Comparing version 0.3.3 to 0.4.0
319
chance.js
@@ -1,2 +0,2 @@ | ||
// Chance.js 0.3.3 | ||
// Chance.js 0.4.0 | ||
// http://chancejs.com | ||
@@ -11,10 +11,17 @@ // (c) 2013 Victor Quinn | ||
if (seed !== undefined) { | ||
this.seed = seed; | ||
// If we were passed a generator rather than a seed, use it. | ||
if (typeof seed === 'function') { | ||
this.random = seed; | ||
} else { | ||
this.seed = seed; | ||
} | ||
} | ||
this.mt = this.mersenne_twister(seed); | ||
}; | ||
// Wrap the MersenneTwister | ||
Chance.prototype.random = function () { | ||
return this.mt.random(this.seed); | ||
// If no generator function was provided, use our MT | ||
if (typeof this.random === 'undefined') { | ||
this.mt = this.mersenne_twister(seed); | ||
this.random = function () { | ||
return this.mt.random(this.seed); | ||
}; | ||
} | ||
}; | ||
@@ -24,4 +31,12 @@ | ||
Chance.prototype.bool = function () { | ||
return this.random() * 100 < 50; | ||
Chance.prototype.bool = function (options) { | ||
options = options || {}; | ||
// likelihood of success (true) | ||
options.likelihood = (typeof options.likelihood !== "undefined") ? options.likelihood : 50; | ||
if (options.likelihood < 0 || options.likelihood > 100) { | ||
throw new RangeError("Chance: Likelihood accepts values from 0 to 100."); | ||
} | ||
return this.random() * 100 < options.likelihood; | ||
}; | ||
@@ -68,3 +83,62 @@ | ||
}; | ||
Chance.prototype.normal = function (options) { | ||
options = options || {}; | ||
// The Marsaglia Polar method | ||
var s, u, v, norm, | ||
mean = options.mean || 0, | ||
dev = (typeof options.dev !== 'undefined') ? options.dev : 1; | ||
do { | ||
// U and V are from the uniform distribution on (-1, 1) | ||
u = this.random() * 2 - 1; | ||
v = this.random() * 2 - 1; | ||
s = u * u + v * v; | ||
} while (s >= 1); | ||
// Compute the standard normal variate | ||
norm = u * Math.sqrt(-2 * Math.log(s) / s); | ||
// Shape and scale | ||
return dev * norm + mean; | ||
}; | ||
// Note, wanted to use "float" or "double" but those are both JS reserved words. | ||
// Note, fixed means N OR LESS digits after the decimal. This because | ||
// It could be 14.9000 but in JavaScript, when this is cast as a number, | ||
// the trailing zeroes are dropped. Left to the consumer if trailing zeroes are | ||
// needed | ||
Chance.prototype.floating = function (options) { | ||
var num, range, buffer; | ||
options = options || {}; | ||
options.fixed = (typeof options.fixed !== "undefined") ? options.fixed : 4; | ||
fixed = Math.pow(10, options.fixed); | ||
if (options.fixed && options.precision) { | ||
throw new RangeError("Chance: Cannot specify both fixed and precision."); | ||
} | ||
if (options.min && options.fixed && options.min < (-9007199254740992 / fixed)) { | ||
throw new RangeError("Chance: Min specified is out of range with fixed. Min" + | ||
"should be, at least, " + (-9007199254740992 / fixed)); | ||
} else if (options.max && options.fixed && options.max > (9007199254740992 / fixed)) { | ||
throw new RangeError("Chance: Max specified is out of range with fixed. Max" + | ||
"should be, at most, " + (9007199254740992 / fixed)); | ||
} | ||
options.min = (typeof options.min !== "undefined") ? options.min : -9007199254740992 / fixed; | ||
options.max = (typeof options.max !== "undefined") ? options.max : 9007199254740992 / fixed; | ||
// Todo - Make this work! | ||
// options.precision = (typeof options.precision !== "undefined") ? options.precision : false; | ||
num = this.integer({min: options.min * fixed, max: options.max * fixed}); | ||
num_fixed = (num / fixed).toFixed(options.fixed); | ||
return parseFloat(num_fixed); | ||
}; | ||
Chance.prototype.character = function (options) { | ||
@@ -292,2 +366,6 @@ options = options || {}; | ||
Chance.prototype.fbid = function (options) { | ||
return '10000' + this.natural({max: 100000000000}).toString(); | ||
}; | ||
Chance.prototype.ip = function () { | ||
@@ -310,2 +388,6 @@ // Todo: This could return some reserved IPs. See http://vq.io/137dgYy | ||
Chance.prototype.twitter = function (options) { | ||
return '@' + this.word(); | ||
}; | ||
// -- End Web -- | ||
@@ -320,2 +402,10 @@ | ||
Chance.prototype.areacode = function (options) { | ||
options = options || {}; | ||
options.parens = (typeof options.parens !== "undefined") ? options.parens : true; | ||
// Don't want area codes to start with 1 | ||
var areacode = this.natural({min: 2, max: 9}).toString() + this.natural({min: 10, max: 98}).toString(); | ||
return options.parens ? '(' + areacode + ')' : areacode; | ||
}; | ||
Chance.prototype.city = function (options) { | ||
@@ -326,72 +416,69 @@ options = options || {}; | ||
Chance.prototype.phone = function (options) { | ||
Chance.prototype.coordinates = function (options) { | ||
options = options || {}; | ||
return this.areacode() + ' ' + this.natural({min: 200, max: 999}) + '-' + this.natural({min: 1000, max: 9999}); | ||
return this.latitude(options) + ', ' + this.longitude(options); | ||
}; | ||
Chance.prototype.areacode = function (options) { | ||
Chance.prototype.latitude = function (options) { | ||
options = options || {}; | ||
options.parens = (typeof options.parens !== "undefined") ? options.parens : true; | ||
// Don't want area codes to start with 1 | ||
var areacode = this.natural({min: 2, max: 9}).toString() + this.natural({min: 10, max: 98}).toString(); | ||
return options.parens ? '(' + areacode + ')' : areacode; | ||
options.fixed = (typeof options.fixed !== "undefined") ? options.fixed : 5; | ||
return this.floating({min: -90, max: 90, fixed: options.fixed}); | ||
}; | ||
Chance.prototype.street = function (options) { | ||
Chance.prototype.longitude = function (options) { | ||
options = options || {}; | ||
options.fixed = (typeof options.fixed !== "undefined") ? options.fixed : 5; | ||
return this.floating({min: 0, max: 180, fixed: options.fixed}); | ||
}; | ||
var street = this.word({syllables: 2}); | ||
street = this.capitalize(street); | ||
street += ' '; | ||
street += options.short_suffix ? | ||
this.street_suffix().abbreviation : | ||
this.street_suffix().name; | ||
return street; | ||
Chance.prototype.phone = function (options) { | ||
options = options || {}; | ||
return this.areacode() + ' ' + this.natural({min: 200, max: 999}) + '-' + this.natural({min: 1000, max: 9999}); | ||
}; | ||
Chance.prototype.street_suffixes = function () { | ||
// These are the most common suffixes. | ||
Chance.prototype.postal = function (options) { | ||
// Postal District | ||
var pd = this.character({pool: "XVTSRPNKLMHJGECBA"}); | ||
// Forward Sortation Area (FSA) | ||
var fsa = pd + this.natural({max: 9}) + this.character({alpha: true, casing: "upper"}); | ||
// Local Delivery Unut (LDU) | ||
var ldu = this.natural({max: 9}) + this.character({alpha: true, casing: "upper"}) + this.natural({max: 9}); | ||
return fsa + " " + ldu; | ||
}; | ||
Chance.prototype.provinces = function () { | ||
return [ | ||
{name: 'Avenue', abbreviation: 'Ave'}, | ||
{name: 'Boulevard', abbreviation: 'Blvd'}, | ||
{name: 'Center', abbreviation: 'Ctr'}, | ||
{name: 'Circle', abbreviation: 'Cir'}, | ||
{name: 'Court', abbreviation: 'Ct'}, | ||
{name: 'Drive', abbreviation: 'Dr'}, | ||
{name: 'Extension', abbreviation: 'Ext'}, | ||
{name: 'Glen', abbreviation: 'Gln'}, | ||
{name: 'Grove', abbreviation: 'Grv'}, | ||
{name: 'Heights', abbreviation: 'Hts'}, | ||
{name: 'Highway', abbreviation: 'Hwy'}, | ||
{name: 'Junction', abbreviation: 'Jct'}, | ||
{name: 'Key', abbreviation: 'Key'}, | ||
{name: 'Lane', abbreviation: 'Ln'}, | ||
{name: 'Loop', abbreviation: 'Loop'}, | ||
{name: 'Manor', abbreviation: 'Mnr'}, | ||
{name: 'Mill', abbreviation: 'Mill'}, | ||
{name: 'Park', abbreviation: 'Park'}, | ||
{name: 'Parkway', abbreviation: 'Pkwy'}, | ||
{name: 'Pass', abbreviation: 'Pass'}, | ||
{name: 'Path', abbreviation: 'Path'}, | ||
{name: 'Pike', abbreviation: 'Pike'}, | ||
{name: 'Place', abbreviation: 'Pl'}, | ||
{name: 'Plaza', abbreviation: 'Plz'}, | ||
{name: 'Point', abbreviation: 'Pt'}, | ||
{name: 'Ridge', abbreviation: 'Rdg'}, | ||
{name: 'River', abbreviation: 'Riv'}, | ||
{name: 'Road', abbreviation: 'Rd'}, | ||
{name: 'Square', abbreviation: 'Sq'}, | ||
{name: 'Street', abbreviation: 'St'}, | ||
{name: 'Terrace', abbreviation: 'Ter'}, | ||
{name: 'Trail', abbreviation: 'Trl'}, | ||
{name: 'Turnpike', abbreviation: 'Tpke'}, | ||
{name: 'View', abbreviation: 'Vw'}, | ||
{name: 'Way', abbreviation: 'Way'} | ||
{name: 'Alberta', abbreviation: 'AB'}, | ||
{name: 'British Columbia', abbreviation: 'BC'}, | ||
{name: 'Manitoba', abbreviation: 'MB'}, | ||
{name: 'New Brunswick', abbreviation: 'NB'}, | ||
{name: 'Newfoundland and Labrador', abbreviation: 'NL'}, | ||
{name: 'Nova Scotia', abbreviation: 'NS'}, | ||
{name: 'Ontario', abbreviation: 'ON'}, | ||
{name: 'Prince Edward Island', abbreviation: 'PE'}, | ||
{name: 'Quebec', abbreviation: 'QC'}, | ||
{name: 'Saskatchewan', abbreviation: 'SK'}, | ||
// The case could be made that the following are not actually provinces | ||
// since they are technically considered "territories" however they all | ||
// look the same on an envelope! | ||
{name: 'Northwest Territories', abbreviation: 'NT'}, | ||
{name: 'Nunavut', abbreviation: 'NU'}, | ||
{name: 'Yukon', abbreviation: 'YT'} | ||
]; | ||
}; | ||
Chance.prototype.street_suffix = function (options) { | ||
return this.pick(this.street_suffixes(options)); | ||
Chance.prototype.province = function (options) { | ||
return (options && options.full) ? | ||
this.pick(this.provinces()).name : | ||
this.pick(this.provinces()).abbreviation; | ||
}; | ||
Chance.prototype.state = function (options) { | ||
return (options && options.full) ? | ||
this.pick(this.states()).name : | ||
this.pick(this.states()).abbreviation; | ||
}; | ||
Chance.prototype.states = function () { | ||
@@ -463,8 +550,59 @@ return [ | ||
Chance.prototype.state = function (options) { | ||
return (options && options.full) ? | ||
this.pick(this.states()).name : | ||
this.pick(this.states()).abbreviation; | ||
Chance.prototype.street = function (options) { | ||
options = options || {}; | ||
var street = this.word({syllables: 2}); | ||
street = this.capitalize(street); | ||
street += ' '; | ||
street += options.short_suffix ? | ||
this.street_suffix().abbreviation : | ||
this.street_suffix().name; | ||
return street; | ||
}; | ||
Chance.prototype.street_suffix = function (options) { | ||
return this.pick(this.street_suffixes(options)); | ||
}; | ||
Chance.prototype.street_suffixes = function () { | ||
// These are the most common suffixes. | ||
return [ | ||
{name: 'Avenue', abbreviation: 'Ave'}, | ||
{name: 'Boulevard', abbreviation: 'Blvd'}, | ||
{name: 'Center', abbreviation: 'Ctr'}, | ||
{name: 'Circle', abbreviation: 'Cir'}, | ||
{name: 'Court', abbreviation: 'Ct'}, | ||
{name: 'Drive', abbreviation: 'Dr'}, | ||
{name: 'Extension', abbreviation: 'Ext'}, | ||
{name: 'Glen', abbreviation: 'Gln'}, | ||
{name: 'Grove', abbreviation: 'Grv'}, | ||
{name: 'Heights', abbreviation: 'Hts'}, | ||
{name: 'Highway', abbreviation: 'Hwy'}, | ||
{name: 'Junction', abbreviation: 'Jct'}, | ||
{name: 'Key', abbreviation: 'Key'}, | ||
{name: 'Lane', abbreviation: 'Ln'}, | ||
{name: 'Loop', abbreviation: 'Loop'}, | ||
{name: 'Manor', abbreviation: 'Mnr'}, | ||
{name: 'Mill', abbreviation: 'Mill'}, | ||
{name: 'Park', abbreviation: 'Park'}, | ||
{name: 'Parkway', abbreviation: 'Pkwy'}, | ||
{name: 'Pass', abbreviation: 'Pass'}, | ||
{name: 'Path', abbreviation: 'Path'}, | ||
{name: 'Pike', abbreviation: 'Pike'}, | ||
{name: 'Place', abbreviation: 'Pl'}, | ||
{name: 'Plaza', abbreviation: 'Plz'}, | ||
{name: 'Point', abbreviation: 'Pt'}, | ||
{name: 'Ridge', abbreviation: 'Rdg'}, | ||
{name: 'River', abbreviation: 'Riv'}, | ||
{name: 'Road', abbreviation: 'Rd'}, | ||
{name: 'Square', abbreviation: 'Sq'}, | ||
{name: 'Street', abbreviation: 'St'}, | ||
{name: 'Terrace', abbreviation: 'Ter'}, | ||
{name: 'Trail', abbreviation: 'Trl'}, | ||
{name: 'Turnpike', abbreviation: 'Tpke'}, | ||
{name: 'View', abbreviation: 'Vw'}, | ||
{name: 'Way', abbreviation: 'Way'} | ||
]; | ||
}; | ||
// Note: only returning US zip codes, internationalization will be a whole | ||
@@ -493,2 +631,18 @@ // other beast to tackle at some point. | ||
Chance.prototype.ampm = function (options) { | ||
options = options || {}; | ||
return this.bool() ? 'am' : 'pm'; | ||
}; | ||
Chance.prototype.hour = function (options) { | ||
options = options || {}; | ||
var max = options.twentyfour ? 24 : 12; | ||
return this.natural({min: 1, max: max}); | ||
}; | ||
Chance.prototype.minute = function (options) { | ||
options = options || {}; | ||
return this.natural({min: 0, max: 59}); | ||
}; | ||
Chance.prototype.month = function (options) { | ||
@@ -517,2 +671,12 @@ options = options || {}; | ||
Chance.prototype.second = function (options) { | ||
options = options || {}; | ||
return this.natural({min: 0, max: 59}); | ||
}; | ||
Chance.prototype.timestamp = function (options) { | ||
options = options || {}; | ||
return this.natural({min: 1, max: parseInt(new Date().getTime() / 1000, 10)}); | ||
}; | ||
Chance.prototype.year = function (options) { | ||
@@ -605,2 +769,21 @@ options = options || {}; | ||
Chance.prototype.dollar = function (options) { | ||
options = options || {}; | ||
// By default, a somewhat more sane max for dollar than all available numbers | ||
options.max = (typeof options.max !== "undefined") ? options.max : 10000; | ||
options.min = (typeof options.min !== "undefined") ? options.min : 0; | ||
var dollar = this.floating({min: options.min, max: options.max, fixed: 2}).toString(), | ||
cents = dollar.split('.')[1]; | ||
if (cents === undefined) { | ||
dollar += '.00'; | ||
} else if (cents.length < 2) { | ||
dollar = dollar + '0'; | ||
} | ||
return '$' + dollar; | ||
}; | ||
Chance.prototype.exp = function (options) { | ||
@@ -681,3 +864,3 @@ options = options || {}; | ||
Chance.prototype.VERSION = "0.3.3"; | ||
Chance.prototype.VERSION = "0.4.0"; | ||
@@ -684,0 +867,0 @@ // Mersenne Twister from https://gist.github.com/banksean/300494 |
@@ -38,6 +38,13 @@ module.exports = function (grunt) { | ||
}, | ||
uglify: { | ||
my_target: { | ||
files: { | ||
'chance.min.js': ['chance.js'] | ||
} | ||
} | ||
}, | ||
watch: { | ||
options: { livereload: true }, | ||
files: js_files, | ||
tasks: ['jshint', 'shell:mocha-phantomjs'] | ||
tasks: ['jshint', 'shell:mocha-phantomjs', 'uglify'] | ||
} | ||
@@ -47,2 +54,3 @@ }); | ||
grunt.loadNpmTasks('grunt-contrib-jshint'); | ||
grunt.loadNpmTasks('grunt-contrib-uglify'); | ||
grunt.loadNpmTasks('grunt-contrib-watch'); | ||
@@ -49,0 +57,0 @@ grunt.loadNpmTasks('grunt-shell'); |
{ | ||
"name": "chance", | ||
"version": "0.3.3", | ||
"main": "./chance.js", | ||
"version": "0.4.0", | ||
"description": "Chance - Utility library to generate anything random", | ||
@@ -26,2 +26,3 @@ "homepage": "http://chancejs.com", | ||
"grunt-mocha-phantomjs": "*", | ||
"grunt-contrib-uglify": "*", | ||
"grunt-contrib-watch": "*", | ||
@@ -28,0 +29,0 @@ "grunt-shell": "*", |
# Chance | ||
[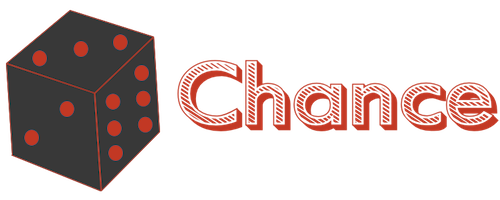](http://chancejs.com) | ||
[](https://travis-ci.org/victorquinn/chancejs) | ||
@@ -53,1 +55,30 @@ | ||
## Author | ||
### Victor Quinn | ||
[http://victorquinn.com](http://victorquinn.com) | ||
Please feel free to reach out to me if you have any questions or suggestions. | ||
### Contributors | ||
THANK YOU! | ||
``` | ||
project : chancejs | ||
repo age : 4 weeks | ||
active : 23 days | ||
commits : 112 | ||
files : 18 | ||
authors : | ||
90 Victor Quinn 80.4% | ||
11 Tim Petricola 9.8% | ||
5 Michael Cordingley 4.5% | ||
2 Kevin Garnett 1.8% | ||
1 Richard Anaya 0.9% | ||
1 leesei 0.9% | ||
1 path411 0.9% | ||
1 qjcg 0.9% | ||
``` | ||
This project is licensed under the [MIT License](http://en.wikipedia.org/wiki/MIT_License) so feel free to hack away :) |
@@ -27,11 +27,8 @@ require.config({ | ||
var assert = chai.assert; | ||
require(['test.basic', 'test.text', 'test.address', 'test.misc', 'test.web', 'test.name', 'test.helpers', 'test.finance', 'test.time'], function () { | ||
require(['test.address', 'test.basic', 'test.finance', 'test.helpers', 'test.misc', 'test.name', 'test.text', 'test.time', 'test.web'], function () { | ||
mocha.reporter('html'); | ||
// Start runner | ||
if (window.mochaPhantomJS) { | ||
mochaPhantomJS.run(); | ||
} else { | ||
mocha.run(); | ||
} | ||
if (window.mochaPhantomJS) { mochaPhantomJS.run(); } | ||
else { mocha.run(); } | ||
}); | ||
@@ -38,0 +35,0 @@ }, function (err) { |
@@ -6,3 +6,3 @@ define(['Chance', 'mocha', 'chai', 'underscore'], function (Chance, mocha, chai, _) { | ||
describe("Address", function () { | ||
var zip, suffix, suffixes, state, address, phone, chance = new Chance(); | ||
var zip, suffix, suffixes, state, address, phone, coordinates, chance = new Chance(); | ||
@@ -81,2 +81,20 @@ describe("Zip", function () { | ||
describe("Province", function () { | ||
it("provinces() returns an array of provinces", function () { | ||
expect(chance.provinces()).to.be.an('array'); | ||
}); | ||
it("province() returns a random (short) province name", function () { | ||
_(1000).times(function () { | ||
expect(chance.province()).to.have.length.below(3); | ||
}); | ||
}); | ||
it("province({full: true}) returns a random (long) province name", function () { | ||
_(1000).times(function () { | ||
expect(chance.province({full: true})).to.have.length.above(2); | ||
}); | ||
}); | ||
}); | ||
describe("Address", function () { | ||
@@ -132,3 +150,36 @@ it("address() returns a string", function () { | ||
}); | ||
describe("Latitude", function () { | ||
it("latitude() looks right", function () { | ||
expect(chance.latitude()).to.be.a('number'); | ||
}); | ||
it("latitude() is in the right range", function () { | ||
_(1000).times(function () { | ||
expect(chance.latitude()).to.be.within(-90, 90); | ||
}); | ||
}); | ||
}); | ||
describe("Longitude", function () { | ||
it("longitude() looks right", function () { | ||
expect(chance.longitude()).to.be.a('number'); | ||
}); | ||
it("longitude() is in the right range", function () { | ||
_(1000).times(function () { | ||
expect(chance.longitude()).to.be.within(0, 180); | ||
}); | ||
}); | ||
}); | ||
describe("Coordinates", function () { | ||
it("coordinates() looks about right", function () { | ||
_(1000).times(function () { | ||
expect(chance.coordinates()).to.be.a('string'); | ||
expect(chance.coordinates().split(',')).to.have.length(2); | ||
}); | ||
}); | ||
}); | ||
}); | ||
}); |
@@ -5,3 +5,3 @@ define(['Chance', 'mocha', 'chai', 'underscore'], function (Chance, mocha, chai, _) { | ||
describe("Basics", function () { | ||
var bool, integer, natural, character, string, chance = new Chance(); | ||
var bool, integer, natural, floating, character, string, temp, chance = new Chance(); | ||
@@ -30,2 +30,29 @@ describe("Bool", function () { | ||
}); | ||
it("takes and obeys likelihood", function () { | ||
var true_count = 0; | ||
_(1000).times(function () { | ||
if (chance.bool({likelihood: 30})) { | ||
true_count++; | ||
} | ||
}); | ||
// Expect it to average around 300 | ||
expect(true_count).to.be.within(200, 400); | ||
true_count = 0; | ||
_(1000).times(function () { | ||
if (chance.bool({likelihood: 99})) { | ||
true_count++; | ||
} | ||
}); | ||
// Expect it to average at 990 | ||
expect(true_count).to.be.above(900); | ||
}); | ||
it("throws an error if likelihood < 0 or > 100", function () { | ||
expect(function () { chance.bool({likelihood: -23}); }).to.throw(RangeError); | ||
expect(function () { chance.bool({likelihood: 7933}); }).to.throw(RangeError); | ||
}); | ||
}); | ||
@@ -144,2 +171,44 @@ | ||
describe("Floating", function () { | ||
it("returns a random floating", function () { | ||
floating = chance.floating(); | ||
expect(floating).to.be.a('number'); | ||
}); | ||
it("can take both a max and min and obey them both", function () { | ||
_(1000).times(function () { | ||
floating = chance.floating({min: 90, max: 100}); | ||
expect(floating).to.be.within(90, 100); | ||
}); | ||
}); | ||
it("won't take fixed + min that would be out of range", function () { | ||
expect(function () { chance.floating({fixed: 13, min: -9007199254740992}); }).to.throw(RangeError); | ||
}); | ||
it("won't take fixed + max that would be out of range", function () { | ||
expect(function () { chance.floating({fixed: 13, max: 9007199254740992}); }).to.throw(RangeError); | ||
}); | ||
it("obeys the fixed parameter, when present", function () { | ||
_(100).times(function () { | ||
floating = chance.floating({fixed: 4}); | ||
floating = floating.toString().split('.')[1] ? floating.toString().split('.')[1] : ''; | ||
expect(floating).to.have.length.below(5); | ||
}); | ||
}); | ||
it("can take fixed and obey it", function () { | ||
_(1000).times(function () { | ||
floating = chance.floating({fixed: 3}); | ||
temp = parseFloat(floating.toFixed(3)); | ||
expect(floating).to.equal(temp); | ||
}); | ||
}); | ||
it("won't take both fixed and precision", function () { | ||
expect(function () { chance.floating({fixed: 2, precision: 8}); }).to.throw(RangeError); | ||
}); | ||
}); | ||
describe("Character", function () { | ||
@@ -233,4 +302,12 @@ it("returns a character", function () { | ||
}); | ||
describe("arbitrary function", function () { | ||
it("will take an arbitrary function for random, use it", function () { | ||
var chance = new Chance(function () { return 123; }); | ||
_(1000).times(function () { | ||
expect(chance.random()).to.equal(123); | ||
}); | ||
}); | ||
}); | ||
}); | ||
}); |
@@ -71,2 +71,24 @@ define(['Chance', 'mocha', 'chai', 'underscore'], function (Chance, mocha, chai, _) { | ||
}); | ||
describe("Dollar", function () { | ||
var dollar, chance = new Chance(); | ||
it("returns a proper dollar amount", function () { | ||
_(1000).times(function () { | ||
dollar = chance.dollar(); | ||
expect(dollar).to.match(/\$[0-9]+\.[0-9]+/); | ||
dollar = parseFloat(dollar.substring(1, dollar.length)); | ||
expect(dollar).to.be.below(10001); | ||
}); | ||
}); | ||
it("obeys min and max", function () { | ||
_(1000).times(function () { | ||
dollar = chance.dollar({max: 20}); | ||
dollar = parseFloat(dollar.substring(1, dollar.length)); | ||
expect(dollar).to.not.be.above(20); | ||
}); | ||
}); | ||
}); | ||
describe("Expiration", function () { | ||
@@ -73,0 +95,0 @@ it("exp() looks correct", function () { |
@@ -5,4 +5,20 @@ define(['Chance', 'mocha', 'chai', 'underscore'], function (Chance, mocha, chai, _) { | ||
describe("Time", function () { | ||
var month, year, chance = new Chance(); | ||
var hour, minute, time, timestamp, month, year, chance = new Chance(); | ||
it("hour() returns a hour", function () { | ||
_(1000).times(function () { | ||
hour = chance.hour(); | ||
expect(hour).to.be.a('number'); | ||
expect(hour).to.be.within(1, 12); | ||
}); | ||
}); | ||
it("minute() returns a minute", function () { | ||
_(1000).times(function () { | ||
minute = chance.minute(); | ||
expect(minute).to.be.a('number'); | ||
expect(minute).to.be.within(0, 59); | ||
}); | ||
}); | ||
it("month() returns a month", function () { | ||
@@ -23,2 +39,10 @@ _(1000).times(function () { | ||
it("timestamp() returns a timestamp", function () { | ||
_(1000).times(function () { | ||
timestamp = chance.timestamp(); | ||
expect(timestamp).to.be.a('number'); | ||
expect(timestamp).to.be.within(1, parseInt(new Date().getTime() / 1000, 10)); | ||
}); | ||
}); | ||
it("year() returns a year, default between today and a century after", function () { | ||
@@ -25,0 +49,0 @@ _(1000).times(function () { |
@@ -5,3 +5,3 @@ define(['Chance', 'mocha', 'chai', 'underscore'], function (Chance, mocha, chai, _) { | ||
describe("Web", function () { | ||
var tld, domain, email, ip, chance = new Chance(); | ||
var tld, domain, email, ip, twitter, chance = new Chance(); | ||
@@ -48,2 +48,8 @@ it("tld() returns a tld", function () { | ||
it("fbid() returns what looks like a Facebook id", function () { | ||
_(1000).times(function () { | ||
expect(chance.fbid()).to.be.a('string'); | ||
}); | ||
}); | ||
it("ip() returns what looks like an IP address", function () { | ||
@@ -56,3 +62,11 @@ _(1000).times(function () { | ||
}); | ||
it("twitter() returns what looks like a Twitter handle", function () { | ||
_(1000).times(function () { | ||
twitter = chance.twitter(); | ||
expect(twitter).to.be.a('string'); | ||
expect(twitter).to.match(/\@[A-Za-z]+/m); | ||
}); | ||
}); | ||
}); | ||
}); |
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Dynamic require
Supply chain riskDynamic require can indicate the package is performing dangerous or unsafe dynamic code execution.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Dynamic require
Supply chain riskDynamic require can indicate the package is performing dangerous or unsafe dynamic code execution.
Found 1 instance in 1 package
101476
18
1816
84
14