</b></code>, <code>bl.<b>readInt8()</b></code>, <code>bl.<b>readUInt8()</b></code></a> | ||
* <a href="#streams">Streams</a> | ||
* <a href="#ctorStream"><code><b>new BufferListStream([ callback ])</b></code></a> | ||
-------------------------------------------------------- | ||
<a name="ctor"></a> | ||
### new BufferList([ callback | Buffer | Buffer array | BufferList | BufferList array | String ]) | ||
The constructor takes an optional callback, if supplied, the callback will be called with an error argument followed by a reference to the **bl** instance, when `bl.end()` is called (i.e. from a piped stream). This is a convenient method of collecting the entire contents of a stream, particularly when the stream is *chunky*, such as a network stream. | ||
### new BufferList([ Buffer | Buffer array | BufferList | BufferList array | String ]) | ||
No arguments are _required_ for the constructor, but you can initialise the list by passing in a single `Buffer` object or an array of `Buffer` objects. | ||
Normally, no arguments are required for the constructor, but you can initialise the list by passing in a single `Buffer` object or an array of `Buffer` object. | ||
`new` is not strictly required, if you don't instantiate a new object, it will be done automatically for you so you can create a new instance simply with: | ||
```js | ||
var bl = require('bl') | ||
var myinstance = bl() | ||
const { BufferList } = require('bl') | ||
const bl = BufferList() | ||
// equivalent to: | ||
var BufferList = require('bl') | ||
var myinstance = new BufferList() | ||
const { BufferList } = require('bl') | ||
const bl = new BufferList() | ||
``` | ||
-------------------------------------------------------- | ||
<a name="isBufferList"></a> | ||
### BufferList.isBufferList(obj) | ||
Determines if the passed object is a `BufferList`. It will return `true` if the passed object is an instance of `BufferList` **or** `BufferListStream` and `false` otherwise. | ||
N.B. this won't return `true` for `BufferList` or `BufferListStream` instances created by versions of this library before this static method was added. | ||
-------------------------------------------------------- | ||
<a name="length"></a> | ||
@@ -204,6 +211,30 @@ ### bl.length | ||
-------------------------------------------------------- | ||
<a name="streams"></a> | ||
### Streams | ||
**bl** is a Node **[Duplex Stream](http://nodejs.org/docs/latest/api/stream.html#stream_class_stream_duplex)**, so it can be read from and written to like a standard Node stream. You can also `pipe()` to and from a **bl** instance. | ||
<a name="ctorStream"></a> | ||
### new BufferListStream([ callback | Buffer | Buffer array | BufferList | BufferList array | String ]) | ||
**BufferListStream** is a Node **[Duplex Stream](http://nodejs.org/docs/latest/api/stream.html#stream_class_stream_duplex)**, so it can be read from and written to like a standard Node stream. You can also `pipe()` to and from a **BufferListStream** instance. | ||
The constructor takes an optional callback, if supplied, the callback will be called with an error argument followed by a reference to the **bl** instance, when `bl.end()` is called (i.e. from a piped stream). This is a convenient method of collecting the entire contents of a stream, particularly when the stream is *chunky*, such as a network stream. | ||
Normally, no arguments are required for the constructor, but you can initialise the list by passing in a single `Buffer` object or an array of `Buffer` object. | ||
`new` is not strictly required, if you don't instantiate a new object, it will be done automatically for you so you can create a new instance simply with: | ||
```js | ||
const { BufferListStream } = require('bl') | ||
const bl = BufferListStream() | ||
// equivalent to: | ||
const { BufferListStream } = require('bl') | ||
const bl = new BufferListStream() | ||
``` | ||
N.B. For backwards compatibility reasons, `BufferListStream` is the **default** export when you `require('bl')`: | ||
```js | ||
const { BufferListStream } = require('bl') | ||
// equivalent to: | ||
const BufferListStream = require('bl') | ||
``` | ||
-------------------------------------------------------- | ||
@@ -219,9 +250,7 @@ | ||
======= | ||
<a name="license"></a> | ||
## License & copyright | ||
Copyright (c) 2013-2018 bl contributors (listed above). | ||
Copyright (c) 2013-2019 bl contributors (listed above). | ||
bl is licensed under the MIT license. All rights not explicitly granted in the MIT license are reserved. See the included LICENSE.md file for more details. |
'use strict' | ||
var tape = require('tape') | ||
, BufferList = require('../') | ||
, Buffer = require('safe-buffer').Buffer | ||
const tape = require('tape') | ||
const BufferList = require('../') | ||
const Buffer = require('safe-buffer').Buffer | ||
tape('indexOf single byte needle', t => { | ||
tape('indexOf single byte needle', (t) => { | ||
const bl = new BufferList(['abcdefg', 'abcdefg', '12345']) | ||
t.equal(bl.indexOf('e'), 4) | ||
@@ -13,28 +14,45 @@ t.equal(bl.indexOf('e', 5), 11) | ||
t.equal(bl.indexOf('5'), 18) | ||
t.end() | ||
}) | ||
tape('indexOf multiple byte needle', t => { | ||
tape('indexOf multiple byte needle', (t) => { | ||
const bl = new BufferList(['abcdefg', 'abcdefg']) | ||
t.equal(bl.indexOf('ef'), 4) | ||
t.equal(bl.indexOf('ef', 5), 11) | ||
t.end() | ||
}) | ||
tape('indexOf multiple byte needles across buffer boundaries', t => { | ||
tape('indexOf multiple byte needles across buffer boundaries', (t) => { | ||
const bl = new BufferList(['abcdefg', 'abcdefg']) | ||
t.equal(bl.indexOf('fgabc'), 5) | ||
t.end() | ||
}) | ||
tape('indexOf takes a buffer list search', t => { | ||
tape('indexOf takes a Uint8Array search', (t) => { | ||
const bl = new BufferList(['abcdefg', 'abcdefg']) | ||
const search = new Uint8Array([102, 103, 97, 98, 99]) // fgabc | ||
t.equal(bl.indexOf(search), 5) | ||
t.end() | ||
}) | ||
tape('indexOf takes a buffer list search', (t) => { | ||
const bl = new BufferList(['abcdefg', 'abcdefg']) | ||
const search = new BufferList('fgabc') | ||
t.equal(bl.indexOf(search), 5) | ||
t.end() | ||
}) | ||
tape('indexOf a zero byte needle', t => { | ||
tape('indexOf a zero byte needle', (t) => { | ||
const b = new BufferList('abcdef') | ||
const buf_empty = Buffer.from('') | ||
const bufEmpty = Buffer.from('') | ||
t.equal(b.indexOf(''), 0) | ||
@@ -44,14 +62,17 @@ t.equal(b.indexOf('', 1), 1) | ||
t.equal(b.indexOf('', Infinity), b.length) | ||
t.equal(b.indexOf(buf_empty), 0) | ||
t.equal(b.indexOf(buf_empty, 1), 1) | ||
t.equal(b.indexOf(buf_empty, b.length + 1), b.length) | ||
t.equal(b.indexOf(buf_empty, Infinity), b.length) | ||
t.equal(b.indexOf(bufEmpty), 0) | ||
t.equal(b.indexOf(bufEmpty, 1), 1) | ||
t.equal(b.indexOf(bufEmpty, b.length + 1), b.length) | ||
t.equal(b.indexOf(bufEmpty, Infinity), b.length) | ||
t.end() | ||
}) | ||
tape('indexOf buffers smaller and larger than the needle', t => { | ||
tape('indexOf buffers smaller and larger than the needle', (t) => { | ||
const bl = new BufferList(['abcdefg', 'a', 'bcdefg', 'a', 'bcfgab']) | ||
t.equal(bl.indexOf('fgabc'), 5) | ||
t.equal(bl.indexOf('fgabc', 6), 12) | ||
t.equal(bl.indexOf('fgabc', 13), -1) | ||
t.end() | ||
@@ -61,3 +82,3 @@ }) | ||
// only present in node 6+ | ||
;(process.version.substr(1).split('.')[0] >= 6) && tape('indexOf latin1 and binary encoding', t => { | ||
;(process.version.substr(1).split('.')[0] >= 6) && tape('indexOf latin1 and binary encoding', (t) => { | ||
const b = new BufferList('abcdef') | ||
@@ -118,11 +139,12 @@ | ||
) | ||
t.end() | ||
}) | ||
tape('indexOf the entire nodejs10 buffer test suite', t => { | ||
tape('indexOf the entire nodejs10 buffer test suite', (t) => { | ||
const b = new BufferList('abcdef') | ||
const buf_a = Buffer.from('a') | ||
const buf_bc = Buffer.from('bc') | ||
const buf_f = Buffer.from('f') | ||
const buf_z = Buffer.from('z') | ||
const bufA = Buffer.from('a') | ||
const bufBc = Buffer.from('bc') | ||
const bufF = Buffer.from('f') | ||
const bufZ = Buffer.from('z') | ||
@@ -149,21 +171,22 @@ const stringComparison = 'abcdef' | ||
t.equal(b.indexOf('z'), -1) | ||
// empty search tests | ||
t.equal(b.indexOf(buf_a), 0) | ||
t.equal(b.indexOf(buf_a, 1), -1) | ||
t.equal(b.indexOf(buf_a, -1), -1) | ||
t.equal(b.indexOf(buf_a, -4), -1) | ||
t.equal(b.indexOf(buf_a, -b.length), 0) | ||
t.equal(b.indexOf(buf_a, NaN), 0) | ||
t.equal(b.indexOf(buf_a, -Infinity), 0) | ||
t.equal(b.indexOf(buf_a, Infinity), -1) | ||
t.equal(b.indexOf(buf_bc), 1) | ||
t.equal(b.indexOf(buf_bc, 2), -1) | ||
t.equal(b.indexOf(buf_bc, -1), -1) | ||
t.equal(b.indexOf(buf_bc, -3), -1) | ||
t.equal(b.indexOf(buf_bc, -5), 1) | ||
t.equal(b.indexOf(buf_bc, NaN), 1) | ||
t.equal(b.indexOf(buf_bc, -Infinity), 1) | ||
t.equal(b.indexOf(buf_bc, Infinity), -1) | ||
t.equal(b.indexOf(buf_f), b.length - 1) | ||
t.equal(b.indexOf(buf_z), -1) | ||
t.equal(b.indexOf(bufA), 0) | ||
t.equal(b.indexOf(bufA, 1), -1) | ||
t.equal(b.indexOf(bufA, -1), -1) | ||
t.equal(b.indexOf(bufA, -4), -1) | ||
t.equal(b.indexOf(bufA, -b.length), 0) | ||
t.equal(b.indexOf(bufA, NaN), 0) | ||
t.equal(b.indexOf(bufA, -Infinity), 0) | ||
t.equal(b.indexOf(bufA, Infinity), -1) | ||
t.equal(b.indexOf(bufBc), 1) | ||
t.equal(b.indexOf(bufBc, 2), -1) | ||
t.equal(b.indexOf(bufBc, -1), -1) | ||
t.equal(b.indexOf(bufBc, -3), -1) | ||
t.equal(b.indexOf(bufBc, -5), 1) | ||
t.equal(b.indexOf(bufBc, NaN), 1) | ||
t.equal(b.indexOf(bufBc, -Infinity), 1) | ||
t.equal(b.indexOf(bufBc, Infinity), -1) | ||
t.equal(b.indexOf(bufF), b.length - 1) | ||
t.equal(b.indexOf(bufZ), -1) | ||
t.equal(b.indexOf(0x61), 0) | ||
@@ -252,2 +275,3 @@ t.equal(b.indexOf(0x61, 1), -1) | ||
Buffer.from('\u039a\u0391abc\u03a3\u03a3\u0395', 'ucs2') | ||
t.equal(6, mixedByteStringUcs2.indexOf('bc', 0, 'ucs2')) | ||
@@ -292,2 +316,3 @@ t.equal(10, mixedByteStringUcs2.indexOf('\u03a3', 0, 'ucs2')) | ||
const mixedByteStringUtf8 = Buffer.from('\u039a\u0391abc\u03a3\u03a3\u0395') | ||
t.equal(5, mixedByteStringUtf8.indexOf('bc')) | ||
@@ -299,7 +324,6 @@ t.equal(5, mixedByteStringUtf8.indexOf('bc', 5)) | ||
// Test complex string indexOf algorithms. Only trigger for long strings. | ||
// Long string that isn't a simple repeat of a shorter string. | ||
let longString = 'A' | ||
for (let i = 66; i < 76; i++) { // from 'B' to 'K' | ||
for (let i = 66; i < 76; i++) { // from 'B' to 'K' | ||
longString = longString + String.fromCharCode(i) + longString | ||
@@ -315,3 +339,3 @@ } | ||
t.equal((i + 15) & ~0xf, index, | ||
`Long ABACABA...-string at index ${i}`) | ||
`Long ABACABA...-string at index ${i}`) | ||
} | ||
@@ -339,3 +363,6 @@ | ||
const allCodePoints = [] | ||
for (let i = 0; i < 65536; i++) allCodePoints[i] = i | ||
for (let i = 0; i < 65536; i++) { | ||
allCodePoints[i] = i | ||
} | ||
const allCharsString = String.fromCharCode.apply(String, allCodePoints) | ||
@@ -361,3 +388,3 @@ const allCharsBufferUtf8 = Buffer.from(allCharsString) | ||
// Find substrings in Utf8. | ||
const lengths = [1, 3, 15]; // Single char, simple and complex. | ||
const lengths = [1, 3, 15] // Single char, simple and complex. | ||
const indices = [0x5, 0x60, 0x400, 0x680, 0x7ee, 0xFF02, 0x16610, 0x2f77b] | ||
@@ -392,4 +419,5 @@ for (let lengthIndex = 0; lengthIndex < lengths.length; lengthIndex++) { | ||
// Find substrings in Usc2. | ||
const lengths = [2, 4, 16]; // Single char, simple and complex. | ||
const lengths = [2, 4, 16] // Single char, simple and complex. | ||
const indices = [0x5, 0x65, 0x105, 0x205, 0x285, 0x2005, 0x2085, 0xfff0] | ||
for (let lengthIndex = 0; lengthIndex < lengths.length; lengthIndex++) { | ||
@@ -416,4 +444,3 @@ for (let i = 0; i < indices.length; i++) { | ||
[] | ||
].forEach(val => { | ||
debugger | ||
].forEach((val) => { | ||
t.throws(() => b.indexOf(val), TypeError, `"${JSON.stringify(val)}" should throw`) | ||
@@ -456,2 +483,3 @@ }) | ||
const buf = Buffer.from('this is a test') | ||
t.equal(buf.indexOf(0x6973), 3) | ||
@@ -472,7 +500,8 @@ t.equal(buf.indexOf(0x697320), 4) | ||
{ | ||
const needle = new Uint8Array([ 0x66, 0x6f, 0x6f ]) | ||
const needle = new Uint8Array([0x66, 0x6f, 0x6f]) | ||
const haystack = new BufferList(Buffer.from('a foo b foo')) | ||
t.equal(haystack.indexOf(needle), 2) | ||
} | ||
t.end() | ||
}) |
361
test/test.js
'use strict' | ||
var tape = require('tape') | ||
, crypto = require('crypto') | ||
, fs = require('fs') | ||
, hash = require('hash_file') | ||
, BufferList = require('../') | ||
, Buffer = require('safe-buffer').Buffer | ||
const tape = require('tape') | ||
const crypto = require('crypto') | ||
const fs = require('fs') | ||
const path = require('path') | ||
const BufferList = require('../') | ||
const Buffer = require('safe-buffer').Buffer | ||
, encodings = | ||
('hex utf8 utf-8 ascii binary base64' | ||
+ (process.browser ? '' : ' ucs2 ucs-2 utf16le utf-16le')).split(' ') | ||
const encodings = | ||
('hex utf8 utf-8 ascii binary base64' + | ||
(process.browser ? '' : ' ucs2 ucs-2 utf16le utf-16le')).split(' ') | ||
// run the indexOf tests | ||
require('./indexOf') | ||
require('./isBufferList') | ||
require('./convert') | ||
tape('single bytes from single buffer', function (t) { | ||
var bl = new BufferList() | ||
const bl = new BufferList() | ||
bl.append(Buffer.from('abcd')) | ||
@@ -33,3 +35,4 @@ | ||
tape('single bytes from multiple buffers', function (t) { | ||
var bl = new BufferList() | ||
const bl = new BufferList() | ||
bl.append(Buffer.from('abcd')) | ||
@@ -52,2 +55,3 @@ bl.append(Buffer.from('efg')) | ||
t.equal(bl.get(9), 106) | ||
t.end() | ||
@@ -57,3 +61,4 @@ }) | ||
tape('multi bytes from single buffer', function (t) { | ||
var bl = new BufferList() | ||
const bl = new BufferList() | ||
bl.append(Buffer.from('abcd')) | ||
@@ -72,3 +77,4 @@ | ||
tape('multi bytes from single buffer (negative indexes)', function (t) { | ||
var bl = new BufferList() | ||
const bl = new BufferList() | ||
bl.append(Buffer.from('buffer')) | ||
@@ -86,3 +92,3 @@ | ||
tape('multiple bytes from multiple buffers', function (t) { | ||
var bl = new BufferList() | ||
const bl = new BufferList() | ||
@@ -107,6 +113,6 @@ bl.append(Buffer.from('abcd')) | ||
tape('multiple bytes from multiple buffer lists', function (t) { | ||
var bl = new BufferList() | ||
const bl = new BufferList() | ||
bl.append(new BufferList([ Buffer.from('abcd'), Buffer.from('efg') ])) | ||
bl.append(new BufferList([ Buffer.from('hi'), Buffer.from('j') ])) | ||
bl.append(new BufferList([Buffer.from('abcd'), Buffer.from('efg')])) | ||
bl.append(new BufferList([Buffer.from('hi'), Buffer.from('j')])) | ||
@@ -127,12 +133,12 @@ t.equal(bl.length, 10) | ||
tape('multiple bytes from crazy nested buffer lists', function (t) { | ||
var bl = new BufferList() | ||
const bl = new BufferList() | ||
bl.append(new BufferList([ | ||
new BufferList([ | ||
new BufferList(Buffer.from('abc')) | ||
, Buffer.from('d') | ||
, new BufferList(Buffer.from('efg')) | ||
]) | ||
, new BufferList([ Buffer.from('hi') ]) | ||
, new BufferList(Buffer.from('j')) | ||
new BufferList([ | ||
new BufferList(Buffer.from('abc')), | ||
Buffer.from('d'), | ||
new BufferList(Buffer.from('efg')) | ||
]), | ||
new BufferList([Buffer.from('hi')]), | ||
new BufferList(Buffer.from('j')) | ||
])) | ||
@@ -153,20 +159,37 @@ | ||
tape('append accepts arrays of Buffers', function (t) { | ||
var bl = new BufferList() | ||
const bl = new BufferList() | ||
bl.append(Buffer.from('abc')) | ||
bl.append([ Buffer.from('def') ]) | ||
bl.append([ Buffer.from('ghi'), Buffer.from('jkl') ]) | ||
bl.append([ Buffer.from('mnop'), Buffer.from('qrstu'), Buffer.from('vwxyz') ]) | ||
bl.append([Buffer.from('def')]) | ||
bl.append([Buffer.from('ghi'), Buffer.from('jkl')]) | ||
bl.append([Buffer.from('mnop'), Buffer.from('qrstu'), Buffer.from('vwxyz')]) | ||
t.equal(bl.length, 26) | ||
t.equal(bl.slice().toString('ascii'), 'abcdefghijklmnopqrstuvwxyz') | ||
t.end() | ||
}) | ||
tape('append accepts arrays of Uint8Arrays', function (t) { | ||
const bl = new BufferList() | ||
bl.append(new Uint8Array([97, 98, 99])) | ||
bl.append([Uint8Array.from([100, 101, 102])]) | ||
bl.append([new Uint8Array([103, 104, 105]), new Uint8Array([106, 107, 108])]) | ||
bl.append([new Uint8Array([109, 110, 111, 112]), new Uint8Array([113, 114, 115, 116, 117]), new Uint8Array([118, 119, 120, 121, 122])]) | ||
t.equal(bl.length, 26) | ||
t.equal(bl.slice().toString('ascii'), 'abcdefghijklmnopqrstuvwxyz') | ||
t.end() | ||
}) | ||
tape('append accepts arrays of BufferLists', function (t) { | ||
var bl = new BufferList() | ||
const bl = new BufferList() | ||
bl.append(Buffer.from('abc')) | ||
bl.append([ new BufferList('def') ]) | ||
bl.append(new BufferList([ Buffer.from('ghi'), new BufferList('jkl') ])) | ||
bl.append([ Buffer.from('mnop'), new BufferList([ Buffer.from('qrstu'), Buffer.from('vwxyz') ]) ]) | ||
bl.append([new BufferList('def')]) | ||
bl.append(new BufferList([Buffer.from('ghi'), new BufferList('jkl')])) | ||
bl.append([Buffer.from('mnop'), new BufferList([Buffer.from('qrstu'), Buffer.from('vwxyz')])]) | ||
t.equal(bl.length, 26) | ||
t.equal(bl.slice().toString('ascii'), 'abcdefghijklmnopqrstuvwxyz') | ||
t.end() | ||
@@ -176,7 +199,9 @@ }) | ||
tape('append chainable', function (t) { | ||
var bl = new BufferList() | ||
const bl = new BufferList() | ||
t.ok(bl.append(Buffer.from('abcd')) === bl) | ||
t.ok(bl.append([ Buffer.from('abcd') ]) === bl) | ||
t.ok(bl.append([Buffer.from('abcd')]) === bl) | ||
t.ok(bl.append(new BufferList(Buffer.from('abcd'))) === bl) | ||
t.ok(bl.append([ new BufferList(Buffer.from('abcd')) ]) === bl) | ||
t.ok(bl.append([new BufferList(Buffer.from('abcd'))]) === bl) | ||
t.end() | ||
@@ -186,9 +211,10 @@ }) | ||
tape('append chainable (test results)', function (t) { | ||
var bl = new BufferList('abc') | ||
.append([ new BufferList('def') ]) | ||
.append(new BufferList([ Buffer.from('ghi'), new BufferList('jkl') ])) | ||
.append([ Buffer.from('mnop'), new BufferList([ Buffer.from('qrstu'), Buffer.from('vwxyz') ]) ]) | ||
const bl = new BufferList('abc') | ||
.append([new BufferList('def')]) | ||
.append(new BufferList([Buffer.from('ghi'), new BufferList('jkl')])) | ||
.append([Buffer.from('mnop'), new BufferList([Buffer.from('qrstu'), Buffer.from('vwxyz')])]) | ||
t.equal(bl.length, 26) | ||
t.equal(bl.slice().toString('ascii'), 'abcdefghijklmnopqrstuvwxyz') | ||
t.end() | ||
@@ -198,3 +224,3 @@ }) | ||
tape('consuming from multiple buffers', function (t) { | ||
var bl = new BufferList() | ||
const bl = new BufferList() | ||
@@ -234,3 +260,3 @@ bl.append(Buffer.from('abcd')) | ||
tape('complete consumption', function (t) { | ||
var bl = new BufferList() | ||
const bl = new BufferList() | ||
@@ -249,6 +275,6 @@ bl.append(Buffer.from('a')) | ||
tape('test readUInt8 / readInt8', function (t) { | ||
var buf1 = Buffer.alloc(1) | ||
, buf2 = Buffer.alloc(3) | ||
, buf3 = Buffer.alloc(3) | ||
, bl = new BufferList() | ||
const buf1 = Buffer.alloc(1) | ||
const buf2 = Buffer.alloc(3) | ||
const buf3 = Buffer.alloc(3) | ||
const bl = new BufferList() | ||
@@ -272,2 +298,3 @@ buf2[1] = 0x3 | ||
t.equal(bl.readInt8(5), 0x42) | ||
t.end() | ||
@@ -277,6 +304,6 @@ }) | ||
tape('test readUInt16LE / readUInt16BE / readInt16LE / readInt16BE', function (t) { | ||
var buf1 = Buffer.alloc(1) | ||
, buf2 = Buffer.alloc(3) | ||
, buf3 = Buffer.alloc(3) | ||
, bl = new BufferList() | ||
const buf1 = Buffer.alloc(1) | ||
const buf2 = Buffer.alloc(3) | ||
const buf3 = Buffer.alloc(3) | ||
const bl = new BufferList() | ||
@@ -304,2 +331,3 @@ buf2[1] = 0x3 | ||
t.equal(bl.readInt16LE(4), 0x4223) | ||
t.end() | ||
@@ -309,6 +337,6 @@ }) | ||
tape('test readUInt32LE / readUInt32BE / readInt32LE / readInt32BE', function (t) { | ||
var buf1 = Buffer.alloc(1) | ||
, buf2 = Buffer.alloc(3) | ||
, buf3 = Buffer.alloc(3) | ||
, bl = new BufferList() | ||
const buf1 = Buffer.alloc(1) | ||
const buf2 = Buffer.alloc(3) | ||
const buf3 = Buffer.alloc(3) | ||
const bl = new BufferList() | ||
@@ -328,2 +356,3 @@ buf2[1] = 0x3 | ||
t.equal(bl.readInt32LE(2), 0x42230403) | ||
t.end() | ||
@@ -333,6 +362,6 @@ }) | ||
tape('test readUIntLE / readUIntBE / readIntLE / readIntBE', function (t) { | ||
var buf1 = Buffer.alloc(1) | ||
, buf2 = Buffer.alloc(3) | ||
, buf3 = Buffer.alloc(3) | ||
, bl = new BufferList() | ||
const buf1 = Buffer.alloc(1) | ||
const buf2 = Buffer.alloc(3) | ||
const buf3 = Buffer.alloc(3) | ||
const bl = new BufferList() | ||
@@ -374,2 +403,3 @@ buf2[0] = 0x2 | ||
t.equal(bl.readIntLE(1, 6), 0x614223040302) | ||
t.end() | ||
@@ -379,6 +409,6 @@ }) | ||
tape('test readFloatLE / readFloatBE', function (t) { | ||
var buf1 = Buffer.alloc(1) | ||
, buf2 = Buffer.alloc(3) | ||
, buf3 = Buffer.alloc(3) | ||
, bl = new BufferList() | ||
const buf1 = Buffer.alloc(1) | ||
const buf2 = Buffer.alloc(3) | ||
const buf3 = Buffer.alloc(3) | ||
const bl = new BufferList() | ||
@@ -395,2 +425,3 @@ buf2[1] = 0x00 | ||
t.equal(bl.readFloatLE(2), 0x01) | ||
t.end() | ||
@@ -400,6 +431,6 @@ }) | ||
tape('test readDoubleLE / readDoubleBE', function (t) { | ||
var buf1 = Buffer.alloc(1) | ||
, buf2 = Buffer.alloc(3) | ||
, buf3 = Buffer.alloc(10) | ||
, bl = new BufferList() | ||
const buf1 = Buffer.alloc(1) | ||
const buf2 = Buffer.alloc(3) | ||
const buf3 = Buffer.alloc(10) | ||
const bl = new BufferList() | ||
@@ -420,2 +451,3 @@ buf2[1] = 0x55 | ||
t.equal(bl.readDoubleLE(2), 0.3333333333333333) | ||
t.end() | ||
@@ -425,3 +457,3 @@ }) | ||
tape('test toString', function (t) { | ||
var bl = new BufferList() | ||
const bl = new BufferList() | ||
@@ -443,4 +475,4 @@ bl.append(Buffer.from('abcd')) | ||
tape('test toString encoding', function (t) { | ||
var bl = new BufferList() | ||
, b = Buffer.from('abcdefghij\xff\x00') | ||
const bl = new BufferList() | ||
const b = Buffer.from('abcdefghij\xff\x00') | ||
@@ -454,4 +486,4 @@ bl.append(Buffer.from('abcd')) | ||
encodings.forEach(function (enc) { | ||
t.equal(bl.toString(enc), b.toString(enc), enc) | ||
}) | ||
t.equal(bl.toString(enc), b.toString(enc), enc) | ||
}) | ||
@@ -462,22 +494,23 @@ t.end() | ||
!process.browser && tape('test stream', function (t) { | ||
var random = crypto.randomBytes(65534) | ||
, rndhash = hash(random, 'md5') | ||
, md5sum = crypto.createHash('md5') | ||
, bl = new BufferList(function (err, buf) { | ||
t.ok(Buffer.isBuffer(buf)) | ||
t.ok(err === null) | ||
t.equal(rndhash, hash(bl.slice(), 'md5')) | ||
t.equal(rndhash, hash(buf, 'md5')) | ||
const random = crypto.randomBytes(65534) | ||
bl.pipe(fs.createWriteStream('/tmp/bl_test_rnd_out.dat')) | ||
.on('close', function () { | ||
var s = fs.createReadStream('/tmp/bl_test_rnd_out.dat') | ||
s.on('data', md5sum.update.bind(md5sum)) | ||
s.on('end', function() { | ||
t.equal(rndhash, md5sum.digest('hex'), 'woohoo! correct hash!') | ||
t.end() | ||
}) | ||
}) | ||
const bl = new BufferList((err, buf) => { | ||
t.ok(Buffer.isBuffer(buf)) | ||
t.ok(err === null) | ||
t.ok(random.equals(bl.slice())) | ||
t.ok(random.equals(buf.slice())) | ||
bl.pipe(fs.createWriteStream('/tmp/bl_test_rnd_out.dat')) | ||
.on('close', function () { | ||
const rndhash = crypto.createHash('md5').update(random).digest('hex') | ||
const md5sum = crypto.createHash('md5') | ||
const s = fs.createReadStream('/tmp/bl_test_rnd_out.dat') | ||
s.on('data', md5sum.update.bind(md5sum)) | ||
s.on('end', function () { | ||
t.equal(rndhash, md5sum.digest('hex'), 'woohoo! correct hash!') | ||
t.end() | ||
}) | ||
}) | ||
}) | ||
@@ -489,9 +522,10 @@ fs.writeFileSync('/tmp/bl_test_rnd.dat', random) | ||
tape('instantiation with Buffer', function (t) { | ||
var buf = crypto.randomBytes(1024) | ||
, buf2 = crypto.randomBytes(1024) | ||
, b = BufferList(buf) | ||
const buf = crypto.randomBytes(1024) | ||
const buf2 = crypto.randomBytes(1024) | ||
let b = BufferList(buf) | ||
t.equal(buf.toString('hex'), b.slice().toString('hex'), 'same buffer') | ||
b = BufferList([ buf, buf2 ]) | ||
t.equal(b.slice().toString('hex'), Buffer.concat([ buf, buf2 ]).toString('hex'), 'same buffer') | ||
b = BufferList([buf, buf2]) | ||
t.equal(b.slice().toString('hex'), Buffer.concat([buf, buf2]).toString('hex'), 'same buffer') | ||
t.end() | ||
@@ -501,4 +535,4 @@ }) | ||
tape('test String appendage', function (t) { | ||
var bl = new BufferList() | ||
, b = Buffer.from('abcdefghij\xff\x00') | ||
const bl = new BufferList() | ||
const b = Buffer.from('abcdefghij\xff\x00') | ||
@@ -512,4 +546,4 @@ bl.append('abcd') | ||
encodings.forEach(function (enc) { | ||
t.equal(bl.toString(enc), b.toString(enc)) | ||
}) | ||
t.equal(bl.toString(enc), b.toString(enc)) | ||
}) | ||
@@ -520,4 +554,4 @@ t.end() | ||
tape('test Number appendage', function (t) { | ||
var bl = new BufferList() | ||
, b = Buffer.from('1234567890') | ||
const bl = new BufferList() | ||
const b = Buffer.from('1234567890') | ||
@@ -530,4 +564,4 @@ bl.append(1234) | ||
encodings.forEach(function (enc) { | ||
t.equal(bl.toString(enc), b.toString(enc)) | ||
}) | ||
t.equal(bl.toString(enc), b.toString(enc)) | ||
}) | ||
@@ -549,6 +583,8 @@ t.end() | ||
t.plan(2) | ||
var inp1 = '\u2600' | ||
, inp2 = '\u2603' | ||
, exp = inp1 + ' and ' + inp2 | ||
, bl = BufferList() | ||
const inp1 = '\u2600' | ||
const inp2 = '\u2603' | ||
const exp = inp1 + ' and ' + inp2 | ||
const bl = BufferList() | ||
bl.write(inp1) | ||
@@ -562,4 +598,4 @@ bl.write(' and ') | ||
tape('should emit finish', function (t) { | ||
var source = BufferList() | ||
, dest = BufferList() | ||
const source = BufferList() | ||
const dest = BufferList() | ||
@@ -576,8 +612,9 @@ source.write('hello') | ||
tape('basic copy', function (t) { | ||
var buf = crypto.randomBytes(1024) | ||
, buf2 = Buffer.alloc(1024) | ||
, b = BufferList(buf) | ||
const buf = crypto.randomBytes(1024) | ||
const buf2 = Buffer.alloc(1024) | ||
const b = BufferList(buf) | ||
b.copy(buf2) | ||
t.equal(b.slice().toString('hex'), buf2.toString('hex'), 'same buffer') | ||
t.end() | ||
@@ -587,5 +624,5 @@ }) | ||
tape('copy after many appends', function (t) { | ||
var buf = crypto.randomBytes(512) | ||
, buf2 = Buffer.alloc(1024) | ||
, b = BufferList(buf) | ||
const buf = crypto.randomBytes(512) | ||
const buf2 = Buffer.alloc(1024) | ||
const b = BufferList(buf) | ||
@@ -595,2 +632,3 @@ b.append(buf) | ||
t.equal(b.slice().toString('hex'), buf2.toString('hex'), 'same buffer') | ||
t.end() | ||
@@ -600,8 +638,9 @@ }) | ||
tape('copy at a precise position', function (t) { | ||
var buf = crypto.randomBytes(1004) | ||
, buf2 = Buffer.alloc(1024) | ||
, b = BufferList(buf) | ||
const buf = crypto.randomBytes(1004) | ||
const buf2 = Buffer.alloc(1024) | ||
const b = BufferList(buf) | ||
b.copy(buf2, 20) | ||
t.equal(b.slice().toString('hex'), buf2.slice(20).toString('hex'), 'same buffer') | ||
t.end() | ||
@@ -611,8 +650,9 @@ }) | ||
tape('copy starting from a precise location', function (t) { | ||
var buf = crypto.randomBytes(10) | ||
, buf2 = Buffer.alloc(5) | ||
, b = BufferList(buf) | ||
const buf = crypto.randomBytes(10) | ||
const buf2 = Buffer.alloc(5) | ||
const b = BufferList(buf) | ||
b.copy(buf2, 0, 5) | ||
t.equal(b.slice(5).toString('hex'), buf2.toString('hex'), 'same buffer') | ||
t.end() | ||
@@ -622,6 +662,6 @@ }) | ||
tape('copy in an interval', function (t) { | ||
var rnd = crypto.randomBytes(10) | ||
, b = BufferList(rnd) // put the random bytes there | ||
, actual = Buffer.alloc(3) | ||
, expected = Buffer.alloc(3) | ||
const rnd = crypto.randomBytes(10) | ||
const b = BufferList(rnd) // put the random bytes there | ||
const actual = Buffer.alloc(3) | ||
const expected = Buffer.alloc(3) | ||
@@ -632,2 +672,3 @@ rnd.copy(expected, 0, 5, 8) | ||
t.equal(actual.toString('hex'), expected.toString('hex'), 'same buffer') | ||
t.end() | ||
@@ -637,5 +678,5 @@ }) | ||
tape('copy an interval between two buffers', function (t) { | ||
var buf = crypto.randomBytes(10) | ||
, buf2 = Buffer.alloc(10) | ||
, b = BufferList(buf) | ||
const buf = crypto.randomBytes(10) | ||
const buf2 = Buffer.alloc(10) | ||
const b = BufferList(buf) | ||
@@ -646,2 +687,3 @@ b.append(buf) | ||
t.equal(b.slice(5, 15).toString('hex'), buf2.toString('hex'), 'same buffer') | ||
t.end() | ||
@@ -651,5 +693,6 @@ }) | ||
tape('shallow slice across buffer boundaries', function (t) { | ||
var bl = new BufferList(['First', 'Second', 'Third']) | ||
const bl = new BufferList(['First', 'Second', 'Third']) | ||
t.equal(bl.shallowSlice(3, 13).toString(), 'stSecondTh') | ||
t.end() | ||
@@ -660,6 +703,8 @@ }) | ||
t.plan(2) | ||
var bl = new BufferList(['First', 'Second', 'Third']) | ||
const bl = new BufferList(['First', 'Second', 'Third']) | ||
t.equal(bl.shallowSlice(5, 10).toString(), 'Secon') | ||
t.equal(bl.shallowSlice(7, 10).toString(), 'con') | ||
t.end() | ||
@@ -670,4 +715,5 @@ }) | ||
t.plan(3) | ||
var bl = new BufferList(['First', 'Second', 'Third']) | ||
const bl = new BufferList(['First', 'Second', 'Third']) | ||
t.equal(bl.shallowSlice(0, 5).toString(), 'First') | ||
@@ -680,4 +726,5 @@ t.equal(bl.shallowSlice(5, 11).toString(), 'Second') | ||
t.plan(4) | ||
var bl = new BufferList(['First', 'Second', 'Third']) | ||
const bl = new BufferList(['First', 'Second', 'Third']) | ||
t.equal(bl.shallowSlice().toString(), 'FirstSecondThird') | ||
@@ -691,5 +738,6 @@ t.equal(bl.shallowSlice(5).toString(), 'SecondThird') | ||
t.plan(1) | ||
var buffers = [Buffer.from('First'), Buffer.from('Second'), Buffer.from('Third')] | ||
var bl = (new BufferList(buffers)).shallowSlice(5, -3) | ||
const buffers = [Buffer.from('First'), Buffer.from('Second'), Buffer.from('Third')] | ||
const bl = (new BufferList(buffers)).shallowSlice(5, -3) | ||
buffers[1].fill('h') | ||
@@ -703,4 +751,6 @@ buffers[2].fill('h') | ||
t.plan(1) | ||
var buffers = [Buffer.from('First'), Buffer.from('Second'), Buffer.from('Third')] | ||
var bl = (new BufferList(buffers)).shallowSlice(0, 0) | ||
const buffers = [Buffer.from('First'), Buffer.from('Second'), Buffer.from('Third')] | ||
const bl = (new BufferList(buffers)).shallowSlice(0, 0) | ||
t.equal(bl.length, 0) | ||
@@ -711,4 +761,6 @@ }) | ||
t.plan(1) | ||
var buffers = [Buffer.from('First'), Buffer.from('Second'), Buffer.from('Third')] | ||
var bl = (new BufferList(buffers)).shallowSlice(10, 10) | ||
const buffers = [Buffer.from('First'), Buffer.from('Second'), Buffer.from('Third')] | ||
const bl = (new BufferList(buffers)).shallowSlice(10, 10) | ||
t.equal(bl.length, 0) | ||
@@ -720,4 +772,4 @@ }) | ||
var bl = new BufferList('abcdefghij\xff\x00') | ||
, dup = bl.duplicate() | ||
const bl = new BufferList('abcdefghij\xff\x00') | ||
const dup = bl.duplicate() | ||
@@ -731,3 +783,4 @@ t.equal(bl.prototype, dup.prototype) | ||
var bl = new BufferList('alsdkfja;lsdkfja;lsdk') | ||
const bl = new BufferList('alsdkfja;lsdkfja;lsdk') | ||
bl.destroy() | ||
@@ -742,4 +795,5 @@ | ||
var bl = new BufferList('alsdkfja;lsdkfja;lsdk') | ||
var err = new Error('kaboom') | ||
const bl = new BufferList('alsdkfja;lsdkfja;lsdk') | ||
const err = new Error('kaboom') | ||
bl.destroy(err) | ||
@@ -757,4 +811,4 @@ bl.on('error', function (_err) { | ||
var bl = new BufferList() | ||
fs.createReadStream(__dirname + '/test.js') | ||
const bl = new BufferList() | ||
fs.createReadStream(path.join(__dirname, '/test.js')) | ||
.pipe(bl) | ||
@@ -766,3 +820,2 @@ | ||
t.equal(bl.length, 0) | ||
}) | ||
@@ -773,4 +826,5 @@ | ||
var bl = new BufferList() | ||
fs.createReadStream(__dirname + '/test.js') | ||
const bl = new BufferList() | ||
fs.createReadStream(path.join(__dirname, '/test.js')) | ||
.pipe(bl) | ||
@@ -790,4 +844,5 @@ | ||
var bl = new BufferList() | ||
fs.createReadStream(__dirname + '/test.js') | ||
const bl = new BufferList() | ||
fs.createReadStream(path.join(__dirname, '/test.js')) | ||
.on('end', onEnd) | ||
@@ -807,6 +862,6 @@ .pipe(bl) | ||
var bl = new BufferList() | ||
, ds = new BufferList() | ||
const bl = new BufferList() | ||
const ds = new BufferList() | ||
fs.createReadStream(__dirname + '/test.js') | ||
fs.createReadStream(path.join(__dirname, '/test.js')) | ||
.on('end', onEnd) | ||
@@ -828,3 +883,2 @@ .pipe(bl) | ||
t.equals(bl.length, 0) | ||
}, 100) | ||
@@ -836,2 +890,3 @@ } | ||
t.plan(2) | ||
fs.createReadStream('/does/not/exist').pipe(BufferList(function (err, data) { | ||
@@ -838,0 +893,0 @@ t.ok(err instanceof Error, 'has error') |
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
62912
10
1460
248
Updatedreadable-stream@^3.4.0