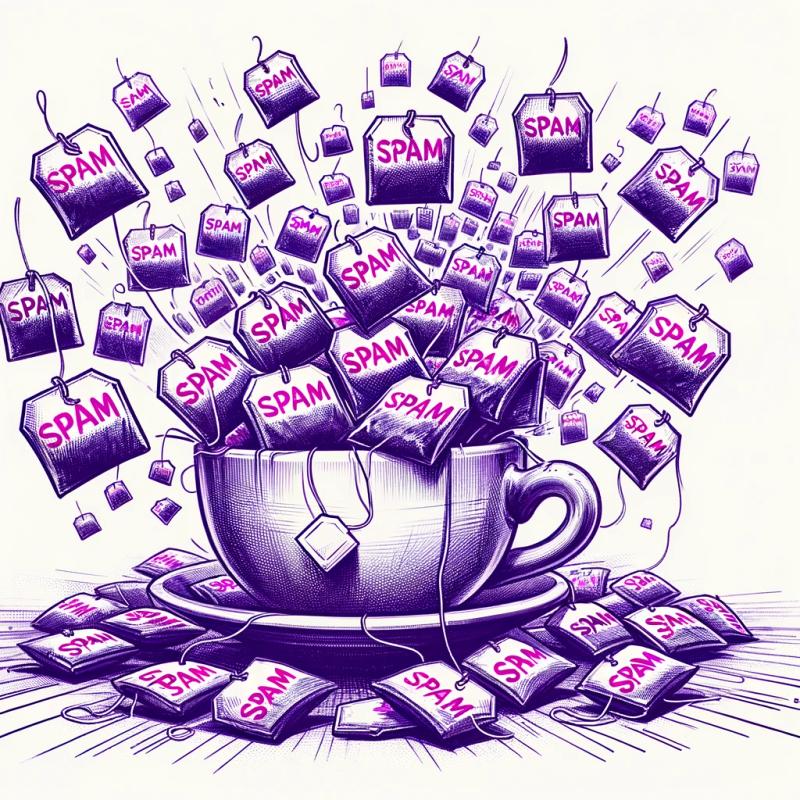
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
broccoli-file-creator
Advanced tools
Readme
Create a file named app/main.js
with "some content goes here":
let writeFile = require('broccoli-file-creator');
let tree = writeFile('/app/main.js', 'some content goes here');
writeFile(filename, content, fileOptions)
filename
{String}
The path of the file to create.
content
{String|Function|Promise}
The contents to write into the file.
writeFile('filename.txt', 'the-content');
writeFile('filename.txt', Promise.resolve('the-content'));
writeFile('filename.txt', () => 'the-content');
writeFile('filename.txt', () => Promise.resolve('the-content'));
note: If a function is provided, it will only be invoked once, on first build
I know, right?
Running the tests:
npm install
npm test
This project is distributed under the MIT license.
FAQs
Broccoli plugin to create a file.
The npm package broccoli-file-creator receives a total of 179,801 weekly downloads. As such, broccoli-file-creator popularity was classified as popular.
We found that broccoli-file-creator demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.