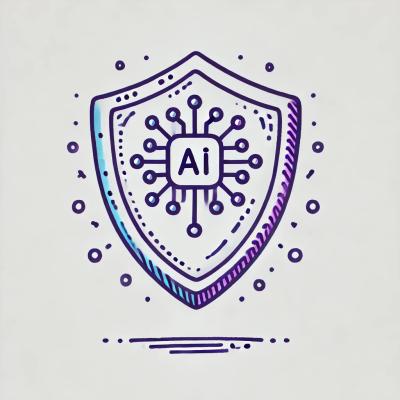
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
boundless-checkbox-group
Advanced tools
A controller view for managing the aggregate state of multiple, related checkboxes.
The most common use case for CheckboxGroup
is a "select all" / children scenario. This particular
configuration is built-in and is activated by passing the selectAll
prop.
npm i boundless-checkbox-group --save
Then use it like:
/** @jsx createElement */
import { createElement, PureComponent } from 'react';
import CheckboxGroup from 'boundless-checkbox-group';
import { filter, map, merge, some } from 'lodash';
export default class CheckboxGroupDemo extends PureComponent {
state = {
items: [ {
inputProps: {
checked: false,
name: 'likes-science',
},
label: 'Science',
}, {
inputProps: {
checked: false,
name: 'likes-math',
},
label: 'Mathematics',
}, {
inputProps: {
checked: false,
name: 'likes-tech',
},
label: 'Technology',
}, {
inputProps: {
checked: false,
name: 'likes-art',
},
label: 'Art',
}, {
inputProps: {
checked: false,
name: 'likes-sports',
},
label: 'Sports',
} ],
}
mutateAll(delta) {
this.setState({
items: map(this.state.items, function transformer(item) {
return merge({}, item, delta);
}),
});
}
mutateOne(name, delta) {
this.setState({
items: map(this.state.items, function transformer(item) {
if (item.inputProps.name !== name) {
return item;
}
return merge({}, item, delta);
}),
});
}
handleAllChecked = () => {
this.mutateAll({ inputProps: { checked: true } });
}
handleAllUnchecked = () => {
this.mutateAll({ inputProps: { checked: false } });
}
handleChildChecked = (name) => {
this.mutateOne(name, { inputProps: { checked: true } });
}
handleChildUnchecked = (name) => {
this.mutateOne(name, { inputProps: { checked: false } });
}
renderFeedback() {
if (some(this.state.items, { inputProps: { checked: true } })) {
const liked = map(filter(this.state.items, { inputProps: { checked: true } }), 'label');
const lastIndex = liked.length - 1;
return (
<p>You said you like: {liked.length === 1 ? liked[0] : [ liked.slice(0, lastIndex).join(', '), 'and', liked.slice(lastIndex) ].join(' ')}.</p>
);
}
}
render() {
return (
<div>
<p>What subjects are you interested in?</p>
<CheckboxGroup
className='checkbox-group-demo'
items={this.state.items}
selectAll={CheckboxGroup.selectAll.AFTER}
selectAllProps={{ label: 'All of the above' }}
onAllChecked={this.handleAllChecked}
onAllUnchecked={this.handleAllUnchecked}
onChildChecked={this.handleChildChecked}
onChildUnchecked={this.handleChildUnchecked} />
<br />
{this.renderFeedback()}
</div>
);
}
}
CheckboxGroup can also just be directly used from the main Boundless library. This is recommended when you're getting started to avoid maintaining the package versions of several components:
npm i boundless --save
the ES6 import
statement then becomes like:
import { CheckboxGroup } from 'boundless';
Note: only top-level props are in the README, for the full list check out the website.
items
· the data wished to be rendered, each item must conform to the Checkbox prop spec
Expects | Default Value |
---|---|
array | [] |
*
· any React-supported attribute
Expects | Default Value |
---|---|
any | n/a |
component
· override the wrapper HTML element if desired
Expects | Default Value |
---|---|
string | 'div' |
onAllChecked
· called when all children become checked (not fired on first render), no return
Expects | Default Value |
---|---|
function | () => {} |
onAllUnchecked
· called when all children become unchecked (not fired on first render), no return
Expects | Default Value |
---|---|
function | () => {} |
onChildChecked
· called when a specific child has become checked, returns the child definition
Expects | Default Value |
---|---|
function | () => {} |
onChildUnchecked
· called when a specific child has become checked, returns the child definition
Expects | Default Value |
---|---|
function | () => {} |
selectAll
· renders a master checkbox that can manipulate the values of all children simultaneously
Expects | Default Value |
---|---|
CheckboxGroup.selectAll.BEFORE or CheckboxGroup.selectAll.AFTER or CheckboxGroup.selectAll.NONE | CheckboxGroup.selectAll.BEFORE |
selectAllProps
· must conform to the Checkbox prop spec
Expects | Default Value |
---|---|
object | {} |
You can see what variables are available to override in variables.styl.
// Redefine any variables as desired, e.g:
color-accent = royalblue
// Bring in the component styles; they will be autoconfigured based on the above
@require "node_modules/boundless-checkbox-group/style"
If desired, a precompiled plain CSS stylesheet is available for customization at /build/style.css
, based on Boundless's default variables.
FAQs
A controller view for managing the aggregate state of multiple, related checkboxes.
The npm package boundless-checkbox-group receives a total of 0 weekly downloads. As such, boundless-checkbox-group popularity was classified as not popular.
We found that boundless-checkbox-group demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.