color-space
Advanced tools
Comparing version 0.1.1 to 0.2.1
785
index.js
//TODO: save hue on setting sat = 0; | ||
var options = { | ||
illuminant: 'D65', | ||
observer: '2' | ||
}; | ||
var rgb = { | ||
hsl: function(rgb) { | ||
var r = rgb[0]/255, | ||
g = rgb[1]/255, | ||
b = rgb[2]/255, | ||
min = Math.min(r, g, b), | ||
max = Math.max(r, g, b), | ||
delta = max - min, | ||
h, s, l; | ||
if (max == min) | ||
h = 0; | ||
else if (r == max) | ||
h = (g - b) / delta; | ||
else if (g == max) | ||
h = 2 + (b - r) / delta; | ||
else if (b == max) | ||
h = 4 + (r - g)/ delta; | ||
h = Math.min(h * 60, 360); | ||
if (h < 0) | ||
h += 360; | ||
l = (min + max) / 2; | ||
if (max == min) | ||
s = 0; | ||
else if (l <= 0.5) | ||
s = delta / (max + min); | ||
else | ||
s = delta / (2 - max - min); | ||
return [h, s * 100, l * 100]; | ||
}, | ||
hsv: function(rgb) { | ||
var r = rgb[0], | ||
g = rgb[1], | ||
b = rgb[2], | ||
min = Math.min(r, g, b), | ||
max = Math.max(r, g, b), | ||
delta = max - min, | ||
h, s, v; | ||
if (max == 0) | ||
s = 0; | ||
else | ||
s = (delta/max * 1000)/10; | ||
if (max == min) | ||
h = 0; | ||
else if (r == max) | ||
h = (g - b) / delta; | ||
else if (g == max) | ||
h = 2 + (b - r) / delta; | ||
else if (b == max) | ||
h = 4 + (r - g) / delta; | ||
h = Math.min(h * 60, 360); | ||
if (h < 0) | ||
h += 360; | ||
v = ((max / 255) * 1000) / 10; | ||
return [h, s, v]; | ||
}, | ||
hwb: function(val) { | ||
var r = val[0], | ||
g = val[1], | ||
b = val[2], | ||
h = rgb.hsl(val)[0], | ||
w = 1/255 * Math.min(r, Math.min(g, b)), | ||
b = 1 - 1/255 * Math.max(r, Math.max(g, b)); | ||
return [h, w * 100, b * 100]; | ||
}, | ||
cmyk: function(rgb) { | ||
var r = rgb[0] / 255, | ||
g = rgb[1] / 255, | ||
b = rgb[2] / 255, | ||
c, m, y, k; | ||
k = Math.min(1 - r, 1 - g, 1 - b); | ||
c = (1 - r - k) / (1 - k) || 0; | ||
m = (1 - g - k) / (1 - k) || 0; | ||
y = (1 - b - k) / (1 - k) || 0; | ||
return [c * 100, m * 100, y * 100, k * 100]; | ||
}, | ||
xyz: function(rgb) { | ||
var r = rgb[0] / 255, | ||
g = rgb[1] / 255, | ||
b = rgb[2] / 255; | ||
// assume sRGB | ||
r = r > 0.04045 ? Math.pow(((r + 0.055) / 1.055), 2.4) : (r / 12.92); | ||
g = g > 0.04045 ? Math.pow(((g + 0.055) / 1.055), 2.4) : (g / 12.92); | ||
b = b > 0.04045 ? Math.pow(((b + 0.055) / 1.055), 2.4) : (b / 12.92); | ||
var x = (r * 0.4124) + (g * 0.3576) + (b * 0.1805); | ||
var y = (r * 0.2126) + (g * 0.7152) + (b * 0.0722); | ||
var z = (r * 0.0193) + (g * 0.1192) + (b * 0.9505); | ||
return [x * 100, y *100, z * 100]; | ||
}, | ||
lab: function(args) { | ||
var xyz = rgb.xyz(args), | ||
x = xyz[0], | ||
y = xyz[1], | ||
z = xyz[2], | ||
l, a, b; | ||
x /= 95.047; | ||
y /= 100; | ||
z /= 108.883; | ||
x = x > 0.008856 ? Math.pow(x, 1/3) : (7.787 * x) + (16 / 116); | ||
y = y > 0.008856 ? Math.pow(y, 1/3) : (7.787 * y) + (16 / 116); | ||
z = z > 0.008856 ? Math.pow(z, 1/3) : (7.787 * z) + (16 / 116); | ||
l = (116 * y) - 16; | ||
a = 500 * (x - y); | ||
b = 200 * (y - z); | ||
return [l, a, b]; | ||
}, | ||
lch: function(args) { | ||
return lab.lch(rgb.lab(args)); | ||
}, | ||
luv: function(args){ | ||
return xyz.luv(rgb.xyz(args)); | ||
}, | ||
lchuv: function(args){ | ||
return luv.lchuv(rgb.luv(args)); | ||
}, | ||
min: [0,0,0], | ||
max: [255,255,255], | ||
channel: ['red', 'green', 'blue'] | ||
}; | ||
var hsl = { | ||
rgb: function(hsl) { | ||
var h = hsl[0] / 360, | ||
s = hsl[1] / 100, | ||
l = hsl[2] / 100, | ||
t1, t2, t3, rgb, val; | ||
if (s == 0) { | ||
val = l * 255; | ||
return [val, val, val]; | ||
} | ||
if (l < 0.5) | ||
t2 = l * (1 + s); | ||
else | ||
t2 = l + s - l * s; | ||
t1 = 2 * l - t2; | ||
rgb = [0, 0, 0]; | ||
for (var i = 0; i < 3; i++) { | ||
t3 = h + 1 / 3 * - (i - 1); | ||
t3 < 0 && t3++; | ||
t3 > 1 && t3--; | ||
if (6 * t3 < 1) | ||
val = t1 + (t2 - t1) * 6 * t3; | ||
else if (2 * t3 < 1) | ||
val = t2; | ||
else if (3 * t3 < 2) | ||
val = t1 + (t2 - t1) * (2 / 3 - t3) * 6; | ||
else | ||
val = t1; | ||
rgb[i] = val * 255; | ||
} | ||
return rgb; | ||
}, | ||
hsv: function(hsl) { | ||
var h = hsl[0], | ||
s = hsl[1] / 100, | ||
l = hsl[2] / 100, | ||
sv, v; | ||
l *= 2; | ||
s *= (l <= 1) ? l : 2 - l; | ||
v = (l + s) / 2; | ||
sv = (2 * s) / (l + s); | ||
return [h, sv * 100, v * 100]; | ||
}, | ||
hwb: function(args) { | ||
return rgb.hwb(hsl.rgb(args)); | ||
}, | ||
cmyk: function(args) { | ||
return rgb.cmyk(hsl.rgb(args)); | ||
}, | ||
xyz: function(arg) { | ||
return rgb.xyz(hsl.rgb(arg)); | ||
}, | ||
lab: function(arg) { | ||
return rgb.lab(hsl.rgb(arg)); | ||
}, | ||
lch: function(arg) { | ||
return rgb.lch(hsl.rgb(arg)); | ||
}, | ||
luv: function(arg) { | ||
return rgb.luv(hsl.rgb(arg)); | ||
}, | ||
min: [0,0,0], | ||
max: [360,100,100], | ||
channel: ['hue', 'saturation', 'lightness'] | ||
}; | ||
var hsv = { | ||
rgb: function(hsv) { | ||
var h = hsv[0] / 60, | ||
s = hsv[1] / 100, | ||
v = hsv[2] / 100, | ||
hi = Math.floor(h) % 6; | ||
var f = h - Math.floor(h), | ||
p = 255 * v * (1 - s), | ||
q = 255 * v * (1 - (s * f)), | ||
t = 255 * v * (1 - (s * (1 - f))), | ||
v = 255 * v; | ||
switch(hi) { | ||
case 0: | ||
return [v, t, p]; | ||
case 1: | ||
return [q, v, p]; | ||
case 2: | ||
return [p, v, t]; | ||
case 3: | ||
return [p, q, v]; | ||
case 4: | ||
return [t, p, v]; | ||
case 5: | ||
return [v, p, q]; | ||
} | ||
}, | ||
hsl: function(hsv) { | ||
var h = hsv[0], | ||
s = hsv[1] / 100, | ||
v = hsv[2] / 100, | ||
sl, l; | ||
l = (2 - s) * v; | ||
sl = s * v; | ||
sl /= (l <= 1) ? l : 2 - l; | ||
sl = sl || 0; | ||
l /= 2; | ||
return [h, sl * 100, l * 100]; | ||
}, | ||
hwb: function(args) { | ||
return rgb.hwb(hsv.rgb(args)) | ||
}, | ||
cmyk: function(args) { | ||
return rgb.cmyk(hsv.rgb(args)); | ||
}, | ||
xyz: function(arg) { | ||
return rgb.xyz(hsv.rgb(arg)); | ||
}, | ||
lab: function(arg) { | ||
return rgb.lab(hsv.rgb(arg)); | ||
}, | ||
lch: function(arg) { | ||
return rgb.lch(hsv.rgb(arg)); | ||
}, | ||
luv: function(arg) { | ||
return rgb.luv(hsv.rgb(arg)); | ||
}, | ||
min: [0,0,0], | ||
max: [360,100,100], | ||
channel: ['hue', 'saturation', 'value'], | ||
alias: ['hsb'] | ||
}; | ||
var hwb = { | ||
// http://dev.w3.org/csswg/css-color/#hwb-to-rgb | ||
rgb: function(hwb) { | ||
var h = hwb[0] / 360, | ||
wh = hwb[1] / 100, | ||
bl = hwb[2] / 100, | ||
ratio = wh + bl, | ||
i, v, f, n; | ||
var r, g, b; | ||
// wh + bl cant be > 1 | ||
if (ratio > 1) { | ||
wh /= ratio; | ||
bl /= ratio; | ||
} | ||
i = Math.floor(6 * h); | ||
v = 1 - bl; | ||
f = 6 * h - i; | ||
if ((i & 0x01) != 0) { | ||
f = 1 - f; | ||
} | ||
n = wh + f * (v - wh); // linear interpolation | ||
switch (i) { | ||
default: | ||
case 6: | ||
case 0: r = v; g = n; b = wh; break; | ||
case 1: r = n; g = v; b = wh; break; | ||
case 2: r = wh; g = v; b = n; break; | ||
case 3: r = wh; g = n; b = v; break; | ||
case 4: r = n; g = wh; b = v; break; | ||
case 5: r = v; g = wh; b = n; break; | ||
} | ||
return [r * 255, g * 255, b * 255]; | ||
}, | ||
hsl: function(args) { | ||
return rgb.hsl(hwb.rgb(args)); | ||
}, | ||
hsv: function(args) { | ||
return rgb.hsv(hwb.rgb(args)); | ||
}, | ||
cmyk: function(args) { | ||
return rgb.cmyk(hwb.rgb(args)); | ||
}, | ||
xyz: function(args) { | ||
return rgb.xyz(hwb.rgb(args)); | ||
}, | ||
lab: function(arg) { | ||
return rgb.lab(hwb.rgb(arg)); | ||
}, | ||
lch: function(arg) { | ||
return rgb.lch(hwb.rgb(arg)); | ||
}, | ||
luv: function(arg) { | ||
return rgb.luv(hwb.rgb(arg)); | ||
}, | ||
min: [0,0,0], | ||
max: [360,100,100], | ||
channel: ['hue', 'whiteness', 'blackness'] | ||
}; | ||
var cmyk = { | ||
rgb: function(cmyk) { | ||
var c = cmyk[0] / 100, | ||
m = cmyk[1] / 100, | ||
y = cmyk[2] / 100, | ||
k = cmyk[3] / 100, | ||
r, g, b; | ||
r = 1 - Math.min(1, c * (1 - k) + k); | ||
g = 1 - Math.min(1, m * (1 - k) + k); | ||
b = 1 - Math.min(1, y * (1 - k) + k); | ||
return [r * 255, g * 255, b * 255]; | ||
}, | ||
hsl: function(args) { | ||
return rgb.hsl(cmyk.rgb(args)); | ||
}, | ||
hsv: function(args) { | ||
return rgb.hsv(cmyk.rgb(args)); | ||
}, | ||
hwb: function(args) { | ||
return rgb.hwb(cmyk.rgb(args)); | ||
}, | ||
xyz: function(arg) { | ||
return rgb.xyz(cmyk.rgb(arg)); | ||
}, | ||
lab: function(arg) { | ||
return rgb.lab(cmyk.rgb(arg)); | ||
}, | ||
lch: function(arg) { | ||
return rgb.lch(cmyk.rgb(arg)); | ||
}, | ||
luv: function(arg) { | ||
return rgb.luv(cmyk.rgb(arg)); | ||
}, | ||
min: [0,0,0,0], | ||
max: [100,100,100,100], | ||
channel: ['cyan', 'magenta', 'yellow', 'black'] | ||
}; | ||
var xyz = { | ||
//TODO: fix this maths so to return 255,255,255 in rgb | ||
rgb: function(xyz) { | ||
var x = xyz[0] / 100, | ||
y = xyz[1] / 100, | ||
z = xyz[2] / 100, | ||
r, g, b; | ||
// assume sRGB | ||
// http://www.brucelindbloom.com/index.html?Eqn_RGB_XYZ_Matrix.html | ||
r = (x * 3.2404542) + (y * -1.5371385) + (z * -0.4985314); | ||
g = (x * -0.9692660) + (y * 1.8760108) + (z * 0.0415560); | ||
b = (x * 0.0556434) + (y * -0.2040259) + (z * 1.0572252); | ||
r = r > 0.0031308 ? ((1.055 * Math.pow(r, 1.0 / 2.4)) - 0.055) | ||
: r = (r * 12.92); | ||
g = g > 0.0031308 ? ((1.055 * Math.pow(g, 1.0 / 2.4)) - 0.055) | ||
: g = (g * 12.92); | ||
b = b > 0.0031308 ? ((1.055 * Math.pow(b, 1.0 / 2.4)) - 0.055) | ||
: b = (b * 12.92); | ||
r = Math.min(Math.max(0, r), 1); | ||
g = Math.min(Math.max(0, g), 1); | ||
b = Math.min(Math.max(0, b), 1); | ||
return [r * 255, g * 255, b * 255]; | ||
}, | ||
hsl: function(arg) { | ||
return rgb.hsl(xyz.rgb(arg)); | ||
}, | ||
hsv: function(arg) { | ||
return rgb.hsv(xyz.rgb(arg)); | ||
}, | ||
hwb: function(arg) { | ||
return rgb.hwb(xyz.rgb(arg)); | ||
}, | ||
cmyk: function(arg) { | ||
return rgb.cmyk(xyz.rgb(arg)); | ||
}, | ||
lab: function(xyz) { | ||
var x = xyz[0], | ||
y = xyz[1], | ||
z = xyz[2], | ||
l, a, b; | ||
x /= 95.047; | ||
y /= 100; | ||
z /= 108.883; | ||
x = x > 0.008856 ? Math.pow(x, 1/3) : (7.787 * x) + (16 / 116); | ||
y = y > 0.008856 ? Math.pow(y, 1/3) : (7.787 * y) + (16 / 116); | ||
z = z > 0.008856 ? Math.pow(z, 1/3) : (7.787 * z) + (16 / 116); | ||
l = (116 * y) - 16; | ||
a = 500 * (x - y); | ||
b = 200 * (y - z); | ||
return [l, a, b]; | ||
}, | ||
lch: function(args) { | ||
return lab.lch(xyz.lab(args)); | ||
}, | ||
//http://www.brucelindbloom.com/index.html?Equations.html | ||
luv: function(arg, i) { | ||
var _u, _v, l, u, v, x, y, z, yn, un, vn; | ||
//get constants | ||
var e = 0.008856451679035631; //(6/29)^3 | ||
var k = 903.2962962962961; //(29/3)^3 | ||
//get illuminant | ||
i = i || options.illuminant; | ||
yn = xyz.illuminant[i][1]; | ||
un = luv.illuminant[i][1]/100; | ||
vn = luv.illuminant[i][2]/100; | ||
x = arg[0]/100, y = arg[1]/100, z = arg[2]/100; | ||
_u = (4 * x) / (x + (15 * y) + (3 * z)); | ||
_v = (9 * y) / (x + (15 * y) + (3 * z)); | ||
var yr = y/yn; | ||
l = yr <= e ? k * yr : 116 * Math.pow(yr, .333333333) - 16; | ||
u = 13 * l * (_u - un); | ||
v = 13 * l * (_v - vn); | ||
return [l, u, v]; | ||
}, | ||
//TODO | ||
cam: function(xyz){ | ||
var x = xyz[0], y = xyz[1], z = xyz[2]; | ||
//Mcat02 | ||
var m =[[0.7328, 0.4296, -0.1624], [-0.7036, 1.6975, 0.0061], [0.0030, 0.0136, 0.9834]]; | ||
//get lms | ||
var L = x*m[0][0] + y*m[0][1] + z*m[0][2]; | ||
var M = x*m[1][0] + y*m[1][1] + z*m[1][2]; | ||
var S = x*m[2][0] + y*m[2][1] + z*m[2][2]; | ||
//calc lc, mc, sc | ||
//FIXME: choose proper d | ||
var d = 0.85; | ||
var Lwr = 100, Mwr = 100, Swr = 100; | ||
var Lc = (Lwr*D/Lw + 1 - D) * L; | ||
var Mc = (Mwr*D/Mw + 1 - D) * M; | ||
var Sc = (Swr*D/Sw + 1 - D) * S; | ||
}, | ||
min: [0,0,0], | ||
max: [96,100,109], | ||
channel: ['lightness','u','v'], | ||
alias: ['ciexyz'], | ||
//Xn, Yn, Zn | ||
illuminant: { | ||
A:[109.85, 100, 35.58], | ||
C: [98.07, 100, 118.23], | ||
E: [100,100,100], | ||
D65: [95.04, 100, 108.88] | ||
} | ||
}; | ||
var lab = { | ||
xyz: function(lab) { | ||
var l = lab[0], | ||
a = lab[1], | ||
b = lab[2], | ||
x, y, z, y2; | ||
if (l <= 8) { | ||
y = (l * 100) / 903.3; | ||
y2 = (7.787 * (y / 100)) + (16 / 116); | ||
} else { | ||
y = 100 * Math.pow((l + 16) / 116, 3); | ||
y2 = Math.pow(y / 100, 1/3); | ||
} | ||
x = x / 95.047 <= 0.008856 ? x = (95.047 * ((a / 500) + y2 - (16 / 116))) / 7.787 : 95.047 * Math.pow((a / 500) + y2, 3); | ||
z = z / 108.883 <= 0.008859 ? z = (108.883 * (y2 - (b / 200) - (16 / 116))) / 7.787 : 108.883 * Math.pow(y2 - (b / 200), 3); | ||
return [x, y, z]; | ||
}, | ||
lch: function(lab) { | ||
var l = lab[0], | ||
a = lab[1], | ||
b = lab[2], | ||
hr, h, c; | ||
hr = Math.atan2(b, a); | ||
h = hr * 360 / 2 / Math.PI; | ||
if (h < 0) { | ||
h += 360; | ||
} | ||
c = Math.sqrt(a * a + b * b); | ||
return [l, c, h]; | ||
}, | ||
luv: function(arg) { | ||
}, | ||
rgb: function(args) { | ||
return xyz.rgb(lab.xyz(args)); | ||
}, | ||
hsl: function(arg) { | ||
return rgb.hsl(lab.rgb(arg)); | ||
}, | ||
hsv: function(arg) { | ||
return rgb.hsv(lab.rgb(arg)); | ||
}, | ||
hwb: function(arg) { | ||
return rgb.hwb(lab.rgb(arg)); | ||
}, | ||
cmyk: function(arg) { | ||
return rgb.cmyk(lab.rgb(arg)); | ||
}, | ||
min: [0,-100,-100], | ||
max: [100,100,100], | ||
channel: ['lightness', 'a', 'b'], | ||
alias: ['cielab'] | ||
}; | ||
//cylindrical lab | ||
var lch = { | ||
lab: function(lch) { | ||
var l = lch[0], | ||
c = lch[1], | ||
h = lch[2], | ||
a, b, hr; | ||
hr = h / 360 * 2 * Math.PI; | ||
a = c * Math.cos(hr); | ||
b = c * Math.sin(hr); | ||
return [l, a, b]; | ||
}, | ||
xyz: function(args) { | ||
return lab.xyz(lch.lab(args)); | ||
}, | ||
rgb: function(args) { | ||
return lab.rgb(lch.lab(args)); | ||
}, | ||
hsl: function(arg) { | ||
return rgb.hsl(lch.rgb(arg)); | ||
}, | ||
hsv: function(arg) { | ||
return rgb.hsv(lch.rgb(arg)); | ||
}, | ||
hwb: function(arg) { | ||
return rgb.hwb(lch.rgb(arg)); | ||
}, | ||
cmyk: function(arg) { | ||
return rgb.cmyk(lch.rgb(arg)); | ||
}, | ||
luv: function(){ | ||
}, | ||
min: [0,0,0], | ||
max: [100,100,360], | ||
channel: ['lightness', 'chroma', 'hue'], | ||
alias: ['cielch', 'lchab'] | ||
}; | ||
//TODO | ||
var luv = { | ||
xyz: function(luv){ | ||
}, | ||
lchuv: function(luv){ | ||
var C = Math.sqrt(); | ||
}, | ||
min: [0,-100,-100], | ||
max: [100,100,100], | ||
channel: ['lightness', 'u', 'v'], | ||
alias: ['cieluv'], | ||
//Yn, un,vn: http://www.optique-ingenieur.org/en/courses/OPI_ang_M07_C02/co/Contenu_08.html | ||
illuminant: { | ||
A:[100, 255.97, 524.29], | ||
C: [100, 200.89, 460.89], | ||
E: [100,100,100], | ||
D65: [100, 197, 468.34] | ||
} | ||
}; | ||
//TODO | ||
//cylindrical luv | ||
var lchuv = { | ||
luv: function(){ | ||
}, | ||
alias: ['cielchuv'] | ||
}; | ||
//TODO | ||
var xyy = { | ||
alias: ['ciexyy'] | ||
}; | ||
//CIECAM02 http://en.wikipedia.org/wiki/CIECAM02 | ||
var cam = { | ||
alias: ['ciecam'] | ||
}; | ||
//@link http://www.boronine.com/husl/ | ||
//TODO | ||
var husl = { | ||
}; | ||
//TODO | ||
var huslp = { | ||
}; | ||
/** | ||
@@ -773,13 +7,12 @@ * @module color-space | ||
module.exports = { | ||
options: options, | ||
rgb: rgb, | ||
hsl: hsl, | ||
hsv: hsv, | ||
hwb: hwb, | ||
cmyk: cmyk, | ||
xyz: xyz, | ||
lab: lab, | ||
lch: lch, | ||
luv: luv, | ||
rgb: require('./rgb'), | ||
hsl: require('./hsl'), | ||
hsv: require('./hsv'), | ||
hwb: require('./hwb'), | ||
cmyk: require('./cmyk'), | ||
xyz: require('./xyz'), | ||
lab: require('./lab'), | ||
lch: require('./lch'), | ||
luv: require('./luv') | ||
// lchuv: lchuv | ||
}; |
{ | ||
"name": "color-space", | ||
"description": "Math & data behind color spaces and color conversions.", | ||
"version": "0.1.1", | ||
"version": "0.2.1", | ||
"author": "Deema Ywanow <dfcreative@gmail.com>", | ||
@@ -6,0 +6,0 @@ "repository": { |
# color-space [](https://travis-ci.org/dfcreative/color-space) | ||
Math and data behind color spaces and color conversions. [Converter & tests](https://rawgit.com/dfcreative/color-space/master/test/index.html). | ||
Math and data behind color spaces and color conversions. [Converter & tests](https://cdn.rawgit.com/dfcreative/color-space/master/test/index.html). | ||
@@ -14,9 +14,24 @@ [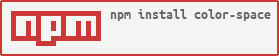](https://nodei.co/npm/color-space/) | ||
To include all spaces at once: | ||
```js | ||
var convert = require('color-space'); | ||
var spaces = require('color-space'); | ||
//convert lab to lch | ||
spaces.lab.lch([80,50,60]); | ||
``` | ||
To include one target space: | ||
```js | ||
var rgb = require('color-space/rgb'); | ||
var hsl = require('color-space/hsl'); | ||
//convert rgb to lch | ||
convert.rgb.lch([200,230,100]); | ||
hsl.lch([200,230,100]); | ||
``` | ||
## API | ||
@@ -29,3 +44,3 @@ | ||
convert[fromSpace][toSpace](array); | ||
spaces[fromSpace][toSpace](array); | ||
``` | ||
@@ -36,6 +51,8 @@ | ||
```js | ||
convert[space].min //channel minimums | ||
convert[space].max //channel maximums | ||
convert[space].channel //channel names | ||
convert[space].alias //alias space names, if any | ||
space.name //space name | ||
space.min //channel minimums | ||
space.max //channel maximums | ||
space.channel //channel names | ||
space.alias //alias space names, if any | ||
space.illuminant //space-specific properties | ||
``` | ||
@@ -56,3 +73,3 @@ | ||
To see details `console.log(convert)`. | ||
To see details `console.log(space)`. | ||
@@ -59,0 +76,0 @@ |
require=(function e(t,n,r){function s(o,u){if(!n[o]){if(!t[o]){var a=typeof require=="function"&&require;if(!u&&a)return a(o,!0);if(i)return i(o,!0);var f=new Error("Cannot find module '"+o+"'");throw f.code="MODULE_NOT_FOUND",f}var l=n[o]={exports:{}};t[o][0].call(l.exports,function(e){var n=t[o][1][e];return s(n?n:e)},l,l.exports,e,t,n,r)}return n[o].exports}var i=typeof require=="function"&&require;for(var o=0;o<r.length;o++)s(r[o]);return s})({"../index":[function(require,module,exports){ | ||
//TODO: save hue on setting sat = 0; | ||
/** | ||
* @module color-space | ||
*/ | ||
module.exports = { | ||
rgb: require('./rgb'), | ||
hsl: require('./hsl'), | ||
hsv: require('./hsv'), | ||
hwb: require('./hwb'), | ||
cmyk: require('./cmyk'), | ||
xyz: require('./xyz'), | ||
lab: require('./lab'), | ||
lch: require('./lch'), | ||
luv: require('./luv') | ||
// lchuv: lchuv | ||
}; | ||
},{"./cmyk":1,"./hsl":2,"./hsv":3,"./hwb":4,"./lab":5,"./lch":6,"./luv":7,"./rgb":14,"./xyz":15}],"assert":[function(require,module,exports){ | ||
// http://wiki.commonjs.org/wiki/Unit_Testing/1.0 | ||
// | ||
// THIS IS NOT TESTED NOR LIKELY TO WORK OUTSIDE V8! | ||
// | ||
// Originally from narwhal.js (http://narwhaljs.org) | ||
// Copyright (c) 2009 Thomas Robinson <280north.com> | ||
// | ||
// Permission is hereby granted, free of charge, to any person obtaining a copy | ||
// of this software and associated documentation files (the 'Software'), to | ||
// deal in the Software without restriction, including without limitation the | ||
// rights to use, copy, modify, merge, publish, distribute, sublicense, and/or | ||
// sell copies of the Software, and to permit persons to whom the Software is | ||
// furnished to do so, subject to the following conditions: | ||
// | ||
// The above copyright notice and this permission notice shall be included in | ||
// all copies or substantial portions of the Software. | ||
// | ||
// THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | ||
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, | ||
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE | ||
// AUTHORS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN | ||
// ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION | ||
// WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. | ||
var options = { | ||
illuminant: 'D65', | ||
observer: '2' | ||
// when used in node, this will actually load the util module we depend on | ||
// versus loading the builtin util module as happens otherwise | ||
// this is a bug in node module loading as far as I am concerned | ||
var util = require('util/'); | ||
var pSlice = Array.prototype.slice; | ||
var hasOwn = Object.prototype.hasOwnProperty; | ||
// 1. The assert module provides functions that throw | ||
// AssertionError's when particular conditions are not met. The | ||
// assert module must conform to the following interface. | ||
var assert = module.exports = ok; | ||
// 2. The AssertionError is defined in assert. | ||
// new assert.AssertionError({ message: message, | ||
// actual: actual, | ||
// expected: expected }) | ||
assert.AssertionError = function AssertionError(options) { | ||
this.name = 'AssertionError'; | ||
this.actual = options.actual; | ||
this.expected = options.expected; | ||
this.operator = options.operator; | ||
if (options.message) { | ||
this.message = options.message; | ||
this.generatedMessage = false; | ||
} else { | ||
this.message = getMessage(this); | ||
this.generatedMessage = true; | ||
} | ||
var stackStartFunction = options.stackStartFunction || fail; | ||
if (Error.captureStackTrace) { | ||
Error.captureStackTrace(this, stackStartFunction); | ||
} | ||
else { | ||
// non v8 browsers so we can have a stacktrace | ||
var err = new Error(); | ||
if (err.stack) { | ||
var out = err.stack; | ||
// try to strip useless frames | ||
var fn_name = stackStartFunction.name; | ||
var idx = out.indexOf('\n' + fn_name); | ||
if (idx >= 0) { | ||
// once we have located the function frame | ||
// we need to strip out everything before it (and its line) | ||
var next_line = out.indexOf('\n', idx + 1); | ||
out = out.substring(next_line + 1); | ||
} | ||
this.stack = out; | ||
} | ||
} | ||
}; | ||
// assert.AssertionError instanceof Error | ||
util.inherits(assert.AssertionError, Error); | ||
var rgb = { | ||
hsl: function(rgb) { | ||
var r = rgb[0]/255, | ||
g = rgb[1]/255, | ||
b = rgb[2]/255, | ||
min = Math.min(r, g, b), | ||
max = Math.max(r, g, b), | ||
delta = max - min, | ||
h, s, l; | ||
function replacer(key, value) { | ||
if (util.isUndefined(value)) { | ||
return '' + value; | ||
} | ||
if (util.isNumber(value) && (isNaN(value) || !isFinite(value))) { | ||
return value.toString(); | ||
} | ||
if (util.isFunction(value) || util.isRegExp(value)) { | ||
return value.toString(); | ||
} | ||
return value; | ||
} | ||
if (max == min) | ||
h = 0; | ||
else if (r == max) | ||
h = (g - b) / delta; | ||
else if (g == max) | ||
h = 2 + (b - r) / delta; | ||
else if (b == max) | ||
h = 4 + (r - g)/ delta; | ||
function truncate(s, n) { | ||
if (util.isString(s)) { | ||
return s.length < n ? s : s.slice(0, n); | ||
} else { | ||
return s; | ||
} | ||
} | ||
h = Math.min(h * 60, 360); | ||
function getMessage(self) { | ||
return truncate(JSON.stringify(self.actual, replacer), 128) + ' ' + | ||
self.operator + ' ' + | ||
truncate(JSON.stringify(self.expected, replacer), 128); | ||
} | ||
if (h < 0) | ||
h += 360; | ||
// At present only the three keys mentioned above are used and | ||
// understood by the spec. Implementations or sub modules can pass | ||
// other keys to the AssertionError's constructor - they will be | ||
// ignored. | ||
l = (min + max) / 2; | ||
// 3. All of the following functions must throw an AssertionError | ||
// when a corresponding condition is not met, with a message that | ||
// may be undefined if not provided. All assertion methods provide | ||
// both the actual and expected values to the assertion error for | ||
// display purposes. | ||
if (max == min) | ||
s = 0; | ||
else if (l <= 0.5) | ||
s = delta / (max + min); | ||
else | ||
s = delta / (2 - max - min); | ||
function fail(actual, expected, message, operator, stackStartFunction) { | ||
throw new assert.AssertionError({ | ||
message: message, | ||
actual: actual, | ||
expected: expected, | ||
operator: operator, | ||
stackStartFunction: stackStartFunction | ||
}); | ||
} | ||
return [h, s * 100, l * 100]; | ||
}, | ||
// EXTENSION! allows for well behaved errors defined elsewhere. | ||
assert.fail = fail; | ||
hsv: function(rgb) { | ||
var r = rgb[0], | ||
g = rgb[1], | ||
b = rgb[2], | ||
min = Math.min(r, g, b), | ||
max = Math.max(r, g, b), | ||
delta = max - min, | ||
h, s, v; | ||
// 4. Pure assertion tests whether a value is truthy, as determined | ||
// by !!guard. | ||
// assert.ok(guard, message_opt); | ||
// This statement is equivalent to assert.equal(true, !!guard, | ||
// message_opt);. To test strictly for the value true, use | ||
// assert.strictEqual(true, guard, message_opt);. | ||
if (max == 0) | ||
s = 0; | ||
else | ||
s = (delta/max * 1000)/10; | ||
function ok(value, message) { | ||
if (!value) fail(value, true, message, '==', assert.ok); | ||
} | ||
assert.ok = ok; | ||
if (max == min) | ||
h = 0; | ||
else if (r == max) | ||
h = (g - b) / delta; | ||
else if (g == max) | ||
h = 2 + (b - r) / delta; | ||
else if (b == max) | ||
h = 4 + (r - g) / delta; | ||
// 5. The equality assertion tests shallow, coercive equality with | ||
// ==. | ||
// assert.equal(actual, expected, message_opt); | ||
h = Math.min(h * 60, 360); | ||
assert.equal = function equal(actual, expected, message) { | ||
if (actual != expected) fail(actual, expected, message, '==', assert.equal); | ||
}; | ||
if (h < 0) | ||
h += 360; | ||
// 6. The non-equality assertion tests for whether two objects are not equal | ||
// with != assert.notEqual(actual, expected, message_opt); | ||
v = ((max / 255) * 1000) / 10; | ||
assert.notEqual = function notEqual(actual, expected, message) { | ||
if (actual == expected) { | ||
fail(actual, expected, message, '!=', assert.notEqual); | ||
} | ||
}; | ||
return [h, s, v]; | ||
}, | ||
// 7. The equivalence assertion tests a deep equality relation. | ||
// assert.deepEqual(actual, expected, message_opt); | ||
hwb: function(val) { | ||
var r = val[0], | ||
g = val[1], | ||
b = val[2], | ||
h = rgb.hsl(val)[0], | ||
w = 1/255 * Math.min(r, Math.min(g, b)), | ||
b = 1 - 1/255 * Math.max(r, Math.max(g, b)); | ||
assert.deepEqual = function deepEqual(actual, expected, message) { | ||
if (!_deepEqual(actual, expected)) { | ||
fail(actual, expected, message, 'deepEqual', assert.deepEqual); | ||
} | ||
}; | ||
return [h, w * 100, b * 100]; | ||
}, | ||
function _deepEqual(actual, expected) { | ||
// 7.1. All identical values are equivalent, as determined by ===. | ||
if (actual === expected) { | ||
return true; | ||
cmyk: function(rgb) { | ||
var r = rgb[0] / 255, | ||
g = rgb[1] / 255, | ||
b = rgb[2] / 255, | ||
c, m, y, k; | ||
} else if (util.isBuffer(actual) && util.isBuffer(expected)) { | ||
if (actual.length != expected.length) return false; | ||
k = Math.min(1 - r, 1 - g, 1 - b); | ||
c = (1 - r - k) / (1 - k) || 0; | ||
m = (1 - g - k) / (1 - k) || 0; | ||
y = (1 - b - k) / (1 - k) || 0; | ||
return [c * 100, m * 100, y * 100, k * 100]; | ||
}, | ||
for (var i = 0; i < actual.length; i++) { | ||
if (actual[i] !== expected[i]) return false; | ||
} | ||
xyz: function(rgb) { | ||
var r = rgb[0] / 255, | ||
g = rgb[1] / 255, | ||
b = rgb[2] / 255; | ||
return true; | ||
// assume sRGB | ||
r = r > 0.04045 ? Math.pow(((r + 0.055) / 1.055), 2.4) : (r / 12.92); | ||
g = g > 0.04045 ? Math.pow(((g + 0.055) / 1.055), 2.4) : (g / 12.92); | ||
b = b > 0.04045 ? Math.pow(((b + 0.055) / 1.055), 2.4) : (b / 12.92); | ||
// 7.2. If the expected value is a Date object, the actual value is | ||
// equivalent if it is also a Date object that refers to the same time. | ||
} else if (util.isDate(actual) && util.isDate(expected)) { | ||
return actual.getTime() === expected.getTime(); | ||
var x = (r * 0.4124) + (g * 0.3576) + (b * 0.1805); | ||
var y = (r * 0.2126) + (g * 0.7152) + (b * 0.0722); | ||
var z = (r * 0.0193) + (g * 0.1192) + (b * 0.9505); | ||
// 7.3 If the expected value is a RegExp object, the actual value is | ||
// equivalent if it is also a RegExp object with the same source and | ||
// properties (`global`, `multiline`, `lastIndex`, `ignoreCase`). | ||
} else if (util.isRegExp(actual) && util.isRegExp(expected)) { | ||
return actual.source === expected.source && | ||
actual.global === expected.global && | ||
actual.multiline === expected.multiline && | ||
actual.lastIndex === expected.lastIndex && | ||
actual.ignoreCase === expected.ignoreCase; | ||
return [x * 100, y *100, z * 100]; | ||
}, | ||
// 7.4. Other pairs that do not both pass typeof value == 'object', | ||
// equivalence is determined by ==. | ||
} else if (!util.isObject(actual) && !util.isObject(expected)) { | ||
return actual == expected; | ||
lab: function(args) { | ||
var xyz = rgb.xyz(args), | ||
x = xyz[0], | ||
y = xyz[1], | ||
z = xyz[2], | ||
l, a, b; | ||
// 7.5 For all other Object pairs, including Array objects, equivalence is | ||
// determined by having the same number of owned properties (as verified | ||
// with Object.prototype.hasOwnProperty.call), the same set of keys | ||
// (although not necessarily the same order), equivalent values for every | ||
// corresponding key, and an identical 'prototype' property. Note: this | ||
// accounts for both named and indexed properties on Arrays. | ||
} else { | ||
return objEquiv(actual, expected); | ||
} | ||
} | ||
x /= 95.047; | ||
y /= 100; | ||
z /= 108.883; | ||
function isArguments(object) { | ||
return Object.prototype.toString.call(object) == '[object Arguments]'; | ||
} | ||
x = x > 0.008856 ? Math.pow(x, 1/3) : (7.787 * x) + (16 / 116); | ||
y = y > 0.008856 ? Math.pow(y, 1/3) : (7.787 * y) + (16 / 116); | ||
z = z > 0.008856 ? Math.pow(z, 1/3) : (7.787 * z) + (16 / 116); | ||
function objEquiv(a, b) { | ||
if (util.isNullOrUndefined(a) || util.isNullOrUndefined(b)) | ||
return false; | ||
// an identical 'prototype' property. | ||
if (a.prototype !== b.prototype) return false; | ||
//~~~I've managed to break Object.keys through screwy arguments passing. | ||
// Converting to array solves the problem. | ||
if (isArguments(a)) { | ||
if (!isArguments(b)) { | ||
return false; | ||
} | ||
a = pSlice.call(a); | ||
b = pSlice.call(b); | ||
return _deepEqual(a, b); | ||
} | ||
try { | ||
var ka = objectKeys(a), | ||
kb = objectKeys(b), | ||
key, i; | ||
} catch (e) {//happens when one is a string literal and the other isn't | ||
return false; | ||
} | ||
// having the same number of owned properties (keys incorporates | ||
// hasOwnProperty) | ||
if (ka.length != kb.length) | ||
return false; | ||
//the same set of keys (although not necessarily the same order), | ||
ka.sort(); | ||
kb.sort(); | ||
//~~~cheap key test | ||
for (i = ka.length - 1; i >= 0; i--) { | ||
if (ka[i] != kb[i]) | ||
return false; | ||
} | ||
//equivalent values for every corresponding key, and | ||
//~~~possibly expensive deep test | ||
for (i = ka.length - 1; i >= 0; i--) { | ||
key = ka[i]; | ||
if (!_deepEqual(a[key], b[key])) return false; | ||
} | ||
return true; | ||
} | ||
l = (116 * y) - 16; | ||
a = 500 * (x - y); | ||
b = 200 * (y - z); | ||
// 8. The non-equivalence assertion tests for any deep inequality. | ||
// assert.notDeepEqual(actual, expected, message_opt); | ||
return [l, a, b]; | ||
assert.notDeepEqual = function notDeepEqual(actual, expected, message) { | ||
if (_deepEqual(actual, expected)) { | ||
fail(actual, expected, message, 'notDeepEqual', assert.notDeepEqual); | ||
} | ||
}; | ||
// 9. The strict equality assertion tests strict equality, as determined by ===. | ||
// assert.strictEqual(actual, expected, message_opt); | ||
assert.strictEqual = function strictEqual(actual, expected, message) { | ||
if (actual !== expected) { | ||
fail(actual, expected, message, '===', assert.strictEqual); | ||
} | ||
}; | ||
// 10. The strict non-equality assertion tests for strict inequality, as | ||
// determined by !==. assert.notStrictEqual(actual, expected, message_opt); | ||
assert.notStrictEqual = function notStrictEqual(actual, expected, message) { | ||
if (actual === expected) { | ||
fail(actual, expected, message, '!==', assert.notStrictEqual); | ||
} | ||
}; | ||
function expectedException(actual, expected) { | ||
if (!actual || !expected) { | ||
return false; | ||
} | ||
if (Object.prototype.toString.call(expected) == '[object RegExp]') { | ||
return expected.test(actual); | ||
} else if (actual instanceof expected) { | ||
return true; | ||
} else if (expected.call({}, actual) === true) { | ||
return true; | ||
} | ||
return false; | ||
} | ||
function _throws(shouldThrow, block, expected, message) { | ||
var actual; | ||
if (util.isString(expected)) { | ||
message = expected; | ||
expected = null; | ||
} | ||
try { | ||
block(); | ||
} catch (e) { | ||
actual = e; | ||
} | ||
message = (expected && expected.name ? ' (' + expected.name + ').' : '.') + | ||
(message ? ' ' + message : '.'); | ||
if (shouldThrow && !actual) { | ||
fail(actual, expected, 'Missing expected exception' + message); | ||
} | ||
if (!shouldThrow && expectedException(actual, expected)) { | ||
fail(actual, expected, 'Got unwanted exception' + message); | ||
} | ||
if ((shouldThrow && actual && expected && | ||
!expectedException(actual, expected)) || (!shouldThrow && actual)) { | ||
throw actual; | ||
} | ||
} | ||
// 11. Expected to throw an error: | ||
// assert.throws(block, Error_opt, message_opt); | ||
assert.throws = function(block, /*optional*/error, /*optional*/message) { | ||
_throws.apply(this, [true].concat(pSlice.call(arguments))); | ||
}; | ||
// EXTENSION! This is annoying to write outside this module. | ||
assert.doesNotThrow = function(block, /*optional*/message) { | ||
_throws.apply(this, [false].concat(pSlice.call(arguments))); | ||
}; | ||
assert.ifError = function(err) { if (err) {throw err;}}; | ||
var objectKeys = Object.keys || function (obj) { | ||
var keys = []; | ||
for (var key in obj) { | ||
if (hasOwn.call(obj, key)) keys.push(key); | ||
} | ||
return keys; | ||
}; | ||
},{"util/":11}],1:[function(require,module,exports){ | ||
var rgb = require('./rgb'); | ||
var cmyk = module.exports = { | ||
name: 'cmyk', | ||
min: [0,0,0,0], | ||
max: [100,100,100,100], | ||
channel: ['cyan', 'magenta', 'yellow', 'black'], | ||
rgb: function(cmyk) { | ||
var c = cmyk[0] / 100, | ||
m = cmyk[1] / 100, | ||
y = cmyk[2] / 100, | ||
k = cmyk[3] / 100, | ||
r, g, b; | ||
r = 1 - Math.min(1, c * (1 - k) + k); | ||
g = 1 - Math.min(1, m * (1 - k) + k); | ||
b = 1 - Math.min(1, y * (1 - k) + k); | ||
return [r * 255, g * 255, b * 255]; | ||
}, | ||
lch: function(args) { | ||
return lab.lch(rgb.lab(args)); | ||
hsl: function(args) { | ||
return rgb.hsl(cmyk.rgb(args)); | ||
}, | ||
luv: function(args){ | ||
return xyz.luv(rgb.xyz(args)); | ||
hsv: function(args) { | ||
return rgb.hsv(cmyk.rgb(args)); | ||
}, | ||
lchuv: function(args){ | ||
return luv.lchuv(rgb.luv(args)); | ||
hwb: function(args) { | ||
return rgb.hwb(cmyk.rgb(args)); | ||
}, | ||
min: [0,0,0], | ||
max: [255,255,255], | ||
channel: ['red', 'green', 'blue'] | ||
xyz: function(arg) { | ||
return rgb.xyz(cmyk.rgb(arg)); | ||
}, | ||
lab: function(arg) { | ||
return rgb.lab(cmyk.rgb(arg)); | ||
}, | ||
lch: function(arg) { | ||
return rgb.lch(cmyk.rgb(arg)); | ||
}, | ||
luv: function(arg) { | ||
return rgb.luv(cmyk.rgb(arg)); | ||
} | ||
}; | ||
},{"./rgb":14}],2:[function(require,module,exports){ | ||
var rgb = require('./rgb'); | ||
var hsl = module.exports = { | ||
name: 'hsl', | ||
min: [0,0,0], | ||
max: [360,100,100], | ||
channel: ['hue', 'saturation', 'lightness'], | ||
var hsl = { | ||
rgb: function(hsl) { | ||
@@ -235,12 +514,14 @@ var h = hsl[0] / 360, | ||
return rgb.luv(hsl.rgb(arg)); | ||
}, | ||
} | ||
}; | ||
},{"./rgb":14}],3:[function(require,module,exports){ | ||
var rgb = require('./rgb'); | ||
var hsv = module.exports = { | ||
name: 'hsv', | ||
min: [0,0,0], | ||
max: [360,100,100], | ||
channel: ['hue', 'saturation', 'lightness'] | ||
}; | ||
channel: ['hue', 'saturation', 'value'], | ||
alias: ['hsb'], | ||
var hsv = { | ||
rgb: function(hsv) { | ||
@@ -312,14 +593,13 @@ var h = hsv[0] / 60, | ||
return rgb.luv(hsv.rgb(arg)); | ||
}, | ||
} | ||
}; | ||
},{"./rgb":14}],4:[function(require,module,exports){ | ||
var rgb = require('./rgb'); | ||
var hwb = module.exports = { | ||
name: 'hwb', | ||
min: [0,0,0], | ||
max: [360,100,100], | ||
channel: ['hue', 'saturation', 'value'], | ||
alias: ['hsb'] | ||
}; | ||
channel: ['hue', 'whiteness', 'blackness'], | ||
var hwb = { | ||
// http://dev.w3.org/csswg/css-color/#hwb-to-rgb | ||
@@ -390,199 +670,16 @@ rgb: function(hwb) { | ||
return rgb.luv(hwb.rgb(arg)); | ||
}, | ||
min: [0,0,0], | ||
max: [360,100,100], | ||
channel: ['hue', 'whiteness', 'blackness'] | ||
} | ||
}; | ||
},{"./rgb":14}],5:[function(require,module,exports){ | ||
var rgb = require('./rgb'); | ||
var xyz = require('./xyz'); | ||
var cmyk = { | ||
rgb: function(cmyk) { | ||
var c = cmyk[0] / 100, | ||
m = cmyk[1] / 100, | ||
y = cmyk[2] / 100, | ||
k = cmyk[3] / 100, | ||
r, g, b; | ||
var lab = module.exports = { | ||
name: 'lab', | ||
min: [0,-100,-100], | ||
max: [100,100,100], | ||
channel: ['lightness', 'a', 'b'], | ||
alias: ['cielab'], | ||
r = 1 - Math.min(1, c * (1 - k) + k); | ||
g = 1 - Math.min(1, m * (1 - k) + k); | ||
b = 1 - Math.min(1, y * (1 - k) + k); | ||
return [r * 255, g * 255, b * 255]; | ||
}, | ||
hsl: function(args) { | ||
return rgb.hsl(cmyk.rgb(args)); | ||
}, | ||
hsv: function(args) { | ||
return rgb.hsv(cmyk.rgb(args)); | ||
}, | ||
hwb: function(args) { | ||
return rgb.hwb(cmyk.rgb(args)); | ||
}, | ||
xyz: function(arg) { | ||
return rgb.xyz(cmyk.rgb(arg)); | ||
}, | ||
lab: function(arg) { | ||
return rgb.lab(cmyk.rgb(arg)); | ||
}, | ||
lch: function(arg) { | ||
return rgb.lch(cmyk.rgb(arg)); | ||
}, | ||
luv: function(arg) { | ||
return rgb.luv(cmyk.rgb(arg)); | ||
}, | ||
min: [0,0,0,0], | ||
max: [100,100,100,100], | ||
channel: ['cyan', 'magenta', 'yellow', 'black'] | ||
}; | ||
var xyz = { | ||
//TODO: fix this maths so to return 255,255,255 in rgb | ||
rgb: function(xyz) { | ||
var x = xyz[0] / 100, | ||
y = xyz[1] / 100, | ||
z = xyz[2] / 100, | ||
r, g, b; | ||
// assume sRGB | ||
// http://www.brucelindbloom.com/index.html?Eqn_RGB_XYZ_Matrix.html | ||
r = (x * 3.2404542) + (y * -1.5371385) + (z * -0.4985314); | ||
g = (x * -0.9692660) + (y * 1.8760108) + (z * 0.0415560); | ||
b = (x * 0.0556434) + (y * -0.2040259) + (z * 1.0572252); | ||
r = r > 0.0031308 ? ((1.055 * Math.pow(r, 1.0 / 2.4)) - 0.055) | ||
: r = (r * 12.92); | ||
g = g > 0.0031308 ? ((1.055 * Math.pow(g, 1.0 / 2.4)) - 0.055) | ||
: g = (g * 12.92); | ||
b = b > 0.0031308 ? ((1.055 * Math.pow(b, 1.0 / 2.4)) - 0.055) | ||
: b = (b * 12.92); | ||
r = Math.min(Math.max(0, r), 1); | ||
g = Math.min(Math.max(0, g), 1); | ||
b = Math.min(Math.max(0, b), 1); | ||
return [r * 255, g * 255, b * 255]; | ||
}, | ||
hsl: function(arg) { | ||
return rgb.hsl(xyz.rgb(arg)); | ||
}, | ||
hsv: function(arg) { | ||
return rgb.hsv(xyz.rgb(arg)); | ||
}, | ||
hwb: function(arg) { | ||
return rgb.hwb(xyz.rgb(arg)); | ||
}, | ||
cmyk: function(arg) { | ||
return rgb.cmyk(xyz.rgb(arg)); | ||
}, | ||
lab: function(xyz) { | ||
var x = xyz[0], | ||
y = xyz[1], | ||
z = xyz[2], | ||
l, a, b; | ||
x /= 95.047; | ||
y /= 100; | ||
z /= 108.883; | ||
x = x > 0.008856 ? Math.pow(x, 1/3) : (7.787 * x) + (16 / 116); | ||
y = y > 0.008856 ? Math.pow(y, 1/3) : (7.787 * y) + (16 / 116); | ||
z = z > 0.008856 ? Math.pow(z, 1/3) : (7.787 * z) + (16 / 116); | ||
l = (116 * y) - 16; | ||
a = 500 * (x - y); | ||
b = 200 * (y - z); | ||
return [l, a, b]; | ||
}, | ||
lch: function(args) { | ||
return lab.lch(xyz.lab(args)); | ||
}, | ||
//http://www.brucelindbloom.com/index.html?Equations.html | ||
luv: function(arg, i) { | ||
var _u, _v, l, u, v, x, y, z, yn, un, vn; | ||
//get constants | ||
var e = 0.008856451679035631; //(6/29)^3 | ||
var k = 903.2962962962961; //(29/3)^3 | ||
//get illuminant | ||
i = i || options.illuminant; | ||
yn = xyz.illuminant[i][1]; | ||
un = luv.illuminant[i][1]/100; | ||
vn = luv.illuminant[i][2]/100; | ||
x = arg[0]/100, y = arg[1]/100, z = arg[2]/100; | ||
_u = (4 * x) / (x + (15 * y) + (3 * z)); | ||
_v = (9 * y) / (x + (15 * y) + (3 * z)); | ||
var yr = y/yn; | ||
l = yr <= e ? k * yr : 116 * Math.pow(yr, .333333333) - 16; | ||
u = 13 * l * (_u - un); | ||
v = 13 * l * (_v - vn); | ||
return [l, u, v]; | ||
}, | ||
//TODO | ||
cam: function(xyz){ | ||
var x = xyz[0], y = xyz[1], z = xyz[2]; | ||
//Mcat02 | ||
var m =[[0.7328, 0.4296, -0.1624], [-0.7036, 1.6975, 0.0061], [0.0030, 0.0136, 0.9834]]; | ||
//get lms | ||
var L = x*m[0][0] + y*m[0][1] + z*m[0][2]; | ||
var M = x*m[1][0] + y*m[1][1] + z*m[1][2]; | ||
var S = x*m[2][0] + y*m[2][1] + z*m[2][2]; | ||
//calc lc, mc, sc | ||
//FIXME: choose proper d | ||
var d = 0.85; | ||
var Lwr = 100, Mwr = 100, Swr = 100; | ||
var Lc = (Lwr*D/Lw + 1 - D) * L; | ||
var Mc = (Mwr*D/Mw + 1 - D) * M; | ||
var Sc = (Swr*D/Sw + 1 - D) * S; | ||
}, | ||
min: [0,0,0], | ||
max: [96,100,109], | ||
channel: ['lightness','u','v'], | ||
alias: ['ciexyz'], | ||
//Xn, Yn, Zn | ||
illuminant: { | ||
A:[109.85, 100, 35.58], | ||
C: [98.07, 100, 118.23], | ||
E: [100,100,100], | ||
D65: [95.04, 100, 108.88] | ||
} | ||
}; | ||
var lab = { | ||
xyz: function(lab) { | ||
@@ -646,14 +743,19 @@ var l = lab[0], | ||
return rgb.cmyk(lab.rgb(arg)); | ||
}, | ||
min: [0,-100,-100], | ||
max: [100,100,100], | ||
channel: ['lightness', 'a', 'b'], | ||
alias: ['cielab'] | ||
} | ||
}; | ||
// | ||
},{"./rgb":14,"./xyz":15}],6:[function(require,module,exports){ | ||
var rgb = require('./rgb'); | ||
var xyz = require('./xyz'); | ||
var lab = require('./lab'); | ||
//cylindrical lab | ||
var lch = { | ||
var lch = module.exports = { | ||
name: 'lch', | ||
min: [0,0,0], | ||
max: [100,100,360], | ||
channel: ['lightness', 'chroma', 'hue'], | ||
alias: ['cielch', 'lchab'], | ||
lab: function(lch) { | ||
@@ -698,22 +800,21 @@ var l = lch[0], | ||
}, | ||
min: [0,0,0], | ||
max: [100,100,360], | ||
channel: ['lightness', 'chroma', 'hue'], | ||
alias: ['cielch', 'lchab'] | ||
} | ||
}; | ||
//Extend rgb space | ||
rgb.lch = function(args) { | ||
return lab.lch(rgb.lab(args)); | ||
}; | ||
//TODO | ||
var luv = { | ||
xyz: function(luv){ | ||
xyz.lch = function(args) { | ||
return lab.lch(xyz.lab(args)); | ||
}; | ||
},{"./lab":5,"./rgb":14,"./xyz":15}],7:[function(require,module,exports){ | ||
var xyz = require('./xyz'); | ||
var rgb = require('./rgb'); | ||
}, | ||
var luv = module.exports = { | ||
name: 'luv', | ||
lchuv: function(luv){ | ||
var C = Math.sqrt(); | ||
}, | ||
min: [0,-100,-100], | ||
@@ -724,3 +825,2 @@ max: [100,100,100], | ||
//Yn, un,vn: http://www.optique-ingenieur.org/en/courses/OPI_ang_M07_C02/co/Contenu_08.html | ||
@@ -732,425 +832,49 @@ illuminant: { | ||
D65: [100, 197, 468.34] | ||
} | ||
}; | ||
}, | ||
xyz: function(luv){ | ||
//TODO | ||
//cylindrical luv | ||
var lchuv = { | ||
luv: function(){ | ||
}, | ||
alias: ['cielchuv'] | ||
}; | ||
//TODO | ||
var xyy = { | ||
alias: ['ciexyy'] | ||
}; | ||
//CIECAM02 http://en.wikipedia.org/wiki/CIECAM02 | ||
var cam = { | ||
alias: ['ciecam'] | ||
}; | ||
//@link http://www.boronine.com/husl/ | ||
//TODO | ||
var husl = { | ||
}; | ||
//TODO | ||
var huslp = { | ||
}; | ||
/** | ||
* @module color-space | ||
*/ | ||
module.exports = { | ||
options: options, | ||
rgb: rgb, | ||
hsl: hsl, | ||
hsv: hsv, | ||
hwb: hwb, | ||
cmyk: cmyk, | ||
xyz: xyz, | ||
lab: lab, | ||
lch: lch, | ||
luv: luv, | ||
// lchuv: lchuv | ||
}; | ||
},{}],"assert":[function(require,module,exports){ | ||
// http://wiki.commonjs.org/wiki/Unit_Testing/1.0 | ||
// | ||
// THIS IS NOT TESTED NOR LIKELY TO WORK OUTSIDE V8! | ||
// | ||
// Originally from narwhal.js (http://narwhaljs.org) | ||
// Copyright (c) 2009 Thomas Robinson <280north.com> | ||
// | ||
// Permission is hereby granted, free of charge, to any person obtaining a copy | ||
// of this software and associated documentation files (the 'Software'), to | ||
// deal in the Software without restriction, including without limitation the | ||
// rights to use, copy, modify, merge, publish, distribute, sublicense, and/or | ||
// sell copies of the Software, and to permit persons to whom the Software is | ||
// furnished to do so, subject to the following conditions: | ||
// | ||
// The above copyright notice and this permission notice shall be included in | ||
// all copies or substantial portions of the Software. | ||
// | ||
// THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | ||
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, | ||
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE | ||
// AUTHORS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN | ||
// ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION | ||
// WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. | ||
// when used in node, this will actually load the util module we depend on | ||
// versus loading the builtin util module as happens otherwise | ||
// this is a bug in node module loading as far as I am concerned | ||
var util = require('util/'); | ||
var pSlice = Array.prototype.slice; | ||
var hasOwn = Object.prototype.hasOwnProperty; | ||
// 1. The assert module provides functions that throw | ||
// AssertionError's when particular conditions are not met. The | ||
// assert module must conform to the following interface. | ||
var assert = module.exports = ok; | ||
// 2. The AssertionError is defined in assert. | ||
// new assert.AssertionError({ message: message, | ||
// actual: actual, | ||
// expected: expected }) | ||
assert.AssertionError = function AssertionError(options) { | ||
this.name = 'AssertionError'; | ||
this.actual = options.actual; | ||
this.expected = options.expected; | ||
this.operator = options.operator; | ||
if (options.message) { | ||
this.message = options.message; | ||
this.generatedMessage = false; | ||
} else { | ||
this.message = getMessage(this); | ||
this.generatedMessage = true; | ||
lchuv: function(luv){ | ||
var C = Math.sqrt(); | ||
} | ||
var stackStartFunction = options.stackStartFunction || fail; | ||
if (Error.captureStackTrace) { | ||
Error.captureStackTrace(this, stackStartFunction); | ||
} | ||
else { | ||
// non v8 browsers so we can have a stacktrace | ||
var err = new Error(); | ||
if (err.stack) { | ||
var out = err.stack; | ||
// try to strip useless frames | ||
var fn_name = stackStartFunction.name; | ||
var idx = out.indexOf('\n' + fn_name); | ||
if (idx >= 0) { | ||
// once we have located the function frame | ||
// we need to strip out everything before it (and its line) | ||
var next_line = out.indexOf('\n', idx + 1); | ||
out = out.substring(next_line + 1); | ||
} | ||
this.stack = out; | ||
} | ||
} | ||
}; | ||
// assert.AssertionError instanceof Error | ||
util.inherits(assert.AssertionError, Error); | ||
function replacer(key, value) { | ||
if (util.isUndefined(value)) { | ||
return '' + value; | ||
} | ||
if (util.isNumber(value) && (isNaN(value) || !isFinite(value))) { | ||
return value.toString(); | ||
} | ||
if (util.isFunction(value) || util.isRegExp(value)) { | ||
return value.toString(); | ||
} | ||
return value; | ||
} | ||
//http://www.brucelindbloom.com/index.html?Equations.html | ||
xyz.luv = function(arg, i) { | ||
var _u, _v, l, u, v, x, y, z, yn, un, vn; | ||
function truncate(s, n) { | ||
if (util.isString(s)) { | ||
return s.length < n ? s : s.slice(0, n); | ||
} else { | ||
return s; | ||
} | ||
} | ||
//get constants | ||
var e = 0.008856451679035631; //(6/29)^3 | ||
var k = 903.2962962962961; //(29/3)^3 | ||
function getMessage(self) { | ||
return truncate(JSON.stringify(self.actual, replacer), 128) + ' ' + | ||
self.operator + ' ' + | ||
truncate(JSON.stringify(self.expected, replacer), 128); | ||
} | ||
//get illuminant | ||
i = i || 'D65'; | ||
// At present only the three keys mentioned above are used and | ||
// understood by the spec. Implementations or sub modules can pass | ||
// other keys to the AssertionError's constructor - they will be | ||
// ignored. | ||
yn = xyz.illuminant[i][1]; | ||
un = luv.illuminant[i][1]/100; | ||
vn = luv.illuminant[i][2]/100; | ||
// 3. All of the following functions must throw an AssertionError | ||
// when a corresponding condition is not met, with a message that | ||
// may be undefined if not provided. All assertion methods provide | ||
// both the actual and expected values to the assertion error for | ||
// display purposes. | ||
x = arg[0]/100, y = arg[1]/100, z = arg[2]/100; | ||
function fail(actual, expected, message, operator, stackStartFunction) { | ||
throw new assert.AssertionError({ | ||
message: message, | ||
actual: actual, | ||
expected: expected, | ||
operator: operator, | ||
stackStartFunction: stackStartFunction | ||
}); | ||
} | ||
_u = (4 * x) / (x + (15 * y) + (3 * z)); | ||
_v = (9 * y) / (x + (15 * y) + (3 * z)); | ||
// EXTENSION! allows for well behaved errors defined elsewhere. | ||
assert.fail = fail; | ||
var yr = y/yn; | ||
// 4. Pure assertion tests whether a value is truthy, as determined | ||
// by !!guard. | ||
// assert.ok(guard, message_opt); | ||
// This statement is equivalent to assert.equal(true, !!guard, | ||
// message_opt);. To test strictly for the value true, use | ||
// assert.strictEqual(true, guard, message_opt);. | ||
l = yr <= e ? k * yr : 116 * Math.pow(yr, .333333333) - 16; | ||
function ok(value, message) { | ||
if (!value) fail(value, true, message, '==', assert.ok); | ||
} | ||
assert.ok = ok; | ||
u = 13 * l * (_u - un); | ||
v = 13 * l * (_v - vn); | ||
// 5. The equality assertion tests shallow, coercive equality with | ||
// ==. | ||
// assert.equal(actual, expected, message_opt); | ||
assert.equal = function equal(actual, expected, message) { | ||
if (actual != expected) fail(actual, expected, message, '==', assert.equal); | ||
return [l, u, v]; | ||
}; | ||
// 6. The non-equality assertion tests for whether two objects are not equal | ||
// with != assert.notEqual(actual, expected, message_opt); | ||
assert.notEqual = function notEqual(actual, expected, message) { | ||
if (actual == expected) { | ||
fail(actual, expected, message, '!=', assert.notEqual); | ||
} | ||
rgb.luv = function(args){ | ||
return xyz.luv(rgb.xyz(args)); | ||
}; | ||
// 7. The equivalence assertion tests a deep equality relation. | ||
// assert.deepEqual(actual, expected, message_opt); | ||
assert.deepEqual = function deepEqual(actual, expected, message) { | ||
if (!_deepEqual(actual, expected)) { | ||
fail(actual, expected, message, 'deepEqual', assert.deepEqual); | ||
} | ||
}; | ||
function _deepEqual(actual, expected) { | ||
// 7.1. All identical values are equivalent, as determined by ===. | ||
if (actual === expected) { | ||
return true; | ||
} else if (util.isBuffer(actual) && util.isBuffer(expected)) { | ||
if (actual.length != expected.length) return false; | ||
for (var i = 0; i < actual.length; i++) { | ||
if (actual[i] !== expected[i]) return false; | ||
} | ||
return true; | ||
// 7.2. If the expected value is a Date object, the actual value is | ||
// equivalent if it is also a Date object that refers to the same time. | ||
} else if (util.isDate(actual) && util.isDate(expected)) { | ||
return actual.getTime() === expected.getTime(); | ||
// 7.3 If the expected value is a RegExp object, the actual value is | ||
// equivalent if it is also a RegExp object with the same source and | ||
// properties (`global`, `multiline`, `lastIndex`, `ignoreCase`). | ||
} else if (util.isRegExp(actual) && util.isRegExp(expected)) { | ||
return actual.source === expected.source && | ||
actual.global === expected.global && | ||
actual.multiline === expected.multiline && | ||
actual.lastIndex === expected.lastIndex && | ||
actual.ignoreCase === expected.ignoreCase; | ||
// 7.4. Other pairs that do not both pass typeof value == 'object', | ||
// equivalence is determined by ==. | ||
} else if (!util.isObject(actual) && !util.isObject(expected)) { | ||
return actual == expected; | ||
// 7.5 For all other Object pairs, including Array objects, equivalence is | ||
// determined by having the same number of owned properties (as verified | ||
// with Object.prototype.hasOwnProperty.call), the same set of keys | ||
// (although not necessarily the same order), equivalent values for every | ||
// corresponding key, and an identical 'prototype' property. Note: this | ||
// accounts for both named and indexed properties on Arrays. | ||
} else { | ||
return objEquiv(actual, expected); | ||
} | ||
} | ||
function isArguments(object) { | ||
return Object.prototype.toString.call(object) == '[object Arguments]'; | ||
} | ||
function objEquiv(a, b) { | ||
if (util.isNullOrUndefined(a) || util.isNullOrUndefined(b)) | ||
return false; | ||
// an identical 'prototype' property. | ||
if (a.prototype !== b.prototype) return false; | ||
//~~~I've managed to break Object.keys through screwy arguments passing. | ||
// Converting to array solves the problem. | ||
if (isArguments(a)) { | ||
if (!isArguments(b)) { | ||
return false; | ||
} | ||
a = pSlice.call(a); | ||
b = pSlice.call(b); | ||
return _deepEqual(a, b); | ||
} | ||
try { | ||
var ka = objectKeys(a), | ||
kb = objectKeys(b), | ||
key, i; | ||
} catch (e) {//happens when one is a string literal and the other isn't | ||
return false; | ||
} | ||
// having the same number of owned properties (keys incorporates | ||
// hasOwnProperty) | ||
if (ka.length != kb.length) | ||
return false; | ||
//the same set of keys (although not necessarily the same order), | ||
ka.sort(); | ||
kb.sort(); | ||
//~~~cheap key test | ||
for (i = ka.length - 1; i >= 0; i--) { | ||
if (ka[i] != kb[i]) | ||
return false; | ||
} | ||
//equivalent values for every corresponding key, and | ||
//~~~possibly expensive deep test | ||
for (i = ka.length - 1; i >= 0; i--) { | ||
key = ka[i]; | ||
if (!_deepEqual(a[key], b[key])) return false; | ||
} | ||
return true; | ||
} | ||
// 8. The non-equivalence assertion tests for any deep inequality. | ||
// assert.notDeepEqual(actual, expected, message_opt); | ||
assert.notDeepEqual = function notDeepEqual(actual, expected, message) { | ||
if (_deepEqual(actual, expected)) { | ||
fail(actual, expected, message, 'notDeepEqual', assert.notDeepEqual); | ||
} | ||
}; | ||
// 9. The strict equality assertion tests strict equality, as determined by ===. | ||
// assert.strictEqual(actual, expected, message_opt); | ||
assert.strictEqual = function strictEqual(actual, expected, message) { | ||
if (actual !== expected) { | ||
fail(actual, expected, message, '===', assert.strictEqual); | ||
} | ||
}; | ||
// 10. The strict non-equality assertion tests for strict inequality, as | ||
// determined by !==. assert.notStrictEqual(actual, expected, message_opt); | ||
assert.notStrictEqual = function notStrictEqual(actual, expected, message) { | ||
if (actual === expected) { | ||
fail(actual, expected, message, '!==', assert.notStrictEqual); | ||
} | ||
}; | ||
function expectedException(actual, expected) { | ||
if (!actual || !expected) { | ||
return false; | ||
} | ||
if (Object.prototype.toString.call(expected) == '[object RegExp]') { | ||
return expected.test(actual); | ||
} else if (actual instanceof expected) { | ||
return true; | ||
} else if (expected.call({}, actual) === true) { | ||
return true; | ||
} | ||
return false; | ||
} | ||
function _throws(shouldThrow, block, expected, message) { | ||
var actual; | ||
if (util.isString(expected)) { | ||
message = expected; | ||
expected = null; | ||
} | ||
try { | ||
block(); | ||
} catch (e) { | ||
actual = e; | ||
} | ||
message = (expected && expected.name ? ' (' + expected.name + ').' : '.') + | ||
(message ? ' ' + message : '.'); | ||
if (shouldThrow && !actual) { | ||
fail(actual, expected, 'Missing expected exception' + message); | ||
} | ||
if (!shouldThrow && expectedException(actual, expected)) { | ||
fail(actual, expected, 'Got unwanted exception' + message); | ||
} | ||
if ((shouldThrow && actual && expected && | ||
!expectedException(actual, expected)) || (!shouldThrow && actual)) { | ||
throw actual; | ||
} | ||
} | ||
// 11. Expected to throw an error: | ||
// assert.throws(block, Error_opt, message_opt); | ||
assert.throws = function(block, /*optional*/error, /*optional*/message) { | ||
_throws.apply(this, [true].concat(pSlice.call(arguments))); | ||
}; | ||
// EXTENSION! This is annoying to write outside this module. | ||
assert.doesNotThrow = function(block, /*optional*/message) { | ||
_throws.apply(this, [false].concat(pSlice.call(arguments))); | ||
}; | ||
assert.ifError = function(err) { if (err) {throw err;}}; | ||
var objectKeys = Object.keys || function (obj) { | ||
var keys = []; | ||
for (var key in obj) { | ||
if (hasOwn.call(obj, key)) keys.push(key); | ||
} | ||
return keys; | ||
}; | ||
},{"util/":4}],1:[function(require,module,exports){ | ||
},{"./rgb":14,"./xyz":15}],8:[function(require,module,exports){ | ||
if (typeof Object.create === 'function') { | ||
@@ -1180,3 +904,3 @@ // implementation from standard node.js 'util' module | ||
},{}],2:[function(require,module,exports){ | ||
},{}],9:[function(require,module,exports){ | ||
// shim for using process in browser | ||
@@ -1269,3 +993,3 @@ | ||
},{}],3:[function(require,module,exports){ | ||
},{}],10:[function(require,module,exports){ | ||
module.exports = function isBuffer(arg) { | ||
@@ -1277,3 +1001,3 @@ return arg && typeof arg === 'object' | ||
} | ||
},{}],4:[function(require,module,exports){ | ||
},{}],11:[function(require,module,exports){ | ||
(function (process,global){ | ||
@@ -1868,3 +1592,3 @@ // Copyright Joyent, Inc. and other Node contributors. | ||
}).call(this,require('_process'),typeof global !== "undefined" ? global : typeof self !== "undefined" ? self : typeof window !== "undefined" ? window : {}) | ||
},{"./support/isBuffer":3,"_process":2,"inherits":1}],5:[function(require,module,exports){ | ||
},{"./support/isBuffer":10,"_process":9,"inherits":8}],12:[function(require,module,exports){ | ||
'use strict'; | ||
@@ -1899,3 +1623,3 @@ | ||
} | ||
},{}],6:[function(require,module,exports){ | ||
},{}],13:[function(require,module,exports){ | ||
var slice = [].slice; | ||
@@ -1910,3 +1634,247 @@ | ||
}; | ||
},{}],"mumath":[function(require,module,exports){ | ||
},{}],14:[function(require,module,exports){ | ||
var rgb = module.exports = { | ||
name: 'rgb', | ||
min: [0,0,0], | ||
max: [255,255,255], | ||
channel: ['red', 'green', 'blue'], | ||
hsl: function(rgb) { | ||
var r = rgb[0]/255, | ||
g = rgb[1]/255, | ||
b = rgb[2]/255, | ||
min = Math.min(r, g, b), | ||
max = Math.max(r, g, b), | ||
delta = max - min, | ||
h, s, l; | ||
if (max == min) | ||
h = 0; | ||
else if (r == max) | ||
h = (g - b) / delta; | ||
else if (g == max) | ||
h = 2 + (b - r) / delta; | ||
else if (b == max) | ||
h = 4 + (r - g)/ delta; | ||
h = Math.min(h * 60, 360); | ||
if (h < 0) | ||
h += 360; | ||
l = (min + max) / 2; | ||
if (max == min) | ||
s = 0; | ||
else if (l <= 0.5) | ||
s = delta / (max + min); | ||
else | ||
s = delta / (2 - max - min); | ||
return [h, s * 100, l * 100]; | ||
}, | ||
hsv: function(rgb) { | ||
var r = rgb[0], | ||
g = rgb[1], | ||
b = rgb[2], | ||
min = Math.min(r, g, b), | ||
max = Math.max(r, g, b), | ||
delta = max - min, | ||
h, s, v; | ||
if (max == 0) | ||
s = 0; | ||
else | ||
s = (delta/max * 1000)/10; | ||
if (max == min) | ||
h = 0; | ||
else if (r == max) | ||
h = (g - b) / delta; | ||
else if (g == max) | ||
h = 2 + (b - r) / delta; | ||
else if (b == max) | ||
h = 4 + (r - g) / delta; | ||
h = Math.min(h * 60, 360); | ||
if (h < 0) | ||
h += 360; | ||
v = ((max / 255) * 1000) / 10; | ||
return [h, s, v]; | ||
}, | ||
hwb: function(val) { | ||
var r = val[0], | ||
g = val[1], | ||
b = val[2], | ||
h = rgb.hsl(val)[0], | ||
w = 1/255 * Math.min(r, Math.min(g, b)), | ||
b = 1 - 1/255 * Math.max(r, Math.max(g, b)); | ||
return [h, w * 100, b * 100]; | ||
}, | ||
cmyk: function(rgb) { | ||
var r = rgb[0] / 255, | ||
g = rgb[1] / 255, | ||
b = rgb[2] / 255, | ||
c, m, y, k; | ||
k = Math.min(1 - r, 1 - g, 1 - b); | ||
c = (1 - r - k) / (1 - k) || 0; | ||
m = (1 - g - k) / (1 - k) || 0; | ||
y = (1 - b - k) / (1 - k) || 0; | ||
return [c * 100, m * 100, y * 100, k * 100]; | ||
}, | ||
xyz: function(rgb) { | ||
var r = rgb[0] / 255, | ||
g = rgb[1] / 255, | ||
b = rgb[2] / 255; | ||
// assume sRGB | ||
r = r > 0.04045 ? Math.pow(((r + 0.055) / 1.055), 2.4) : (r / 12.92); | ||
g = g > 0.04045 ? Math.pow(((g + 0.055) / 1.055), 2.4) : (g / 12.92); | ||
b = b > 0.04045 ? Math.pow(((b + 0.055) / 1.055), 2.4) : (b / 12.92); | ||
var x = (r * 0.4124) + (g * 0.3576) + (b * 0.1805); | ||
var y = (r * 0.2126) + (g * 0.7152) + (b * 0.0722); | ||
var z = (r * 0.0193) + (g * 0.1192) + (b * 0.9505); | ||
return [x * 100, y *100, z * 100]; | ||
}, | ||
lab: function(args) { | ||
var xyz = rgb.xyz(args), | ||
x = xyz[0], | ||
y = xyz[1], | ||
z = xyz[2], | ||
l, a, b; | ||
x /= 95.047; | ||
y /= 100; | ||
z /= 108.883; | ||
x = x > 0.008856 ? Math.pow(x, 1/3) : (7.787 * x) + (16 / 116); | ||
y = y > 0.008856 ? Math.pow(y, 1/3) : (7.787 * y) + (16 / 116); | ||
z = z > 0.008856 ? Math.pow(z, 1/3) : (7.787 * z) + (16 / 116); | ||
l = (116 * y) - 16; | ||
a = 500 * (x - y); | ||
b = 200 * (y - z); | ||
return [l, a, b]; | ||
} | ||
}; | ||
},{}],15:[function(require,module,exports){ | ||
var rgb = require('./rgb'); | ||
var xyz = module.exports = { | ||
name: 'xyz', | ||
min: [0,0,0], | ||
max: [96,100,109], | ||
channel: ['lightness','u','v'], | ||
alias: ['ciexyz'], | ||
//Xn, Yn, Zn | ||
illuminant: { | ||
A:[109.85, 100, 35.58], | ||
C: [98.07, 100, 118.23], | ||
E: [100,100,100], | ||
D65: [95.04, 100, 108.88] | ||
}, | ||
//TODO: fix this maths so to return 255,255,255 in rgb | ||
rgb: function(xyz) { | ||
var x = xyz[0] / 100, | ||
y = xyz[1] / 100, | ||
z = xyz[2] / 100, | ||
r, g, b; | ||
// assume sRGB | ||
// http://www.brucelindbloom.com/index.html?Eqn_RGB_XYZ_Matrix.html | ||
r = (x * 3.2404542) + (y * -1.5371385) + (z * -0.4985314); | ||
g = (x * -0.9692660) + (y * 1.8760108) + (z * 0.0415560); | ||
b = (x * 0.0556434) + (y * -0.2040259) + (z * 1.0572252); | ||
r = r > 0.0031308 ? ((1.055 * Math.pow(r, 1.0 / 2.4)) - 0.055) | ||
: r = (r * 12.92); | ||
g = g > 0.0031308 ? ((1.055 * Math.pow(g, 1.0 / 2.4)) - 0.055) | ||
: g = (g * 12.92); | ||
b = b > 0.0031308 ? ((1.055 * Math.pow(b, 1.0 / 2.4)) - 0.055) | ||
: b = (b * 12.92); | ||
r = Math.min(Math.max(0, r), 1); | ||
g = Math.min(Math.max(0, g), 1); | ||
b = Math.min(Math.max(0, b), 1); | ||
return [r * 255, g * 255, b * 255]; | ||
}, | ||
hsl: function(arg) { | ||
return rgb.hsl(xyz.rgb(arg)); | ||
}, | ||
hsv: function(arg) { | ||
return rgb.hsv(xyz.rgb(arg)); | ||
}, | ||
hwb: function(arg) { | ||
return rgb.hwb(xyz.rgb(arg)); | ||
}, | ||
cmyk: function(arg) { | ||
return rgb.cmyk(xyz.rgb(arg)); | ||
}, | ||
lab: function(xyz) { | ||
var x = xyz[0], | ||
y = xyz[1], | ||
z = xyz[2], | ||
l, a, b; | ||
x /= 95.047; | ||
y /= 100; | ||
z /= 108.883; | ||
x = x > 0.008856 ? Math.pow(x, 1/3) : (7.787 * x) + (16 / 116); | ||
y = y > 0.008856 ? Math.pow(y, 1/3) : (7.787 * y) + (16 / 116); | ||
z = z > 0.008856 ? Math.pow(z, 1/3) : (7.787 * z) + (16 / 116); | ||
l = (116 * y) - 16; | ||
a = 500 * (x - y); | ||
b = 200 * (y - z); | ||
return [l, a, b]; | ||
}, | ||
//TODO | ||
cam: function(xyz){ | ||
var x = xyz[0], y = xyz[1], z = xyz[2]; | ||
//Mcat02 | ||
var m =[[0.7328, 0.4296, -0.1624], [-0.7036, 1.6975, 0.0061], [0.0030, 0.0136, 0.9834]]; | ||
//get lms | ||
var L = x*m[0][0] + y*m[0][1] + z*m[0][2]; | ||
var M = x*m[1][0] + y*m[1][1] + z*m[1][2]; | ||
var S = x*m[2][0] + y*m[2][1] + z*m[2][2]; | ||
//calc lc, mc, sc | ||
//FIXME: choose proper d | ||
var d = 0.85; | ||
var Lwr = 100, Mwr = 100, Swr = 100; | ||
var Lc = (Lwr*D/Lw + 1 - D) * L; | ||
var Mc = (Mwr*D/Mw + 1 - D) * M; | ||
var Sc = (Swr*D/Sw + 1 - D) * S; | ||
} | ||
}; | ||
},{"./rgb":14}],"mumath":[function(require,module,exports){ | ||
/** | ||
@@ -2276,2 +2244,2 @@ * Simple math utils. | ||
exports.prev = pseudos.prev; | ||
},{"matches-selector":5,"tiny-element":6}]},{},[]); | ||
},{"matches-selector":12,"tiny-element":13}]},{},[]); |
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
No License Found
License(Experimental) License information could not be found.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
223450
23
7915
80
0
1