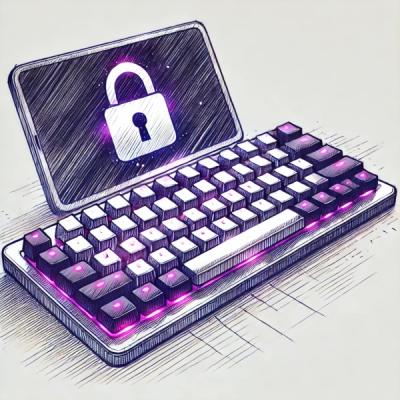
Research
Security News
Malicious npm Package Typosquats react-login-page to Deploy Keylogger
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
chromedriver
Advanced tools
Package description
The chromedriver npm package provides a way to manage and control the ChromeDriver, which is a standalone server that implements the WebDriver protocol for Chrome. It is used for automating web applications for testing purposes, enabling you to interact with web pages and perform various browser automation tasks.
Browser Automation
This code sample demonstrates how to use the chromedriver package with Selenium WebDriver to automate a simple browser task. It opens Google, searches for 'webdriver', and waits for the search results page to load.
const { Builder, By, Key, until } = require('selenium-webdriver');
const chrome = require('selenium-webdriver/chrome');
const chromedriver = require('chromedriver');
chrome.setDefaultService(new chrome.ServiceBuilder(chromedriver.path).build());
(async function example() {
let driver = await new Builder().forBrowser('chrome').build();
try {
await driver.get('http://www.google.com/ncr');
await driver.findElement(By.name('q')).sendKeys('webdriver', Key.RETURN);
await driver.wait(until.titleIs('webdriver - Google Search'), 1000);
} finally {
await driver.quit();
}
})();
Headless Browser Testing
This code sample shows how to run Chrome in headless mode using the chromedriver package. Headless mode is useful for running tests in environments without a graphical user interface.
const { Builder, By, Key, until } = require('selenium-webdriver');
const chrome = require('selenium-webdriver/chrome');
const chromedriver = require('chromedriver');
chrome.setDefaultService(new chrome.ServiceBuilder(chromedriver.path).build());
(async function example() {
let options = new chrome.Options();
options.addArguments('headless');
let driver = await new Builder().forBrowser('chrome').setChromeOptions(options).build();
try {
await driver.get('http://www.google.com/ncr');
await driver.findElement(By.name('q')).sendKeys('webdriver', Key.RETURN);
await driver.wait(until.titleIs('webdriver - Google Search'), 1000);
} finally {
await driver.quit();
}
})();
Taking Screenshots
This code sample demonstrates how to take a screenshot of the current browser window using the chromedriver package. The screenshot is saved as a PNG file.
const { Builder, By, Key, until } = require('selenium-webdriver');
const chrome = require('selenium-webdriver/chrome');
const chromedriver = require('chromedriver');
chrome.setDefaultService(new chrome.ServiceBuilder(chromedriver.path).build());
(async function example() {
let driver = await new Builder().forBrowser('chrome').build();
try {
await driver.get('http://www.google.com/ncr');
await driver.findElement(By.name('q')).sendKeys('webdriver', Key.RETURN);
await driver.wait(until.titleIs('webdriver - Google Search'), 1000);
let screenshot = await driver.takeScreenshot();
require('fs').writeFileSync('screenshot.png', screenshot, 'base64');
} finally {
await driver.quit();
}
})();
The geckodriver package provides a way to manage and control the GeckoDriver, which is a WebDriver implementation for Firefox. It is similar to chromedriver but is specifically designed for automating Firefox browser tasks.
The edgedriver package provides a way to manage and control the EdgeDriver, which is a WebDriver implementation for Microsoft Edge. It offers similar functionalities to chromedriver but is tailored for automating tasks in the Edge browser.
The selenium-webdriver package is a JavaScript implementation of the WebDriver API, which allows you to write tests that interact with various browsers, including Chrome, Firefox, and Edge. It provides a higher-level API compared to chromedriver and can be used with different browser drivers.
Readme
An NPM wrapper for Selenium ChromeDriver.
npm install chromedriver
Or grab the source and
node ./install.js
What this is really doing is just grabbing a particular "blessed" (by this module) version of ChromeDriver. As new versions are released and vetted, this module will be updated accordingly.
The package has been set up to fetch and run ChromeDriver for MacOS (darwin), Linux based platforms (as identified by nodejs), and Windows. If you spot any platform weirdnesses, let us know or send a patch.
bin/chromedriver [arguments]
And npm will install a link to the binary in node_modules/.bin
as
it is wont to do.
The package exports a path
string that contains the path to the
chromdriver binary/executable.
Below is an example of using this package via node.
var childProcess = require('child_process');
var chromedriver = require('chromedriver');
var binPath = chromedriver.path;
var childArgs = [
'some argument'
];
childProcess.execFile(binPath, childArgs, function(err, stdout, stderr) {
// handle results
});
You can also use the start and stop methods:
var chromedriver = require('chromedriver');
chromedriver.start();
//run your tests
chromedriver.stop();
The NPM package version tracks the version of chromedriver that will be installed, with an additional build number that is used for revisions to the installer.
Chromedriver is not a library for NodeJS.
This is an NPM wrapper and can be used to conveniently make ChromeDriver available It is not a Node JS wrapper.
Questions, comments, bug reports, and pull requests are all welcome. Submit them at the project on GitHub.
Bug reports that include steps-to-reproduce (including code) are the best. Even better, make them in the form of pull requests.
Thanks for Obvious and their PhantomJS project for heavy inspiration! Check their project on Github.
Licensed under the Apache License, Version 2.0.
FAQs
Unknown package
The npm package chromedriver receives a total of 511,365 weekly downloads. As such, chromedriver popularity was classified as popular.
We found that chromedriver demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
Security News
The JavaScript community has launched the e18e initiative to improve ecosystem performance by cleaning up dependency trees, speeding up critical parts of the ecosystem, and documenting lighter alternatives to established tools.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.