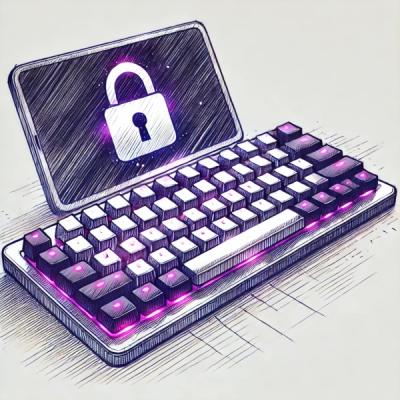
Research
Security News
Malicious npm Package Typosquats react-login-page to Deploy Keylogger
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
chober
Advanced tools
Readme
A collection of methods that are used by programmers every day. We decided to put all the methods together so as not to copy them from project to project.
Install with npm.
$ npm i chober
or nstall with script
.
<script src="chober.min.js"></script>
import { isEmpty } from 'chober';
isEmpty([]);
// => true
import _c from 'chober';
_c.isEmpty([]);
// => true
_c.clone({ key: 'value' });
// => { key: 'value' }
If you import one method, other methods will not be imported.
import isEmpty from 'chober/lib/isEmpty'; // ~600B
isEmpty([]);
// => true
_c
binds to the window
.
<script src="chober.min.js"></script>
_c.isEmpty([]);
// => true
If you want to add your methods, read the instructions below.
git clone https://github.com/BrooonS/chober.git
.lib
folder. Example: lib/yourMethod.js
.index.js
.npm run build
command.Example
// lib/yourMethod.js
/**
* Method to sum two numbers.
*
* @param {Number} a First number.
* @param {Number} b Second number.
* @returns {Number}
*
* @example
* math(2, 2)
* // => 4
*/
function math(a, b) {
return a + b;
}
export default math;
// index.js
import yourMethod from './lib/yourMethod';
export {
... // other methods
yourMethod,
};
class Chober {
constructor() {
Object.assign(this, {
... // other methods
yourMethod,
});
}
}
Clone any item.
item
Any
clone([1, null, '3'])
// => [1, null, '3']
Useful for implementing behavior that should only happen after a repeated action has completed.
func
function
delay
Number
window.addEventListener('scroll', debounce(() => {
console.log(Math.random());
}, 100));
Returns the first item of array or `number` items.
array
Array
number
Number
first([1, null, '3'])
// => [1]
first([1, null, '3'], 2)
// => [1, null]
Format number.
number
Number,String
symbol
String
formatNumer(1234)
// => '1 234'
formatNumer('1234', ',')
// => '1,234'
Get cookie.
key
String
getCookie('someCookie')
Get only numbers from string.
string
String
getNumbers('+7 (123) 456-78-90')
// => '71234567890'
Get absolute coordinates of an element.
element
HTMLelement
getOffset(document.querySelector('#element'))
Get query from url.
arrayFields
Array
// http://github.com/?value=test&field=hi&field=hello
getQuery()
// => { value: 'test', field: ['hi', 'hello'] }
Get scrollbar width.
Invert object.
object
Object
invertObject({ key: 'value' })
// => { value: 'key' }
Check is empty object, string, array or other type.
item
*
isEmpty({ test: 'some value' })
// => false
isEmpty([1, null, '3'])
// => false
isEmpty('qwe')
// => false
isEmpty(true)
// => true
isEmpty(1)
// => true
isEmpty(undefined)
// => true
isEmpty(null)
// => true
Preload an image by its path.
imgPath
String
preloadImage('some/path/to/img')
Remove cookie.
key
String
removeCookie('testCookie')
Scroll to element in DOM.
selector
String
Set cookie.
key
String
value
String
expireIn
Number
setCookie('name', 'value', 60000) // 60000 - one minute
© Valery Strelets
FAQs
Unknown package
We found that chober demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
Security News
The JavaScript community has launched the e18e initiative to improve ecosystem performance by cleaning up dependency trees, speeding up critical parts of the ecosystem, and documenting lighter alternatives to established tools.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.