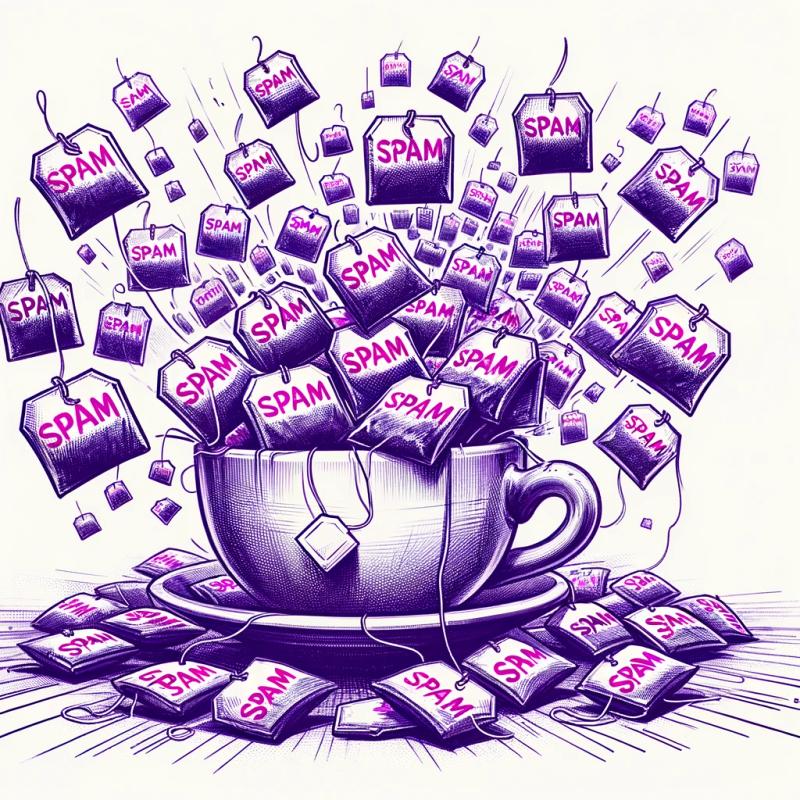
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
byteark-player-react
Advanced tools
Readme
You can try on the demo page.
This library is distributed via NPM. You may install from NPM or Yarn.
# For NPM
npm install --save byteark-player-react
# For Yarn
yarn add byteark-player-react
# For pnpm
pnpm add byteark-player-react
Include ByteArkPlayerContainer
component into your component.
import React from 'react'
import { render } from 'react-dom'
import { ByteArkPlayerContainer } from 'byteark-player-react'
import type { ByteArkPlayerContainerProps } from 'byteark-player-react'
const App = () => {
const playerOptions: ByteArkPlayerContainerProps = {
autoplay: 'any',
fluid: true,
aspectRatio: '16:9',
poster: 'https://stream-image.byteark.com/image/video-cover-480p/7/K/7KPloVWgN.png',
sources: [
{
src: 'https://byteark-playertzxedwv.stream-playlist.byteark.com/streams/ToIkm61TMn4Q/playlist.m3u8',
type: 'application/x-mpegURL',
title: 'Big Buck Bunny',
videoId: 'ToIkm61TMn4Q',
poster: 'https://stream-image.byteark.com/image/video-cover-480p/7/K/7KPloVWgN.png'
}
]
}
return <ByteArkPlayerContainer {...playerOptions} />
}
render(<App />, document.getElementById('root'))
If the video will be displayed on the fixed-size container, you may want to use fill mode instead.
import React from 'react'
import { render } from 'react-dom'
import { ByteArkPlayerContainer } from 'byteark-player-react'
import type { ByteArkPlayerContainerProps } from 'byteark-player-react'
const App = () => {
const playerOptions: ByteArkPlayerContainerProps = {
autoplay: 'any',
fill: true,
poster: 'https://stream-image.byteark.com/image/video-cover-480p/7/K/7KPloVWgN.png',
sources: [
{
src: 'https://byteark-playertzxedwv.stream-playlist.byteark.com/streams/ToIkm61TMn4Q/playlist.m3u8',
type: 'application/x-mpegURL',
title: 'Big Buck Bunny',
videoId: 'ToIkm61TMn4Q',
poster: 'https://stream-image.byteark.com/image/video-cover-480p/7/K/7KPloVWgN.png'
}
]
}
return <ByteArkPlayerContainer {...playerOptions} />
}
render(<App />, document.getElementById('root'))
Following properties are the properties that can be updated to the player, without re-creating the player instance. Additional properties will be passed to player.
Name | Type | Default | Description |
---|---|---|---|
autoplay | Boolean/String | true | Autoplay the video after player is created. (true/false/'muted'/'play'/'any') |
aspectRatio | String | - | Use with fluid layout mode, to inform expected video's aspect ratio (16:9) |
controls | Boolean | true | Show/hide the controls bar. |
fill | Boolean | - | Use fill layout mode. |
fluid | Boolean | - | Use fluid layout mode. |
loop | Boolean | - | Restart the video playback after plays to the end. |
muted | Boolean | - | Play the video without sounds. |
playerSlugId | String | - | SlugId of player created via api player server service |
playerVersion | String | 1.0 | Version of the player to use. |
playbackRate | Number | 1.0 | Playback speed. 1.0 means original speed. |
playsinline | Boolean | true | Should be true so custom controls available on all platforms, including iOS. |
poster | String | - | Image to be show before the video is playing. |
preload | String | - | Preload the video before play. ('none'/'metadata'/'auto') |
responsive | Boolean | - | Auto show/hide controls depending on the screen size. |
seekButtons | Boolean | false | Show 10 seconds seek buttons and allow double-tap to seek on mobile. |
sources | Array | - | Array of video source object to be played. |
volume | Number | - | Video's volume, between 0 to 1. |
You can also use other props not listed here, but appear as VideoJS's options. However, changing props will not effective after the player is created.
The source
object has 2 required fields, and more optional field:
Name | Type | Description |
---|---|---|
src | String | URL to the video. |
type | String | Video content type. |
title | String | Video title to display on the player. |
videoId | String | Video's ID, usually used on Analytics. |
poster | String | Poster image URL for the video. |
To provide multiple version of sources, you can use array of source objects.
We also provide some callback properties, so you can inject some behaviours directly to the ByteArk Player, and also, to the VideoJS's instance.
Name | Type | Callback Parameters | Description |
---|---|---|---|
onPlayerLoaded | Function | - | Callback function to be called when loaded player's resources. |
onPlayerLoadingError | Function | ({error: ByteArkPlayerContainerError, originalError: ByteArkPlayerError) | Callback function to be called when there're an error about loading player. |
onPlayerSetup | Function | - | Callback function to be called when player is setup. |
onPlayerSetupError | Function | ({error: ByteArkPlayerContainerError, originalError: ByteArkPlayerError) | Callback function to be called when there're an error when setup player. |
onReady | Function | (player: ByteArkPlayer) | Callback function to be called when a player instance is ready. |
onPlayerCreated | Function | (player: ByteArkPlayer) | Callback function to be called when a player instance is created. |
We also provide some ways to custom the appearance of the video placeholder, and some advance behaviours.
Name | Type | Description |
---|---|---|
createPlaceholderFunction | Function | Custom video placeholder. This function should return a React component. |
createPlayerFunction | Function | Custom video instance. This function should return a VideoJS's player instance. |
lazyload | Boolean | The player loads its asset files once it got rendered on the webpage. By passing this prop, the player then loads its asset files once the user clicked on the player instead. |
playerEndpoint | String | Endpoint to the video player (without version part). |
playerJsFileName | String | File name of player's JS. |
playerCssFileName | String | File name of player's CSS. |
import { ByteArkPlayerContainer } from 'byteark-player-react'
const App = () => {
// An Advanced Props Example
return
<ByteArkPlayerContainer
{...playerOptions}
playerEndpoint='my-custom-endpoint'
lazyload />
}
You may access the player instance from onReady
callback parameter.
// This following example allows user to play/pause the video playback
// from custom buttons outside.
import React from 'react'
import { render } from 'react-dom'
import { ByteArkPlayerContainer } from 'byteark-player-react'
import type { ByteArkPlayerContainerProps, ByteArkPlayer } from 'byteark-player-react'
const App = () => {
const playerOptions: ByteArkPlayerContainerProps = {
autoplay: 'any',
fluid: true,
sources: [
{
src: 'https://byteark-playertzxedwv.stream-playlist.byteark.com/streams/ToIkm61TMn4Q/playlist.m3u8',
type: 'application/x-mpegURL',
title: 'Big Buck Bunny',
videoId: 'ToIkm61TMn4Q'
}
]
}
let playerInstance = null
const onReady = (newPlayerInstance: ByteArkPlayer) => {
playerInstance = newPlayerInstance
}
return <div>
<ByteArkPlayerContainer {...playerOptions} onReady={onReady} />
<button onClick={() => playerInstance?.play()}>Play</button>
<button onClick={() => playerInstance?.pause()}>Pause</button>
</div>
}
render(<App />, document.getElementById('root'))
import React from 'react'
import { render } from 'react-dom'
import { ByteArkPlayerContainer } from 'byteark-player-react'
import type { ByteArkPlayerContainerProps, ByteArkPlayer } from 'byteark-player-react'
const App = () => {
const playerOptions: ByteArkPlayerContainerProps = {
autoplay: 'any',
fluid: true,
sources: [
{
src: 'https://byteark-playertzxedwv.stream-playlist.byteark.com/streams/ToIkm61TMn4Q/playlist.m3u8',
type: 'application/x-mpegURL',
title: 'Big Buck Bunny',
videoId: 'ToIkm61TMn4Q'
}
]
}
const onReady = (newPlayerInstance: ByteArkPlayer) => {
// The player is ready! Initialize plugins here.
}
return <ByteArkPlayerContainer {...playerOptions} onReady={onReady} />
}
render(<App />, document.getElementById('root'))
import React from 'react'
import { render } from 'react-dom'
import { ByteArkPlayerContainer } from 'byteark-player-react'
import type { ByteArkPlayerContainerProps, ByteArkPlayer } from 'byteark-player-react'
const App = () => {
const playerOptions: ByteArkPlayerContainerProps = {
autoplay: 'any',
fluid: true,
sources: [
{
src: 'https://byteark-playertzxedwv.stream-playlist.byteark.com/streams/ToIkm61TMn4Q/playlist.m3u8',
type: 'application/x-mpegURL',
title: 'Big Buck Bunny',
videoId: 'ToIkm61TMn4Q'
}
],
html5: {
hlsjs: {
xhrSetup: function(xhr: XMLHttpRequest, url: string) {
xhr.withCredentials = true
}
}
}
}
const onReady = (newPlayerInstance: ByteArkPlayer) => {
// The player is ready! Initialize plugins here.
}
return <ByteArkPlayerContainer {...playerOptions} onReady={onReady} />
}
MIT © ByteArk Co. Ltd.
FAQs
ByteArk Player Container for React
The npm package byteark-player-react receives a total of 230 weekly downloads. As such, byteark-player-react popularity was classified as not popular.
We found that byteark-player-react demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.