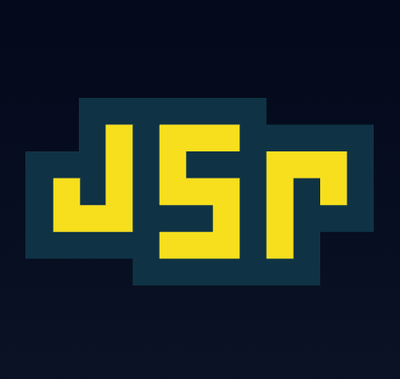
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
clean-css
Advanced tools
clean-css is a fast and efficient CSS optimizer for Node.js and the web. It minifies CSS by removing whitespace, comments, and unnecessary characters, merging identical rules, and applying other optimization techniques. It can be used to reduce the size of CSS files, which can lead to faster page load times and improved performance.
Minification
This feature allows you to minify CSS by removing unnecessary characters and whitespace, which results in smaller file sizes.
const CleanCSS = require('clean-css');
new CleanCSS().minify('a { font-weight: bold; }', function (error, output) {
console.log(output.styles);
});
Source Map Generation
clean-css can generate source maps for the minified CSS, which is useful for debugging minified code in the browser.
const CleanCSS = require('clean-css');
new CleanCSS({ sourceMap: true, sourceMapInlineSources: true }).minify({ 'styles.css': { styles: 'a { color: #000 }' } }, function (error, output) {
console.log(output.sourceMap.toString());
});
Merging of Identical CSS Rules
This feature merges identical CSS rules into one to reduce file size and redundancy in the code.
const CleanCSS = require('clean-css');
new CleanCSS().minify('a { color: red; } a { color: red; }', function (error, output) {
console.log(output.styles);
});
Optimizing CSS Properties
clean-css can optimize CSS properties, such as converting hex color codes to shorter names when possible.
const CleanCSS = require('clean-css');
new CleanCSS().minify('a { color: #FF0000; }', function (error, output) {
console.log(output.styles);
});
cssnano is a modular CSS minifier that utilizes PostCSS. It offers optimizations like reducing calc() references, z-index rebasing, and discarding unused at-rules. Compared to clean-css, cssnano provides a plugin system and can be more configurable due to its modular nature.
uglifycss is a simple CSS minifier that works both in Node.js and the browser. It is less feature-rich compared to clean-css and does not support source maps, but it is straightforward to use for basic minification tasks.
csso (CSS Optimizer) is a CSS minifier with structural optimizations. It can restructure CSS rules to reduce file size and also merge selectors and properties. csso is known for its aggressive optimization techniques, which sometimes can be more efficient than clean-css, but may also require careful testing to ensure the final CSS behaves as expected.
Clean-css is a fast and efficient Node.js library for minifying CSS files.
According to tests it is one of the best available.
Node.js 0.10+ (tested on CentOS, Ubuntu, OS X 10.6+, and Windows 7+) or io.js 3.0+
npm install clean-css
Clean-css accepts the following command line arguments (please make sure
you use <source-file>
as the very last argument to avoid potential issues):
cleancss [options] source-file, [source-file, ...]
-h, --help output usage information
-v, --version output the version number
-b, --keep-line-breaks Keep line breaks
-c, --compatibility [ie7|ie8] Force compatibility mode (see Readme for advanced examples)
-d, --debug Shows debug information (minification time & compression efficiency)
-o, --output [output-file] Use [output-file] as output instead of STDOUT
-r, --root [root-path] Set a root path to which resolve absolute @import rules
-s, --skip-import Disable @import processing
-t, --timeout [seconds] Per connection timeout when fetching remote @imports (defaults to 5 seconds)
--rounding-precision [n] Rounds to `N` decimal places. Defaults to 2. -1 disables rounding
--s0 Remove all special comments, i.e. /*! comment */
--s1 Remove all special comments but the first one
--semantic-merging Enables unsafe mode by assuming BEM-like semantic stylesheets (warning, this may break your styling!)
--skip-advanced Disable advanced optimizations - ruleset reordering & merging
--skip-aggressive-merging Disable properties merging based on their order
--skip-import-from [rules] Disable @import processing for specified rules
--skip-media-merging Disable @media merging
--skip-rebase Disable URLs rebasing
--skip-restructuring Disable restructuring optimizations
--skip-shorthand-compacting Disable shorthand compacting
--source-map Enables building input's source map
--source-map-inline-sources Enables inlining sources inside source maps
To minify a public.css file into public-min.css do:
cleancss -o public-min.css public.css
To minify the same public.css into the standard output skip the -o
parameter:
cleancss public.css
More likely you would like to concatenate a couple of files. If you are on a Unix-like system:
cat one.css two.css three.css | cleancss -o merged-and-minified.css
On Windows:
type one.css two.css three.css | cleancss -o merged-and-minified.css
Or even gzip the result at once:
cat one.css two.css three.css | cleancss | gzip -9 -c > merged-minified-and-gzipped.css.gz
var CleanCSS = require('clean-css');
var source = 'a{font-weight:bold;}';
var minified = new CleanCSS().minify(source).styles;
CleanCSS constructor accepts a hash as a parameter, i.e.,
new CleanCSS(options)
with the following options available:
advanced
- set to false to disable advanced optimizations - selector & property merging, reduction, etc.aggressiveMerging
- set to false to disable aggressive merging of properties.benchmark
- turns on benchmarking mode measuring time spent on cleaning up (run npm run bench
to see example)compatibility
- enables compatibility mode, see below for more examplesdebug
- set to true to get minification statistics under stats
property (see test/custom-test.js
for examples)inliner
- a hash of options for @import
inliner, see test/protocol-imports-test.js for examples, or this comment for a proxy use case.keepBreaks
- whether to keep line breaks (default is false)keepSpecialComments
- *
for keeping all (default), 1
for keeping first one only, 0
for removing allmediaMerging
- whether to merge @media
at-rules (default is true)processImport
- whether to process @import
rulesprocessImportFrom
- a list of @import
rules, can be ['all']
(default), ['local']
, ['remote']
, or a blacklisted path e.g. ['!fonts.googleapis.com']
rebase
- set to false to skip URL rebasingrelativeTo
- path to resolve relative @import
rules and URLsrestructuring
- set to false to disable restructuring in advanced optimizationsroot
- path to resolve absolute @import
rules and rebase relative URLsroundingPrecision
- rounding precision; defaults to 2
; -1
disables roundingsemanticMerging
- set to true to enable semantic merging mode which assumes BEM-like content (default is false as it's highly likely this will break your stylesheets - use with caution!)shorthandCompacting
- set to false to skip shorthand compacting (default is true unless sourceMap is set when it's false)sourceMap
- exposes source map under sourceMap
property, e.g. new CleanCSS().minify(source).sourceMap
(default is false)
If input styles are a product of CSS preprocessor (Less, Sass) an input source map can be passed as a string.sourceMapInlineSources
- set to true to inline sources inside a source map's sourcesContent
field (defaults to false)
It is also required to process inlined sources from input source maps.target
- path to a folder or an output file to which rebase all URLsThe output of minify
method (or the 2nd argument to passed callback) is a hash containing the following fields:
styles
- optimized output CSS as a stringsourceMap
- output source map (if requested with sourceMap
option)errors
- a list of errors raisedwarnings
- a list of warnings raisedstats
- a hash of statistic information (if requested with debug
option):
originalSize
- original content size (after import inlining)minifiedSize
- optimized content sizetimeSpent
- time spent on optimizationsefficiency
- a ratio of output size to input size (e.g. 25% if content was reduced from 100 bytes to 75 bytes)@import
s are processed correctly?In order to inline remote @import
statements you need to provide a callback to minify method, e.g.:
var CleanCSS = require('clean-css');
var source = '@import url(http://path/to/remote/styles);';
new CleanCSS().minify(source, function (errors, minified) {
// minified.styles
});
This is due to a fact, that, while local files can be read synchronously, remote resources can only be processed asynchronously.
If you don't provide a callback, then remote @import
s will be left intact.
First clone the source, then run:
npm run bench
for clean-css benchmarks (see test/bench.js for details)npm run browserify
to create the browser-ready clean-css versionnpm run check
to check JS sources with JSHintnpm test
for the test suiteSee CONTRIBUTING.md.
Use the /*!
notation instead of the standard one /*
:
/*!
Important comments included in minified output.
*/
Clean-css will handle it automatically for you (since version 1.1) in the following cases:
-o
/--output
to rebase URLs as relative to the output file.-r
/--root
to rebase URLs as absolute from the given root path.-r
/--root
takes precendence.relativeTo
and target
options for relative rebase (same as 1 in CLI).relativeTo
and root
options for absolute rebase (same as 2 in CLI).root
takes precendence over target
as in CLI.Source maps are supported since version 3.0.
Additionally to mapping original CSS files, clean-css also supports input source maps, so minified styles can be mapped into their Less or Sass sources directly.
Source maps are generated using source-map module from Mozilla.
To generate a source map, use --source-map
switch, e.g.:
cleancss --source-map --output styles.min.css styles.css
Name of the output file is required, so a map file, named by adding .map
suffix to output file name, can be created (styles.min.css.map in this case).
To generate a source map, use sourceMap: true
option, e.g.:
new CleanCSS({ sourceMap: true, target: pathToOutputDirectory })
.minify(source, function (minified) {
// access minified.sourceMap for SourceMapGenerator object
// see https://github.com/mozilla/source-map/#sourcemapgenerator for more details
// see https://github.com/jakubpawlowicz/clean-css/blob/master/bin/cleancss#L114 on how it's used in clean-css' CLI
});
Using API you can also pass an input source map directly:
new CleanCSS({ sourceMap: inputSourceMapAsString, target: pathToOutputDirectory })
.minify(source, function (minified) {
// access minified.sourceMap to access SourceMapGenerator object
// see https://github.com/mozilla/source-map/#sourcemapgenerator for more details
// see https://github.com/jakubpawlowicz/clean-css/blob/master/bin/cleancss#L114 on how it's used in clean-css' CLI
});
Or even multiple input source maps at once (available since version 3.1):
new CleanCSS({ sourceMap: true, target: pathToOutputDirectory }).minify({
'path/to/source/1': {
styles: '...styles...',
sourceMap: '...source-map...'
},
'path/to/source/2': {
styles: '...styles...',
sourceMap: '...source-map...'
}
}, function (minified) {
// access minified.sourceMap as above
});
new CleanCSS().minify(['path/to/file/one', 'path/to/file/two']);
new CleanCSS().minify({
'path/to/file/one': {
styles: 'contents of file one'
},
'path/to/file/two': {
styles: 'contents of file two'
}
});
Compatibility settings are controlled by --compatibility
switch (CLI) and compatibility
option (library mode).
In both modes the following values are allowed:
'ie7'
- Internet Explorer 7 compatibility mode'ie8'
- Internet Explorer 8 compatibility mode''
or '*'
(default) - Internet Explorer 9+ compatibility modeSince clean-css 3 a fine grained control is available over those settings, with the following options available:
'[+-]colors.opacity'
- - turn on (+) / off (-) rgba()
/ hsla()
declarations removal'[+-]properties.backgroundClipMerging'
- turn on / off background-clip merging into shorthand'[+-]properties.backgroundOriginMerging'
- turn on / off background-origin merging into shorthand'[+-]properties.backgroundSizeMerging'
- turn on / off background-size merging into shorthand'[+-]properties.colors'
- turn on / off any color optimizations'[+-]properties.ieBangHack'
- turn on / off IE bang hack removal'[+-]properties.iePrefixHack'
- turn on / off IE prefix hack removal'[+-]properties.ieSuffixHack'
- turn on / off IE suffix hack removal'[+-]properties.merging'
- turn on / off property merging based on understandability'[+-]properties.spaceAfterClosingBrace'
- turn on / off removing space after closing brace - url() no-repeat
into url()no-repeat
'[+-]properties.urlQuotes'
- turn on / off url()
quoting'[+-]properties.zeroUnits'
- turn on / off units removal after a 0
value'[+-]selectors.adjacentSpace'
- turn on / off extra space before nav
element'[+-]selectors.ie7Hack'
- turn on / off IE7 selector hack removal (*+html...
)'[+-]selectors.special'
- a regular expression with all special, unmergeable selectors (leave it empty unless you know what you are doing)'[+-]units.ch'
- turn on / off treating ch
as a proper unit'[+-]units.in'
- turn on / off treating in
as a proper unit'[+-]units.pc'
- turn on / off treating pc
as a proper unit'[+-]units.pt'
- turn on / off treating pt
as a proper unit'[+-]units.rem'
- turn on / off treating rem
as a proper unit'[+-]units.vh'
- turn on / off treating vh
as a proper unit'[+-]units.vm'
- turn on / off treating vm
as a proper unit'[+-]units.vmax'
- turn on / off treating vmax
as a proper unit'[+-]units.vmin'
- turn on / off treating vmin
as a proper unit'[+-]units.vm'
- turn on / off treating vm
as a proper unitFor example, using --compatibility 'ie8,+units.rem'
will ensure IE8 compatibility while enabling rem
units so the following style margin:0px 0rem
can be shortened to margin:0
, while in pure IE8 mode it can't be.
To pass a single off (-) switch in CLI please use the following syntax --compatibility *,-units.rem
.
In library mode you can also pass compatibility
as a hash of options.
All advanced optimizations are dispatched here, and this is what they do:
recursivelyOptimizeBlocks
- does all the following operations on a block (think @media
or @keyframe
at-rules);recursivelyOptimizeProperties
- optimizes properties in rulesets and "flat at-rules" (like @font-face) by splitting them into components (e.g. margin
into margin-(*)
), optimizing, and rebuilding them back. You may want to use shorthandCompacting
option to control whether you want to turn multiple (long-hand) properties into a shorthand ones;removeDuplicates
- gets rid of duplicate rulesets with exactly the same set of properties (think of including the same Sass / Less partial twice for no good reason);mergeAdjacent
- merges adjacent rulesets with the same selector or rules;reduceNonAdjacent
- identifies which properties are overridden in same-selector non-adjacent rulesets, and removes them;mergeNonAdjacentBySelector
- identifies same-selector non-adjacent rulesets which can be moved (!) to be merged, requires all intermediate rulesets to not redefine the moved properties, or if redefined to be either more coarse grained (e.g. margin
vs margin-top
) or have the same value;mergeNonAdjacentByBody
- same as the one above but for same-rules non-adjacent rulesets;restructure
- tries to reorganize different-selector different-rules rulesets so they take less space, e.g. .one{padding:0}.two{margin:0}.one{margin-bottom:3px}
into .two{margin:0}.one{padding:0;margin-bottom:3px}
;removeDuplicateMediaQueries
- removes duplicated @media
at-rules;mergeMediaQueries
- merges non-adjacent @media
at-rules by same rules as mergeNonAdjacentBy*
above;@import
processing, namely introducing the --skip-import
/
processImport
options.@import
processing inside comments.sys
package.Clean-css is released under the MIT License.
FAQs
A well-tested CSS minifier
The npm package clean-css receives a total of 13,698,919 weekly downloads. As such, clean-css popularity was classified as popular.
We found that clean-css demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.