Comparing version 1.0.0-rc.10 to 1.0.0-rc.11
@@ -6,4 +6,4 @@ /** | ||
*/ | ||
import type { Node, Element } from 'domhandler'; | ||
import type { Cheerio } from '../cheerio'; | ||
import type { AnyNode, Element } from 'domhandler'; | ||
import type { Cheerio } from '../cheerio.js'; | ||
/** | ||
@@ -25,3 +25,3 @@ * Method for getting attributes. Gets the attribute value for only the first | ||
*/ | ||
export declare function attr<T extends Node>(this: Cheerio<T>, name: string): string | undefined; | ||
export declare function attr<T extends AnyNode>(this: Cheerio<T>, name: string): string | undefined; | ||
/** | ||
@@ -42,3 +42,3 @@ * Method for getting all attributes and their values of the first element in | ||
*/ | ||
export declare function attr<T extends Node>(this: Cheerio<T>): Record<string, string>; | ||
export declare function attr<T extends AnyNode>(this: Cheerio<T>): Record<string, string> | undefined; | ||
/** | ||
@@ -62,3 +62,3 @@ * Method for setting attributes. Sets the attribute value for only the first | ||
*/ | ||
export declare function attr<T extends Node>(this: Cheerio<T>, name: string, value?: string | null | ((this: Element, i: number, attrib: string) => string | null)): Cheerio<T>; | ||
export declare function attr<T extends AnyNode>(this: Cheerio<T>, name: string, value?: string | null | ((this: Element, i: number, attrib: string) => string | null)): Cheerio<T>; | ||
/** | ||
@@ -81,3 +81,3 @@ * Method for setting multiple attributes at once. Sets the attribute value for | ||
*/ | ||
export declare function attr<T extends Node>(this: Cheerio<T>, values: Record<string, string | null>): Cheerio<T>; | ||
export declare function attr<T extends AnyNode>(this: Cheerio<T>, values: Record<string, string | null>): Cheerio<T>; | ||
interface StyleProp { | ||
@@ -108,11 +108,26 @@ length: number; | ||
*/ | ||
export declare function prop<T extends Node>(this: Cheerio<T>, name: 'tagName' | 'nodeName'): T extends Element ? string : undefined; | ||
export declare function prop<T extends Node>(this: Cheerio<T>, name: 'innerHTML' | 'outerHTML'): string | null; | ||
export declare function prop<T extends Node>(this: Cheerio<T>, name: 'style'): StyleProp; | ||
export declare function prop<T extends Node, K extends keyof Element>(this: Cheerio<T>, name: K): Element[K]; | ||
export declare function prop<T extends Node, K extends keyof Element>(this: Cheerio<T>, name: K, value: Element[K] | ((this: Element, i: number, prop: K) => Element[keyof Element])): Cheerio<T>; | ||
export declare function prop<T extends Node>(this: Cheerio<T>, name: Record<string, string | Element[keyof Element] | boolean>): Cheerio<T>; | ||
export declare function prop<T extends Node>(this: Cheerio<T>, name: string, value: string | boolean | null | ((this: Element, i: number, prop: string) => string | boolean)): Cheerio<T>; | ||
export declare function prop<T extends Node>(this: Cheerio<T>, name: string): string; | ||
export declare function prop<T extends AnyNode>(this: Cheerio<T>, name: 'tagName' | 'nodeName'): T extends Element ? string : undefined; | ||
export declare function prop<T extends AnyNode>(this: Cheerio<T>, name: 'innerHTML' | 'outerHTML' | 'innerText' | 'textContent'): string | null; | ||
/** Get a parsed CSS style object. */ | ||
export declare function prop<T extends AnyNode>(this: Cheerio<T>, name: 'style'): StyleProp; | ||
/** | ||
* Resolve `href` or `src` of supported elements. Requires the `baseURI` option | ||
* to be set, and a global `URL` object to be part of the environment. | ||
* | ||
* @example With `baseURI` set to `'https://example.com'`: | ||
* | ||
* ```js | ||
* $('<img src="image.png">).prop('src'); | ||
* //=> 'https://example.com/image.png' | ||
* ``` | ||
*/ | ||
export declare function prop<T extends AnyNode>(this: Cheerio<T>, name: 'href' | 'src'): string | undefined; | ||
/** Get a property of an element. */ | ||
export declare function prop<T extends AnyNode, K extends keyof Element>(this: Cheerio<T>, name: K): Element[K]; | ||
/** Set a property of an element. */ | ||
export declare function prop<T extends AnyNode, K extends keyof Element>(this: Cheerio<T>, name: K, value: Element[K] | ((this: Element, i: number, prop: K) => Element[keyof Element])): Cheerio<T>; | ||
export declare function prop<T extends AnyNode>(this: Cheerio<T>, name: Record<string, string | Element[keyof Element] | boolean>): Cheerio<T>; | ||
export declare function prop<T extends AnyNode>(this: Cheerio<T>, name: string, value: string | boolean | null | ((this: Element, i: number, prop: string) => string | boolean)): Cheerio<T>; | ||
export declare function prop<T extends AnyNode>(this: Cheerio<T>, name: string): string; | ||
/** | ||
* Method for getting data attributes, for only the first element in the matched set. | ||
@@ -129,6 +144,6 @@ * | ||
* @param name - Name of the data attribute. | ||
* @returns The data attribute's value. | ||
* @returns The data attribute's value, or `undefined` if the attribute does not exist. | ||
* @see {@link https://api.jquery.com/data/} | ||
*/ | ||
export declare function data<T extends Node>(this: Cheerio<T>, name: string): unknown | undefined; | ||
export declare function data<T extends AnyNode>(this: Cheerio<T>, name: string): unknown | undefined; | ||
/** | ||
@@ -146,6 +161,6 @@ * Method for getting all of an element's data attributes, for only the first | ||
* | ||
* @returns The data attribute's values. | ||
* @returns A map with all of the data attributes. | ||
* @see {@link https://api.jquery.com/data/} | ||
*/ | ||
export declare function data<T extends Node>(this: Cheerio<T>): Record<string, unknown>; | ||
export declare function data<T extends AnyNode>(this: Cheerio<T>): Record<string, unknown>; | ||
/** | ||
@@ -169,3 +184,3 @@ * Method for setting data attributes, for only the first element in the matched set. | ||
*/ | ||
export declare function data<T extends Node>(this: Cheerio<T>, name: string, value: unknown): Cheerio<T>; | ||
export declare function data<T extends AnyNode>(this: Cheerio<T>, name: string, value: unknown): Cheerio<T>; | ||
/** | ||
@@ -189,3 +204,3 @@ * Method for setting multiple data attributes at once, for only the first | ||
*/ | ||
export declare function data<T extends Node>(this: Cheerio<T>, values: Record<string, unknown>): Cheerio<T>; | ||
export declare function data<T extends AnyNode>(this: Cheerio<T>, values: Record<string, unknown>): Cheerio<T>; | ||
/** | ||
@@ -206,3 +221,3 @@ * Method for getting the value of input, select, and textarea. Note: Support | ||
*/ | ||
export declare function val<T extends Node>(this: Cheerio<T>): string | undefined | string[]; | ||
export declare function val<T extends AnyNode>(this: Cheerio<T>): string | undefined | string[]; | ||
/** | ||
@@ -224,3 +239,3 @@ * Method for setting the value of input, select, and textarea. Note: Support | ||
*/ | ||
export declare function val<T extends Node>(this: Cheerio<T>, value: string | string[]): Cheerio<T>; | ||
export declare function val<T extends AnyNode>(this: Cheerio<T>, value: string | string[]): Cheerio<T>; | ||
/** | ||
@@ -245,5 +260,5 @@ * Method for removing attributes by `name`. | ||
*/ | ||
export declare function removeAttr<T extends Node>(this: Cheerio<T>, name: string): Cheerio<T>; | ||
export declare function removeAttr<T extends AnyNode>(this: Cheerio<T>, name: string): Cheerio<T>; | ||
/** | ||
* Check to see if *any* of the matched elements have the given `className`. | ||
* Check to see if _any_ of the matched elements have the given `className`. | ||
* | ||
@@ -268,3 +283,3 @@ * @category Attributes | ||
*/ | ||
export declare function hasClass<T extends Node>(this: Cheerio<T>, className: string): boolean; | ||
export declare function hasClass<T extends AnyNode>(this: Cheerio<T>, className: string): boolean; | ||
/** | ||
@@ -288,3 +303,3 @@ * Adds class(es) to all of the matched elements. Also accepts a `function`. | ||
*/ | ||
export declare function addClass<T extends Node, R extends ArrayLike<T>>(this: R, value?: string | ((this: Element, i: number, className: string) => string | undefined)): R; | ||
export declare function addClass<T extends AnyNode, R extends ArrayLike<T>>(this: R, value?: string | ((this: Element, i: number, className: string) => string | undefined)): R; | ||
/** | ||
@@ -309,3 +324,3 @@ * Removes one or more space-separated classes from the selected elements. If no | ||
*/ | ||
export declare function removeClass<T extends Node, R extends ArrayLike<T>>(this: R, name?: string | ((this: Element, i: number, className: string) => string | undefined)): R; | ||
export declare function removeClass<T extends AnyNode, R extends ArrayLike<T>>(this: R, name?: string | ((this: Element, i: number, className: string) => string | undefined)): R; | ||
/** | ||
@@ -331,4 +346,4 @@ * Add or remove class(es) from the matched elements, depending on either the | ||
*/ | ||
export declare function toggleClass<T extends Node, R extends ArrayLike<T>>(this: R, value?: string | ((this: Element, i: number, className: string, stateVal?: boolean) => string), stateVal?: boolean): R; | ||
export declare function toggleClass<T extends AnyNode, R extends ArrayLike<T>>(this: R, value?: string | ((this: Element, i: number, className: string, stateVal?: boolean) => string), stateVal?: boolean): R; | ||
export {}; | ||
//# sourceMappingURL=attributes.d.ts.map |
@@ -9,4 +9,5 @@ "use strict"; | ||
exports.toggleClass = exports.removeClass = exports.addClass = exports.hasClass = exports.removeAttr = exports.val = exports.data = exports.prop = exports.attr = void 0; | ||
var static_1 = require("../static"); | ||
var utils_1 = require("../utils"); | ||
var static_js_1 = require("../static.js"); | ||
var utils_js_1 = require("../utils.js"); | ||
var domutils_1 = require("domutils"); | ||
var hasOwn = Object.prototype.hasOwnProperty; | ||
@@ -30,3 +31,3 @@ var rspace = /\s+/; | ||
var _a; | ||
if (!elem || !utils_1.isTag(elem)) | ||
if (!elem || !(0, utils_js_1.isTag)(elem)) | ||
return undefined; | ||
@@ -44,7 +45,7 @@ (_a = elem.attribs) !== null && _a !== void 0 ? _a : (elem.attribs = {}); | ||
if (elem.name === 'option' && name === 'value') { | ||
return static_1.text(elem.children); | ||
return (0, static_js_1.text)(elem.children); | ||
} | ||
// Mimic DOM with default value for radios/checkboxes | ||
if (elem.name === 'input' && | ||
(elem.attribs.type === 'radio' || elem.attribs.type === 'checkbox') && | ||
(elem.attribs['type'] === 'radio' || elem.attribs['type'] === 'checkbox') && | ||
name === 'value') { | ||
@@ -68,3 +69,3 @@ return 'on'; | ||
else { | ||
el.attribs[name] = "" + value; | ||
el.attribs[name] = "".concat(value); | ||
} | ||
@@ -81,9 +82,9 @@ } | ||
} | ||
return utils_1.domEach(this, function (el, i) { | ||
if (utils_1.isTag(el)) | ||
return (0, utils_js_1.domEach)(this, function (el, i) { | ||
if ((0, utils_js_1.isTag)(el)) | ||
setAttr(el, name, value.call(el, i, el.attribs[name])); | ||
}); | ||
} | ||
return utils_1.domEach(this, function (el) { | ||
if (!utils_1.isTag(el)) | ||
return (0, utils_js_1.domEach)(this, function (el) { | ||
if (!(0, utils_js_1.isTag)(el)) | ||
return; | ||
@@ -111,3 +112,3 @@ if (typeof name === 'object') { | ||
* @category Attributes | ||
* @param el - Elenent to get the prop of. | ||
* @param el - Element to get the prop of. | ||
* @param name - Name of the prop. | ||
@@ -117,3 +118,3 @@ * @returns The prop's value. | ||
function getProp(el, name, xmlMode) { | ||
if (!el || !utils_1.isTag(el)) | ||
if (!el || !(0, utils_js_1.isTag)(el)) | ||
return; | ||
@@ -141,3 +142,3 @@ return name in el | ||
else { | ||
setAttr(el, name, !xmlMode && rboolean.test(name) ? (value ? '' : null) : "" + value); | ||
setAttr(el, name, !xmlMode && rboolean.test(name) ? (value ? '' : null) : "".concat(value)); | ||
} | ||
@@ -147,2 +148,3 @@ } | ||
var _this = this; | ||
var _a; | ||
if (typeof name === 'string' && value === undefined) { | ||
@@ -162,4 +164,31 @@ switch (name) { | ||
var el = this[0]; | ||
return utils_1.isTag(el) ? el.name.toUpperCase() : undefined; | ||
return (0, utils_js_1.isTag)(el) ? el.name.toUpperCase() : undefined; | ||
} | ||
case 'href': | ||
case 'src': { | ||
var el = this[0]; | ||
if (!(0, utils_js_1.isTag)(el)) { | ||
return undefined; | ||
} | ||
var prop_1 = (_a = el.attribs) === null || _a === void 0 ? void 0 : _a[name]; | ||
/* eslint-disable node/no-unsupported-features/node-builtins */ | ||
if (typeof URL !== 'undefined' && | ||
((name === 'href' && (el.tagName === 'a' || el.name === 'link')) || | ||
(name === 'src' && | ||
(el.tagName === 'img' || | ||
el.tagName === 'iframe' || | ||
el.tagName === 'audio' || | ||
el.tagName === 'video' || | ||
el.tagName === 'source'))) && | ||
prop_1 !== undefined && | ||
this.options.baseURI) { | ||
return new URL(prop_1, this.options.baseURI).href; | ||
} | ||
/* eslint-enable node/no-unsupported-features/node-builtins */ | ||
return prop_1; | ||
} | ||
case 'innerText': | ||
return (0, domutils_1.innerText)(this[0]); | ||
case 'textContent': | ||
return (0, domutils_1.textContent)(this[0]); | ||
case 'outerHTML': | ||
@@ -178,9 +207,10 @@ return this.clone().wrap('<container />').parent().html(); | ||
} | ||
return utils_1.domEach(this, function (el, i) { | ||
if (utils_1.isTag(el)) | ||
return (0, utils_js_1.domEach)(this, function (el, i) { | ||
if ((0, utils_js_1.isTag)(el)) { | ||
setProp(el, name, value.call(el, i, getProp(el, name, _this.options.xmlMode)), _this.options.xmlMode); | ||
} | ||
}); | ||
} | ||
return utils_1.domEach(this, function (el) { | ||
if (!utils_1.isTag(el)) | ||
return (0, utils_js_1.domEach)(this, function (el) { | ||
if (!(0, utils_js_1.isTag)(el)) | ||
return; | ||
@@ -222,9 +252,9 @@ if (typeof name === 'object') { | ||
* and (if present) cache the value in the node's internal data store. If no | ||
* attribute name is specified, read *all* HTML5 `data-*` attributes in this manner. | ||
* attribute name is specified, read _all_ HTML5 `data-*` attributes in this manner. | ||
* | ||
* @private | ||
* @category Attributes | ||
* @param el - Elenent to get the data attribute of. | ||
* @param el - Element to get the data attribute of. | ||
* @param name - Name of the data attribute. | ||
* @returns The data attribute's value, or a map with all of the data attribute. | ||
* @returns The data attribute's value, or a map with all of the data attributes. | ||
*/ | ||
@@ -240,7 +270,7 @@ function readData(el, name) { | ||
jsNames = domNames.map(function (domName) { | ||
return utils_1.camelCase(domName.slice(dataAttrPrefix.length)); | ||
return (0, utils_js_1.camelCase)(domName.slice(dataAttrPrefix.length)); | ||
}); | ||
} | ||
else { | ||
domNames = [dataAttrPrefix + utils_1.cssCase(name)]; | ||
domNames = [dataAttrPrefix + (0, utils_js_1.cssCase)(name)]; | ||
jsNames = [name]; | ||
@@ -276,3 +306,3 @@ } | ||
var elem = this[0]; | ||
if (!elem || !utils_1.isTag(elem)) | ||
if (!elem || !(0, utils_js_1.isTag)(elem)) | ||
return; | ||
@@ -287,4 +317,4 @@ var dataEl = elem; | ||
if (typeof name === 'object' || value !== undefined) { | ||
utils_1.domEach(this, function (el) { | ||
if (utils_1.isTag(el)) | ||
(0, utils_js_1.domEach)(this, function (el) { | ||
if ((0, utils_js_1.isTag)(el)) { | ||
if (typeof name === 'object') | ||
@@ -294,2 +324,3 @@ setData(el, name); | ||
setData(el, name, value); | ||
} | ||
}); | ||
@@ -307,3 +338,3 @@ return this; | ||
var element = this[0]; | ||
if (!element || !utils_1.isTag(element)) | ||
if (!element || !(0, utils_js_1.isTag)(element)) | ||
return querying ? undefined : this; | ||
@@ -322,3 +353,3 @@ switch (element.name) { | ||
for (var i = 0; i < values.length; i++) { | ||
this.find("option[value=\"" + values[i] + "\"]").attr('selected', ''); | ||
this.find("option[value=\"".concat(values[i], "\"]")).attr('selected', ''); | ||
} | ||
@@ -328,3 +359,3 @@ return this; | ||
return this.attr('multiple') | ||
? option.toArray().map(function (el) { return static_1.text(el.children); }) | ||
? option.toArray().map(function (el) { return (0, static_js_1.text)(el.children); }) | ||
: option.attr('value'); | ||
@@ -385,4 +416,4 @@ } | ||
var _loop_1 = function (i) { | ||
utils_1.domEach(this_1, function (elem) { | ||
if (utils_1.isTag(elem)) | ||
(0, utils_js_1.domEach)(this_1, function (elem) { | ||
if ((0, utils_js_1.isTag)(elem)) | ||
removeAttribute(elem, attrNames[i]); | ||
@@ -399,3 +430,3 @@ }); | ||
/** | ||
* Check to see if *any* of the matched elements have the given `className`. | ||
* Check to see if _any_ of the matched elements have the given `className`. | ||
* | ||
@@ -422,3 +453,3 @@ * @category Attributes | ||
return this.toArray().some(function (elem) { | ||
var clazz = utils_1.isTag(elem) && elem.attribs.class; | ||
var clazz = (0, utils_js_1.isTag)(elem) && elem.attribs['class']; | ||
var idx = -1; | ||
@@ -459,5 +490,5 @@ if (clazz && className.length) { | ||
if (typeof value === 'function') { | ||
return utils_1.domEach(this, function (el, i) { | ||
if (utils_1.isTag(el)) { | ||
var className = el.attribs.class || ''; | ||
return (0, utils_js_1.domEach)(this, function (el, i) { | ||
if ((0, utils_js_1.isTag)(el)) { | ||
var className = el.attribs['class'] || ''; | ||
addClass.call([el], value.call(el, i, className)); | ||
@@ -475,3 +506,3 @@ } | ||
// If selected element isn't a tag, move on | ||
if (!utils_1.isTag(el)) | ||
if (!(0, utils_js_1.isTag)(el)) | ||
continue; | ||
@@ -484,7 +515,7 @@ // If we don't already have classes — always set xmlMode to false here, as it doesn't matter for classes | ||
else { | ||
var setClass = " " + className + " "; | ||
var setClass = " ".concat(className, " "); | ||
// Check if class already exists | ||
for (var j = 0; j < classNames.length; j++) { | ||
var appendClass = classNames[j] + " "; | ||
if (!setClass.includes(" " + appendClass)) | ||
var appendClass = "".concat(classNames[j], " "); | ||
if (!setClass.includes(" ".concat(appendClass))) | ||
setClass += appendClass; | ||
@@ -520,5 +551,6 @@ } | ||
if (typeof name === 'function') { | ||
return utils_1.domEach(this, function (el, i) { | ||
if (utils_1.isTag(el)) | ||
removeClass.call([el], name.call(el, i, el.attribs.class || '')); | ||
return (0, utils_js_1.domEach)(this, function (el, i) { | ||
if ((0, utils_js_1.isTag)(el)) { | ||
removeClass.call([el], name.call(el, i, el.attribs['class'] || '')); | ||
} | ||
}); | ||
@@ -529,11 +561,11 @@ } | ||
var removeAll = arguments.length === 0; | ||
return utils_1.domEach(this, function (el) { | ||
if (!utils_1.isTag(el)) | ||
return (0, utils_js_1.domEach)(this, function (el) { | ||
if (!(0, utils_js_1.isTag)(el)) | ||
return; | ||
if (removeAll) { | ||
// Short circuit the remove all case as this is the nice one | ||
el.attribs.class = ''; | ||
el.attribs['class'] = ''; | ||
} | ||
else { | ||
var elClasses = splitNames(el.attribs.class); | ||
var elClasses = splitNames(el.attribs['class']); | ||
var changed = false; | ||
@@ -553,3 +585,3 @@ for (var j = 0; j < numClasses; j++) { | ||
if (changed) { | ||
el.attribs.class = elClasses.join(' '); | ||
el.attribs['class'] = elClasses.join(' '); | ||
} | ||
@@ -583,5 +615,5 @@ } | ||
if (typeof value === 'function') { | ||
return utils_1.domEach(this, function (el, i) { | ||
if (utils_1.isTag(el)) { | ||
toggleClass.call([el], value.call(el, i, el.attribs.class || '', stateVal), stateVal); | ||
return (0, utils_js_1.domEach)(this, function (el, i) { | ||
if ((0, utils_js_1.isTag)(el)) { | ||
toggleClass.call([el], value.call(el, i, el.attribs['class'] || '', stateVal), stateVal); | ||
} | ||
@@ -600,5 +632,5 @@ }); | ||
// If selected element isn't a tag, move on | ||
if (!utils_1.isTag(el)) | ||
if (!(0, utils_js_1.isTag)(el)) | ||
continue; | ||
var elementClasses = splitNames(el.attribs.class); | ||
var elementClasses = splitNames(el.attribs['class']); | ||
// Check if class already exists | ||
@@ -617,3 +649,3 @@ for (var j = 0; j < numClasses; j++) { | ||
} | ||
el.attribs.class = elementClasses.join(' '); | ||
el.attribs['class'] = elementClasses.join(' '); | ||
} | ||
@@ -623,1 +655,2 @@ return this; | ||
exports.toggleClass = toggleClass; | ||
//# sourceMappingURL=attributes.js.map |
@@ -1,3 +0,3 @@ | ||
import type { Element, Node } from 'domhandler'; | ||
import type { Cheerio } from '../cheerio'; | ||
import type { Element, AnyNode } from 'domhandler'; | ||
import type { Cheerio } from '../cheerio.js'; | ||
/** | ||
@@ -7,7 +7,7 @@ * Get the value of a style property for the first element in the set of matched elements. | ||
* @category CSS | ||
* @param names - Optionally the names of the property of interest. | ||
* @param names - Optionally the names of the properties of interest. | ||
* @returns A map of all of the style properties. | ||
* @see {@link https://api.jquery.com/css/} | ||
*/ | ||
export declare function css<T extends Node>(this: Cheerio<T>, names?: string[]): Record<string, string>; | ||
export declare function css<T extends AnyNode>(this: Cheerio<T>, names?: string[]): Record<string, string> | undefined; | ||
/** | ||
@@ -21,3 +21,3 @@ * Get the value of a style property for the first element in the set of matched elements. | ||
*/ | ||
export declare function css<T extends Node>(this: Cheerio<T>, name: string): string | undefined; | ||
export declare function css<T extends AnyNode>(this: Cheerio<T>, name: string): string | undefined; | ||
/** | ||
@@ -32,3 +32,3 @@ * Set one CSS property for every matched element. | ||
*/ | ||
export declare function css<T extends Node>(this: Cheerio<T>, prop: string, val: string | ((this: Element, i: number, style: string) => string | undefined)): Cheerio<T>; | ||
export declare function css<T extends AnyNode>(this: Cheerio<T>, prop: string, val: string | ((this: Element, i: number, style: string) => string | undefined)): Cheerio<T>; | ||
/** | ||
@@ -38,8 +38,7 @@ * Set multiple CSS properties for every matched element. | ||
* @category CSS | ||
* @param prop - The name of the property. | ||
* @param val - The new value. | ||
* @param map - A map of property names and values. | ||
* @returns The instance itself. | ||
* @see {@link https://api.jquery.com/css/} | ||
*/ | ||
export declare function css<T extends Node>(this: Cheerio<T>, prop: Record<string, string>): Cheerio<T>; | ||
export declare function css<T extends AnyNode>(this: Cheerio<T>, map: Record<string, string>): Cheerio<T>; | ||
//# sourceMappingURL=css.d.ts.map |
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
exports.css = void 0; | ||
var utils_1 = require("../utils"); | ||
var utils_js_1 = require("../utils.js"); | ||
/** | ||
* Set multiple CSS properties for every matched element. | ||
* | ||
* @category CSS | ||
* @param prop - The names of the properties. | ||
* @param val - The new values. | ||
* @returns The instance itself. | ||
* @see {@link https://api.jquery.com/css/} | ||
*/ | ||
function css(prop, val) { | ||
@@ -9,4 +18,4 @@ if ((prop != null && val != null) || | ||
(typeof prop === 'object' && !Array.isArray(prop))) { | ||
return utils_1.domEach(this, function (el, i) { | ||
if (utils_1.isTag(el)) { | ||
return (0, utils_js_1.domEach)(this, function (el, i) { | ||
if ((0, utils_js_1.isTag)(el)) { | ||
// `prop` can't be an array here anymore. | ||
@@ -17,2 +26,5 @@ setCss(el, prop, val, i); | ||
} | ||
if (this.length === 0) { | ||
return undefined; | ||
} | ||
return getCss(this[0], prop); | ||
@@ -40,3 +52,3 @@ } | ||
} | ||
el.attribs.style = stringify(styles); | ||
el.attribs['style'] = stringify(styles); | ||
} | ||
@@ -50,5 +62,5 @@ else if (typeof prop === 'object') { | ||
function getCss(el, prop) { | ||
if (!el || !utils_1.isTag(el)) | ||
if (!el || !(0, utils_js_1.isTag)(el)) | ||
return; | ||
var styles = parse(el.attribs.style); | ||
var styles = parse(el.attribs['style']); | ||
if (typeof prop === 'string') { | ||
@@ -77,3 +89,3 @@ return styles[prop]; | ||
function stringify(obj) { | ||
return Object.keys(obj).reduce(function (str, prop) { return "" + str + (str ? ' ' : '') + prop + ": " + obj[prop] + ";"; }, ''); | ||
return Object.keys(obj).reduce(function (str, prop) { return "".concat(str).concat(str ? ' ' : '').concat(prop, ": ").concat(obj[prop], ";"); }, ''); | ||
} | ||
@@ -92,10 +104,21 @@ /** | ||
return {}; | ||
return styles.split(';').reduce(function (obj, str) { | ||
var obj = {}; | ||
var key; | ||
for (var _i = 0, _a = styles.split(';'); _i < _a.length; _i++) { | ||
var str = _a[_i]; | ||
var n = str.indexOf(':'); | ||
// Skip if there is no :, or if it is the first/last character | ||
if (n < 1 || n === str.length - 1) | ||
return obj; | ||
obj[str.slice(0, n).trim()] = str.slice(n + 1).trim(); | ||
return obj; | ||
}, {}); | ||
// If there is no :, or if it is the first/last character, add to the previous item's value | ||
if (n < 1 || n === str.length - 1) { | ||
var trimmed = str.trimEnd(); | ||
if (trimmed.length > 0 && key !== undefined) { | ||
obj[key] += ";".concat(trimmed); | ||
} | ||
} | ||
else { | ||
key = str.slice(0, n).trim(); | ||
obj[key] = str.slice(n + 1).trim(); | ||
} | ||
} | ||
return obj; | ||
} | ||
//# sourceMappingURL=css.js.map |
@@ -1,3 +0,3 @@ | ||
import type { Node } from 'domhandler'; | ||
import type { Cheerio } from '../cheerio'; | ||
import type { AnyNode } from 'domhandler'; | ||
import type { Cheerio } from '../cheerio.js'; | ||
/** | ||
@@ -7,6 +7,13 @@ * Encode a set of form elements as a string for submission. | ||
* @category Forms | ||
* @example | ||
* | ||
* ```js | ||
* $('<form><input name="foo" value="bar" /></form>').serialize(); | ||
* //=> 'foo=bar' | ||
* ``` | ||
* | ||
* @returns The serialized form. | ||
* @see {@link https://api.jquery.com/serialize/} | ||
*/ | ||
export declare function serialize<T extends Node>(this: Cheerio<T>): string; | ||
export declare function serialize<T extends AnyNode>(this: Cheerio<T>): string; | ||
interface SerializedField { | ||
@@ -30,4 +37,4 @@ name: string; | ||
*/ | ||
export declare function serializeArray<T extends Node>(this: Cheerio<T>): SerializedField[]; | ||
export declare function serializeArray<T extends AnyNode>(this: Cheerio<T>): SerializedField[]; | ||
export {}; | ||
//# sourceMappingURL=forms.d.ts.map |
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
exports.serializeArray = exports.serialize = void 0; | ||
var utils_1 = require("../utils"); | ||
var utils_js_1 = require("../utils.js"); | ||
/* | ||
@@ -16,2 +16,9 @@ * https://github.com/jquery/jquery/blob/2.1.3/src/manipulation/var/rcheckableType.js | ||
* @category Forms | ||
* @example | ||
* | ||
* ```js | ||
* $('<form><input name="foo" value="bar" /></form>').serialize(); | ||
* //=> 'foo=bar' | ||
* ``` | ||
* | ||
* @returns The serialized form. | ||
@@ -25,3 +32,3 @@ * @see {@link https://api.jquery.com/serialize/} | ||
var retArr = arr.map(function (data) { | ||
return encodeURIComponent(data.name) + "=" + encodeURIComponent(data.value); | ||
return "".concat(encodeURIComponent(data.name), "=").concat(encodeURIComponent(data.value)); | ||
}); | ||
@@ -51,3 +58,3 @@ // Return the resulting serialization | ||
var $elem = _this._make(elem); | ||
if (utils_1.isTag(elem) && elem.name === 'form') { | ||
if ((0, utils_js_1.isTag)(elem) && elem.name === 'form') { | ||
return $elem.find(submittableSelector).toArray(); | ||
@@ -88,1 +95,2 @@ } | ||
exports.serializeArray = serializeArray; | ||
//# sourceMappingURL=forms.js.map |
@@ -6,5 +6,5 @@ /** | ||
*/ | ||
import { Node } from 'domhandler'; | ||
import type { Cheerio } from '../cheerio'; | ||
import type { BasicAcceptedElems, AcceptedElems } from '../types'; | ||
import { AnyNode } from 'domhandler'; | ||
import type { Cheerio } from '../cheerio.js'; | ||
import type { BasicAcceptedElems, AcceptedElems } from '../types.js'; | ||
/** | ||
@@ -19,3 +19,3 @@ * Create an array of nodes, recursing into arrays and parsing strings if necessary. | ||
*/ | ||
export declare function _makeDomArray<T extends Node>(this: Cheerio<T>, elem?: BasicAcceptedElems<Node>, clone?: boolean): Node[]; | ||
export declare function _makeDomArray<T extends AnyNode>(this: Cheerio<T>, elem?: BasicAcceptedElems<AnyNode>, clone?: boolean): AnyNode[]; | ||
/** | ||
@@ -42,3 +42,3 @@ * Insert every element in the set of matched elements to the end of the target. | ||
*/ | ||
export declare function appendTo<T extends Node>(this: Cheerio<T>, target: BasicAcceptedElems<Node>): Cheerio<T>; | ||
export declare function appendTo<T extends AnyNode>(this: Cheerio<T>, target: BasicAcceptedElems<AnyNode>): Cheerio<T>; | ||
/** | ||
@@ -65,5 +65,5 @@ * Insert every element in the set of matched elements to the beginning of the target. | ||
*/ | ||
export declare function prependTo<T extends Node>(this: Cheerio<T>, target: BasicAcceptedElems<Node>): Cheerio<T>; | ||
export declare function prependTo<T extends AnyNode>(this: Cheerio<T>, target: BasicAcceptedElems<AnyNode>): Cheerio<T>; | ||
/** | ||
* Inserts content as the *last* child of each of the selected elements. | ||
* Inserts content as the _last_ child of each of the selected elements. | ||
* | ||
@@ -86,5 +86,5 @@ * @category Manipulation | ||
*/ | ||
export declare const append: <T extends Node>(this: Cheerio<T>, ...elems: [(this: Node, i: number, html: string) => BasicAcceptedElems<Node>] | BasicAcceptedElems<Node>[]) => Cheerio<T>; | ||
export declare const append: <T extends AnyNode>(this: Cheerio<T>, ...elems: [(this: AnyNode, i: number, html: string) => BasicAcceptedElems<AnyNode>] | BasicAcceptedElems<AnyNode>[]) => Cheerio<T>; | ||
/** | ||
* Inserts content as the *first* child of each of the selected elements. | ||
* Inserts content as the _first_ child of each of the selected elements. | ||
* | ||
@@ -107,3 +107,3 @@ * @category Manipulation | ||
*/ | ||
export declare const prepend: <T extends Node>(this: Cheerio<T>, ...elems: [(this: Node, i: number, html: string) => BasicAcceptedElems<Node>] | BasicAcceptedElems<Node>[]) => Cheerio<T>; | ||
export declare const prepend: <T extends AnyNode>(this: Cheerio<T>, ...elems: [(this: AnyNode, i: number, html: string) => BasicAcceptedElems<AnyNode>] | BasicAcceptedElems<AnyNode>[]) => Cheerio<T>; | ||
/** | ||
@@ -151,3 +151,3 @@ * The .wrap() function can take any string or object that could be passed to | ||
*/ | ||
export declare const wrap: <T extends Node>(this: Cheerio<T>, wrapper: AcceptedElems<Node>) => Cheerio<T>; | ||
export declare const wrap: <T extends AnyNode>(this: Cheerio<T>, wrapper: AcceptedElems<AnyNode>) => Cheerio<T>; | ||
/** | ||
@@ -196,3 +196,3 @@ * The .wrapInner() function can take any string or object that could be passed | ||
*/ | ||
export declare const wrapInner: <T extends Node>(this: Cheerio<T>, wrapper: AcceptedElems<Node>) => Cheerio<T>; | ||
export declare const wrapInner: <T extends AnyNode>(this: Cheerio<T>, wrapper: AcceptedElems<AnyNode>) => Cheerio<T>; | ||
/** | ||
@@ -236,3 +236,3 @@ * The .unwrap() function, removes the parents of the set of matched elements | ||
*/ | ||
export declare function unwrap<T extends Node>(this: Cheerio<T>, selector?: string): Cheerio<T>; | ||
export declare function unwrap<T extends AnyNode>(this: Cheerio<T>, selector?: string): Cheerio<T>; | ||
/** | ||
@@ -289,3 +289,3 @@ * The .wrapAll() function can take any string or object that could be passed to | ||
*/ | ||
export declare function wrapAll<T extends Node>(this: Cheerio<T>, wrapper: AcceptedElems<T>): Cheerio<T>; | ||
export declare function wrapAll<T extends AnyNode>(this: Cheerio<T>, wrapper: AcceptedElems<T>): Cheerio<T>; | ||
/** | ||
@@ -313,3 +313,3 @@ * Insert content next to each element in the set of matched elements. | ||
*/ | ||
export declare function after<T extends Node>(this: Cheerio<T>, ...elems: [(this: Node, i: number, html: string) => BasicAcceptedElems<Node>] | BasicAcceptedElems<Node>[]): Cheerio<T>; | ||
export declare function after<T extends AnyNode>(this: Cheerio<T>, ...elems: [(this: AnyNode, i: number, html: string) => BasicAcceptedElems<AnyNode>] | BasicAcceptedElems<AnyNode>[]): Cheerio<T>; | ||
/** | ||
@@ -336,3 +336,3 @@ * Insert every element in the set of matched elements after the target. | ||
*/ | ||
export declare function insertAfter<T extends Node>(this: Cheerio<T>, target: BasicAcceptedElems<Node>): Cheerio<T>; | ||
export declare function insertAfter<T extends AnyNode>(this: Cheerio<T>, target: BasicAcceptedElems<AnyNode>): Cheerio<T>; | ||
/** | ||
@@ -360,3 +360,3 @@ * Insert content previous to each element in the set of matched elements. | ||
*/ | ||
export declare function before<T extends Node>(this: Cheerio<T>, ...elems: [(this: Node, i: number, html: string) => BasicAcceptedElems<Node>] | BasicAcceptedElems<Node>[]): Cheerio<T>; | ||
export declare function before<T extends AnyNode>(this: Cheerio<T>, ...elems: [(this: AnyNode, i: number, html: string) => BasicAcceptedElems<AnyNode>] | BasicAcceptedElems<AnyNode>[]): Cheerio<T>; | ||
/** | ||
@@ -383,3 +383,3 @@ * Insert every element in the set of matched elements before the target. | ||
*/ | ||
export declare function insertBefore<T extends Node>(this: Cheerio<T>, target: BasicAcceptedElems<Node>): Cheerio<T>; | ||
export declare function insertBefore<T extends AnyNode>(this: Cheerio<T>, target: BasicAcceptedElems<AnyNode>): Cheerio<T>; | ||
/** | ||
@@ -405,3 +405,3 @@ * Removes the set of matched elements from the DOM and all their children. | ||
*/ | ||
export declare function remove<T extends Node>(this: Cheerio<T>, selector?: string): Cheerio<T>; | ||
export declare function remove<T extends AnyNode>(this: Cheerio<T>, selector?: string): Cheerio<T>; | ||
/** | ||
@@ -428,3 +428,3 @@ * Replaces matched elements with `content`. | ||
*/ | ||
export declare function replaceWith<T extends Node>(this: Cheerio<T>, content: AcceptedElems<Node>): Cheerio<T>; | ||
export declare function replaceWith<T extends AnyNode>(this: Cheerio<T>, content: AcceptedElems<AnyNode>): Cheerio<T>; | ||
/** | ||
@@ -445,6 +445,5 @@ * Empties an element, removing all its children. | ||
*/ | ||
export declare function empty<T extends Node>(this: Cheerio<T>): Cheerio<T>; | ||
export declare function empty<T extends AnyNode>(this: Cheerio<T>): Cheerio<T>; | ||
/** | ||
* Gets an HTML content string from the first selected element. If `htmlString` | ||
* is specified, each selected element's content is replaced by the new content. | ||
* Gets an HTML content string from the first selected element. | ||
* | ||
@@ -462,8 +461,22 @@ * @category Manipulation | ||
* | ||
* @param str - If specified used to replace selection's contents. | ||
* @returns The HTML content string. | ||
* @see {@link https://api.jquery.com/html/} | ||
*/ | ||
export declare function html<T extends AnyNode>(this: Cheerio<T>): string | null; | ||
/** | ||
* Replaces each selected element's content with the specified content. | ||
* | ||
* @category Manipulation | ||
* @example | ||
* | ||
* ```js | ||
* $('.orange').html('<li class="mango">Mango</li>').html(); | ||
* //=> <li class="mango">Mango</li> | ||
* ``` | ||
* | ||
* @param str - The content to replace selection's contents with. | ||
* @returns The instance itself. | ||
* @see {@link https://api.jquery.com/html/} | ||
*/ | ||
export declare function html<T extends Node>(this: Cheerio<T>): string | null; | ||
export declare function html<T extends Node>(this: Cheerio<T>, str: string | Cheerio<T>): Cheerio<T>; | ||
export declare function html<T extends AnyNode>(this: Cheerio<T>, str: string | Cheerio<T>): Cheerio<T>; | ||
/** | ||
@@ -475,7 +488,6 @@ * Turns the collection to a string. Alias for `.html()`. | ||
*/ | ||
export declare function toString<T extends Node>(this: Cheerio<T>): string; | ||
export declare function toString<T extends AnyNode>(this: Cheerio<T>): string; | ||
/** | ||
* Get the combined text contents of each element in the set of matched | ||
* elements, including their descendants. If `textString` is specified, each | ||
* selected element's content is replaced by the new text content. | ||
* elements, including their descendants. | ||
* | ||
@@ -495,9 +507,23 @@ * @category Manipulation | ||
* | ||
* @param str - If specified replacement for the selected element's contents. | ||
* @returns The instance itself when setting text, otherwise the rendered document. | ||
* @returns The text contents of the collection. | ||
* @see {@link https://api.jquery.com/text/} | ||
*/ | ||
export declare function text<T extends Node>(this: Cheerio<T>): string; | ||
export declare function text<T extends Node>(this: Cheerio<T>, str: string | ((this: Node, i: number, text: string) => string)): Cheerio<T>; | ||
export declare function text<T extends AnyNode>(this: Cheerio<T>): string; | ||
/** | ||
* Set the content of each element in the set of matched elements to the specified text. | ||
* | ||
* @category Manipulation | ||
* @example | ||
* | ||
* ```js | ||
* $('.orange').text('Orange'); | ||
* //=> <div class="orange">Orange</div> | ||
* ``` | ||
* | ||
* @param str - The text to set as the content of each matched element. | ||
* @returns The instance itself. | ||
* @see {@link https://api.jquery.com/text/} | ||
*/ | ||
export declare function text<T extends AnyNode>(this: Cheerio<T>, str: string | ((this: AnyNode, i: number, text: string) => string)): Cheerio<T>; | ||
/** | ||
* Clone the cheerio object. | ||
@@ -515,3 +541,3 @@ * | ||
*/ | ||
export declare function clone<T extends Node>(this: Cheerio<T>): Cheerio<T>; | ||
export declare function clone<T extends AnyNode>(this: Cheerio<T>): Cheerio<T>; | ||
//# sourceMappingURL=manipulation.d.ts.map |
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
exports.clone = exports.text = exports.toString = exports.html = exports.empty = exports.replaceWith = exports.remove = exports.insertBefore = exports.before = exports.insertAfter = exports.after = exports.wrapAll = exports.unwrap = exports.wrapInner = exports.wrap = exports.prepend = exports.append = exports.prependTo = exports.appendTo = exports._makeDomArray = void 0; | ||
var tslib_1 = require("tslib"); | ||
var domhandler_1 = require("domhandler"); | ||
/** | ||
@@ -11,7 +7,18 @@ * Methods for modifying the DOM structure. | ||
*/ | ||
var domhandler_2 = require("domhandler"); | ||
var parse_1 = tslib_1.__importStar(require("../parse")); | ||
var static_1 = require("../static"); | ||
var utils_1 = require("../utils"); | ||
var htmlparser2_1 = require("htmlparser2"); | ||
var __spreadArray = (this && this.__spreadArray) || function (to, from, pack) { | ||
if (pack || arguments.length === 2) for (var i = 0, l = from.length, ar; i < l; i++) { | ||
if (ar || !(i in from)) { | ||
if (!ar) ar = Array.prototype.slice.call(from, 0, i); | ||
ar[i] = from[i]; | ||
} | ||
} | ||
return to.concat(ar || Array.prototype.slice.call(from)); | ||
}; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
exports.clone = exports.text = exports.toString = exports.html = exports.empty = exports.replaceWith = exports.remove = exports.insertBefore = exports.before = exports.insertAfter = exports.after = exports.wrapAll = exports.unwrap = exports.wrapInner = exports.wrap = exports.prepend = exports.append = exports.prependTo = exports.appendTo = exports._makeDomArray = void 0; | ||
var domhandler_1 = require("domhandler"); | ||
var parse_js_1 = require("../parse.js"); | ||
var static_js_1 = require("../static.js"); | ||
var utils_js_1 = require("../utils.js"); | ||
var domutils_1 = require("domutils"); | ||
/** | ||
@@ -31,4 +38,4 @@ * Create an array of nodes, recursing into arrays and parsing strings if necessary. | ||
} | ||
if (utils_1.isCheerio(elem)) { | ||
return clone ? utils_1.cloneDom(elem.get()) : elem.get(); | ||
if ((0, utils_js_1.isCheerio)(elem)) { | ||
return clone ? (0, utils_js_1.cloneDom)(elem.get()) : elem.get(); | ||
} | ||
@@ -39,5 +46,5 @@ if (Array.isArray(elem)) { | ||
if (typeof elem === 'string') { | ||
return parse_1.default(elem, this.options, false).children; | ||
return this._parse(elem, this.options, false, null).children; | ||
} | ||
return clone ? utils_1.cloneDom([elem]) : [elem]; | ||
return clone ? (0, utils_js_1.cloneDom)([elem]) : [elem]; | ||
} | ||
@@ -53,7 +60,7 @@ exports._makeDomArray = _makeDomArray; | ||
var lastIdx = this.length - 1; | ||
return utils_1.domEach(this, function (el, i) { | ||
if (!domhandler_1.hasChildren(el)) | ||
return (0, utils_js_1.domEach)(this, function (el, i) { | ||
if (!(0, domhandler_1.hasChildren)(el)) | ||
return; | ||
var domSrc = typeof elems[0] === 'function' | ||
? elems[0].call(el, i, static_1.html(el.children)) | ||
? elems[0].call(el, i, _this._render(el.children)) | ||
: elems; | ||
@@ -80,8 +87,10 @@ var dom = _this._makeDomArray(domSrc, i < lastIdx); | ||
var _a, _b; | ||
var spliceArgs = tslib_1.__spreadArray([ | ||
var spliceArgs = __spreadArray([ | ||
spliceIdx, | ||
spliceCount | ||
], newElems); | ||
var prev = array[spliceIdx - 1] || null; | ||
var next = array[spliceIdx + spliceCount] || null; | ||
], newElems, true); | ||
var prev = spliceIdx === 0 ? null : array[spliceIdx - 1]; | ||
var next = spliceIdx + spliceCount >= array.length | ||
? null | ||
: array[spliceIdx + spliceCount]; | ||
/* | ||
@@ -95,3 +104,4 @@ * Before splicing in new elements, ensure they do not already appear in the | ||
if (oldParent) { | ||
var prevIdx = oldParent.children.indexOf(newElems[idx]); | ||
var oldSiblings = oldParent.children; | ||
var prevIdx = oldSiblings.indexOf(node); | ||
if (prevIdx > -1) { | ||
@@ -111,4 +121,4 @@ oldParent.children.splice(prevIdx, 1); | ||
} | ||
node.prev = newElems[idx - 1] || prev; | ||
node.next = newElems[idx + 1] || next; | ||
node.prev = idx === 0 ? prev : newElems[idx - 1]; | ||
node.next = idx === newElems.length - 1 ? next : newElems[idx + 1]; | ||
} | ||
@@ -145,3 +155,3 @@ if (prev) { | ||
function appendTo(target) { | ||
var appendTarget = utils_1.isCheerio(target) ? target : this._make(target); | ||
var appendTarget = (0, utils_js_1.isCheerio)(target) ? target : this._make(target); | ||
appendTarget.append(this); | ||
@@ -173,3 +183,3 @@ return this; | ||
function prependTo(target) { | ||
var prependTarget = utils_1.isCheerio(target) ? target : this._make(target); | ||
var prependTarget = (0, utils_js_1.isCheerio)(target) ? target : this._make(target); | ||
prependTarget.prepend(this); | ||
@@ -180,3 +190,3 @@ return this; | ||
/** | ||
* Inserts content as the *last* child of each of the selected elements. | ||
* Inserts content as the _last_ child of each of the selected elements. | ||
* | ||
@@ -203,3 +213,3 @@ * @category Manipulation | ||
/** | ||
* Inserts content as the *first* child of each of the selected elements. | ||
* Inserts content as the _first_ child of each of the selected elements. | ||
* | ||
@@ -233,7 +243,7 @@ * @category Manipulation | ||
? wrapper.call(el, i, el) | ||
: typeof wrapper === 'string' && !utils_1.isHtml(wrapper) | ||
: typeof wrapper === 'string' && !(0, utils_js_1.isHtml)(wrapper) | ||
? lastParent.find(wrapper).clone() | ||
: wrapper; | ||
var wrapperDom = this._makeDomArray(wrap_1, i < lastIdx)[0]; | ||
if (!wrapperDom || !htmlparser2_1.DomUtils.hasChildren(wrapperDom)) | ||
if (!wrapperDom || !(0, domhandler_1.hasChildren)(wrapperDom)) | ||
continue; | ||
@@ -248,3 +258,3 @@ var elInsertLocation = wrapperDom; | ||
var child = elInsertLocation.children[j]; | ||
if (utils_1.isTag(child)) { | ||
if ((0, utils_js_1.isTag)(child)) { | ||
elInsertLocation = child; | ||
@@ -310,3 +320,3 @@ j = 0; | ||
var index = siblings.indexOf(el); | ||
parse_1.update([el], elInsertLocation); | ||
(0, parse_js_1.update)([el], elInsertLocation); | ||
/* | ||
@@ -363,6 +373,6 @@ * The previous operation removed the current element from the `siblings` | ||
exports.wrapInner = _wrap(function (el, elInsertLocation, wrapperDom) { | ||
if (!domhandler_1.hasChildren(el)) | ||
if (!(0, domhandler_1.hasChildren)(el)) | ||
return; | ||
parse_1.update(el.children, elInsertLocation); | ||
parse_1.update(wrapperDom, el); | ||
(0, parse_js_1.update)(el.children, elInsertLocation); | ||
(0, parse_js_1.update)(wrapperDom, el); | ||
}); | ||
@@ -529,5 +539,5 @@ /** | ||
var lastIdx = this.length - 1; | ||
return utils_1.domEach(this, function (el, i) { | ||
return (0, utils_js_1.domEach)(this, function (el, i) { | ||
var parent = el.parent; | ||
if (!htmlparser2_1.DomUtils.hasChildren(el) || !parent) { | ||
if (!(0, domhandler_1.hasChildren)(el) || !parent) { | ||
return; | ||
@@ -542,3 +552,3 @@ } | ||
var domSrc = typeof elems[0] === 'function' | ||
? elems[0].call(el, i, static_1.html(el.children)) | ||
? elems[0].call(el, i, _this._render(el.children)) | ||
: elems; | ||
@@ -629,5 +639,5 @@ var dom = _this._makeDomArray(domSrc, i < lastIdx); | ||
var lastIdx = this.length - 1; | ||
return utils_1.domEach(this, function (el, i) { | ||
return (0, utils_js_1.domEach)(this, function (el, i) { | ||
var parent = el.parent; | ||
if (!htmlparser2_1.DomUtils.hasChildren(el) || !parent) { | ||
if (!(0, domhandler_1.hasChildren)(el) || !parent) { | ||
return; | ||
@@ -642,3 +652,3 @@ } | ||
var domSrc = typeof elems[0] === 'function' | ||
? elems[0].call(el, i, static_1.html(el.children)) | ||
? elems[0].call(el, i, _this._render(el.children)) | ||
: elems; | ||
@@ -678,3 +688,3 @@ var dom = _this._makeDomArray(domSrc, i < lastIdx); | ||
var clones = []; | ||
utils_1.domEach(targetArr, function (el) { | ||
(0, utils_js_1.domEach)(targetArr, function (el) { | ||
var clonedSelf = _this.clone().toArray(); | ||
@@ -721,4 +731,4 @@ var parent = el.parent; | ||
var elems = selector ? this.filter(selector) : this; | ||
utils_1.domEach(elems, function (el) { | ||
htmlparser2_1.DomUtils.removeElement(el); | ||
(0, utils_js_1.domEach)(elems, function (el) { | ||
(0, domutils_1.removeElement)(el); | ||
el.prev = el.next = el.parent = null; | ||
@@ -752,3 +762,3 @@ }); | ||
var _this = this; | ||
return utils_1.domEach(this, function (el, i) { | ||
return (0, utils_js_1.domEach)(this, function (el, i) { | ||
var parent = el.parent; | ||
@@ -765,3 +775,3 @@ if (!parent) { | ||
*/ | ||
parse_1.update(dom, null); | ||
(0, parse_js_1.update)(dom, null); | ||
var index = siblings.indexOf(el); | ||
@@ -792,4 +802,4 @@ // Completely remove old element | ||
function empty() { | ||
return utils_1.domEach(this, function (el) { | ||
if (!htmlparser2_1.DomUtils.hasChildren(el)) | ||
return (0, utils_js_1.domEach)(this, function (el) { | ||
if (!(0, domhandler_1.hasChildren)(el)) | ||
return; | ||
@@ -804,12 +814,11 @@ el.children.forEach(function (child) { | ||
function html(str) { | ||
var _this = this; | ||
if (str === undefined) { | ||
var el = this[0]; | ||
if (!el || !htmlparser2_1.DomUtils.hasChildren(el)) | ||
if (!el || !(0, domhandler_1.hasChildren)(el)) | ||
return null; | ||
return static_1.html(el.children, this.options); | ||
return this._render(el.children); | ||
} | ||
// Keep main options unchanged | ||
var opts = tslib_1.__assign(tslib_1.__assign({}, this.options), { context: null }); | ||
return utils_1.domEach(this, function (el) { | ||
if (!htmlparser2_1.DomUtils.hasChildren(el)) | ||
return (0, utils_js_1.domEach)(this, function (el) { | ||
if (!(0, domhandler_1.hasChildren)(el)) | ||
return; | ||
@@ -819,7 +828,6 @@ el.children.forEach(function (child) { | ||
}); | ||
opts.context = el; | ||
var content = utils_1.isCheerio(str) | ||
var content = (0, utils_js_1.isCheerio)(str) | ||
? str.toArray() | ||
: parse_1.default("" + str, opts, false).children; | ||
parse_1.update(content, el); | ||
: _this._parse("".concat(str), _this.options, false, el).children; | ||
(0, parse_js_1.update)(content, el); | ||
}); | ||
@@ -835,3 +843,3 @@ } | ||
function toString() { | ||
return static_1.html(this, this.options); | ||
return this._render(this); | ||
} | ||
@@ -843,13 +851,13 @@ exports.toString = toString; | ||
if (str === undefined) { | ||
return static_1.text(this); | ||
return (0, static_js_1.text)(this); | ||
} | ||
if (typeof str === 'function') { | ||
// Function support | ||
return utils_1.domEach(this, function (el, i) { | ||
text.call(_this._make(el), str.call(el, i, static_1.text([el]))); | ||
return (0, utils_js_1.domEach)(this, function (el, i) { | ||
return _this._make(el).text(str.call(el, i, (0, static_js_1.text)([el]))); | ||
}); | ||
} | ||
// Append text node to each selected elements | ||
return utils_1.domEach(this, function (el) { | ||
if (!htmlparser2_1.DomUtils.hasChildren(el)) | ||
return (0, utils_js_1.domEach)(this, function (el) { | ||
if (!(0, domhandler_1.hasChildren)(el)) | ||
return; | ||
@@ -859,4 +867,4 @@ el.children.forEach(function (child) { | ||
}); | ||
var textNode = new domhandler_2.Text(str); | ||
parse_1.update(textNode, el); | ||
var textNode = new domhandler_1.Text("".concat(str)); | ||
(0, parse_js_1.update)(textNode, el); | ||
}); | ||
@@ -879,4 +887,5 @@ } | ||
function clone() { | ||
return this._make(utils_1.cloneDom(this.get())); | ||
return this._make((0, utils_js_1.cloneDom)(this.get())); | ||
} | ||
exports.clone = clone; | ||
//# sourceMappingURL=manipulation.js.map |
@@ -6,5 +6,5 @@ /** | ||
*/ | ||
import { Node, Element, Document } from 'domhandler'; | ||
import type { Cheerio } from '../cheerio'; | ||
import type { AcceptedFilters } from '../types'; | ||
import { AnyNode, Element, Document } from 'domhandler'; | ||
import type { Cheerio } from '../cheerio.js'; | ||
import type { AcceptedFilters } from '../types.js'; | ||
/** | ||
@@ -28,3 +28,3 @@ * Get the descendants of each element in the current set of matched elements, | ||
*/ | ||
export declare function find<T extends Node>(this: Cheerio<T>, selectorOrHaystack?: string | Cheerio<Element> | Element): Cheerio<Element>; | ||
export declare function find<T extends AnyNode>(this: Cheerio<T>, selectorOrHaystack?: string | Cheerio<Element> | Element): Cheerio<Element>; | ||
/** | ||
@@ -46,3 +46,3 @@ * Get the parent of each element in the current set of matched elements, | ||
*/ | ||
export declare const parent: <T extends Node>(this: Cheerio<T>, selector?: AcceptedFilters<Element> | undefined) => Cheerio<Element>; | ||
export declare const parent: <T extends AnyNode>(this: Cheerio<T>, selector?: AcceptedFilters<Element> | undefined) => Cheerio<Element>; | ||
/** | ||
@@ -66,3 +66,3 @@ * Get a set of parents filtered by `selector` of each element in the current | ||
*/ | ||
export declare const parents: <T extends Node>(this: Cheerio<T>, selector?: AcceptedFilters<Element> | undefined) => Cheerio<Element>; | ||
export declare const parents: <T extends AnyNode>(this: Cheerio<T>, selector?: AcceptedFilters<Element> | undefined) => Cheerio<Element>; | ||
/** | ||
@@ -85,3 +85,3 @@ * Get the ancestors of each element in the current set of matched elements, up | ||
*/ | ||
export declare const parentsUntil: <T extends Node>(this: Cheerio<T>, selector?: AcceptedFilters<Element> | null | undefined, filterSelector?: AcceptedFilters<Element> | undefined) => Cheerio<Element>; | ||
export declare const parentsUntil: <T extends AnyNode>(this: Cheerio<T>, selector?: AcceptedFilters<Element> | null | undefined, filterSelector?: AcceptedFilters<Element> | undefined) => Cheerio<Element>; | ||
/** | ||
@@ -112,3 +112,3 @@ * For each element in the set, get the first element that matches the selector | ||
*/ | ||
export declare function closest<T extends Node>(this: Cheerio<T>, selector?: AcceptedFilters<Node>): Cheerio<Node>; | ||
export declare function closest<T extends AnyNode>(this: Cheerio<T>, selector?: AcceptedFilters<Element>): Cheerio<AnyNode>; | ||
/** | ||
@@ -129,3 +129,3 @@ * Gets the next sibling of the first selected element, optionally filtered by a selector. | ||
*/ | ||
export declare const next: <T extends Node>(this: Cheerio<T>, selector?: AcceptedFilters<Element> | undefined) => Cheerio<Element>; | ||
export declare const next: <T extends AnyNode>(this: Cheerio<T>, selector?: AcceptedFilters<Element> | undefined) => Cheerio<Element>; | ||
/** | ||
@@ -149,3 +149,3 @@ * Gets all the following siblings of the first selected element, optionally | ||
*/ | ||
export declare const nextAll: <T extends Node>(this: Cheerio<T>, selector?: AcceptedFilters<Element> | undefined) => Cheerio<Element>; | ||
export declare const nextAll: <T extends AnyNode>(this: Cheerio<T>, selector?: AcceptedFilters<Element> | undefined) => Cheerio<Element>; | ||
/** | ||
@@ -168,3 +168,3 @@ * Gets all the following siblings up to but not including the element matched | ||
*/ | ||
export declare const nextUntil: <T extends Node>(this: Cheerio<T>, selector?: AcceptedFilters<Element> | null | undefined, filterSelector?: AcceptedFilters<Element> | undefined) => Cheerio<Element>; | ||
export declare const nextUntil: <T extends AnyNode>(this: Cheerio<T>, selector?: AcceptedFilters<Element> | null | undefined, filterSelector?: AcceptedFilters<Element> | undefined) => Cheerio<Element>; | ||
/** | ||
@@ -186,3 +186,3 @@ * Gets the previous sibling of the first selected element optionally filtered | ||
*/ | ||
export declare const prev: <T extends Node>(this: Cheerio<T>, selector?: AcceptedFilters<Element> | undefined) => Cheerio<Element>; | ||
export declare const prev: <T extends AnyNode>(this: Cheerio<T>, selector?: AcceptedFilters<Element> | undefined) => Cheerio<Element>; | ||
/** | ||
@@ -207,3 +207,3 @@ * Gets all the preceding siblings of the first selected element, optionally | ||
*/ | ||
export declare const prevAll: <T extends Node>(this: Cheerio<T>, selector?: AcceptedFilters<Element> | undefined) => Cheerio<Element>; | ||
export declare const prevAll: <T extends AnyNode>(this: Cheerio<T>, selector?: AcceptedFilters<Element> | undefined) => Cheerio<Element>; | ||
/** | ||
@@ -226,3 +226,3 @@ * Gets all the preceding siblings up to but not including the element matched | ||
*/ | ||
export declare const prevUntil: <T extends Node>(this: Cheerio<T>, selector?: AcceptedFilters<Element> | null | undefined, filterSelector?: AcceptedFilters<Element> | undefined) => Cheerio<Element>; | ||
export declare const prevUntil: <T extends AnyNode>(this: Cheerio<T>, selector?: AcceptedFilters<Element> | null | undefined, filterSelector?: AcceptedFilters<Element> | undefined) => Cheerio<Element>; | ||
/** | ||
@@ -247,5 +247,5 @@ * Get the siblings of each element (excluding the element) in the set of | ||
*/ | ||
export declare const siblings: <T extends Node>(this: Cheerio<T>, selector?: AcceptedFilters<Element> | undefined) => Cheerio<Element>; | ||
export declare const siblings: <T extends AnyNode>(this: Cheerio<T>, selector?: AcceptedFilters<Element> | undefined) => Cheerio<Element>; | ||
/** | ||
* Gets the children of the first selected element. | ||
* Gets the element children of each element in the set of matched elements. | ||
* | ||
@@ -267,3 +267,3 @@ * @category Traversing | ||
*/ | ||
export declare const children: <T extends Node>(this: Cheerio<T>, selector?: AcceptedFilters<Element> | undefined) => Cheerio<Element>; | ||
export declare const children: <T extends AnyNode>(this: Cheerio<T>, selector?: AcceptedFilters<Element> | undefined) => Cheerio<Element>; | ||
/** | ||
@@ -284,3 +284,3 @@ * Gets the children of each element in the set of matched elements, including | ||
*/ | ||
export declare function contents<T extends Node>(this: Cheerio<T>): Cheerio<Node>; | ||
export declare function contents<T extends AnyNode>(this: Cheerio<T>): Cheerio<AnyNode>; | ||
/** | ||
@@ -402,5 +402,5 @@ * Iterates over a cheerio object, executing a function for each matched | ||
/** | ||
* Checks the current list of elements and returns `true` if *any* of the | ||
* Checks the current list of elements and returns `true` if _any_ of the | ||
* elements match the selector. If using an element or Cheerio selection, | ||
* returns `true` if *any* of the elements match. If using a predicate function, | ||
* returns `true` if _any_ of the elements match. If using a predicate function, | ||
* the function is executed in the context of the selected element, so `this` | ||
@@ -448,3 +448,3 @@ * refers to the current element. | ||
*/ | ||
export declare function not<T extends Node>(this: Cheerio<T>, match: AcceptedFilters<T>): Cheerio<T>; | ||
export declare function not<T extends AnyNode>(this: Cheerio<T>, match: AcceptedFilters<T>): Cheerio<T>; | ||
/** | ||
@@ -474,3 +474,3 @@ * Filters the set of matched elements to only those which have the given DOM | ||
*/ | ||
export declare function has(this: Cheerio<Node | Element>, selectorOrHaystack: string | Cheerio<Element> | Element): Cheerio<Node | Element>; | ||
export declare function has(this: Cheerio<AnyNode | Element>, selectorOrHaystack: string | Cheerio<Element> | Element): Cheerio<AnyNode | Element>; | ||
/** | ||
@@ -490,3 +490,3 @@ * Will select the first element of a cheerio object. | ||
*/ | ||
export declare function first<T extends Node>(this: Cheerio<T>): Cheerio<T>; | ||
export declare function first<T extends AnyNode>(this: Cheerio<T>): Cheerio<T>; | ||
/** | ||
@@ -542,3 +542,3 @@ * Will select the last element of a cheerio object. | ||
*/ | ||
export declare function get<T>(this: Cheerio<T>, i: number): T; | ||
export declare function get<T>(this: Cheerio<T>, i: number): T | undefined; | ||
/** | ||
@@ -590,3 +590,3 @@ * Retrieve all elements matched by the Cheerio object, as an array. | ||
*/ | ||
export declare function index<T extends Node>(this: Cheerio<T>, selectorOrNeedle?: string | Cheerio<Node> | Node): number; | ||
export declare function index<T extends AnyNode>(this: Cheerio<T>, selectorOrNeedle?: string | Cheerio<AnyNode> | AnyNode): number; | ||
/** | ||
@@ -630,3 +630,3 @@ * Gets the elements matching the specified range (0-based position). | ||
*/ | ||
export declare function end<T>(this: Cheerio<T>): Cheerio<Node>; | ||
export declare function end<T>(this: Cheerio<T>): Cheerio<AnyNode>; | ||
/** | ||
@@ -648,3 +648,3 @@ * Add elements to the set of matched elements. | ||
*/ | ||
export declare function add<S extends Node, T extends Node>(this: Cheerio<T>, other: string | Cheerio<S> | S | S[], context?: Cheerio<S> | string): Cheerio<S | T>; | ||
export declare function add<S extends AnyNode, T extends AnyNode>(this: Cheerio<T>, other: string | Cheerio<S> | S | S[], context?: Cheerio<S> | string): Cheerio<S | T>; | ||
/** | ||
@@ -666,3 +666,3 @@ * Add the previous set of elements on the stack to the current set, optionally | ||
*/ | ||
export declare function addBack<T extends Node>(this: Cheerio<T>, selector?: string): Cheerio<Node>; | ||
export declare function addBack<T extends AnyNode>(this: Cheerio<T>, selector?: string): Cheerio<AnyNode>; | ||
//# sourceMappingURL=traversing.d.ts.map |
@@ -7,11 +7,41 @@ "use strict"; | ||
*/ | ||
var __createBinding = (this && this.__createBinding) || (Object.create ? (function(o, m, k, k2) { | ||
if (k2 === undefined) k2 = k; | ||
var desc = Object.getOwnPropertyDescriptor(m, k); | ||
if (!desc || ("get" in desc ? !m.__esModule : desc.writable || desc.configurable)) { | ||
desc = { enumerable: true, get: function() { return m[k]; } }; | ||
} | ||
Object.defineProperty(o, k2, desc); | ||
}) : (function(o, m, k, k2) { | ||
if (k2 === undefined) k2 = k; | ||
o[k2] = m[k]; | ||
})); | ||
var __setModuleDefault = (this && this.__setModuleDefault) || (Object.create ? (function(o, v) { | ||
Object.defineProperty(o, "default", { enumerable: true, value: v }); | ||
}) : function(o, v) { | ||
o["default"] = v; | ||
}); | ||
var __importStar = (this && this.__importStar) || function (mod) { | ||
if (mod && mod.__esModule) return mod; | ||
var result = {}; | ||
if (mod != null) for (var k in mod) if (k !== "default" && Object.prototype.hasOwnProperty.call(mod, k)) __createBinding(result, mod, k); | ||
__setModuleDefault(result, mod); | ||
return result; | ||
}; | ||
var __spreadArray = (this && this.__spreadArray) || function (to, from, pack) { | ||
if (pack || arguments.length === 2) for (var i = 0, l = from.length, ar; i < l; i++) { | ||
if (ar || !(i in from)) { | ||
if (!ar) ar = Array.prototype.slice.call(from, 0, i); | ||
ar[i] = from[i]; | ||
} | ||
} | ||
return to.concat(ar || Array.prototype.slice.call(from)); | ||
}; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
exports.addBack = exports.add = exports.end = exports.slice = exports.index = exports.toArray = exports.get = exports.eq = exports.last = exports.first = exports.has = exports.not = exports.is = exports.filterArray = exports.filter = exports.map = exports.each = exports.contents = exports.children = exports.siblings = exports.prevUntil = exports.prevAll = exports.prev = exports.nextUntil = exports.nextAll = exports.next = exports.closest = exports.parentsUntil = exports.parents = exports.parent = exports.find = void 0; | ||
var tslib_1 = require("tslib"); | ||
var domhandler_1 = require("domhandler"); | ||
var select = tslib_1.__importStar(require("cheerio-select")); | ||
var utils_1 = require("../utils"); | ||
var static_1 = require("../static"); | ||
var htmlparser2_1 = require("htmlparser2"); | ||
var uniqueSort = htmlparser2_1.DomUtils.uniqueSort; | ||
var select = __importStar(require("cheerio-select")); | ||
var utils_js_1 = require("../utils.js"); | ||
var static_js_1 = require("../static.js"); | ||
var domutils_1 = require("domutils"); | ||
var reSiblingSelector = /^\s*[~+]/; | ||
@@ -43,6 +73,6 @@ /** | ||
if (typeof selectorOrHaystack !== 'string') { | ||
var haystack = utils_1.isCheerio(selectorOrHaystack) | ||
var haystack = (0, utils_js_1.isCheerio)(selectorOrHaystack) | ||
? selectorOrHaystack.toArray() | ||
: [selectorOrHaystack]; | ||
return this._make(haystack.filter(function (elem) { return context.some(function (node) { return static_1.contains(node, elem); }); })); | ||
return this._make(haystack.filter(function (elem) { return context.some(function (node) { return (0, static_js_1.contains)(node, elem); }); })); | ||
} | ||
@@ -55,3 +85,8 @@ var elems = reSiblingSelector.test(selectorOrHaystack) | ||
root: (_a = this._root) === null || _a === void 0 ? void 0 : _a[0], | ||
// Pass options that are recognized by `cheerio-select` | ||
xmlMode: this.options.xmlMode, | ||
lowerCaseTags: this.options.lowerCaseTags, | ||
lowerCaseAttributeNames: this.options.lowerCaseAttributeNames, | ||
pseudos: this.options.pseudos, | ||
quirksMode: this.options.quirksMode, | ||
}; | ||
@@ -124,3 +159,3 @@ return this._make(select.select(selectorOrHaystack, elems, options)); | ||
var matched = []; | ||
utils_1.domEach(elems, function (elem) { | ||
(0, utils_js_1.domEach)(elems, function (elem) { | ||
for (var next_1; (next_1 = nextElem(elem)); elem = next_1) { | ||
@@ -134,3 +169,3 @@ // FIXME: `matched` might contain duplicates here and the index is too large. | ||
return matched; | ||
}).apply(void 0, tslib_1.__spreadArray([nextElem], postFns)); | ||
}).apply(void 0, __spreadArray([nextElem], postFns, false)); | ||
return function (selector, filterSelector) { | ||
@@ -172,3 +207,3 @@ var _this = this; | ||
var parent = _a.parent; | ||
return (parent && !domhandler_1.isDocument(parent) ? parent : null); | ||
return (parent && !(0, domhandler_1.isDocument)(parent) ? parent : null); | ||
}, _removeDuplicates); | ||
@@ -195,3 +230,3 @@ /** | ||
var matched = []; | ||
while (elem.parent && !domhandler_1.isDocument(elem.parent)) { | ||
while (elem.parent && !(0, domhandler_1.isDocument)(elem.parent)) { | ||
matched.push(elem.parent); | ||
@@ -201,3 +236,3 @@ elem = elem.parent; | ||
return matched; | ||
}, uniqueSort, function (elems) { return elems.reverse(); }); | ||
}, domutils_1.uniqueSort, function (elems) { return elems.reverse(); }); | ||
/** | ||
@@ -222,4 +257,4 @@ * Get the ancestors of each element in the current set of matched elements, up | ||
var parent = _a.parent; | ||
return (parent && !domhandler_1.isDocument(parent) ? parent : null); | ||
}, uniqueSort, function (elems) { return elems.reverse(); }); | ||
return (parent && !(0, domhandler_1.isDocument)(parent) ? parent : null); | ||
}, domutils_1.uniqueSort, function (elems) { return elems.reverse(); }); | ||
/** | ||
@@ -251,3 +286,3 @@ * For each element in the set, get the first element that matches the selector | ||
function closest(selector) { | ||
var _this = this; | ||
var _a; | ||
var set = []; | ||
@@ -257,10 +292,14 @@ if (!selector) { | ||
} | ||
utils_1.domEach(this, function (elem) { | ||
var _a; | ||
while (elem && elem.type !== 'root') { | ||
if (!selector || | ||
filterArray([elem], selector, _this.options.xmlMode, (_a = _this._root) === null || _a === void 0 ? void 0 : _a[0]) | ||
.length) { | ||
var selectOpts = { | ||
xmlMode: this.options.xmlMode, | ||
root: (_a = this._root) === null || _a === void 0 ? void 0 : _a[0], | ||
}; | ||
var selectFn = typeof selector === 'string' | ||
? function (elem) { return select.is(elem, selector, selectOpts); } | ||
: getFilterFn(selector); | ||
(0, utils_js_1.domEach)(this, function (elem) { | ||
while (elem && (0, utils_js_1.isTag)(elem)) { | ||
if (selectFn(elem, 0)) { | ||
// Do not add duplicate elements to the set | ||
if (elem && !set.includes(elem)) { | ||
if (!set.includes(elem)) { | ||
set.push(elem); | ||
@@ -291,3 +330,3 @@ } | ||
*/ | ||
exports.next = _singleMatcher(function (elem) { return htmlparser2_1.DomUtils.nextElementSibling(elem); }); | ||
exports.next = _singleMatcher(function (elem) { return (0, domutils_1.nextElementSibling)(elem); }); | ||
/** | ||
@@ -315,3 +354,3 @@ * Gets all the following siblings of the first selected element, optionally | ||
elem = elem.next; | ||
if (utils_1.isTag(elem)) | ||
if ((0, utils_js_1.isTag)(elem)) | ||
matched.push(elem); | ||
@@ -338,3 +377,3 @@ } | ||
*/ | ||
exports.nextUntil = _matchUntil(function (el) { return htmlparser2_1.DomUtils.nextElementSibling(el); }, _removeDuplicates); | ||
exports.nextUntil = _matchUntil(function (el) { return (0, domutils_1.nextElementSibling)(el); }, _removeDuplicates); | ||
/** | ||
@@ -356,3 +395,3 @@ * Gets the previous sibling of the first selected element optionally filtered | ||
*/ | ||
exports.prev = _singleMatcher(function (elem) { return htmlparser2_1.DomUtils.prevElementSibling(elem); }); | ||
exports.prev = _singleMatcher(function (elem) { return (0, domutils_1.prevElementSibling)(elem); }); | ||
/** | ||
@@ -381,3 +420,3 @@ * Gets all the preceding siblings of the first selected element, optionally | ||
elem = elem.prev; | ||
if (utils_1.isTag(elem)) | ||
if ((0, utils_js_1.isTag)(elem)) | ||
matched.push(elem); | ||
@@ -404,3 +443,3 @@ } | ||
*/ | ||
exports.prevUntil = _matchUntil(function (el) { return htmlparser2_1.DomUtils.prevElementSibling(el); }, _removeDuplicates); | ||
exports.prevUntil = _matchUntil(function (el) { return (0, domutils_1.prevElementSibling)(el); }, _removeDuplicates); | ||
/** | ||
@@ -426,6 +465,6 @@ * Get the siblings of each element (excluding the element) in the set of | ||
exports.siblings = _matcher(function (elem) { | ||
return htmlparser2_1.DomUtils.getSiblings(elem).filter(function (el) { return utils_1.isTag(el) && el !== elem; }); | ||
}, uniqueSort); | ||
return (0, domutils_1.getSiblings)(elem).filter(function (el) { return (0, utils_js_1.isTag)(el) && el !== elem; }); | ||
}, domutils_1.uniqueSort); | ||
/** | ||
* Gets the children of the first selected element. | ||
* Gets the element children of each element in the set of matched elements. | ||
* | ||
@@ -447,3 +486,3 @@ * @category Traversing | ||
*/ | ||
exports.children = _matcher(function (elem) { return htmlparser2_1.DomUtils.getChildren(elem).filter(utils_1.isTag); }, _removeDuplicates); | ||
exports.children = _matcher(function (elem) { return (0, domutils_1.getChildren)(elem).filter(utils_js_1.isTag); }, _removeDuplicates); | ||
/** | ||
@@ -466,3 +505,3 @@ * Gets the children of each element in the set of matched elements, including | ||
var elems = this.toArray().reduce(function (newElems, elem) { | ||
return domhandler_1.hasChildren(elem) ? newElems.concat(elem.children) : newElems; | ||
return (0, domhandler_1.hasChildren)(elem) ? newElems.concat(elem.children) : newElems; | ||
}, []); | ||
@@ -553,3 +592,3 @@ return this._make(elems); | ||
} | ||
if (utils_1.isCheerio(match)) { | ||
if ((0, utils_js_1.isCheerio)(match)) { | ||
return function (el) { return Array.prototype.includes.call(match, el); }; | ||
@@ -573,5 +612,5 @@ } | ||
/** | ||
* Checks the current list of elements and returns `true` if *any* of the | ||
* Checks the current list of elements and returns `true` if _any_ of the | ||
* elements match the selector. If using an element or Cheerio selection, | ||
* returns `true` if *any* of the elements match. If using a predicate function, | ||
* returns `true` if _any_ of the elements match. If using a predicate function, | ||
* the function is executed in the context of the selected element, so `this` | ||
@@ -588,3 +627,3 @@ * refers to the current element. | ||
return typeof selector === 'string' | ||
? select.some(nodes.filter(utils_1.isTag), selector, this.options) | ||
? select.some(nodes.filter(utils_js_1.isTag), selector, this.options) | ||
: selector | ||
@@ -669,3 +708,3 @@ ? nodes.some(getFilterFn(selector)) | ||
? // Using the `:has` selector here short-circuits searches. | ||
":has(" + selectorOrHaystack + ")" | ||
":has(".concat(selectorOrHaystack, ")") | ||
: function (_, el) { return _this._make(el).find(selectorOrHaystack).length > 0; }); | ||
@@ -793,4 +832,5 @@ } | ||
else { | ||
// eslint-disable-next-line @typescript-eslint/no-this-alias | ||
$haystack = this; | ||
needle = utils_1.isCheerio(selectorOrNeedle) | ||
needle = (0, utils_js_1.isCheerio)(selectorOrNeedle) | ||
? selectorOrNeedle[0] | ||
@@ -866,3 +906,3 @@ : selectorOrNeedle; | ||
var selection = this._make(other, context); | ||
var contents = uniqueSort(tslib_1.__spreadArray(tslib_1.__spreadArray([], this.get()), selection.get())); | ||
var contents = (0, domutils_1.uniqueSort)(__spreadArray(__spreadArray([], this.get(), true), selection.get(), true)); | ||
return this._make(contents); | ||
@@ -893,1 +933,2 @@ } | ||
exports.addBack = addBack; | ||
//# sourceMappingURL=traversing.js.map |
@@ -1,9 +0,10 @@ | ||
import { InternalOptions } from './options'; | ||
import type { Node, Document } from 'domhandler'; | ||
import { BasicAcceptedElems } from './types'; | ||
import * as Attributes from './api/attributes'; | ||
import * as Traversing from './api/traversing'; | ||
import * as Manipulation from './api/manipulation'; | ||
import * as Css from './api/css'; | ||
import * as Forms from './api/forms'; | ||
/// <reference types="node" /> | ||
import type { InternalOptions } from './options.js'; | ||
import type { AnyNode, Document, ParentNode } from 'domhandler'; | ||
import type { BasicAcceptedElems } from './types.js'; | ||
import * as Attributes from './api/attributes.js'; | ||
import * as Traversing from './api/traversing.js'; | ||
import * as Manipulation from './api/manipulation.js'; | ||
import * as Css from './api/css.js'; | ||
import * as Forms from './api/forms.js'; | ||
declare type AttributesType = typeof Attributes; | ||
@@ -14,3 +15,3 @@ declare type TraversingType = typeof Traversing; | ||
declare type FormsType = typeof Forms; | ||
export declare class Cheerio<T> implements ArrayLike<T> { | ||
export declare abstract class Cheerio<T> implements ArrayLike<T> { | ||
length: number; | ||
@@ -24,17 +25,14 @@ [index: number]: T; | ||
*/ | ||
_root: Cheerio<Document> | undefined; | ||
/** @function */ | ||
find: typeof Traversing.find; | ||
_root: Cheerio<Document> | null; | ||
/** | ||
* Instance of cheerio. Methods are specified in the modules. Usage of this | ||
* constructor is not recommended. Please use $.load instead. | ||
* constructor is not recommended. Please use `$.load` instead. | ||
* | ||
* @private | ||
* @param selector - The new selection. | ||
* @param context - Context of the selection. | ||
* @param elements - The new selection. | ||
* @param root - Sets the root node. | ||
* @param options - Options for the instance. | ||
*/ | ||
constructor(selector?: T extends Node ? BasicAcceptedElems<T> : Cheerio<T> | T[], context?: BasicAcceptedElems<Node> | null, root?: BasicAcceptedElems<Document> | null, options?: InternalOptions); | ||
prevObject: Cheerio<Node> | undefined; | ||
constructor(elements: ArrayLike<T> | undefined, root: Cheerio<Document> | null, options: InternalOptions); | ||
prevObject: Cheerio<any> | undefined; | ||
/** | ||
@@ -48,3 +46,21 @@ * Make a cheerio object. | ||
*/ | ||
_make<T>(dom: Cheerio<T> | T[] | T | string, context?: BasicAcceptedElems<Node>): Cheerio<T>; | ||
abstract _make<T>(dom: ArrayLike<T> | T | string, context?: BasicAcceptedElems<AnyNode>): Cheerio<T>; | ||
/** | ||
* Parses some content. | ||
* | ||
* @private | ||
* @param content - Content to parse. | ||
* @param options - Options for parsing. | ||
* @param isDocument - Allows parser to be switched to fragment mode. | ||
* @returns A document containing the `content`. | ||
*/ | ||
abstract _parse(content: string | Document | AnyNode | AnyNode[] | Buffer, options: InternalOptions, isDocument: boolean, context: ParentNode | null): Document; | ||
/** | ||
* Render an element or a set of elements. | ||
* | ||
* @private | ||
* @param dom - DOM to render. | ||
* @returns The rendered DOM. | ||
*/ | ||
abstract _render(dom: AnyNode | ArrayLike<AnyNode>): string; | ||
} | ||
@@ -51,0 +67,0 @@ export interface Cheerio<T> extends AttributesType, TraversingType, ManipulationType, CssType, FormsType, Iterable<T> { |
"use strict"; | ||
var __createBinding = (this && this.__createBinding) || (Object.create ? (function(o, m, k, k2) { | ||
if (k2 === undefined) k2 = k; | ||
var desc = Object.getOwnPropertyDescriptor(m, k); | ||
if (!desc || ("get" in desc ? !m.__esModule : desc.writable || desc.configurable)) { | ||
desc = { enumerable: true, get: function() { return m[k]; } }; | ||
} | ||
Object.defineProperty(o, k2, desc); | ||
}) : (function(o, m, k, k2) { | ||
if (k2 === undefined) k2 = k; | ||
o[k2] = m[k]; | ||
})); | ||
var __setModuleDefault = (this && this.__setModuleDefault) || (Object.create ? (function(o, v) { | ||
Object.defineProperty(o, "default", { enumerable: true, value: v }); | ||
}) : function(o, v) { | ||
o["default"] = v; | ||
}); | ||
var __importStar = (this && this.__importStar) || function (mod) { | ||
if (mod && mod.__esModule) return mod; | ||
var result = {}; | ||
if (mod != null) for (var k in mod) if (k !== "default" && Object.prototype.hasOwnProperty.call(mod, k)) __createBinding(result, mod, k); | ||
__setModuleDefault(result, mod); | ||
return result; | ||
}; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
exports.Cheerio = void 0; | ||
var tslib_1 = require("tslib"); | ||
var parse_1 = tslib_1.__importDefault(require("./parse")); | ||
var options_1 = tslib_1.__importDefault(require("./options")); | ||
var utils_1 = require("./utils"); | ||
var Attributes = tslib_1.__importStar(require("./api/attributes")); | ||
var Traversing = tslib_1.__importStar(require("./api/traversing")); | ||
var Manipulation = tslib_1.__importStar(require("./api/manipulation")); | ||
var Css = tslib_1.__importStar(require("./api/css")); | ||
var Forms = tslib_1.__importStar(require("./api/forms")); | ||
var Attributes = __importStar(require("./api/attributes.js")); | ||
var Traversing = __importStar(require("./api/traversing.js")); | ||
var Manipulation = __importStar(require("./api/manipulation.js")); | ||
var Css = __importStar(require("./api/css.js")); | ||
var Forms = __importStar(require("./api/forms.js")); | ||
var Cheerio = /** @class */ (function () { | ||
/** | ||
* Instance of cheerio. Methods are specified in the modules. Usage of this | ||
* constructor is not recommended. Please use $.load instead. | ||
* constructor is not recommended. Please use `$.load` instead. | ||
* | ||
* @private | ||
* @param selector - The new selection. | ||
* @param context - Context of the selection. | ||
* @param elements - The new selection. | ||
* @param root - Sets the root node. | ||
* @param options - Options for the instance. | ||
*/ | ||
function Cheerio(selector, context, root, options) { | ||
var _this = this; | ||
if (options === void 0) { options = options_1.default; } | ||
function Cheerio(elements, root, options) { | ||
this.length = 0; | ||
this.options = options; | ||
// $(), $(null), $(undefined), $(false) | ||
if (!selector) | ||
return this; | ||
if (root) { | ||
if (typeof root === 'string') | ||
root = parse_1.default(root, this.options, false); | ||
this._root = new this.constructor(root, null, null, this.options); | ||
// Add a cyclic reference, so that calling methods on `_root` never fails. | ||
this._root._root = this._root; | ||
} | ||
// $($) | ||
if (utils_1.isCheerio(selector)) | ||
return selector; | ||
var elements = typeof selector === 'string' && utils_1.isHtml(selector) | ||
? // $(<html>) | ||
parse_1.default(selector, this.options, false).children | ||
: isNode(selector) | ||
? // $(dom) | ||
[selector] | ||
: Array.isArray(selector) | ||
? // $([dom]) | ||
selector | ||
: null; | ||
this._root = root; | ||
if (elements) { | ||
elements.forEach(function (elem, idx) { | ||
_this[idx] = elem; | ||
}); | ||
for (var idx = 0; idx < elements.length; idx++) { | ||
this[idx] = elements[idx]; | ||
} | ||
this.length = elements.length; | ||
return this; | ||
} | ||
// We know that our selector is a string now. | ||
var search = selector; | ||
var searchContext = !context | ||
? // If we don't have a context, maybe we have a root, from loading | ||
this._root | ||
: typeof context === 'string' | ||
? utils_1.isHtml(context) | ||
? // $('li', '<ul>...</ul>') | ||
this._make(parse_1.default(context, this.options, false)) | ||
: // $('li', 'ul') | ||
((search = context + " " + search), this._root) | ||
: utils_1.isCheerio(context) | ||
? // $('li', $) | ||
context | ||
: // $('li', node), $('li', [nodes]) | ||
this._make(context); | ||
// If we still don't have a context, return | ||
if (!searchContext) | ||
return this; | ||
/* | ||
* #id, .class, tag | ||
*/ | ||
// @ts-expect-error No good way to type this — we will always return `Cheerio<Element>` here. | ||
return searchContext.find(search); | ||
} | ||
/** | ||
* Make a cheerio object. | ||
* | ||
* @private | ||
* @param dom - The contents of the new object. | ||
* @param context - The context of the new object. | ||
* @returns The new cheerio object. | ||
*/ | ||
Cheerio.prototype._make = function (dom, context) { | ||
var cheerio = new this.constructor(dom, context, this._root, this.options); | ||
cheerio.prevObject = this; | ||
return cheerio; | ||
}; | ||
return Cheerio; | ||
@@ -110,7 +66,2 @@ }()); | ||
Object.assign(Cheerio.prototype, Attributes, Traversing, Manipulation, Css, Forms); | ||
function isNode(obj) { | ||
return (!!obj.name || | ||
obj.type === 'root' || | ||
obj.type === 'text' || | ||
obj.type === 'comment'); | ||
} | ||
//# sourceMappingURL=cheerio.js.map |
@@ -0,1 +1,2 @@ | ||
/// <reference types="node" /> | ||
/** | ||
@@ -6,3 +7,3 @@ * The main types of Cheerio objects. | ||
*/ | ||
export type { Cheerio } from './cheerio'; | ||
export type { Cheerio } from './cheerio.js'; | ||
/** | ||
@@ -13,4 +14,4 @@ * Types used in signatures of Cheerio methods. | ||
*/ | ||
export * from './types'; | ||
export type { CheerioOptions, HTMLParser2Options, Parse5Options, } from './options'; | ||
export * from './types.js'; | ||
export type { CheerioOptions, HTMLParser2Options, Parse5Options, } from './options.js'; | ||
/** | ||
@@ -21,6 +22,20 @@ * Re-exporting all of the node types. | ||
*/ | ||
export type { Node, NodeWithChildren, Element, Document } from 'domhandler'; | ||
export * from './load'; | ||
declare const _default: import("./load").CheerioAPI; | ||
export type { Node, AnyNode, ParentNode, Element, Document } from 'domhandler'; | ||
export type { CheerioAPI } from './load.js'; | ||
/** | ||
* Create a querying function, bound to a document created from the provided markup. | ||
* | ||
* Note that similar to web browser contexts, this operation may introduce | ||
* `<html>`, `<head>`, and `<body>` elements; set `isDocument` to `false` to | ||
* switch to fragment mode and disable this. | ||
* | ||
* @param content - Markup to be loaded. | ||
* @param options - Options for the created instance. | ||
* @param isDocument - Allows parser to be switched to fragment mode. | ||
* @returns The loaded document. | ||
* @see {@link https://cheerio.js.org#loading} for additional usage information. | ||
*/ | ||
export declare const load: (content: string | import("domhandler").AnyNode | import("domhandler").AnyNode[] | Buffer, options?: import("./options.js").CheerioOptions | null | undefined, isDocument?: boolean) => import("./load.js").CheerioAPI; | ||
declare const _default: import("./load.js").CheerioAPI; | ||
/** | ||
* The default cheerio instance. | ||
@@ -31,3 +46,4 @@ * | ||
export default _default; | ||
import * as staticMethods from './static'; | ||
export { html, xml, text } from './static.js'; | ||
import * as staticMethods from './static.js'; | ||
/** | ||
@@ -34,0 +50,0 @@ * In order to promote consistency with the jQuery library, users are encouraged |
"use strict"; | ||
var __createBinding = (this && this.__createBinding) || (Object.create ? (function(o, m, k, k2) { | ||
if (k2 === undefined) k2 = k; | ||
var desc = Object.getOwnPropertyDescriptor(m, k); | ||
if (!desc || ("get" in desc ? !m.__esModule : desc.writable || desc.configurable)) { | ||
desc = { enumerable: true, get: function() { return m[k]; } }; | ||
} | ||
Object.defineProperty(o, k2, desc); | ||
}) : (function(o, m, k, k2) { | ||
if (k2 === undefined) k2 = k; | ||
o[k2] = m[k]; | ||
})); | ||
var __setModuleDefault = (this && this.__setModuleDefault) || (Object.create ? (function(o, v) { | ||
Object.defineProperty(o, "default", { enumerable: true, value: v }); | ||
}) : function(o, v) { | ||
o["default"] = v; | ||
}); | ||
var __exportStar = (this && this.__exportStar) || function(m, exports) { | ||
for (var p in m) if (p !== "default" && !Object.prototype.hasOwnProperty.call(exports, p)) __createBinding(exports, m, p); | ||
}; | ||
var __importStar = (this && this.__importStar) || function (mod) { | ||
if (mod && mod.__esModule) return mod; | ||
var result = {}; | ||
if (mod != null) for (var k in mod) if (k !== "default" && Object.prototype.hasOwnProperty.call(mod, k)) __createBinding(result, mod, k); | ||
__setModuleDefault(result, mod); | ||
return result; | ||
}; | ||
var __importDefault = (this && this.__importDefault) || function (mod) { | ||
return (mod && mod.__esModule) ? mod : { "default": mod }; | ||
}; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
exports.root = exports.parseHTML = exports.merge = exports.contains = void 0; | ||
var tslib_1 = require("tslib"); | ||
exports.root = exports.parseHTML = exports.merge = exports.contains = exports.text = exports.xml = exports.html = exports.load = void 0; | ||
/** | ||
@@ -10,6 +38,33 @@ * Types used in signatures of Cheerio methods. | ||
*/ | ||
tslib_1.__exportStar(require("./types"), exports); | ||
tslib_1.__exportStar(require("./load"), exports); | ||
var load_1 = require("./load"); | ||
__exportStar(require("./types.js"), exports); | ||
var load_js_1 = require("./load.js"); | ||
var parse_js_1 = require("./parse.js"); | ||
var parse5_adapter_js_1 = require("./parsers/parse5-adapter.js"); | ||
var dom_serializer_1 = __importDefault(require("dom-serializer")); | ||
var htmlparser2_1 = require("htmlparser2"); | ||
var parse = (0, parse_js_1.getParse)(function (content, options, isDocument, context) { | ||
return options.xmlMode || options._useHtmlParser2 | ||
? (0, htmlparser2_1.parseDocument)(content, options) | ||
: (0, parse5_adapter_js_1.parseWithParse5)(content, options, isDocument, context); | ||
}); | ||
// Duplicate docs due to https://github.com/TypeStrong/typedoc/issues/1616 | ||
/** | ||
* Create a querying function, bound to a document created from the provided markup. | ||
* | ||
* Note that similar to web browser contexts, this operation may introduce | ||
* `<html>`, `<head>`, and `<body>` elements; set `isDocument` to `false` to | ||
* switch to fragment mode and disable this. | ||
* | ||
* @param content - Markup to be loaded. | ||
* @param options - Options for the created instance. | ||
* @param isDocument - Allows parser to be switched to fragment mode. | ||
* @returns The loaded document. | ||
* @see {@link https://cheerio.js.org#loading} for additional usage information. | ||
*/ | ||
exports.load = (0, load_js_1.getLoad)(parse, function (dom, options) { | ||
return options.xmlMode || options._useHtmlParser2 | ||
? (0, dom_serializer_1.default)(dom, options) | ||
: (0, parse5_adapter_js_1.renderWithParse5)(dom); | ||
}); | ||
/** | ||
* The default cheerio instance. | ||
@@ -19,4 +74,8 @@ * | ||
*/ | ||
exports.default = load_1.load([]); | ||
var staticMethods = tslib_1.__importStar(require("./static")); | ||
exports.default = (0, exports.load)([]); | ||
var static_js_1 = require("./static.js"); | ||
Object.defineProperty(exports, "html", { enumerable: true, get: function () { return static_js_1.html; } }); | ||
Object.defineProperty(exports, "xml", { enumerable: true, get: function () { return static_js_1.xml; } }); | ||
Object.defineProperty(exports, "text", { enumerable: true, get: function () { return static_js_1.text; } }); | ||
var staticMethods = __importStar(require("./static.js")); | ||
/** | ||
@@ -84,1 +143,2 @@ * In order to promote consistency with the jQuery library, users are encouraged | ||
exports.root = staticMethods.root; | ||
//# sourceMappingURL=index.js.map |
/// <reference types="node" /> | ||
import { CheerioOptions, InternalOptions } from './options'; | ||
import * as staticMethods from './static'; | ||
import { Cheerio } from './cheerio'; | ||
import type { Node, Document, Element } from 'domhandler'; | ||
import type * as Load from './load'; | ||
import { SelectorType, BasicAcceptedElems } from './types'; | ||
import { CheerioOptions, InternalOptions } from './options.js'; | ||
import * as staticMethods from './static.js'; | ||
import { Cheerio } from './cheerio.js'; | ||
import type { AnyNode, Document, Element } from 'domhandler'; | ||
import type { SelectorType, BasicAcceptedElems } from './types.js'; | ||
declare type StaticType = typeof staticMethods; | ||
declare type LoadType = typeof Load; | ||
/** | ||
@@ -15,3 +13,3 @@ * A querying function, bound to a document created from the provided markup. | ||
*/ | ||
export interface CheerioAPI extends StaticType, LoadType { | ||
export interface CheerioAPI extends StaticType { | ||
/** | ||
@@ -44,3 +42,3 @@ * This selector method is the starting point for traversing and manipulating | ||
*/ | ||
<T extends Node, S extends string>(selector?: S | BasicAcceptedElems<T>, context?: BasicAcceptedElems<Node> | null, root?: BasicAcceptedElems<Document>, options?: CheerioOptions): Cheerio<S extends SelectorType ? Element : T>; | ||
<T extends AnyNode, S extends string>(selector?: S | BasicAcceptedElems<T>, context?: BasicAcceptedElems<AnyNode> | null, root?: BasicAcceptedElems<Document>, options?: CheerioOptions): Cheerio<S extends SelectorType ? Element : T>; | ||
/** | ||
@@ -60,17 +58,6 @@ * The root the document was originally loaded with. | ||
fn: typeof Cheerio.prototype; | ||
load: ReturnType<typeof getLoad>; | ||
} | ||
/** | ||
* Create a querying function, bound to a document created from the provided | ||
* markup. Note that similar to web browser contexts, this operation may | ||
* introduce `<html>`, `<head>`, and `<body>` elements; set `isDocument` to | ||
* `false` to switch to fragment mode and disable this. | ||
* | ||
* @param content - Markup to be loaded. | ||
* @param options - Options for the created instance. | ||
* @param isDocument - Allows parser to be switched to fragment mode. | ||
* @returns The loaded document. | ||
* @see {@link https://cheerio.js.org#loading} for additional usage information. | ||
*/ | ||
export declare function load(content: string | Node | Node[] | Buffer, options?: CheerioOptions | null, isDocument?: boolean): CheerioAPI; | ||
export declare function getLoad(parse: typeof Cheerio.prototype._parse, render: (dom: AnyNode | ArrayLike<AnyNode>, options: InternalOptions) => string): (content: string | AnyNode | AnyNode[] | Buffer, options?: CheerioOptions | null | undefined, isDocument?: boolean) => CheerioAPI; | ||
export {}; | ||
//# sourceMappingURL=load.d.ts.map |
224
lib/load.js
"use strict"; | ||
var __extends = (this && this.__extends) || (function () { | ||
var extendStatics = function (d, b) { | ||
extendStatics = Object.setPrototypeOf || | ||
({ __proto__: [] } instanceof Array && function (d, b) { d.__proto__ = b; }) || | ||
function (d, b) { for (var p in b) if (Object.prototype.hasOwnProperty.call(b, p)) d[p] = b[p]; }; | ||
return extendStatics(d, b); | ||
}; | ||
return function (d, b) { | ||
if (typeof b !== "function" && b !== null) | ||
throw new TypeError("Class extends value " + String(b) + " is not a constructor or null"); | ||
extendStatics(d, b); | ||
function __() { this.constructor = d; } | ||
d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __()); | ||
}; | ||
})(); | ||
var __assign = (this && this.__assign) || function () { | ||
__assign = Object.assign || function(t) { | ||
for (var s, i = 1, n = arguments.length; i < n; i++) { | ||
s = arguments[i]; | ||
for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p)) | ||
t[p] = s[p]; | ||
} | ||
return t; | ||
}; | ||
return __assign.apply(this, arguments); | ||
}; | ||
var __createBinding = (this && this.__createBinding) || (Object.create ? (function(o, m, k, k2) { | ||
if (k2 === undefined) k2 = k; | ||
var desc = Object.getOwnPropertyDescriptor(m, k); | ||
if (!desc || ("get" in desc ? !m.__esModule : desc.writable || desc.configurable)) { | ||
desc = { enumerable: true, get: function() { return m[k]; } }; | ||
} | ||
Object.defineProperty(o, k2, desc); | ||
}) : (function(o, m, k, k2) { | ||
if (k2 === undefined) k2 = k; | ||
o[k2] = m[k]; | ||
})); | ||
var __setModuleDefault = (this && this.__setModuleDefault) || (Object.create ? (function(o, v) { | ||
Object.defineProperty(o, "default", { enumerable: true, value: v }); | ||
}) : function(o, v) { | ||
o["default"] = v; | ||
}); | ||
var __importStar = (this && this.__importStar) || function (mod) { | ||
if (mod && mod.__esModule) return mod; | ||
var result = {}; | ||
if (mod != null) for (var k in mod) if (k !== "default" && Object.prototype.hasOwnProperty.call(mod, k)) __createBinding(result, mod, k); | ||
__setModuleDefault(result, mod); | ||
return result; | ||
}; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
exports.load = void 0; | ||
var tslib_1 = require("tslib"); | ||
var options_1 = tslib_1.__importStar(require("./options")); | ||
var staticMethods = tslib_1.__importStar(require("./static")); | ||
var cheerio_1 = require("./cheerio"); | ||
var parse_1 = tslib_1.__importDefault(require("./parse")); | ||
/** | ||
* Create a querying function, bound to a document created from the provided | ||
* markup. Note that similar to web browser contexts, this operation may | ||
* introduce `<html>`, `<head>`, and `<body>` elements; set `isDocument` to | ||
* `false` to switch to fragment mode and disable this. | ||
* | ||
* @param content - Markup to be loaded. | ||
* @param options - Options for the created instance. | ||
* @param isDocument - Allows parser to be switched to fragment mode. | ||
* @returns The loaded document. | ||
* @see {@link https://cheerio.js.org#loading} for additional usage information. | ||
*/ | ||
function load(content, options, isDocument) { | ||
if (isDocument === void 0) { isDocument = true; } | ||
if (content == null) { | ||
throw new Error('cheerio.load() expects a string'); | ||
} | ||
var internalOpts = tslib_1.__assign(tslib_1.__assign({}, options_1.default), options_1.flatten(options)); | ||
var root = parse_1.default(content, internalOpts, isDocument); | ||
/** Create an extended class here, so that extensions only live on one instance. */ | ||
var LoadedCheerio = /** @class */ (function (_super) { | ||
tslib_1.__extends(LoadedCheerio, _super); | ||
function LoadedCheerio() { | ||
return _super !== null && _super.apply(this, arguments) || this; | ||
exports.getLoad = void 0; | ||
var options_js_1 = __importStar(require("./options.js")); | ||
var staticMethods = __importStar(require("./static.js")); | ||
var cheerio_js_1 = require("./cheerio.js"); | ||
var utils_js_1 = require("./utils.js"); | ||
function getLoad(parse, render) { | ||
/** | ||
* Create a querying function, bound to a document created from the provided markup. | ||
* | ||
* Note that similar to web browser contexts, this operation may introduce | ||
* `<html>`, `<head>`, and `<body>` elements; set `isDocument` to `false` to | ||
* switch to fragment mode and disable this. | ||
* | ||
* @param content - Markup to be loaded. | ||
* @param options - Options for the created instance. | ||
* @param isDocument - Allows parser to be switched to fragment mode. | ||
* @returns The loaded document. | ||
* @see {@link https://cheerio.js.org#loading} for additional usage information. | ||
*/ | ||
return function load(content, options, isDocument) { | ||
if (isDocument === void 0) { isDocument = true; } | ||
if (content == null) { | ||
throw new Error('cheerio.load() expects a string'); | ||
} | ||
return LoadedCheerio; | ||
}(cheerio_1.Cheerio)); | ||
function initialize(selector, context, r, opts) { | ||
if (r === void 0) { r = root; } | ||
return new LoadedCheerio(selector, context, r, tslib_1.__assign(tslib_1.__assign({}, internalOpts), options_1.flatten(opts))); | ||
} | ||
// Add in static methods & properties | ||
Object.assign(initialize, staticMethods, { | ||
load: load, | ||
// `_root` and `_options` are used in static methods. | ||
_root: root, | ||
_options: internalOpts, | ||
// Add `fn` for plugins | ||
fn: LoadedCheerio.prototype, | ||
// Add the prototype here to maintain `instanceof` behavior. | ||
prototype: LoadedCheerio.prototype, | ||
}); | ||
return initialize; | ||
var internalOpts = __assign(__assign({}, options_js_1.default), (0, options_js_1.flatten)(options)); | ||
var initialRoot = parse(content, internalOpts, isDocument, null); | ||
/** Create an extended class here, so that extensions only live on one instance. */ | ||
var LoadedCheerio = /** @class */ (function (_super) { | ||
__extends(LoadedCheerio, _super); | ||
function LoadedCheerio() { | ||
return _super !== null && _super.apply(this, arguments) || this; | ||
} | ||
LoadedCheerio.prototype._make = function (selector, context) { | ||
var cheerio = initialize(selector, context); | ||
cheerio.prevObject = this; | ||
return cheerio; | ||
}; | ||
LoadedCheerio.prototype._parse = function (content, options, isDocument, context) { | ||
return parse(content, options, isDocument, context); | ||
}; | ||
LoadedCheerio.prototype._render = function (dom) { | ||
return render(dom, this.options); | ||
}; | ||
return LoadedCheerio; | ||
}(cheerio_js_1.Cheerio)); | ||
function initialize(selector, context, root, opts) { | ||
if (root === void 0) { root = initialRoot; } | ||
// $($) | ||
if (selector && (0, utils_js_1.isCheerio)(selector)) | ||
return selector; | ||
var options = __assign(__assign({}, internalOpts), (0, options_js_1.flatten)(opts)); | ||
var r = typeof root === 'string' | ||
? [parse(root, options, false, null)] | ||
: 'length' in root | ||
? root | ||
: [root]; | ||
var rootInstance = (0, utils_js_1.isCheerio)(r) | ||
? r | ||
: new LoadedCheerio(r, null, options); | ||
// Add a cyclic reference, so that calling methods on `_root` never fails. | ||
rootInstance._root = rootInstance; | ||
// $(), $(null), $(undefined), $(false) | ||
if (!selector) { | ||
return new LoadedCheerio(undefined, rootInstance, options); | ||
} | ||
var elements = typeof selector === 'string' && (0, utils_js_1.isHtml)(selector) | ||
? // $(<html>) | ||
parse(selector, options, false, null).children | ||
: isNode(selector) | ||
? // $(dom) | ||
[selector] | ||
: Array.isArray(selector) | ||
? // $([dom]) | ||
selector | ||
: undefined; | ||
var instance = new LoadedCheerio(elements, rootInstance, options); | ||
if (elements) { | ||
return instance; | ||
} | ||
if (typeof selector !== 'string') { | ||
throw new Error('Unexpected type of selector'); | ||
} | ||
// We know that our selector is a string now. | ||
var search = selector; | ||
var searchContext = !context | ||
? // If we don't have a context, maybe we have a root, from loading | ||
rootInstance | ||
: typeof context === 'string' | ||
? (0, utils_js_1.isHtml)(context) | ||
? // $('li', '<ul>...</ul>') | ||
new LoadedCheerio([parse(context, options, false, null)], rootInstance, options) | ||
: // $('li', 'ul') | ||
((search = "".concat(context, " ").concat(search)), rootInstance) | ||
: (0, utils_js_1.isCheerio)(context) | ||
? // $('li', $) | ||
context | ||
: // $('li', node), $('li', [nodes]) | ||
new LoadedCheerio(Array.isArray(context) ? context : [context], rootInstance, options); | ||
// If we still don't have a context, return | ||
if (!searchContext) | ||
return instance; | ||
/* | ||
* #id, .class, tag | ||
*/ | ||
return searchContext.find(search); | ||
} | ||
// Add in static methods & properties | ||
Object.assign(initialize, staticMethods, { | ||
load: load, | ||
// `_root` and `_options` are used in static methods. | ||
_root: initialRoot, | ||
_options: internalOpts, | ||
// Add `fn` for plugins | ||
fn: LoadedCheerio.prototype, | ||
// Add the prototype here to maintain `instanceof` behavior. | ||
prototype: LoadedCheerio.prototype, | ||
}); | ||
return initialize; | ||
}; | ||
} | ||
exports.load = load; | ||
exports.getLoad = getLoad; | ||
function isNode(obj) { | ||
return (!!obj.name || | ||
obj.type === 'root' || | ||
obj.type === 'text' || | ||
obj.type === 'comment'); | ||
} | ||
//# sourceMappingURL=load.js.map |
@@ -0,4 +1,10 @@ | ||
/// <reference types="node" /> | ||
import type { DomHandlerOptions } from 'domhandler'; | ||
import type { ParserOptions } from 'htmlparser2'; | ||
/** Options accepted by htmlparser2, the default parser for XML. */ | ||
import type { Options as SelectOptions } from 'cheerio-select'; | ||
/** | ||
* Options accepted by htmlparser2, the default parser for XML. | ||
* | ||
* @see https://github.com/fb55/htmlparser2/wiki/Parser-options | ||
*/ | ||
export interface HTMLParser2Options extends DomHandlerOptions, ParserOptions { | ||
@@ -13,20 +19,73 @@ } | ||
} | ||
/** Internal options for Cheerio. */ | ||
export interface InternalOptions extends HTMLParser2Options, Parse5Options { | ||
_useHtmlParser2?: boolean; | ||
} | ||
/** | ||
* Options accepted by Cheerio. | ||
* | ||
* Please note that parser-specific options are *only recognized* if the | ||
* Please note that parser-specific options are _only recognized_ if the | ||
* relevant parser is used. | ||
*/ | ||
export interface CheerioOptions extends HTMLParser2Options, Parse5Options { | ||
/** Suggested way of configuring htmlparser2 when wanting to parse XML. */ | ||
/** Recommended way of configuring htmlparser2 when wanting to parse XML. */ | ||
xml?: HTMLParser2Options | boolean; | ||
/** The base URI for the document. Used for the `href` and `src` props. */ | ||
baseURI?: string | URL; | ||
/** | ||
* Is the document in quirks mode? | ||
* | ||
* This will lead to `.className` and `#id` being case-insensitive. | ||
* | ||
* @default false | ||
*/ | ||
quirksMode?: SelectOptions['quirksMode']; | ||
/** | ||
* Extension point for pseudo-classes. | ||
* | ||
* Maps from names to either strings of functions. | ||
* | ||
* - A string value is a selector that the element must match to be selected. | ||
* - A function is called with the element as its first argument, and optional | ||
* parameters second. If it returns true, the element is selected. | ||
* | ||
* @example | ||
* | ||
* ```js | ||
* const $ = cheerio.load( | ||
* '<div class="foo"></div><div data-bar="boo"></div>', | ||
* { | ||
* pseudos: { | ||
* // `:foo` is an alias for `div.foo` | ||
* foo: 'div.foo', | ||
* // `:bar(val)` is equivalent to `[data-bar=val s]` | ||
* bar: (el, val) => el.attribs['data-bar'] === val, | ||
* }, | ||
* } | ||
* ); | ||
* | ||
* $(':foo').length; // 1 | ||
* $('div:bar(boo)').length; // 1 | ||
* $('div:bar(baz)').length; // 0 | ||
* ``` | ||
*/ | ||
pseudos?: SelectOptions['pseudos']; | ||
} | ||
/** Internal options for Cheerio. */ | ||
export interface InternalOptions extends Omit<CheerioOptions, 'xml'> { | ||
/** | ||
* Whether to use htmlparser2. | ||
* | ||
* This is set to true if `xml` is set to true. | ||
*/ | ||
_useHtmlParser2?: boolean; | ||
} | ||
declare const defaultOpts: CheerioOptions; | ||
/** Cheerio default options. */ | ||
export default defaultOpts; | ||
/** | ||
* Flatten the options for Cheerio. | ||
* | ||
* This will set `_useHtmlParser2` to true if `xml` is set to true. | ||
* | ||
* @param options - The options to flatten. | ||
* @returns The flattened options. | ||
*/ | ||
export declare function flatten(options?: CheerioOptions | null): InternalOptions | undefined; | ||
//# sourceMappingURL=options.d.ts.map |
"use strict"; | ||
var __assign = (this && this.__assign) || function () { | ||
__assign = Object.assign || function(t) { | ||
for (var s, i = 1, n = arguments.length; i < n; i++) { | ||
s = arguments[i]; | ||
for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p)) | ||
t[p] = s[p]; | ||
} | ||
return t; | ||
}; | ||
return __assign.apply(this, arguments); | ||
}; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
exports.flatten = void 0; | ||
var tslib_1 = require("tslib"); | ||
var defaultOpts = { | ||
@@ -15,2 +25,10 @@ xml: false, | ||
}; | ||
/** | ||
* Flatten the options for Cheerio. | ||
* | ||
* This will set `_useHtmlParser2` to true if `xml` is set to true. | ||
* | ||
* @param options - The options to flatten. | ||
* @returns The flattened options. | ||
*/ | ||
function flatten(options) { | ||
@@ -20,5 +38,6 @@ return (options === null || options === void 0 ? void 0 : options.xml) | ||
? xmlModeDefault | ||
: tslib_1.__assign(tslib_1.__assign({}, xmlModeDefault), options.xml) | ||
: __assign(__assign({}, xmlModeDefault), options.xml) | ||
: options !== null && options !== void 0 ? options : undefined; | ||
} | ||
exports.flatten = flatten; | ||
//# sourceMappingURL=options.js.map |
/// <reference types="node" /> | ||
import { Node, Document, NodeWithChildren } from 'domhandler'; | ||
import type { InternalOptions } from './options'; | ||
export default function parse(content: string | Document | Node | Node[] | Buffer, options: InternalOptions, isDocument: boolean): Document; | ||
import { AnyNode, Document, ParentNode } from 'domhandler'; | ||
import type { InternalOptions } from './options.js'; | ||
/** | ||
* Get the parse function with options. | ||
* | ||
* @param parser - The parser function. | ||
* @returns The parse function with options. | ||
*/ | ||
export declare function getParse(parser: (content: string, options: InternalOptions, isDocument: boolean, context: ParentNode | null) => Document): (content: string | Document | AnyNode | AnyNode[] | Buffer, options: InternalOptions, isDocument: boolean, context: ParentNode | null) => Document; | ||
/** | ||
* Update the dom structure, for one changed layer. | ||
@@ -12,3 +18,3 @@ * | ||
*/ | ||
export declare function update(newChilds: Node[] | Node, parent: NodeWithChildren | null): Node | null; | ||
export declare function update(newChilds: AnyNode[] | AnyNode, parent: ParentNode | null): ParentNode | null; | ||
//# sourceMappingURL=parse.d.ts.map |
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
exports.update = void 0; | ||
var htmlparser2_1 = require("htmlparser2"); | ||
var htmlparser2_adapter_1 = require("./parsers/htmlparser2-adapter"); | ||
var parse5_adapter_1 = require("./parsers/parse5-adapter"); | ||
exports.update = exports.getParse = void 0; | ||
var domutils_1 = require("domutils"); | ||
var domhandler_1 = require("domhandler"); | ||
/* | ||
* Parser | ||
/** | ||
* Get the parse function with options. | ||
* | ||
* @param parser - The parser function. | ||
* @returns The parse function with options. | ||
*/ | ||
function parse(content, options, isDocument) { | ||
if (typeof Buffer !== 'undefined' && Buffer.isBuffer(content)) { | ||
content = content.toString(); | ||
} | ||
if (typeof content === 'string') { | ||
return options.xmlMode || options._useHtmlParser2 | ||
? htmlparser2_adapter_1.parse(content, options) | ||
: parse5_adapter_1.parse(content, options, isDocument); | ||
} | ||
var doc = content; | ||
if (!Array.isArray(doc) && domhandler_1.isDocument(doc)) { | ||
// If `doc` is already a root, just return it | ||
return doc; | ||
} | ||
// Add conent to new root element | ||
var root = new domhandler_1.Document([]); | ||
// Update the DOM using the root | ||
update(doc, root); | ||
return root; | ||
function getParse(parser) { | ||
/** | ||
* Parse a HTML string or a node. | ||
* | ||
* @param content - The HTML string or node. | ||
* @param options - The parser options. | ||
* @param isDocument - If `content` is a document. | ||
* @param context - The context node in the DOM tree. | ||
* @returns The parsed document node. | ||
*/ | ||
return function parse(content, options, isDocument, context) { | ||
if (typeof Buffer !== 'undefined' && Buffer.isBuffer(content)) { | ||
content = content.toString(); | ||
} | ||
if (typeof content === 'string') { | ||
return parser(content, options, isDocument, context); | ||
} | ||
var doc = content; | ||
if (!Array.isArray(doc) && (0, domhandler_1.isDocument)(doc)) { | ||
// If `doc` is already a root, just return it | ||
return doc; | ||
} | ||
// Add conent to new root element | ||
var root = new domhandler_1.Document([]); | ||
// Update the DOM using the root | ||
update(doc, root); | ||
return root; | ||
}; | ||
} | ||
exports.default = parse; | ||
exports.getParse = getParse; | ||
/** | ||
@@ -54,3 +64,3 @@ * Update the dom structure, for one changed layer. | ||
if (node.parent && node.parent.children !== arr) { | ||
htmlparser2_1.DomUtils.removeElement(node); | ||
(0, domutils_1.removeElement)(node); | ||
} | ||
@@ -69,1 +79,2 @@ if (parent) { | ||
exports.update = update; | ||
//# sourceMappingURL=parse.js.map |
@@ -1,9 +0,20 @@ | ||
import { Node, Document } from 'domhandler'; | ||
import type { InternalOptions } from '../options'; | ||
interface Parse5Options extends InternalOptions { | ||
context?: Node; | ||
} | ||
export declare function parse(content: string, options: Parse5Options, isDocument?: boolean): Document; | ||
export declare function render(dom: Node | ArrayLike<Node>): string; | ||
export {}; | ||
import { AnyNode, Document, ParentNode } from 'domhandler'; | ||
import type { InternalOptions } from '../options.js'; | ||
/** | ||
* Parse the content with `parse5` in the context of the given `ParentNode`. | ||
* | ||
* @param content - The content to parse. | ||
* @param options - A set of options to use to parse. | ||
* @param isDocument - Whether to parse the content as a full HTML document. | ||
* @param context - The context in which to parse the content. | ||
* @returns The parsed content. | ||
*/ | ||
export declare function parseWithParse5(content: string, options: InternalOptions, isDocument: boolean, context: ParentNode | null): Document; | ||
/** | ||
* Renders the given DOM tree with `parse5` and returns the result as a string. | ||
* | ||
* @param dom - The DOM tree to render. | ||
* @returns The rendered document. | ||
*/ | ||
export declare function renderWithParse5(dom: AnyNode | ArrayLike<AnyNode>): string; | ||
//# sourceMappingURL=parse5-adapter.d.ts.map |
"use strict"; | ||
var __spreadArray = (this && this.__spreadArray) || function (to, from, pack) { | ||
if (pack || arguments.length === 2) for (var i = 0, l = from.length, ar; i < l; i++) { | ||
if (ar || !(i in from)) { | ||
if (!ar) ar = Array.prototype.slice.call(from, 0, i); | ||
ar[i] = from[i]; | ||
} | ||
} | ||
return to.concat(ar || Array.prototype.slice.call(from)); | ||
}; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
exports.render = exports.parse = void 0; | ||
var tslib_1 = require("tslib"); | ||
exports.renderWithParse5 = exports.parseWithParse5 = void 0; | ||
var domhandler_1 = require("domhandler"); | ||
var parse5_1 = require("parse5"); | ||
var parse5_htmlparser2_tree_adapter_1 = tslib_1.__importDefault(require("parse5-htmlparser2-tree-adapter")); | ||
function parse(content, options, isDocument) { | ||
var parse5_htmlparser2_tree_adapter_1 = require("parse5-htmlparser2-tree-adapter"); | ||
/** | ||
* Parse the content with `parse5` in the context of the given `ParentNode`. | ||
* | ||
* @param content - The content to parse. | ||
* @param options - A set of options to use to parse. | ||
* @param isDocument - Whether to parse the content as a full HTML document. | ||
* @param context - The context in which to parse the content. | ||
* @returns The parsed content. | ||
*/ | ||
function parseWithParse5(content, options, isDocument, context) { | ||
var opts = { | ||
@@ -13,14 +30,18 @@ scriptingEnabled: typeof options.scriptingEnabled === 'boolean' | ||
: true, | ||
treeAdapter: parse5_htmlparser2_tree_adapter_1.default, | ||
treeAdapter: parse5_htmlparser2_tree_adapter_1.adapter, | ||
sourceCodeLocationInfo: options.sourceCodeLocationInfo, | ||
}; | ||
var context = options.context; | ||
// @ts-expect-error The tree adapter unfortunately doesn't return the exact types. | ||
return isDocument | ||
? parse5_1.parse(content, opts) | ||
: // @ts-expect-error Same issue again. | ||
parse5_1.parseFragment(context, content, opts); | ||
? (0, parse5_1.parse)(content, opts) | ||
: (0, parse5_1.parseFragment)(context, content, opts); | ||
} | ||
exports.parse = parse; | ||
function render(dom) { | ||
exports.parseWithParse5 = parseWithParse5; | ||
var renderOpts = { treeAdapter: parse5_htmlparser2_tree_adapter_1.adapter }; | ||
/** | ||
* Renders the given DOM tree with `parse5` and returns the result as a string. | ||
* | ||
* @param dom - The DOM tree to render. | ||
* @returns The rendered document. | ||
*/ | ||
function renderWithParse5(dom) { | ||
var _a; | ||
@@ -35,9 +56,14 @@ /* | ||
var node = nodes[index]; | ||
if (domhandler_1.isDocument(node)) { | ||
(_a = Array.prototype.splice).call.apply(_a, tslib_1.__spreadArray([nodes, index, 1], node.children)); | ||
if ((0, domhandler_1.isDocument)(node)) { | ||
(_a = Array.prototype.splice).call.apply(_a, __spreadArray([nodes, index, 1], node.children, false)); | ||
} | ||
} | ||
// @ts-expect-error Types don't align here either. | ||
return parse5_1.serialize({ children: nodes }, { treeAdapter: parse5_htmlparser2_tree_adapter_1.default }); | ||
var result = ''; | ||
for (var index = 0; index < nodes.length; index += 1) { | ||
var node = nodes[index]; | ||
result += (0, parse5_1.serializeOuter)(node, renderOpts); | ||
} | ||
return result; | ||
} | ||
exports.render = render; | ||
exports.renderWithParse5 = renderWithParse5; | ||
//# sourceMappingURL=parse5-adapter.js.map |
@@ -0,4 +1,5 @@ | ||
import type { BasicAcceptedElems } from './types.js'; | ||
import type { CheerioAPI, Cheerio } from '.'; | ||
import { Node, Document } from 'domhandler'; | ||
import { CheerioOptions } from './options'; | ||
import type { AnyNode, Document } from 'domhandler'; | ||
import { CheerioOptions } from './options.js'; | ||
/** | ||
@@ -10,3 +11,3 @@ * Renders the document. | ||
*/ | ||
export declare function html(this: CheerioAPI | void, options?: CheerioOptions): string; | ||
export declare function html(this: CheerioAPI, options?: CheerioOptions): string; | ||
/** | ||
@@ -19,3 +20,3 @@ * Renders the document. | ||
*/ | ||
export declare function html(this: CheerioAPI | void, dom?: string | ArrayLike<Node> | Node, options?: CheerioOptions): string; | ||
export declare function html(this: CheerioAPI, dom?: BasicAcceptedElems<AnyNode>, options?: CheerioOptions): string; | ||
/** | ||
@@ -27,10 +28,14 @@ * Render the document as XML. | ||
*/ | ||
export declare function xml(this: CheerioAPI, dom?: string | ArrayLike<Node> | Node): string; | ||
export declare function xml(this: CheerioAPI, dom?: BasicAcceptedElems<AnyNode>): string; | ||
/** | ||
* Render the document as text. | ||
* | ||
* This returns the `textContent` of the passed elements. The result will | ||
* include the contents of `script` and `stype` elements. To avoid this, use | ||
* `.prop('innerText')` instead. | ||
* | ||
* @param elements - Elements to render. | ||
* @returns The rendered document. | ||
*/ | ||
export declare function text(this: CheerioAPI | void, elements?: ArrayLike<Node>): string; | ||
export declare function text(this: CheerioAPI | void, elements?: ArrayLike<AnyNode>): string; | ||
/** | ||
@@ -48,3 +53,3 @@ * Parses a string into an array of DOM nodes. The `context` argument has no | ||
*/ | ||
export declare function parseHTML(this: CheerioAPI, data: string, context?: unknown | boolean, keepScripts?: boolean): Node[]; | ||
export declare function parseHTML(this: CheerioAPI, data: string, context?: unknown | boolean, keepScripts?: boolean): AnyNode[]; | ||
export declare function parseHTML(this: CheerioAPI, data?: '' | null): null; | ||
@@ -76,3 +81,3 @@ /** | ||
*/ | ||
export declare function contains(container: Node, contained: Node): boolean; | ||
export declare function contains(container: AnyNode, contained: AnyNode): boolean; | ||
interface WritableArrayLike<T> extends ArrayLike<T> { | ||
@@ -79,0 +84,0 @@ length: number; |
"use strict"; | ||
var __assign = (this && this.__assign) || function () { | ||
__assign = Object.assign || function(t) { | ||
for (var s, i = 1, n = arguments.length; i < n; i++) { | ||
s = arguments[i]; | ||
for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p)) | ||
t[p] = s[p]; | ||
} | ||
return t; | ||
}; | ||
return __assign.apply(this, arguments); | ||
}; | ||
var __createBinding = (this && this.__createBinding) || (Object.create ? (function(o, m, k, k2) { | ||
if (k2 === undefined) k2 = k; | ||
var desc = Object.getOwnPropertyDescriptor(m, k); | ||
if (!desc || ("get" in desc ? !m.__esModule : desc.writable || desc.configurable)) { | ||
desc = { enumerable: true, get: function() { return m[k]; } }; | ||
} | ||
Object.defineProperty(o, k2, desc); | ||
}) : (function(o, m, k, k2) { | ||
if (k2 === undefined) k2 = k; | ||
o[k2] = m[k]; | ||
})); | ||
var __setModuleDefault = (this && this.__setModuleDefault) || (Object.create ? (function(o, v) { | ||
Object.defineProperty(o, "default", { enumerable: true, value: v }); | ||
}) : function(o, v) { | ||
o["default"] = v; | ||
}); | ||
var __importStar = (this && this.__importStar) || function (mod) { | ||
if (mod && mod.__esModule) return mod; | ||
var result = {}; | ||
if (mod != null) for (var k in mod) if (k !== "default" && Object.prototype.hasOwnProperty.call(mod, k)) __createBinding(result, mod, k); | ||
__setModuleDefault(result, mod); | ||
return result; | ||
}; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
exports.merge = exports.contains = exports.root = exports.parseHTML = exports.text = exports.xml = exports.html = void 0; | ||
var tslib_1 = require("tslib"); | ||
var options_1 = tslib_1.__importStar(require("./options")); | ||
var cheerio_select_1 = require("cheerio-select"); | ||
var htmlparser2_1 = require("htmlparser2"); | ||
var parse5_adapter_1 = require("./parsers/parse5-adapter"); | ||
var htmlparser2_adapter_1 = require("./parsers/htmlparser2-adapter"); | ||
var domutils_1 = require("domutils"); | ||
var options_js_1 = __importStar(require("./options.js")); | ||
/** | ||
@@ -19,13 +49,5 @@ * Helper function to render a DOM. | ||
function render(that, dom, options) { | ||
var _a; | ||
var toRender = dom | ||
? typeof dom === 'string' | ||
? cheerio_select_1.select(dom, (_a = that === null || that === void 0 ? void 0 : that._root) !== null && _a !== void 0 ? _a : [], options) | ||
: dom | ||
: that === null || that === void 0 ? void 0 : that._root.children; | ||
if (!toRender) | ||
if (!that) | ||
return ''; | ||
return options.xmlMode || options._useHtmlParser2 | ||
? htmlparser2_adapter_1.render(toRender, options) | ||
: parse5_adapter_1.render(toRender); | ||
return that(dom !== null && dom !== void 0 ? dom : that._root.children, null, undefined, options).toString(); | ||
} | ||
@@ -38,4 +60,5 @@ /** | ||
*/ | ||
function isOptions(dom) { | ||
return (typeof dom === 'object' && | ||
function isOptions(dom, options) { | ||
return (!options && | ||
typeof dom === 'object' && | ||
dom != null && | ||
@@ -52,6 +75,3 @@ !('length' in dom) && | ||
*/ | ||
if (!options && isOptions(dom)) { | ||
options = dom; | ||
dom = undefined; | ||
} | ||
var toRender = isOptions(dom) ? ((options = dom), undefined) : dom; | ||
/* | ||
@@ -61,4 +81,4 @@ * Sometimes `$.html()` is used without preloading html, | ||
*/ | ||
var opts = tslib_1.__assign(tslib_1.__assign(tslib_1.__assign({}, options_1.default), (this ? this._options : {})), options_1.flatten(options !== null && options !== void 0 ? options : {})); | ||
return render(this || undefined, dom, opts); | ||
var opts = __assign(__assign(__assign({}, options_js_1.default), this === null || this === void 0 ? void 0 : this._options), (0, options_js_1.flatten)(options !== null && options !== void 0 ? options : {})); | ||
return render(this, toRender, opts); | ||
} | ||
@@ -73,3 +93,3 @@ exports.html = html; | ||
function xml(dom) { | ||
var options = tslib_1.__assign(tslib_1.__assign({}, this._options), { xmlMode: true }); | ||
var options = __assign(__assign({}, this._options), { xmlMode: true }); | ||
return render(this, dom, options); | ||
@@ -81,2 +101,6 @@ } | ||
* | ||
* This returns the `textContent` of the passed elements. The result will | ||
* include the contents of `script` and `stype` elements. To avoid this, use | ||
* `.prop('innerText')` instead. | ||
* | ||
* @param elements - Elements to render. | ||
@@ -89,11 +113,3 @@ * @returns The rendered document. | ||
for (var i = 0; i < elems.length; i++) { | ||
var elem = elems[i]; | ||
if (htmlparser2_1.DomUtils.isText(elem)) | ||
ret += elem.data; | ||
else if (htmlparser2_1.DomUtils.hasChildren(elem) && | ||
elem.type !== htmlparser2_1.ElementType.Comment && | ||
elem.type !== htmlparser2_1.ElementType.Script && | ||
elem.type !== htmlparser2_1.ElementType.Style) { | ||
ret += text(elem.children); | ||
} | ||
ret += (0, domutils_1.textContent)(elems[i]); | ||
} | ||
@@ -111,3 +127,3 @@ return ret; | ||
} | ||
var parsed = this.load(data, options_1.default, false); | ||
var parsed = this.load(data, options_js_1.default, false); | ||
if (!keepScripts) { | ||
@@ -196,2 +212,4 @@ parsed('script').remove(); | ||
/** | ||
* Checks if an object is array-like. | ||
* | ||
* @param item - Item to check. | ||
@@ -217,1 +235,2 @@ * @returns Indicates if the item is array-like. | ||
} | ||
//# sourceMappingURL=static.js.map |
@@ -9,8 +9,8 @@ declare type LowercaseLetters = 'a' | 'b' | 'c' | 'd' | 'e' | 'f' | 'g' | 'h' | 'i' | 'j' | 'k' | 'l' | 'm' | 'n' | 'o' | 'p' | 'q' | 'r' | 's' | 't' | 'u' | 'v' | 'w' | 'x' | 'y' | 'z'; | ||
export declare type SelectorType = `${SelectorSpecial}${AlphaNumeric}${string}` | `${AlphaNumeric}${string}`; | ||
import type { Cheerio } from './cheerio'; | ||
import type { Node } from 'domhandler'; | ||
import type { Cheerio } from './cheerio.js'; | ||
import type { AnyNode } from 'domhandler'; | ||
/** Elements that can be passed to manipulation methods. */ | ||
export declare type BasicAcceptedElems<T extends Node> = Cheerio<T> | T[] | T | string; | ||
export declare type BasicAcceptedElems<T extends AnyNode> = Cheerio<T> | T[] | T | string; | ||
/** Elements that can be passed to manipulation methods, including functions. */ | ||
export declare type AcceptedElems<T extends Node> = BasicAcceptedElems<T> | ((this: T, i: number, el: T) => BasicAcceptedElems<T>); | ||
export declare type AcceptedElems<T extends AnyNode> = BasicAcceptedElems<T> | ((this: T, i: number, el: T) => BasicAcceptedElems<T>); | ||
/** Function signature, for traversal methods. */ | ||
@@ -17,0 +17,0 @@ export declare type FilterFunction<T> = (this: T, i: number, el: T) => boolean; |
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
//# sourceMappingURL=types.js.map |
@@ -1,4 +0,3 @@ | ||
import { DomUtils } from 'htmlparser2'; | ||
import { Node } from 'domhandler'; | ||
import type { Cheerio } from './cheerio'; | ||
import { type AnyNode } from 'domhandler'; | ||
import type { Cheerio } from './cheerio.js'; | ||
/** | ||
@@ -11,6 +10,6 @@ * Check if the DOM element is a tag. | ||
* @category Utils | ||
* @param type - DOM node to check. | ||
* @param type - The DOM node to check. | ||
* @returns Whether the node is a tag. | ||
*/ | ||
export declare const isTag: typeof DomUtils.isTag; | ||
export { isTag } from 'domhandler'; | ||
/** | ||
@@ -29,3 +28,3 @@ * Checks if an object is a Cheerio instance. | ||
* @category Utils | ||
* @param str - String to be converted. | ||
* @param str - The string to be converted. | ||
* @returns String in camel case notation. | ||
@@ -40,3 +39,3 @@ */ | ||
* @category Utils | ||
* @param str - String to be converted. | ||
* @param str - The string to be converted. | ||
* @returns String in "CSS case". | ||
@@ -52,7 +51,7 @@ */ | ||
* @category Utils | ||
* @param array - Array to iterate over. | ||
* @param array - The array to iterate over. | ||
* @param fn - Function to call. | ||
* @returns The original instance. | ||
*/ | ||
export declare function domEach<T extends Node, Arr extends ArrayLike<T> = Cheerio<T>>(array: Arr, fn: (elem: T, index: number) => void): Arr; | ||
export declare function domEach<T extends AnyNode, Arr extends ArrayLike<T> = Cheerio<T>>(array: Arr, fn: (elem: T, index: number) => void): Arr; | ||
/** | ||
@@ -64,12 +63,15 @@ * Create a deep copy of the given DOM structure. Sets the parents of the copies | ||
* @category Utils | ||
* @param dom - The htmlparser2-compliant DOM structure. | ||
* @param dom - The domhandler-compliant DOM structure. | ||
* @returns - The cloned DOM. | ||
*/ | ||
export declare function cloneDom<T extends Node>(dom: T | T[]): T[]; | ||
export declare function cloneDom<T extends AnyNode>(dom: T | T[]): T[]; | ||
/** | ||
* Check if string is HTML. | ||
* | ||
* Tests for a `<` within a string, immediate followed by a letter and | ||
* eventually followed by a `>`. | ||
* | ||
* @private | ||
* @category Utils | ||
* @param str - String to check. | ||
* @param str - The string to check. | ||
* @returns Indicates if `str` is HTML. | ||
@@ -76,0 +78,0 @@ */ |
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
exports.isHtml = exports.cloneDom = exports.domEach = exports.cssCase = exports.camelCase = exports.isCheerio = exports.isTag = void 0; | ||
var htmlparser2_1 = require("htmlparser2"); | ||
var domhandler_1 = require("domhandler"); | ||
@@ -13,6 +12,7 @@ /** | ||
* @category Utils | ||
* @param type - DOM node to check. | ||
* @param type - The DOM node to check. | ||
* @returns Whether the node is a tag. | ||
*/ | ||
exports.isTag = htmlparser2_1.DomUtils.isTag; | ||
var domhandler_2 = require("domhandler"); | ||
Object.defineProperty(exports, "isTag", { enumerable: true, get: function () { return domhandler_2.isTag; } }); | ||
/** | ||
@@ -34,3 +34,3 @@ * Checks if an object is a Cheerio instance. | ||
* @category Utils | ||
* @param str - String to be converted. | ||
* @param str - The string to be converted. | ||
* @returns String in camel case notation. | ||
@@ -48,3 +48,3 @@ */ | ||
* @category Utils | ||
* @param str - String to be converted. | ||
* @param str - The string to be converted. | ||
* @returns String in "CSS case". | ||
@@ -63,3 +63,3 @@ */ | ||
* @category Utils | ||
* @param array - Array to iterate over. | ||
* @param array - The array to iterate over. | ||
* @param fn - Function to call. | ||
@@ -81,3 +81,3 @@ * @returns The original instance. | ||
* @category Utils | ||
* @param dom - The htmlparser2-compliant DOM structure. | ||
* @param dom - The domhandler-compliant DOM structure. | ||
* @returns - The cloned DOM. | ||
@@ -87,4 +87,4 @@ */ | ||
var clone = 'length' in dom | ||
? Array.prototype.map.call(dom, function (el) { return domhandler_1.cloneNode(el, true); }) | ||
: [domhandler_1.cloneNode(dom, true)]; | ||
? Array.prototype.map.call(dom, function (el) { return (0, domhandler_1.cloneNode)(el, true); }) | ||
: [(0, domhandler_1.cloneNode)(dom, true)]; | ||
// Add a root node around the cloned nodes | ||
@@ -98,21 +98,32 @@ var root = new domhandler_1.Document(clone); | ||
exports.cloneDom = cloneDom; | ||
var CharacterCodes; | ||
(function (CharacterCodes) { | ||
CharacterCodes[CharacterCodes["LowerA"] = 97] = "LowerA"; | ||
CharacterCodes[CharacterCodes["LowerZ"] = 122] = "LowerZ"; | ||
CharacterCodes[CharacterCodes["UpperA"] = 65] = "UpperA"; | ||
CharacterCodes[CharacterCodes["UpperZ"] = 90] = "UpperZ"; | ||
CharacterCodes[CharacterCodes["Exclamation"] = 33] = "Exclamation"; | ||
})(CharacterCodes || (CharacterCodes = {})); | ||
/** | ||
* A simple way to check for HTML strings. Tests for a `<` within a string, | ||
* immediate followed by a letter and eventually followed by a `>`. | ||
* | ||
* @private | ||
*/ | ||
var quickExpr = /<[a-zA-Z][^]*>/; | ||
/** | ||
* Check if string is HTML. | ||
* | ||
* Tests for a `<` within a string, immediate followed by a letter and | ||
* eventually followed by a `>`. | ||
* | ||
* @private | ||
* @category Utils | ||
* @param str - String to check. | ||
* @param str - The string to check. | ||
* @returns Indicates if `str` is HTML. | ||
*/ | ||
function isHtml(str) { | ||
// Run the regex | ||
return quickExpr.test(str); | ||
var tagStart = str.indexOf('<'); | ||
if (tagStart < 0 || tagStart > str.length - 3) | ||
return false; | ||
var tagChar = str.charCodeAt(tagStart + 1); | ||
return (((tagChar >= CharacterCodes.LowerA && tagChar <= CharacterCodes.LowerZ) || | ||
(tagChar >= CharacterCodes.UpperA && tagChar <= CharacterCodes.UpperZ) || | ||
tagChar === CharacterCodes.Exclamation) && | ||
str.includes('>', tagStart + 2)); | ||
} | ||
exports.isHtml = isHtml; | ||
//# sourceMappingURL=utils.js.map |
{ | ||
"name": "cheerio", | ||
"version": "1.0.0-rc.10", | ||
"version": "1.0.0-rc.11", | ||
"description": "Tiny, fast, and elegant implementation of core jQuery designed specifically for the server", | ||
@@ -29,2 +29,13 @@ "author": "Matt Mueller <mattmuelle@gmail.com>", | ||
"types": "lib/index.d.ts", | ||
"module": "lib/esm/index.js", | ||
"exports": { | ||
".": { | ||
"require": "./lib/index.js", | ||
"import": "./lib/esm/index.js" | ||
}, | ||
"./lib/slim": { | ||
"require": "./lib/slim.js", | ||
"import": "./lib/esm/slim.js" | ||
} | ||
}, | ||
"files": [ | ||
@@ -37,39 +48,36 @@ "lib" | ||
"dependencies": { | ||
"cheerio-select": "^1.5.0", | ||
"dom-serializer": "^1.3.2", | ||
"domhandler": "^4.2.0", | ||
"htmlparser2": "^6.1.0", | ||
"parse5": "^6.0.1", | ||
"parse5-htmlparser2-tree-adapter": "^6.0.1", | ||
"tslib": "^2.2.0" | ||
"cheerio-select": "^2.1.0", | ||
"dom-serializer": "^2.0.0", | ||
"domhandler": "^5.0.3", | ||
"domutils": "^3.0.1", | ||
"htmlparser2": "^8.0.1", | ||
"parse5": "^7.0.0", | ||
"parse5-htmlparser2-tree-adapter": "^7.0.0", | ||
"tslib": "^2.4.0" | ||
}, | ||
"devDependencies": { | ||
"@octokit/graphql": "^4.6.2", | ||
"@types/benchmark": "^2.1.0", | ||
"@types/jest": "^26.0.23", | ||
"@types/jsdom": "^16.2.10", | ||
"@types/node": "^15.12.1", | ||
"@types/node-fetch": "^2.5.10", | ||
"@types/parse5": "^6.0.0", | ||
"@types/parse5-htmlparser2-tree-adapter": "^6.0.0", | ||
"@typescript-eslint/eslint-plugin": "^4.26.0", | ||
"@typescript-eslint/parser": "^4.26.0", | ||
"@octokit/graphql": "^4.8.0", | ||
"@types/benchmark": "^2.1.1", | ||
"@types/jest": "^27.5.0", | ||
"@types/node": "^17.0.35", | ||
"@typescript-eslint/eslint-plugin": "^5.25.0", | ||
"@typescript-eslint/parser": "^5.25.0", | ||
"benchmark": "^2.1.4", | ||
"eslint": "^7.28.0", | ||
"eslint-config-prettier": "^8.3.0", | ||
"eslint-plugin-jest": "^24.3.6", | ||
"eslint-plugin-jsdoc": "^35.1.3", | ||
"eslint": "^8.15.0", | ||
"eslint-config-prettier": "^8.5.0", | ||
"eslint-plugin-jest": "^26.2.2", | ||
"eslint-plugin-jsdoc": "^39.3.0", | ||
"eslint-plugin-node": "^11.1.0", | ||
"husky": "^4.3.8", | ||
"jest": "^27.0.4", | ||
"husky": "^8.0.1", | ||
"jest": "^27.5.1", | ||
"jquery": "^3.6.0", | ||
"jsdom": "^16.6.0", | ||
"lint-staged": "^11.0.0", | ||
"node-fetch": "^2.6.1", | ||
"prettier": "^2.3.1", | ||
"prettier-plugin-jsdoc": "0.3.22", | ||
"ts-jest": "^27.0.3", | ||
"ts-node": "^10.0.0", | ||
"typedoc": "^0.20.36", | ||
"typescript": "^4.2.4" | ||
"jsdom": "^19.0.0", | ||
"lint-staged": "^12.4.1", | ||
"prettier": "^2.6.2", | ||
"prettier-plugin-jsdoc": "0.3.38", | ||
"ts-jest": "^27.1.4", | ||
"ts-node": "^10.7.0", | ||
"typedoc": "^0.22.15", | ||
"typescript": "^4.6.4", | ||
"undici": "^5.2.0" | ||
}, | ||
@@ -86,10 +94,12 @@ "scripts": { | ||
"format:prettier": "npm run format:prettier:raw -- --write", | ||
"format:prettier:raw": "prettier \"**/*.{js,ts,md,json,yml}\" --ignore-path .gitignore", | ||
"format:prettier:raw": "prettier \"**/*.{{m,c,}js,ts,md,json,yml}\" --ignore-path .gitignore", | ||
"build:docs": "typedoc --hideGenerator src/index.ts", | ||
"benchmark": "ts-node benchmark/benchmark.ts --regex \"^(?!.*highmem)\"", | ||
"benchmark": "npm run build:cjs && ts-node benchmark/benchmark.ts --regex \"^(?!.*highmem)\"", | ||
"update-sponsors": "ts-node scripts/fetch-sponsors.ts", | ||
"bench": "npm run benchmark", | ||
"pre-commit": "lint-staged", | ||
"build": "tsc", | ||
"prepublishOnly": "npm run build" | ||
"build": "npm run build:cjs && npm run build:esm", | ||
"build:cjs": "tsc --sourceRoot https://raw.githubusercontent.com/cheeriojs/cheerio/$(git rev-parse HEAD)/src/", | ||
"build:esm": "npm run build:cjs -- --module esnext --target es2019 --outDir lib/esm && echo '{\"type\":\"module\"}' > lib/esm/package.json", | ||
"prepublishOnly": "npm run build", | ||
"prepare": "husky install" | ||
}, | ||
@@ -115,4 +125,8 @@ "prettier": { | ||
"/__fixtures__/" | ||
] | ||
], | ||
"coverageProvider": "v8", | ||
"moduleNameMapper": { | ||
"^(.*)\\.js$": "$1" | ||
} | ||
} | ||
} |
@@ -56,3 +56,3 @@ <h1 align="center">cheerio</h1> | ||
Cheerio parses markup and provides an API for traversing/manipulating the resulting data structure. It does not interpret the result as a web browser does. Specifically, it does _not_ produce a visual rendering, apply CSS, load external resources, or execute JavaScript. This makes Cheerio **much, much faster than other solutions**. If your use case requires any of this functionality, you should consider projects like [Puppeteer](https://github.com/puppeteer/puppeteer) or [JSDom](https://github.com/jsdom/jsdom). | ||
Cheerio parses markup and provides an API for traversing/manipulating the resulting data structure. It does not interpret the result as a web browser does. Specifically, it does _not_ produce a visual rendering, apply CSS, load external resources, or execute JavaScript which is common for a SPA (single page application). This makes Cheerio **much, much faster than other solutions**. If your use case requires any of this functionality, you should consider browser automation software like [Puppeteer](https://github.com/puppeteer/puppeteer) and [Playwright](https://github.com/microsoft/playwright) or DOM emulation projects like [JSDom](https://github.com/jsdom/jsdom). | ||
@@ -138,2 +138,7 @@ ## API | ||
#### Using `htmlparser2` | ||
Cheerio ships with two parsers, `parse5` and `htmlparser2`. The | ||
former is the default for HTML, the latter the default for XML. | ||
Some users may wish to parse markup with the `htmlparser2` library, and | ||
@@ -161,2 +166,9 @@ traverse/manipulate the resulting structure with Cheerio. This may be the case | ||
If you want to save some bytes, you can use Cheerio's _slim_ export, which | ||
always uses `htmlparser2`: | ||
```js | ||
const cheerio = require('cheerio/lib/slim'); | ||
``` | ||
### Selectors | ||
@@ -216,17 +228,2 @@ | ||
By default, `html` will leave some tags open. Sometimes you may instead want to render a valid XML document. For example, you might parse the following XML snippet: | ||
```js | ||
const $ = cheerio.load( | ||
'<media:thumbnail url="http://www.foo.com/keyframe.jpg" width="75" height="50" time="12:05:01.123"/>' | ||
); | ||
``` | ||
... and later want to render to XML. To do this, you can use the 'xml' utility function: | ||
```js | ||
$.xml(); | ||
//=> <media:thumbnail url="http://www.foo.com/keyframe.jpg" width="75" height="50" time="12:05:01.123"/> | ||
``` | ||
You may also render the text content of a Cheerio object using the `text` static method: | ||
@@ -253,3 +250,3 @@ | ||
If you're using TypeScript, you should also add a type definition for your new method: | ||
If you're using TypeScript, you should add a type definition for your new method: | ||
@@ -293,4 +290,23 @@ ```ts | ||
<a href="https://substack.com/" target="_blank"></a> | ||
<a href="https://www.airbnb.com/" target="_blank">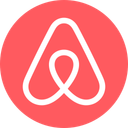</a> | ||
<a href="https://substack.com/" target="_blank" rel="noopener noreferrer"> | ||
<img style="max-height:128px;max-width:128px" src="https://avatars.githubusercontent.com/u/53023767?v=4&s=128" title="Substack" alt="Substack"></img> | ||
</a> | ||
<a href="https://cryptocasinos.com/" target="_blank" rel="noopener noreferrer"> | ||
<img style="max-height:128px;max-width:128px" src="https://images.opencollective.com/cryptocasinos/99b168e/logo.png" title="CryptoCasinos" alt="CryptoCasinos"></img> | ||
</a> | ||
<a href="https://www.casinoonlineaams.com" target="_blank" rel="noopener noreferrer"> | ||
<img style="max-height:128px;max-width:128px" src="https://images.opencollective.com/casinoonlineaamscom/da74236/logo.png" title="Casinoonlineaams.com" alt="Casinoonlineaams.com"></img> | ||
</a> | ||
<a href="https://casinofiables.com/" target="_blank" rel="noopener noreferrer"> | ||
<img style="max-height:128px;max-width:128px" src="https://images.opencollective.com/casinofiables-com/b824bab/logo.png" title="Casinofiables.com" alt="Casinofiables.com"></img> | ||
</a> | ||
<a href="https://apify.com/" target="_blank" rel="noopener noreferrer"> | ||
<img style="max-height:128px;max-width:128px" src="https://avatars.githubusercontent.com/u/24586296?v=4&s=128" title="Apify" alt="Apify"></img> | ||
</a> | ||
<a href="https://freebets.ltd.uk" target="_blank" rel="noopener noreferrer"> | ||
<img style="max-height:128px;max-width:128px" src="https://images.opencollective.com/freebets/e21c41b/logo.png" title="Free Bets" alt="Free Bets"></img> | ||
</a> | ||
<a href="https://casinoutansvensklicens.co/" target="_blank" rel="noopener noreferrer"> | ||
<img style="max-height:128px;max-width:128px" src="https://images.opencollective.com/casino-utan-svensk-licens3/f7e9357/logo.png" title="Casino utan svensk licens" alt="Casino utan svensk licens"></img> | ||
</a> | ||
@@ -305,4 +321,23 @@ <!-- END SPONSORS --> | ||
<a href="https://medium.com/norch" target="_blank">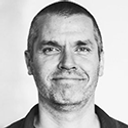</a> | ||
<a href="https://nishant-singh.com" target="_blank"></a> | ||
<a href="https://www.airbnb.com/" target="_blank" rel="noopener noreferrer"> | ||
<img style="max-height:128px;max-width:128px" src="https://images.opencollective.com/airbnb/d327d66/logo.png" title="Airbnb" alt="Airbnb"></img> | ||
</a> | ||
<a href="https://kafidoff.com" target="_blank" rel="noopener noreferrer"> | ||
<img style="max-height:128px;max-width:128px" src="https://images.opencollective.com/kafidoff-vasy/d7ff85c/avatar.png" title="Vasy Kafidoff" alt="Vasy Kafidoff"></img> | ||
</a> | ||
<a href="https://medium.com/norch" target="_blank" rel="noopener noreferrer"> | ||
<img style="max-height:128px;max-width:128px" src="https://images.opencollective.com/espenklem/6075b19/avatar.png" title="Espen Klem" alt="Espen Klem"></img> | ||
</a> | ||
<a href="https://jarrodldavis.com" target="_blank" rel="noopener noreferrer"> | ||
<img style="max-height:128px;max-width:128px" src="https://avatars.githubusercontent.com/u/235875?v=4&s=128" title="Jarrod Davis" alt="Jarrod Davis"></img> | ||
</a> | ||
<a href="https://nishant-singh.com" target="_blank" rel="noopener noreferrer"> | ||
<img style="max-height:128px;max-width:128px" src="https://avatars.githubusercontent.com/u/10304344?u=2f98c0a745b5352c6e758b9a5bc7a9d9d4e3e969&v=4&s=128" title="Nishant Singh" alt="Nishant Singh"></img> | ||
</a> | ||
<a href="https://github.com/gauthamchandra" target="_blank" rel="noopener noreferrer"> | ||
<img style="max-height:128px;max-width:128px" src="https://avatars.githubusercontent.com/u/5430280?u=1115bcd3ed7aa8b2a62ff28f62ee4c2b92729903&v=4&s=128" title="Gautham Chandra" alt="Gautham Chandra"></img> | ||
</a> | ||
<a href="http://www.dr-chuck.com/" target="_blank" rel="noopener noreferrer"> | ||
<img style="max-height:128px;max-width:128px" src="https://avatars.githubusercontent.com/u/1197222?u=d6dc85c064736ab851c6d9e3318dcdd1be00fb2c&v=4&s=128" title="Charles Severance" alt="Charles Severance"></img> | ||
</a> | ||
@@ -309,0 +344,0 @@ <!-- END SPONSORS --> |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
557627
24
124
10983
355
8
+ Addeddomutils@^3.0.1
+ Addedcheerio-select@2.1.0(transitive)
+ Addedcss-select@5.1.0(transitive)
+ Addeddom-serializer@2.0.0(transitive)
+ Addeddomhandler@5.0.3(transitive)
+ Addeddomutils@3.1.0(transitive)
+ Addedentities@4.5.0(transitive)
+ Addedhtmlparser2@8.0.2(transitive)
+ Addedparse5@7.2.0(transitive)
+ Addedparse5-htmlparser2-tree-adapter@7.1.0(transitive)
+ Addedtslib@2.8.0(transitive)
- Removedcheerio-select@1.6.0(transitive)
- Removedcss-select@4.3.0(transitive)
- Removeddom-serializer@1.4.1(transitive)
- Removeddomhandler@4.3.1(transitive)
- Removeddomutils@2.8.0(transitive)
- Removedentities@2.2.0(transitive)
- Removedhtmlparser2@6.1.0(transitive)
- Removedparse5@6.0.1(transitive)
- Removedparse5-htmlparser2-tree-adapter@6.0.1(transitive)
- Removedtslib@2.8.1(transitive)
Updatedcheerio-select@^2.1.0
Updateddom-serializer@^2.0.0
Updateddomhandler@^5.0.3
Updatedhtmlparser2@^8.0.1
Updatedparse5@^7.0.0
Updatedtslib@^2.4.0