Comparing version 3.0.3 to 3.1.0
@@ -38,6 +38,7 @@ #!/usr/bin/env node | ||
var path | ||
if (file.name !== 'test-buffer-iterator.js') | ||
if (file.name !== 'test-buffer-iterator.js') { | ||
path = __dirname + '/../test/node/' + file.name | ||
else | ||
} else { | ||
path = __dirname + '/../test/es6/' + file.name | ||
} | ||
@@ -64,3 +65,3 @@ hyperquest(file.download_url, httpOpts) | ||
// comment out require('common') | ||
line = line.replace(/(var common = require.*)/, '// $1') | ||
line = line.replace(/(var common = require.*)/, 'var common = {};') | ||
@@ -67,0 +68,0 @@ // require browser buffer |
436
index.js
@@ -68,9 +68,7 @@ /*! | ||
function Buffer (subject, encoding, noZero) { | ||
if (!(this instanceof Buffer)) | ||
return new Buffer(subject, encoding, noZero) | ||
if (!(this instanceof Buffer)) return new Buffer(subject, encoding, noZero) | ||
var type = typeof subject | ||
var length | ||
// Find the length | ||
var length | ||
if (type === 'number') { | ||
@@ -80,5 +78,5 @@ length = +subject | ||
length = Buffer.byteLength(subject, encoding) | ||
} else if (type === 'object' && subject !== null) { // assume object is array-like | ||
if (subject.type === 'Buffer' && isArray(subject.data)) | ||
subject = subject.data | ||
} else if (type === 'object' && subject !== null) { | ||
// assume object is array-like | ||
if (subject.type === 'Buffer' && isArray(subject.data)) subject = subject.data | ||
length = +subject.length | ||
@@ -89,10 +87,9 @@ } else { | ||
if (length > kMaxLength) | ||
throw new RangeError('Attempt to allocate Buffer larger than maximum ' + | ||
'size: 0x' + kMaxLength.toString(16) + ' bytes') | ||
if (length > kMaxLength) { | ||
throw new RangeError('Attempt to allocate Buffer larger than maximum size: 0x' + | ||
kMaxLength.toString(16) + ' bytes') | ||
} | ||
if (length < 0) | ||
length = 0 | ||
else | ||
length >>>= 0 // Coerce to uint32. | ||
if (length < 0) length = 0 | ||
else length >>>= 0 // coerce to uint32 | ||
@@ -118,7 +115,9 @@ var self = this | ||
if (Buffer.isBuffer(subject)) { | ||
for (i = 0; i < length; i++) | ||
for (i = 0; i < length; i++) { | ||
self[i] = subject.readUInt8(i) | ||
} | ||
} else { | ||
for (i = 0; i < length; i++) | ||
for (i = 0; i < length; i++) { | ||
self[i] = ((subject[i] % 256) + 256) % 256 | ||
} | ||
} | ||
@@ -133,4 +132,3 @@ } else if (type === 'string') { | ||
if (length > 0 && length <= Buffer.poolSize) | ||
self.parent = rootParent | ||
if (length > 0 && length <= Buffer.poolSize) self.parent = rootParent | ||
@@ -141,4 +139,3 @@ return self | ||
function SlowBuffer (subject, encoding, noZero) { | ||
if (!(this instanceof SlowBuffer)) | ||
return new SlowBuffer(subject, encoding, noZero) | ||
if (!(this instanceof SlowBuffer)) return new SlowBuffer(subject, encoding, noZero) | ||
@@ -150,9 +147,10 @@ var buf = new Buffer(subject, encoding, noZero) | ||
Buffer.isBuffer = function (b) { | ||
Buffer.isBuffer = function isBuffer (b) { | ||
return !!(b != null && b._isBuffer) | ||
} | ||
Buffer.compare = function (a, b) { | ||
if (!Buffer.isBuffer(a) || !Buffer.isBuffer(b)) | ||
Buffer.compare = function compare (a, b) { | ||
if (!Buffer.isBuffer(a) || !Buffer.isBuffer(b)) { | ||
throw new TypeError('Arguments must be Buffers') | ||
} | ||
@@ -173,3 +171,3 @@ if (a === b) return 0 | ||
Buffer.isEncoding = function (encoding) { | ||
Buffer.isEncoding = function isEncoding (encoding) { | ||
switch (String(encoding).toLowerCase()) { | ||
@@ -193,3 +191,3 @@ case 'hex': | ||
Buffer.concat = function (list, totalLength) { | ||
Buffer.concat = function concat (list, totalLength) { | ||
if (!isArray(list)) throw new TypeError('Usage: Buffer.concat(list[, length])') | ||
@@ -221,3 +219,3 @@ | ||
Buffer.byteLength = function (str, encoding) { | ||
Buffer.byteLength = function byteLength (str, encoding) { | ||
var ret | ||
@@ -258,3 +256,3 @@ str = str + '' | ||
// toString(encoding, start=0, end=buffer.length) | ||
Buffer.prototype.toString = function (encoding, start, end) { | ||
Buffer.prototype.toString = function toString (encoding, start, end) { | ||
var loweredCase = false | ||
@@ -295,4 +293,3 @@ | ||
default: | ||
if (loweredCase) | ||
throw new TypeError('Unknown encoding: ' + encoding) | ||
if (loweredCase) throw new TypeError('Unknown encoding: ' + encoding) | ||
encoding = (encoding + '').toLowerCase() | ||
@@ -304,3 +301,3 @@ loweredCase = true | ||
Buffer.prototype.equals = function (b) { | ||
Buffer.prototype.equals = function equals (b) { | ||
if (!Buffer.isBuffer(b)) throw new TypeError('Argument must be a Buffer') | ||
@@ -311,3 +308,3 @@ if (this === b) return true | ||
Buffer.prototype.inspect = function () { | ||
Buffer.prototype.inspect = function inspect () { | ||
var str = '' | ||
@@ -317,4 +314,3 @@ var max = exports.INSPECT_MAX_BYTES | ||
str = this.toString('hex', 0, max).match(/.{2}/g).join(' ') | ||
if (this.length > max) | ||
str += ' ... ' | ||
if (this.length > max) str += ' ... ' | ||
} | ||
@@ -324,3 +320,3 @@ return '<Buffer ' + str + '>' | ||
Buffer.prototype.compare = function (b) { | ||
Buffer.prototype.compare = function compare (b) { | ||
if (!Buffer.isBuffer(b)) throw new TypeError('Argument must be a Buffer') | ||
@@ -331,4 +327,45 @@ if (this === b) return 0 | ||
Buffer.prototype.indexOf = function indexOf (val, byteOffset) { | ||
if (byteOffset > 0x7fffffff) byteOffset = 0x7fffffff | ||
else if (byteOffset < -0x80000000) byteOffset = -0x80000000 | ||
byteOffset >>= 0 | ||
if (this.length === 0) return -1 | ||
if (byteOffset >= this.length) return -1 | ||
// Negative offsets start from the end of the buffer | ||
if (byteOffset < 0) byteOffset = Math.max(this.length + byteOffset, 0) | ||
if (typeof val === 'string') { | ||
if (val.length === 0) return -1 // special case: looking for empty string always fails | ||
return String.prototype.indexOf.call(this, val, byteOffset) | ||
} | ||
if (Buffer.isBuffer(val)) { | ||
return arrayIndexOf(this, val, byteOffset) | ||
} | ||
if (typeof val === 'number') { | ||
if (Buffer.TYPED_ARRAY_SUPPORT && Uint8Array.prototype.indexOf === 'function') { | ||
return Uint8Array.prototype.indexOf.call(this, val, byteOffset) | ||
} | ||
return arrayIndexOf(this, [ val ], byteOffset) | ||
} | ||
function arrayIndexOf (arr, val, byteOffset) { | ||
var foundIndex = -1 | ||
for (var i = 0; byteOffset + i < arr.length; i++) { | ||
if (arr[byteOffset + i] === val[foundIndex === -1 ? 0 : i - foundIndex]) { | ||
if (foundIndex === -1) foundIndex = i | ||
if (i - foundIndex + 1 === val.length) return byteOffset + foundIndex | ||
} else { | ||
foundIndex = -1 | ||
} | ||
} | ||
return -1 | ||
} | ||
throw new TypeError('val must be string, number or Buffer') | ||
} | ||
// `get` will be removed in Node 0.13+ | ||
Buffer.prototype.get = function (offset) { | ||
Buffer.prototype.get = function get (offset) { | ||
console.log('.get() is deprecated. Access using array indexes instead.') | ||
@@ -339,3 +376,3 @@ return this.readUInt8(offset) | ||
// `set` will be removed in Node 0.13+ | ||
Buffer.prototype.set = function (v, offset) { | ||
Buffer.prototype.set = function set (v, offset) { | ||
console.log('.set() is deprecated. Access using array indexes instead.') | ||
@@ -365,5 +402,5 @@ return this.writeUInt8(v, offset) | ||
for (var i = 0; i < length; i++) { | ||
var byte = parseInt(string.substr(i * 2, 2), 16) | ||
if (isNaN(byte)) throw new Error('Invalid hex string') | ||
buf[offset + i] = byte | ||
var parsed = parseInt(string.substr(i * 2, 2), 16) | ||
if (isNaN(parsed)) throw new Error('Invalid hex string') | ||
buf[offset + i] = parsed | ||
} | ||
@@ -397,3 +434,3 @@ return i | ||
Buffer.prototype.write = function (string, offset, length, encoding) { | ||
Buffer.prototype.write = function write (string, offset, length, encoding) { | ||
// Support both (string, offset, length, encoding) | ||
@@ -415,4 +452,5 @@ // and the legacy (string, encoding, offset, length) | ||
if (length < 0 || offset < 0 || offset > this.length) | ||
if (length < 0 || offset < 0 || offset > this.length) { | ||
throw new RangeError('attempt to write outside buffer bounds') | ||
} | ||
@@ -460,3 +498,3 @@ var remaining = this.length - offset | ||
Buffer.prototype.toJSON = function () { | ||
Buffer.prototype.toJSON = function toJSON () { | ||
return { | ||
@@ -535,3 +573,3 @@ type: 'Buffer', | ||
Buffer.prototype.slice = function (start, end) { | ||
Buffer.prototype.slice = function slice (start, end) { | ||
var len = this.length | ||
@@ -543,4 +581,3 @@ start = ~~start | ||
start += len | ||
if (start < 0) | ||
start = 0 | ||
if (start < 0) start = 0 | ||
} else if (start > len) { | ||
@@ -552,4 +589,3 @@ start = len | ||
end += len | ||
if (end < 0) | ||
end = 0 | ||
if (end < 0) end = 0 | ||
} else if (end > len) { | ||
@@ -559,4 +595,3 @@ end = len | ||
if (end < start) | ||
end = start | ||
if (end < start) end = start | ||
@@ -574,4 +609,3 @@ var newBuf | ||
if (newBuf.length) | ||
newBuf.parent = this.parent || this | ||
if (newBuf.length) newBuf.parent = this.parent || this | ||
@@ -585,13 +619,10 @@ return newBuf | ||
function checkOffset (offset, ext, length) { | ||
if ((offset % 1) !== 0 || offset < 0) | ||
throw new RangeError('offset is not uint') | ||
if (offset + ext > length) | ||
throw new RangeError('Trying to access beyond buffer length') | ||
if ((offset % 1) !== 0 || offset < 0) throw new RangeError('offset is not uint') | ||
if (offset + ext > length) throw new RangeError('Trying to access beyond buffer length') | ||
} | ||
Buffer.prototype.readUIntLE = function (offset, byteLength, noAssert) { | ||
Buffer.prototype.readUIntLE = function readUIntLE (offset, byteLength, noAssert) { | ||
offset = offset >>> 0 | ||
byteLength = byteLength >>> 0 | ||
if (!noAssert) | ||
checkOffset(offset, byteLength, this.length) | ||
if (!noAssert) checkOffset(offset, byteLength, this.length) | ||
@@ -601,4 +632,5 @@ var val = this[offset] | ||
var i = 0 | ||
while (++i < byteLength && (mul *= 0x100)) | ||
while (++i < byteLength && (mul *= 0x100)) { | ||
val += this[offset + i] * mul | ||
} | ||
@@ -608,12 +640,14 @@ return val | ||
Buffer.prototype.readUIntBE = function (offset, byteLength, noAssert) { | ||
Buffer.prototype.readUIntBE = function readUIntBE (offset, byteLength, noAssert) { | ||
offset = offset >>> 0 | ||
byteLength = byteLength >>> 0 | ||
if (!noAssert) | ||
if (!noAssert) { | ||
checkOffset(offset, byteLength, this.length) | ||
} | ||
var val = this[offset + --byteLength] | ||
var mul = 1 | ||
while (byteLength > 0 && (mul *= 0x100)) | ||
while (byteLength > 0 && (mul *= 0x100)) { | ||
val += this[offset + --byteLength] * mul | ||
} | ||
@@ -623,23 +657,19 @@ return val | ||
Buffer.prototype.readUInt8 = function (offset, noAssert) { | ||
if (!noAssert) | ||
checkOffset(offset, 1, this.length) | ||
Buffer.prototype.readUInt8 = function readUInt8 (offset, noAssert) { | ||
if (!noAssert) checkOffset(offset, 1, this.length) | ||
return this[offset] | ||
} | ||
Buffer.prototype.readUInt16LE = function (offset, noAssert) { | ||
if (!noAssert) | ||
checkOffset(offset, 2, this.length) | ||
Buffer.prototype.readUInt16LE = function readUInt16LE (offset, noAssert) { | ||
if (!noAssert) checkOffset(offset, 2, this.length) | ||
return this[offset] | (this[offset + 1] << 8) | ||
} | ||
Buffer.prototype.readUInt16BE = function (offset, noAssert) { | ||
if (!noAssert) | ||
checkOffset(offset, 2, this.length) | ||
Buffer.prototype.readUInt16BE = function readUInt16BE (offset, noAssert) { | ||
if (!noAssert) checkOffset(offset, 2, this.length) | ||
return (this[offset] << 8) | this[offset + 1] | ||
} | ||
Buffer.prototype.readUInt32LE = function (offset, noAssert) { | ||
if (!noAssert) | ||
checkOffset(offset, 4, this.length) | ||
Buffer.prototype.readUInt32LE = function readUInt32LE (offset, noAssert) { | ||
if (!noAssert) checkOffset(offset, 4, this.length) | ||
@@ -652,17 +682,15 @@ return ((this[offset]) | | ||
Buffer.prototype.readUInt32BE = function (offset, noAssert) { | ||
if (!noAssert) | ||
checkOffset(offset, 4, this.length) | ||
Buffer.prototype.readUInt32BE = function readUInt32BE (offset, noAssert) { | ||
if (!noAssert) checkOffset(offset, 4, this.length) | ||
return (this[offset] * 0x1000000) + | ||
((this[offset + 1] << 16) | | ||
(this[offset + 2] << 8) | | ||
this[offset + 3]) | ||
((this[offset + 1] << 16) | | ||
(this[offset + 2] << 8) | | ||
this[offset + 3]) | ||
} | ||
Buffer.prototype.readIntLE = function (offset, byteLength, noAssert) { | ||
Buffer.prototype.readIntLE = function readIntLE (offset, byteLength, noAssert) { | ||
offset = offset >>> 0 | ||
byteLength = byteLength >>> 0 | ||
if (!noAssert) | ||
checkOffset(offset, byteLength, this.length) | ||
if (!noAssert) checkOffset(offset, byteLength, this.length) | ||
@@ -672,8 +700,8 @@ var val = this[offset] | ||
var i = 0 | ||
while (++i < byteLength && (mul *= 0x100)) | ||
while (++i < byteLength && (mul *= 0x100)) { | ||
val += this[offset + i] * mul | ||
} | ||
mul *= 0x80 | ||
if (val >= mul) | ||
val -= Math.pow(2, 8 * byteLength) | ||
if (val >= mul) val -= Math.pow(2, 8 * byteLength) | ||
@@ -683,7 +711,6 @@ return val | ||
Buffer.prototype.readIntBE = function (offset, byteLength, noAssert) { | ||
Buffer.prototype.readIntBE = function readIntBE (offset, byteLength, noAssert) { | ||
offset = offset >>> 0 | ||
byteLength = byteLength >>> 0 | ||
if (!noAssert) | ||
checkOffset(offset, byteLength, this.length) | ||
if (!noAssert) checkOffset(offset, byteLength, this.length) | ||
@@ -693,8 +720,8 @@ var i = byteLength | ||
var val = this[offset + --i] | ||
while (i > 0 && (mul *= 0x100)) | ||
while (i > 0 && (mul *= 0x100)) { | ||
val += this[offset + --i] * mul | ||
} | ||
mul *= 0x80 | ||
if (val >= mul) | ||
val -= Math.pow(2, 8 * byteLength) | ||
if (val >= mul) val -= Math.pow(2, 8 * byteLength) | ||
@@ -704,13 +731,10 @@ return val | ||
Buffer.prototype.readInt8 = function (offset, noAssert) { | ||
if (!noAssert) | ||
checkOffset(offset, 1, this.length) | ||
if (!(this[offset] & 0x80)) | ||
return (this[offset]) | ||
Buffer.prototype.readInt8 = function readInt8 (offset, noAssert) { | ||
if (!noAssert) checkOffset(offset, 1, this.length) | ||
if (!(this[offset] & 0x80)) return (this[offset]) | ||
return ((0xff - this[offset] + 1) * -1) | ||
} | ||
Buffer.prototype.readInt16LE = function (offset, noAssert) { | ||
if (!noAssert) | ||
checkOffset(offset, 2, this.length) | ||
Buffer.prototype.readInt16LE = function readInt16LE (offset, noAssert) { | ||
if (!noAssert) checkOffset(offset, 2, this.length) | ||
var val = this[offset] | (this[offset + 1] << 8) | ||
@@ -720,5 +744,4 @@ return (val & 0x8000) ? val | 0xFFFF0000 : val | ||
Buffer.prototype.readInt16BE = function (offset, noAssert) { | ||
if (!noAssert) | ||
checkOffset(offset, 2, this.length) | ||
Buffer.prototype.readInt16BE = function readInt16BE (offset, noAssert) { | ||
if (!noAssert) checkOffset(offset, 2, this.length) | ||
var val = this[offset + 1] | (this[offset] << 8) | ||
@@ -728,43 +751,37 @@ return (val & 0x8000) ? val | 0xFFFF0000 : val | ||
Buffer.prototype.readInt32LE = function (offset, noAssert) { | ||
if (!noAssert) | ||
checkOffset(offset, 4, this.length) | ||
Buffer.prototype.readInt32LE = function readInt32LE (offset, noAssert) { | ||
if (!noAssert) checkOffset(offset, 4, this.length) | ||
return (this[offset]) | | ||
(this[offset + 1] << 8) | | ||
(this[offset + 2] << 16) | | ||
(this[offset + 3] << 24) | ||
(this[offset + 1] << 8) | | ||
(this[offset + 2] << 16) | | ||
(this[offset + 3] << 24) | ||
} | ||
Buffer.prototype.readInt32BE = function (offset, noAssert) { | ||
if (!noAssert) | ||
checkOffset(offset, 4, this.length) | ||
Buffer.prototype.readInt32BE = function readInt32BE (offset, noAssert) { | ||
if (!noAssert) checkOffset(offset, 4, this.length) | ||
return (this[offset] << 24) | | ||
(this[offset + 1] << 16) | | ||
(this[offset + 2] << 8) | | ||
(this[offset + 3]) | ||
(this[offset + 1] << 16) | | ||
(this[offset + 2] << 8) | | ||
(this[offset + 3]) | ||
} | ||
Buffer.prototype.readFloatLE = function (offset, noAssert) { | ||
if (!noAssert) | ||
checkOffset(offset, 4, this.length) | ||
Buffer.prototype.readFloatLE = function readFloatLE (offset, noAssert) { | ||
if (!noAssert) checkOffset(offset, 4, this.length) | ||
return ieee754.read(this, offset, true, 23, 4) | ||
} | ||
Buffer.prototype.readFloatBE = function (offset, noAssert) { | ||
if (!noAssert) | ||
checkOffset(offset, 4, this.length) | ||
Buffer.prototype.readFloatBE = function readFloatBE (offset, noAssert) { | ||
if (!noAssert) checkOffset(offset, 4, this.length) | ||
return ieee754.read(this, offset, false, 23, 4) | ||
} | ||
Buffer.prototype.readDoubleLE = function (offset, noAssert) { | ||
if (!noAssert) | ||
checkOffset(offset, 8, this.length) | ||
Buffer.prototype.readDoubleLE = function readDoubleLE (offset, noAssert) { | ||
if (!noAssert) checkOffset(offset, 8, this.length) | ||
return ieee754.read(this, offset, true, 52, 8) | ||
} | ||
Buffer.prototype.readDoubleBE = function (offset, noAssert) { | ||
if (!noAssert) | ||
checkOffset(offset, 8, this.length) | ||
Buffer.prototype.readDoubleBE = function readDoubleBE (offset, noAssert) { | ||
if (!noAssert) checkOffset(offset, 8, this.length) | ||
return ieee754.read(this, offset, false, 52, 8) | ||
@@ -779,8 +796,7 @@ } | ||
Buffer.prototype.writeUIntLE = function (value, offset, byteLength, noAssert) { | ||
Buffer.prototype.writeUIntLE = function writeUIntLE (value, offset, byteLength, noAssert) { | ||
value = +value | ||
offset = offset >>> 0 | ||
byteLength = byteLength >>> 0 | ||
if (!noAssert) | ||
checkInt(this, value, offset, byteLength, Math.pow(2, 8 * byteLength), 0) | ||
if (!noAssert) checkInt(this, value, offset, byteLength, Math.pow(2, 8 * byteLength), 0) | ||
@@ -790,4 +806,5 @@ var mul = 1 | ||
this[offset] = value & 0xFF | ||
while (++i < byteLength && (mul *= 0x100)) | ||
while (++i < byteLength && (mul *= 0x100)) { | ||
this[offset + i] = (value / mul) >>> 0 & 0xFF | ||
} | ||
@@ -797,8 +814,7 @@ return offset + byteLength | ||
Buffer.prototype.writeUIntBE = function (value, offset, byteLength, noAssert) { | ||
Buffer.prototype.writeUIntBE = function writeUIntBE (value, offset, byteLength, noAssert) { | ||
value = +value | ||
offset = offset >>> 0 | ||
byteLength = byteLength >>> 0 | ||
if (!noAssert) | ||
checkInt(this, value, offset, byteLength, Math.pow(2, 8 * byteLength), 0) | ||
if (!noAssert) checkInt(this, value, offset, byteLength, Math.pow(2, 8 * byteLength), 0) | ||
@@ -808,4 +824,5 @@ var i = byteLength - 1 | ||
this[offset + i] = value & 0xFF | ||
while (--i >= 0 && (mul *= 0x100)) | ||
while (--i >= 0 && (mul *= 0x100)) { | ||
this[offset + i] = (value / mul) >>> 0 & 0xFF | ||
} | ||
@@ -815,7 +832,6 @@ return offset + byteLength | ||
Buffer.prototype.writeUInt8 = function (value, offset, noAssert) { | ||
Buffer.prototype.writeUInt8 = function writeUInt8 (value, offset, noAssert) { | ||
value = +value | ||
offset = offset >>> 0 | ||
if (!noAssert) | ||
checkInt(this, value, offset, 1, 0xff, 0) | ||
if (!noAssert) checkInt(this, value, offset, 1, 0xff, 0) | ||
if (!Buffer.TYPED_ARRAY_SUPPORT) value = Math.floor(value) | ||
@@ -834,23 +850,25 @@ this[offset] = value | ||
Buffer.prototype.writeUInt16LE = function (value, offset, noAssert) { | ||
Buffer.prototype.writeUInt16LE = function writeUInt16LE (value, offset, noAssert) { | ||
value = +value | ||
offset = offset >>> 0 | ||
if (!noAssert) | ||
checkInt(this, value, offset, 2, 0xffff, 0) | ||
if (!noAssert) checkInt(this, value, offset, 2, 0xffff, 0) | ||
if (Buffer.TYPED_ARRAY_SUPPORT) { | ||
this[offset] = value | ||
this[offset + 1] = (value >>> 8) | ||
} else objectWriteUInt16(this, value, offset, true) | ||
} else { | ||
objectWriteUInt16(this, value, offset, true) | ||
} | ||
return offset + 2 | ||
} | ||
Buffer.prototype.writeUInt16BE = function (value, offset, noAssert) { | ||
Buffer.prototype.writeUInt16BE = function writeUInt16BE (value, offset, noAssert) { | ||
value = +value | ||
offset = offset >>> 0 | ||
if (!noAssert) | ||
checkInt(this, value, offset, 2, 0xffff, 0) | ||
if (!noAssert) checkInt(this, value, offset, 2, 0xffff, 0) | ||
if (Buffer.TYPED_ARRAY_SUPPORT) { | ||
this[offset] = (value >>> 8) | ||
this[offset + 1] = value | ||
} else objectWriteUInt16(this, value, offset, false) | ||
} else { | ||
objectWriteUInt16(this, value, offset, false) | ||
} | ||
return offset + 2 | ||
@@ -866,7 +884,6 @@ } | ||
Buffer.prototype.writeUInt32LE = function (value, offset, noAssert) { | ||
Buffer.prototype.writeUInt32LE = function writeUInt32LE (value, offset, noAssert) { | ||
value = +value | ||
offset = offset >>> 0 | ||
if (!noAssert) | ||
checkInt(this, value, offset, 4, 0xffffffff, 0) | ||
if (!noAssert) checkInt(this, value, offset, 4, 0xffffffff, 0) | ||
if (Buffer.TYPED_ARRAY_SUPPORT) { | ||
@@ -877,11 +894,12 @@ this[offset + 3] = (value >>> 24) | ||
this[offset] = value | ||
} else objectWriteUInt32(this, value, offset, true) | ||
} else { | ||
objectWriteUInt32(this, value, offset, true) | ||
} | ||
return offset + 4 | ||
} | ||
Buffer.prototype.writeUInt32BE = function (value, offset, noAssert) { | ||
Buffer.prototype.writeUInt32BE = function writeUInt32BE (value, offset, noAssert) { | ||
value = +value | ||
offset = offset >>> 0 | ||
if (!noAssert) | ||
checkInt(this, value, offset, 4, 0xffffffff, 0) | ||
if (!noAssert) checkInt(this, value, offset, 4, 0xffffffff, 0) | ||
if (Buffer.TYPED_ARRAY_SUPPORT) { | ||
@@ -892,16 +910,17 @@ this[offset] = (value >>> 24) | ||
this[offset + 3] = value | ||
} else objectWriteUInt32(this, value, offset, false) | ||
} else { | ||
objectWriteUInt32(this, value, offset, false) | ||
} | ||
return offset + 4 | ||
} | ||
Buffer.prototype.writeIntLE = function (value, offset, byteLength, noAssert) { | ||
Buffer.prototype.writeIntLE = function writeIntLE (value, offset, byteLength, noAssert) { | ||
value = +value | ||
offset = offset >>> 0 | ||
if (!noAssert) { | ||
checkInt(this, | ||
value, | ||
offset, | ||
byteLength, | ||
Math.pow(2, 8 * byteLength - 1) - 1, | ||
-Math.pow(2, 8 * byteLength - 1)) | ||
checkInt( | ||
this, value, offset, byteLength, | ||
Math.pow(2, 8 * byteLength - 1) - 1, | ||
-Math.pow(2, 8 * byteLength - 1) | ||
) | ||
} | ||
@@ -913,4 +932,5 @@ | ||
this[offset] = value & 0xFF | ||
while (++i < byteLength && (mul *= 0x100)) | ||
while (++i < byteLength && (mul *= 0x100)) { | ||
this[offset + i] = ((value / mul) >> 0) - sub & 0xFF | ||
} | ||
@@ -920,12 +940,11 @@ return offset + byteLength | ||
Buffer.prototype.writeIntBE = function (value, offset, byteLength, noAssert) { | ||
Buffer.prototype.writeIntBE = function writeIntBE (value, offset, byteLength, noAssert) { | ||
value = +value | ||
offset = offset >>> 0 | ||
if (!noAssert) { | ||
checkInt(this, | ||
value, | ||
offset, | ||
byteLength, | ||
Math.pow(2, 8 * byteLength - 1) - 1, | ||
-Math.pow(2, 8 * byteLength - 1)) | ||
checkInt( | ||
this, value, offset, byteLength, | ||
Math.pow(2, 8 * byteLength - 1) - 1, | ||
-Math.pow(2, 8 * byteLength - 1) | ||
) | ||
} | ||
@@ -937,4 +956,5 @@ | ||
this[offset + i] = value & 0xFF | ||
while (--i >= 0 && (mul *= 0x100)) | ||
while (--i >= 0 && (mul *= 0x100)) { | ||
this[offset + i] = ((value / mul) >> 0) - sub & 0xFF | ||
} | ||
@@ -944,7 +964,6 @@ return offset + byteLength | ||
Buffer.prototype.writeInt8 = function (value, offset, noAssert) { | ||
Buffer.prototype.writeInt8 = function writeInt8 (value, offset, noAssert) { | ||
value = +value | ||
offset = offset >>> 0 | ||
if (!noAssert) | ||
checkInt(this, value, offset, 1, 0x7f, -0x80) | ||
if (!noAssert) checkInt(this, value, offset, 1, 0x7f, -0x80) | ||
if (!Buffer.TYPED_ARRAY_SUPPORT) value = Math.floor(value) | ||
@@ -956,31 +975,32 @@ if (value < 0) value = 0xff + value + 1 | ||
Buffer.prototype.writeInt16LE = function (value, offset, noAssert) { | ||
Buffer.prototype.writeInt16LE = function writeInt16LE (value, offset, noAssert) { | ||
value = +value | ||
offset = offset >>> 0 | ||
if (!noAssert) | ||
checkInt(this, value, offset, 2, 0x7fff, -0x8000) | ||
if (!noAssert) checkInt(this, value, offset, 2, 0x7fff, -0x8000) | ||
if (Buffer.TYPED_ARRAY_SUPPORT) { | ||
this[offset] = value | ||
this[offset + 1] = (value >>> 8) | ||
} else objectWriteUInt16(this, value, offset, true) | ||
} else { | ||
objectWriteUInt16(this, value, offset, true) | ||
} | ||
return offset + 2 | ||
} | ||
Buffer.prototype.writeInt16BE = function (value, offset, noAssert) { | ||
Buffer.prototype.writeInt16BE = function writeInt16BE (value, offset, noAssert) { | ||
value = +value | ||
offset = offset >>> 0 | ||
if (!noAssert) | ||
checkInt(this, value, offset, 2, 0x7fff, -0x8000) | ||
if (!noAssert) checkInt(this, value, offset, 2, 0x7fff, -0x8000) | ||
if (Buffer.TYPED_ARRAY_SUPPORT) { | ||
this[offset] = (value >>> 8) | ||
this[offset + 1] = value | ||
} else objectWriteUInt16(this, value, offset, false) | ||
} else { | ||
objectWriteUInt16(this, value, offset, false) | ||
} | ||
return offset + 2 | ||
} | ||
Buffer.prototype.writeInt32LE = function (value, offset, noAssert) { | ||
Buffer.prototype.writeInt32LE = function writeInt32LE (value, offset, noAssert) { | ||
value = +value | ||
offset = offset >>> 0 | ||
if (!noAssert) | ||
checkInt(this, value, offset, 4, 0x7fffffff, -0x80000000) | ||
if (!noAssert) checkInt(this, value, offset, 4, 0x7fffffff, -0x80000000) | ||
if (Buffer.TYPED_ARRAY_SUPPORT) { | ||
@@ -991,11 +1011,12 @@ this[offset] = value | ||
this[offset + 3] = (value >>> 24) | ||
} else objectWriteUInt32(this, value, offset, true) | ||
} else { | ||
objectWriteUInt32(this, value, offset, true) | ||
} | ||
return offset + 4 | ||
} | ||
Buffer.prototype.writeInt32BE = function (value, offset, noAssert) { | ||
Buffer.prototype.writeInt32BE = function writeInt32BE (value, offset, noAssert) { | ||
value = +value | ||
offset = offset >>> 0 | ||
if (!noAssert) | ||
checkInt(this, value, offset, 4, 0x7fffffff, -0x80000000) | ||
if (!noAssert) checkInt(this, value, offset, 4, 0x7fffffff, -0x80000000) | ||
if (value < 0) value = 0xffffffff + value + 1 | ||
@@ -1007,3 +1028,5 @@ if (Buffer.TYPED_ARRAY_SUPPORT) { | ||
this[offset + 3] = value | ||
} else objectWriteUInt32(this, value, offset, false) | ||
} else { | ||
objectWriteUInt32(this, value, offset, false) | ||
} | ||
return offset + 4 | ||
@@ -1019,4 +1042,5 @@ } | ||
function writeFloat (buf, value, offset, littleEndian, noAssert) { | ||
if (!noAssert) | ||
if (!noAssert) { | ||
checkIEEE754(buf, value, offset, 4, 3.4028234663852886e+38, -3.4028234663852886e+38) | ||
} | ||
ieee754.write(buf, value, offset, littleEndian, 23, 4) | ||
@@ -1026,7 +1050,7 @@ return offset + 4 | ||
Buffer.prototype.writeFloatLE = function (value, offset, noAssert) { | ||
Buffer.prototype.writeFloatLE = function writeFloatLE (value, offset, noAssert) { | ||
return writeFloat(this, value, offset, true, noAssert) | ||
} | ||
Buffer.prototype.writeFloatBE = function (value, offset, noAssert) { | ||
Buffer.prototype.writeFloatBE = function writeFloatBE (value, offset, noAssert) { | ||
return writeFloat(this, value, offset, false, noAssert) | ||
@@ -1036,4 +1060,5 @@ } | ||
function writeDouble (buf, value, offset, littleEndian, noAssert) { | ||
if (!noAssert) | ||
if (!noAssert) { | ||
checkIEEE754(buf, value, offset, 8, 1.7976931348623157E+308, -1.7976931348623157E+308) | ||
} | ||
ieee754.write(buf, value, offset, littleEndian, 52, 8) | ||
@@ -1043,7 +1068,7 @@ return offset + 8 | ||
Buffer.prototype.writeDoubleLE = function (value, offset, noAssert) { | ||
Buffer.prototype.writeDoubleLE = function writeDoubleLE (value, offset, noAssert) { | ||
return writeDouble(this, value, offset, true, noAssert) | ||
} | ||
Buffer.prototype.writeDoubleBE = function (value, offset, noAssert) { | ||
Buffer.prototype.writeDoubleBE = function writeDoubleBE (value, offset, noAssert) { | ||
return writeDouble(this, value, offset, false, noAssert) | ||
@@ -1053,3 +1078,3 @@ } | ||
// copy(targetBuffer, targetStart=0, sourceStart=0, sourceEnd=buffer.length) | ||
Buffer.prototype.copy = function (target, target_start, start, end) { | ||
Buffer.prototype.copy = function copy (target, target_start, start, end) { | ||
var self = this // source | ||
@@ -1068,4 +1093,5 @@ | ||
// Fatal error conditions | ||
if (target_start < 0) | ||
if (target_start < 0) { | ||
throw new RangeError('targetStart out of bounds') | ||
} | ||
if (start < 0 || start >= self.length) throw new RangeError('sourceStart out of bounds') | ||
@@ -1075,6 +1101,6 @@ if (end < 0) throw new RangeError('sourceEnd out of bounds') | ||
// Are we oob? | ||
if (end > this.length) | ||
end = this.length | ||
if (target.length - target_start < end - start) | ||
if (end > this.length) end = this.length | ||
if (target.length - target_start < end - start) { | ||
end = target.length - target_start + start | ||
} | ||
@@ -1095,3 +1121,3 @@ var len = end - start | ||
// fill(value, start=0, end=buffer.length) | ||
Buffer.prototype.fill = function (value, start, end) { | ||
Buffer.prototype.fill = function fill (value, start, end) { | ||
if (!value) value = 0 | ||
@@ -1130,3 +1156,3 @@ if (!start) start = 0 | ||
*/ | ||
Buffer.prototype.toArrayBuffer = function () { | ||
Buffer.prototype.toArrayBuffer = function toArrayBuffer () { | ||
if (typeof Uint8Array !== 'undefined') { | ||
@@ -1155,3 +1181,3 @@ if (Buffer.TYPED_ARRAY_SUPPORT) { | ||
*/ | ||
Buffer._augment = function (arr) { | ||
Buffer._augment = function _augment (arr) { | ||
arr.constructor = Buffer | ||
@@ -1174,2 +1200,3 @@ arr._isBuffer = true | ||
arr.compare = BP.compare | ||
arr.indexOf = BP.indexOf | ||
arr.copy = BP.copy | ||
@@ -1362,4 +1389,3 @@ arr.slice = BP.slice | ||
for (var i = 0; i < length; i++) { | ||
if ((i + offset >= dst.length) || (i >= src.length)) | ||
break | ||
if ((i + offset >= dst.length) || (i >= src.length)) break | ||
dst[i + offset] = src[i] | ||
@@ -1366,0 +1392,0 @@ } |
{ | ||
"name": "buffer", | ||
"description": "Node.js Buffer API, for the browser", | ||
"version": "3.0.3", | ||
"version": "3.1.0", | ||
"author": { | ||
@@ -24,3 +24,3 @@ "name": "Feross Aboukhadijeh", | ||
"benchmark": "^1.0.0", | ||
"browserify": "^7.0.3", | ||
"browserify": "^9.0.3", | ||
"concat-stream": "^1.4.7", | ||
@@ -33,3 +33,3 @@ "hyperquest": "^1.0.1", | ||
"through2": "^0.6.3", | ||
"zuul": "^1.12.0" | ||
"zuul": "^2.0.0" | ||
}, | ||
@@ -36,0 +36,0 @@ "homepage": "https://github.com/feross/buffer", |
@@ -280,2 +280,9 @@ # buffer [![travis][travis-image]][travis-url] [![npm][npm-image]][npm-url] [![downloads][downloads-image]][npm-url] [![gratipay][gratipay-image]][gratipay-url] | ||
## JavaScript Standard Style | ||
This module uses [JavaScript Standard Style](https://github.com/feross/standard). | ||
[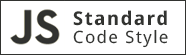](https://github.com/feross/standard) | ||
## credit | ||
@@ -282,0 +289,0 @@ |
var Buffer = require('../../').Buffer | ||
if (process.env.OBJECT_IMPL) return | ||
// var common = require('../common'); | ||
if (process.env.OBJECT_IMPL) Buffer.TYPED_ARRAY_SUPPORT = false | ||
var common = {}; | ||
var assert = require('assert'); | ||
@@ -5,0 +5,0 @@ |
var Buffer = require('../../').Buffer | ||
if (process.env.OBJECT_IMPL) Buffer.TYPED_ARRAY_SUPPORT = false | ||
// var common = require('../common'); | ||
var common = {}; | ||
var assert = require('assert'); | ||
@@ -27,1 +27,2 @@ | ||
} | ||
var Buffer = require('../../').Buffer | ||
if (process.env.OBJECT_IMPL) Buffer.TYPED_ARRAY_SUPPORT = false | ||
// var common = require('../common'); | ||
var common = {}; | ||
var assert = require('assert'); | ||
@@ -5,0 +5,0 @@ |
var Buffer = require('../../').Buffer | ||
if (process.env.OBJECT_IMPL) Buffer.TYPED_ARRAY_SUPPORT = false | ||
// var common = require('../common'); | ||
var common = {}; | ||
var assert = require('assert'); | ||
@@ -5,0 +5,0 @@ |
var Buffer = require('../../').Buffer | ||
if (process.env.OBJECT_IMPL) Buffer.TYPED_ARRAY_SUPPORT = false | ||
// var common = require('../common'); | ||
var common = {}; | ||
var assert = require('assert'); | ||
@@ -5,0 +5,0 @@ |
var Buffer = require('../../').Buffer | ||
if (process.env.OBJECT_IMPL) Buffer.TYPED_ARRAY_SUPPORT = false | ||
// var common = require('../common'); | ||
var common = {}; | ||
var assert = require('assert'); | ||
@@ -5,0 +5,0 @@ |
var Buffer = require('../../').Buffer | ||
if (process.env.OBJECT_IMPL) Buffer.TYPED_ARRAY_SUPPORT = false | ||
// var common = require('../common'); | ||
var common = {}; | ||
var assert = require('assert'); | ||
@@ -1113,12 +1113,16 @@ | ||
// Test truncation after decode | ||
// var crypto = require('crypto'); | ||
if (common.hasCrypto) { | ||
// Test truncation after decode | ||
var crypto = require('crypto'); | ||
var b1 = new Buffer('YW55=======', 'base64'); | ||
var b2 = new Buffer('YW55', 'base64'); | ||
var b1 = new Buffer('YW55=======', 'base64'); | ||
var b2 = new Buffer('YW55', 'base64'); | ||
assert.equal( | ||
1 /*crypto.createHash('sha1').update(b1).digest('hex')*/, | ||
1 /*crypto.createHash('sha1').update(b2).digest('hex')*/ | ||
); | ||
assert.equal( | ||
1 /*crypto.createHash('sha1').update(b1).digest('hex')*/, | ||
1 /*crypto.createHash('sha1').update(b2).digest('hex')*/ | ||
); | ||
} else { | ||
// console.log('1..0 # Skipped: missing crypto'); | ||
} | ||
@@ -1177,1 +1181,6 @@ // Test Compare | ||
var ps = Buffer.poolSize; | ||
Buffer.poolSize = 0; | ||
assert.equal(Buffer(1).parent, undefined); | ||
Buffer.poolSize = ps; | ||
@@ -110,9 +110,4 @@ var B = require('../').Buffer | ||
var r = reads.shift() | ||
if (isnan(r)) | ||
t.pass('equal') | ||
else | ||
t.equal( | ||
v1[readfn](0, true), | ||
r | ||
) | ||
if (isnan(r)) t.pass('equal') | ||
else t.equal(v1[readfn](0, true), r) | ||
} | ||
@@ -119,0 +114,0 @@ } |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
3181
295
121993
22