command-line-args
Advanced tools
Comparing version 4.0.7 to 5.0.0-alpha.0
@@ -21,3 +21,3 @@ 'use strict' | ||
* { name: "verbose" }, | ||
* { name: "depth"} | ||
* { name: "depth" } | ||
* ] | ||
@@ -84,3 +84,3 @@ * ``` | ||
/** | ||
* getopt-style short option names. Can be any single character (unicode included) except a digit or hypen. | ||
* getopt-style short option names. Can be any single character (unicode included) except a digit or hyphen. | ||
* | ||
@@ -124,2 +124,8 @@ * ```js | ||
/** | ||
* Identical to `multiple` but with greedy parsing disabled. | ||
* @type {boolean} | ||
*/ | ||
this.lazyMultiple = definition.lazyMultiple | ||
/** | ||
* Any unclaimed command-line args will be set on this option. This flag is typically set on the most commonly-used option to make for more concise usage (i.e. `$ myapp *.js` instead of `$ myapp --files *.js`). | ||
@@ -234,7 +240,15 @@ * | ||
isBoolean (value) { | ||
isBoolean () { | ||
return this.type === Boolean || (t.isFunction(this.type) && this.type.name === 'Boolean') | ||
} | ||
isMultiple () { | ||
return this.multiple || this.lazyMultiple | ||
} | ||
static create (def) { | ||
const result = new this(def) | ||
return result | ||
} | ||
} | ||
module.exports = OptionDefinition |
'use strict' | ||
const arrayify = require('array-back') | ||
const option = require('./option') | ||
const Definition = require('./definition') | ||
const option = require('./option-util') | ||
const t = require('typical') | ||
@@ -9,3 +8,2 @@ | ||
* @module definitions | ||
* @private | ||
*/ | ||
@@ -17,8 +15,2 @@ | ||
class Definitions extends Array { | ||
load (definitions) { | ||
this.clear() | ||
arrayify(definitions).forEach(def => this.push(new Definition(def))) | ||
this.validate() | ||
} | ||
clear () { | ||
@@ -32,7 +24,7 @@ this.length = 0 | ||
*/ | ||
validate (argv) { | ||
validate () { | ||
const someHaveNoName = this.some(def => !def.name) | ||
if (someHaveNoName) { | ||
halt( | ||
'NAME_MISSING', | ||
'INVALID_DEFINITIONS', | ||
'Invalid option definitions: the `name` property is required on each definition' | ||
@@ -45,3 +37,3 @@ ) | ||
halt( | ||
'INVALID_TYPE', | ||
'INVALID_DEFINITIONS', | ||
'Invalid option definitions: the `type` property must be a setter fuction (default: `Boolean`)' | ||
@@ -59,3 +51,3 @@ ) | ||
halt( | ||
'INVALID_ALIAS', | ||
'INVALID_DEFINITIONS', | ||
'Invalid option definition: to avoid ambiguity an alias cannot be numeric [--' + invalidOption.name + ' alias is -' + invalidOption.alias + ']' | ||
@@ -71,3 +63,3 @@ ) | ||
halt( | ||
'INVALID_ALIAS', | ||
'INVALID_DEFINITIONS', | ||
'Invalid option definition: an alias must be a single character' | ||
@@ -83,3 +75,3 @@ ) | ||
halt( | ||
'INVALID_ALIAS', | ||
'INVALID_DEFINITIONS', | ||
'Invalid option definition: an alias cannot be "-"' | ||
@@ -92,3 +84,3 @@ ) | ||
halt( | ||
'DUPLICATE_NAME', | ||
'INVALID_DEFINITIONS', | ||
'Two or more option definitions have the same name' | ||
@@ -101,3 +93,3 @@ ) | ||
halt( | ||
'DUPLICATE_ALIAS', | ||
'INVALID_DEFINITIONS', | ||
'Two or more option definitions have the same alias' | ||
@@ -110,9 +102,21 @@ ) | ||
halt( | ||
'DUPLICATE_DEFAULT_OPTION', | ||
'INVALID_DEFINITIONS', | ||
'Only one option definition can be the defaultOption' | ||
) | ||
} | ||
const defaultBoolean = this.some(def => { | ||
invalidOption = def | ||
return def.isBoolean() && def.defaultOption | ||
}) | ||
if (defaultBoolean) { | ||
halt( | ||
'INVALID_DEFINITIONS', | ||
`A boolean option ["${invalidOption.name}"] can not also be the defaultOption.` | ||
) | ||
} | ||
} | ||
/** | ||
* Get definition by option arg (e.g. `--one` or `-o`) | ||
* @param {string} | ||
@@ -122,5 +126,9 @@ * @returns {Definition} | ||
get (arg) { | ||
return option.short.test(arg) | ||
? this.find(def => def.alias === option.short.name(arg)) | ||
: this.find(def => def.name === option.long.name(arg)) | ||
if (option.isOption(arg)) { | ||
return option.short.test(arg) | ||
? this.find(def => def.alias === option.short.name(arg)) | ||
: this.find(def => def.name === option.long.name(arg)) | ||
} else { | ||
return this.find(def => def.name === arg) | ||
} | ||
} | ||
@@ -142,2 +150,13 @@ | ||
} | ||
whereDefaultValueSet () { | ||
return this.filter(def => t.isDefined(def.defaultValue)) | ||
} | ||
static from (definitions) { | ||
if (definitions instanceof this) return definitions | ||
const Definition = require('./definition') | ||
const result = super.from(arrayify(definitions), def => Definition.create(def)) | ||
result.validate() | ||
return result | ||
} | ||
} | ||
@@ -144,0 +163,0 @@ |
'use strict' | ||
const _value = new WeakMap() | ||
const arrayify = require('array-back') | ||
const t = require('typical') | ||
const Definition = require('./definition') | ||
class ArgRegExp extends RegExp { | ||
name (arg) { | ||
return arg.match(this)[1] | ||
/** | ||
* Encapsulates behaviour (defined by an OptionDefinition) when setting values | ||
*/ | ||
class Option { | ||
constructor (definition) { | ||
this.definition = new Definition(definition) | ||
this.state = null /* set or default */ | ||
this.resetToDefault() | ||
} | ||
get () { | ||
return _value.get(this) | ||
} | ||
set (val) { | ||
this._set(val, 'set') | ||
} | ||
_set (val, state) { | ||
const def = this.definition | ||
if (def.multiple || def.lazyMultiple) { | ||
/* don't add null or undefined to a multiple */ | ||
if (val !== null && val !== undefined) { | ||
const arr = this.get() | ||
if (this.state === 'default') arr.length = 0 | ||
arr.push(def.type(val)) | ||
this.state = state | ||
} | ||
} else { | ||
/* throw if already set on a singlar defaultOption */ | ||
if (def.defaultOption && !def.multiple && this.state === 'set') { | ||
const err = new Error('Singular defaultOption already set with: ' + this.get()) | ||
err.name = 'ALREADY_SET' | ||
err.value = val | ||
throw err | ||
} else if (val === null || val === undefined) { | ||
_value.set(this, val) | ||
/* required to make 'partial: defaultOption with value equal to defaultValue 2' pass */ | ||
if (!(def.defaultOption && !def.multiple)) { | ||
this.state = state | ||
} | ||
} else { | ||
_value.set(this, def.type(val)) | ||
this.state = state | ||
} | ||
} | ||
} | ||
resetToDefault () { | ||
if (t.isDefined(this.definition.defaultValue)) { | ||
if (this.definition.isMultiple()) { | ||
_value.set(this, arrayify(this.definition.defaultValue).slice()) | ||
} else { | ||
_value.set(this, this.definition.defaultValue) | ||
} | ||
} else { | ||
if (this.definition.isMultiple()) { | ||
_value.set(this, []) | ||
} else { | ||
_value.set(this, null) | ||
} | ||
} | ||
this.state = 'default' | ||
} | ||
static create (definition) { | ||
definition = new Definition(definition) | ||
if (definition.isBoolean()) { | ||
return require('./option-flag').create(definition) | ||
} else { | ||
return new this(definition) | ||
} | ||
} | ||
} | ||
exports.short = new ArgRegExp('^-([^\\d-])$') | ||
exports.long = new ArgRegExp('^--(\\S+)') | ||
exports.combined = new ArgRegExp('^-([^\\d-]{2,})$') | ||
exports.isOption = arg => exports.short.test(arg) || exports.long.test(arg) | ||
exports.optEquals = new ArgRegExp('^(--\\S+?)=(.*)') | ||
exports.VALUE_MARKER = '552f3a31-14cd-4ced-bd67-656a659e9efb' // must be unique | ||
module.exports = Option |
'use strict' | ||
const t = require('typical') | ||
const arrayify = require('array-back') | ||
const Option = require('./option') | ||
class OutputValue { | ||
constructor (value) { | ||
this.value = value | ||
this.hasDefaultArrayValue = false | ||
this.valueSource = 'unknown' | ||
} | ||
/** | ||
* A map of { DefinitionNameString: Option }. By default, an Output has an `_unknown` property and any options with defaultValues. | ||
*/ | ||
class Output extends Map { | ||
constructor (definitions) { | ||
super() | ||
const Definitions = require('./definitions') | ||
/** | ||
* @type {OptionDefinitions} | ||
*/ | ||
this.definitions = Definitions.from(definitions) | ||
isDefined () { | ||
return t.isDefined(this.value) | ||
} | ||
} | ||
class Output { | ||
constructor (definitions, options) { | ||
this.options = options || {} | ||
this.output = {} | ||
this.unknown = [] | ||
this.definitions = definitions | ||
this._assignDefaultValues() | ||
} | ||
_assignDefaultValues () { | ||
this.definitions.forEach(def => { | ||
if (t.isDefined(def.defaultValue)) { | ||
if (def.multiple) { | ||
this.output[def.name] = new OutputValue(arrayify(def.defaultValue)) | ||
this.output[def.name].hasDefaultArrayValue = true | ||
} else { | ||
this.output[def.name] = new OutputValue(def.defaultValue) | ||
} | ||
this.output[def.name].valueSource = 'default' | ||
} | ||
}) | ||
} | ||
setFlag (optionArg) { | ||
const def = this.definitions.get(optionArg) | ||
if (def) { | ||
this.output[def.name] = this.output[def.name] || new OutputValue() | ||
const outputValue = this.output[def.name] | ||
if (def.multiple) outputValue.value = outputValue.value || [] | ||
/* for boolean types, set value to `true`. For all other types run value through setter function. */ | ||
if (def.isBoolean()) { | ||
if (Array.isArray(outputValue.value)) { | ||
outputValue.value.push(true) | ||
} else { | ||
outputValue.value = true | ||
} | ||
return true | ||
} else { | ||
if (!Array.isArray(outputValue.value) && outputValue.valueSource === 'unknown') outputValue.value = null | ||
return false | ||
} | ||
} else { | ||
this.unknown.push(optionArg) | ||
return true | ||
/* by default, an Output has an `_unknown` property and any options with defaultValues */ | ||
this.set('_unknown', Option.create({ name: '_unknown', multiple: true })) | ||
for (const def of this.definitions.whereDefaultValueSet()) { | ||
this.set(def.name, Option.create(def)) | ||
} | ||
} | ||
setOptionValue (optionArg, value) { | ||
const ValueArg = require('./value-arg') | ||
const valueArg = new ValueArg(value) | ||
const def = this.definitions.get(optionArg) | ||
this.output[def.name] = this.output[def.name] || new OutputValue() | ||
const outputValue = this.output[def.name] | ||
if (def.multiple) outputValue.value = outputValue.value || [] | ||
/* run value through setter function. */ | ||
valueArg.value = def.type(valueArg.value) | ||
outputValue.valueSource = 'argv' | ||
if (Array.isArray(outputValue.value)) { | ||
if (outputValue.hasDefaultArrayValue) { | ||
outputValue.value = [ valueArg.value ] | ||
outputValue.hasDefaultArrayValue = false | ||
} else { | ||
outputValue.value.push(valueArg.value) | ||
} | ||
return false | ||
} else { | ||
outputValue.value = valueArg.value | ||
return true | ||
toObject (options) { | ||
options = options || {} | ||
const output = {} | ||
for (const item of this) { | ||
const name = item[0] | ||
const option = item[1] | ||
if (name === '_unknown' && !option.get().length) continue | ||
output[name] = option.get() | ||
} | ||
} | ||
/** | ||
* Return `true` when an option value was set and is not a multiple. Return `false` if option was a multiple or if a value was not yet set. | ||
*/ | ||
setValue (value) { | ||
const ValueArg = require('./value-arg') | ||
const valueArg = new ValueArg(value) | ||
/* use the defaultOption */ | ||
const def = this.definitions.getDefault() | ||
/* handle unknown values in the case a value was already set on a defaultOption */ | ||
if (def) { | ||
const currentValue = this.output[def.name] | ||
if (valueArg.isDefined() && currentValue && t.isDefined(currentValue.value)) { | ||
if (def.multiple) { | ||
/* in the case we're setting an --option=value value on a multiple defaultOption, tag the value onto the previous unknown */ | ||
if (valueArg.isOptionValueNotationValue && this.unknown.length) { | ||
this.unknown[this.unknown.length - 1] += `=${valueArg.value}` | ||
return true | ||
} | ||
} else { | ||
/* currentValue has already been set by argv,log this value as unknown and move on */ | ||
if (currentValue.valueSource === 'argv') { | ||
this.unknown.push(valueArg.value) | ||
return true | ||
} | ||
} | ||
} | ||
return this.setOptionValue(`--${def.name}`, value) | ||
} else { | ||
if (valueArg.isOptionValueNotationValue) { | ||
this.unknown[this.unknown.length - 1] += `=${valueArg.value}` | ||
} else { | ||
this.unknown.push(valueArg.value) | ||
} | ||
return true | ||
} | ||
} | ||
get (name) { | ||
return this.output[name] && this.output[name].value | ||
} | ||
toObject () { | ||
let output = Object.assign({}, this.output) | ||
if (this.options.partial && this.unknown.length) { | ||
output._unknown = this.unknown | ||
} | ||
for (const prop in output) { | ||
if (prop !== '_unknown') { | ||
output[prop] = output[prop].value | ||
} | ||
} | ||
if (options.skipUnknown) delete output._unknown | ||
return output | ||
@@ -149,0 +35,0 @@ } |
{ | ||
"name": "command-line-args", | ||
"version": "4.0.7", | ||
"version": "5.0.0-alpha.0", | ||
"description": "A mature, feature-complete library to parse command-line options.", | ||
"repository": "https://github.com/75lb/command-line-args.git", | ||
"main": "lib/command-line-args.js", | ||
"bin": "bin/cli.js", | ||
"scripts": { | ||
"test": "test-runner test/*.js", | ||
"docs": "jsdoc2md -l off -t jsdoc2md/README.hbs lib/*.js > README.md; echo", | ||
"cover": "istanbul cover ./node_modules/.bin/test-runner test/*.js && cat coverage/lcov.info | ./node_modules/.bin/coveralls && rm -rf coverage; echo" | ||
"test": "test-runner test/*.js test/internals/*.js", | ||
"docs": "jsdoc2md index.js > doc/API.md && jsdoc2md lib/definition.js > doc/option-definition.md", | ||
"cover": "istanbul cover ./node_modules/.bin/test-runner test/internal/*.js test/external/*.js && cat coverage/lcov.info | ./node_modules/.bin/coveralls #&& rm -rf coverage; echo" | ||
}, | ||
@@ -28,12 +26,16 @@ "keywords": [ | ||
"license": "MIT", | ||
"engines": { | ||
"node": ">=4.0.0" | ||
}, | ||
"devDependencies": { | ||
"coveralls": "^2.13.1", | ||
"jsdoc-to-markdown": "^3.0.0", | ||
"test-runner": "^0.4.1" | ||
"coveralls": "^3.0.0", | ||
"jsdoc-to-markdown": "^3.0.3", | ||
"test-runner": "^0.5.0-1" | ||
}, | ||
"dependencies": { | ||
"array-back": "^2.0.0", | ||
"find-replace": "^1.0.3", | ||
"find-replace": "^2.0.1", | ||
"lodash.camelcase": "^4.3.0", | ||
"typical": "^2.6.1" | ||
} | ||
} |
327
README.md
@@ -15,3 +15,3 @@ [](https://www.npmjs.org/package/command-line-args) | ||
## Synopsis | ||
You can set options using the main notation standards (getopt, getopt_long, etc.). These commands are all equivalent, setting the same values: | ||
You can set options using the main notation standards ([learn more](https://github.com/75lb/command-line-args/wiki/Notation-rules)). These commands are all equivalent, setting the same values: | ||
``` | ||
@@ -24,6 +24,5 @@ $ example --verbose --timeout=1000 --src one.js --src two.js | ||
To access the values, first describe the options your app accepts (see [option definitions](#optiondefinition-)). | ||
To access the values, first create a list of [option definitions](https://github.com/75lb/command-line-args/blob/next/doc/option-definition.md) describing your accepted options. The [`type`](https://github.com/75lb/command-line-args/blob/next/doc/option-definition.md#optiontype--function) property is a setter function (the value supplied is passed through this), giving you full control over the value received. | ||
```js | ||
const commandLineArgs = require('command-line-args') | ||
const optionDefinitions = [ | ||
@@ -35,6 +34,6 @@ { name: 'verbose', alias: 'v', type: Boolean }, | ||
``` | ||
The [`type`](#optiontype--function) property is a setter function (the value supplied is passed through this), giving you full control over the value received. | ||
Next, parse the options using [commandLineArgs()](#commandlineargsdefinitions-argv--object-): | ||
Next, parse the options using [commandLineArgs()](https://github.com/75lb/command-line-args/blob/next/doc/API.md#commandlineargsoptiondefinitions-options--object-): | ||
```js | ||
const commandLineArgs = require('command-line-args') | ||
const options = commandLineArgs(optionDefinitions) | ||
@@ -55,68 +54,18 @@ ``` | ||
When dealing with large amounts of options it often makes sense to [group](#optiongroup--string--arraystring) them. | ||
## Usage guide generation | ||
A usage guide can be generated using [command-line-usage](https://github.com/75lb/command-line-usage), for example: | ||
A usage guide (typically printed when `--help` is set) can be generated using [command-line-usage](https://github.com/75lb/command-line-usage). See the examples below and [read the documentation](https://github.com/75lb/command-line-usage) for instructions how to create them. | ||
A typical usage guide example. | ||
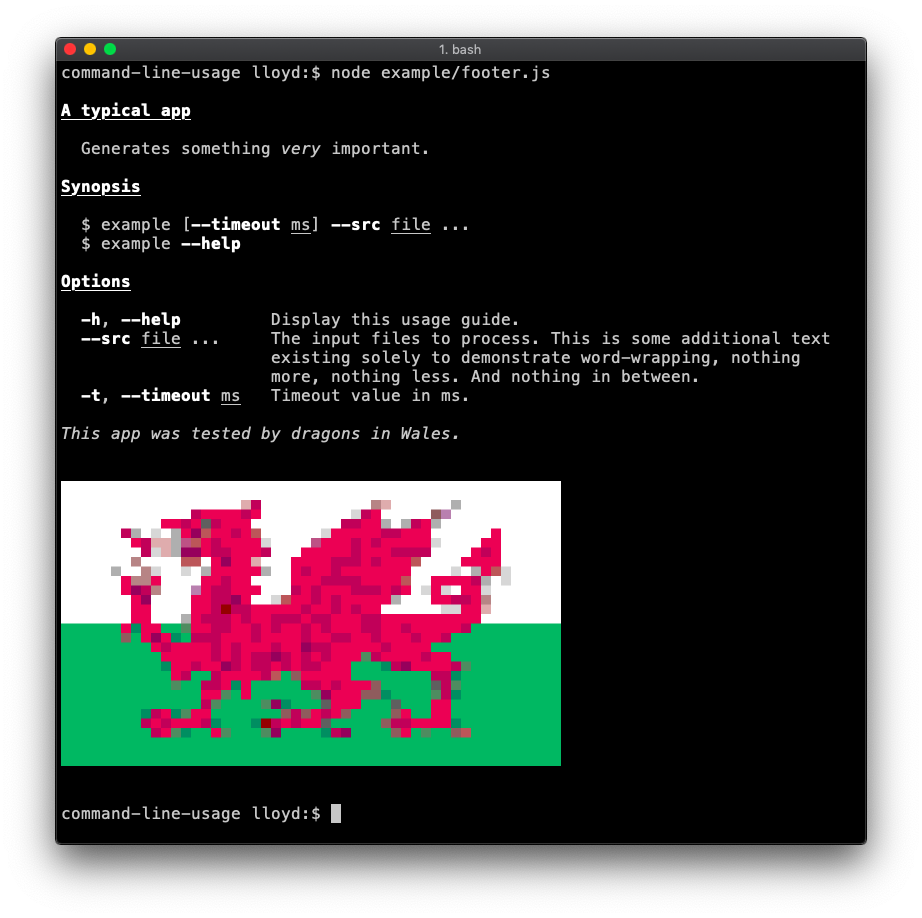 | ||
### Notation rules | ||
The [polymer-cli](https://github.com/Polymer/polymer-cli/) usage guide is a good real-life example. | ||
Notation rules for setting command-line options. | ||
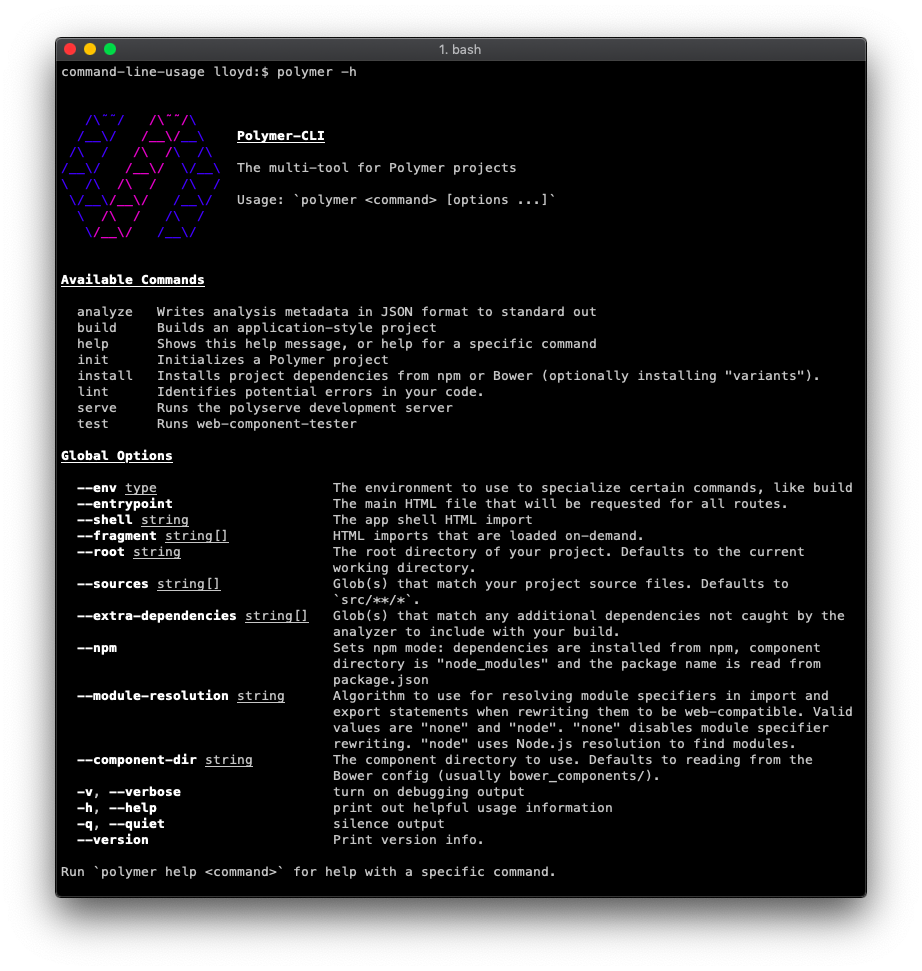 | ||
* Argument order is insignificant. Whether you set `--example` at the beginning or end of the arg list makes no difference. | ||
* Options with a [type](#optiontype--function) of `Boolean` do not need to supply a value. Setting `--flag` or `-f` will set that option's value to `true`. This is the only [type](#optiontype--function) with special behaviour. | ||
* Three ways to set an option value | ||
* `--option value` | ||
* `--option=value` | ||
* `-o value` | ||
* Two ways to a set list of values (on options with [multiple](#optionmultiple--boolean) set) | ||
* `--list one two three` | ||
* `--list one --list two --list three` | ||
* Short options ([alias](#optionalias--string)) can be set in groups. The following are equivalent: | ||
* `-a -b -c` | ||
* `-abc` | ||
## Further Reading | ||
### Ambiguous values | ||
There is plenty more to learn, please see [the wiki](https://github.com/75lb/command-line-args/wiki) for further examples and documentation. | ||
Imagine we are using "grep-tool" to search for the string `'-f'`: | ||
``` | ||
$ grep-tool --search -f | ||
``` | ||
We have an issue here: command-line-args will assume we are setting two options (`--search` and `-f`). In actuality, we are passing one option (`--search`) and one value (`-f`). In cases like this, avoid ambiguity by using `--option=value` notation: | ||
``` | ||
$ grep-tool --search=-f | ||
``` | ||
### Partial parsing | ||
By default, if the user sets an option without a valid [definition](#exp_module_definition--OptionDefinition) an `UNKNOWN_OPTION` exception is thrown. However, in some cases you may only be interested in a subset of the options wishing to pass the remainder to another library. See [here](https://github.com/75lb/command-line-args/blob/master/example/mocha.js) for a example showing where this might be necessary. | ||
To enable partial parsing, set `partial: true` in the method options: | ||
```js | ||
const optionDefinitions = [ | ||
{ name: 'value', type: Number } | ||
] | ||
const options = commandLineArgs(optionDefinitions, { partial: true }) | ||
``` | ||
Now, should any unknown args be passed at the command line: | ||
``` | ||
$ example --milk --value 2 --bread cheese | ||
``` | ||
They will be returned in the `_unknown` property of the `commandLineArgs` output with no exceptions thrown: | ||
```js | ||
{ | ||
value: 2, | ||
_unknown: [ '--milk', '--bread', 'cheese'] | ||
} | ||
``` | ||
## Install | ||
@@ -128,252 +77,4 @@ | ||
# API Reference | ||
<a name="exp_module_command-line-args--commandLineArgs"></a> | ||
### commandLineArgs(optionDefinitions, [options]) ⇒ <code>object</code> ⏏ | ||
Returns an object containing all options set on the command line. By default it parses the global [`process.argv`](https://nodejs.org/api/process.html#process_process_argv) array. | ||
By default, an exception is thrown if the user sets an unknown option (one without a valid [definition](#exp_module_definition--OptionDefinition)). To enable __partial parsing__, invoke `commandLineArgs` with the `partial` option - all unknown arguments will be returned in the `_unknown` property. | ||
**Kind**: Exported function | ||
**Throws**: | ||
- `UNKNOWN_OPTION` if `options.partial` is false and the user set an undefined option | ||
- `NAME_MISSING` if an option definition is missing the required `name` property | ||
- `INVALID_TYPE` if an option definition has a `type` value that's not a function | ||
- `INVALID_ALIAS` if an alias is numeric, a hyphen or a length other than 1 | ||
- `DUPLICATE_NAME` if an option definition name was used more than once | ||
- `DUPLICATE_ALIAS` if an option definition alias was used more than once | ||
- `DUPLICATE_DEFAULT_OPTION` if more than one option definition has `defaultOption: true` | ||
| Param | Type | Description | | ||
| --- | --- | --- | | ||
| optionDefinitions | <code>[Array.<definition>](#module_definition)</code> | An array of [OptionDefinition](#exp_module_definition--OptionDefinition) objects | | ||
| [options] | <code>object</code> | Options. | | ||
| [options.argv] | <code>Array.<string></code> | An array of strings, which if passed will be parsed instead of `process.argv`. | | ||
| [options.partial] | <code>boolean</code> | If `true`, an array of unknown arguments is returned in the `_unknown` property of the output. | | ||
<a name="exp_module_definition--OptionDefinition"></a> | ||
## OptionDefinition ⏏ | ||
Describes a command-line option. Additionally, you can add `description` and `typeLabel` properties and make use of [command-line-usage](https://github.com/75lb/command-line-usage). | ||
**Kind**: Exported class | ||
* [OptionDefinition](#exp_module_definition--OptionDefinition) ⏏ | ||
* [.name](#module_definition--OptionDefinition.OptionDefinition+name) : <code>string</code> | ||
* [.type](#module_definition--OptionDefinition.OptionDefinition+type) : <code>function</code> | ||
* [.alias](#module_definition--OptionDefinition.OptionDefinition+alias) : <code>string</code> | ||
* [.multiple](#module_definition--OptionDefinition.OptionDefinition+multiple) : <code>boolean</code> | ||
* [.defaultOption](#module_definition--OptionDefinition.OptionDefinition+defaultOption) : <code>boolean</code> | ||
* [.defaultValue](#module_definition--OptionDefinition.OptionDefinition+defaultValue) : <code>\*</code> | ||
* [.group](#module_definition--OptionDefinition.OptionDefinition+group) : <code>string</code> \| <code>Array.<string></code> | ||
<a name="module_definition--OptionDefinition.OptionDefinition+name"></a> | ||
### option.name : <code>string</code> | ||
The only required definition property is `name`, so the simplest working example is | ||
```js | ||
[ | ||
{ name: "file" }, | ||
{ name: "verbose" }, | ||
{ name: "depth"} | ||
] | ||
``` | ||
In this case, the value of each option will be either a Boolean or string. | ||
| # | Command line args | .parse() output | | ||
| --- | -------------------- | ------------ | | ||
| 1 | `--file` | `{ file: true }` | | ||
| 2 | `--file lib.js --verbose` | `{ file: "lib.js", verbose: true }` | | ||
| 3 | `--verbose very` | `{ verbose: "very" }` | | ||
| 4 | `--depth 2` | `{ depth: "2" }` | | ||
Unicode option names and aliases are valid, for example: | ||
```js | ||
[ | ||
{ name: 'один' }, | ||
{ name: '两' }, | ||
{ name: 'три', alias: 'т' } | ||
] | ||
``` | ||
**Kind**: instance property of <code>[OptionDefinition](#exp_module_definition--OptionDefinition)</code> | ||
<a name="module_definition--OptionDefinition.OptionDefinition+type"></a> | ||
### option.type : <code>function</code> | ||
The `type` value is a setter function (you receive the output from this), enabling you to be specific about the type and value received. | ||
You can use a class, if you like: | ||
```js | ||
const fs = require('fs') | ||
function FileDetails(filename){ | ||
if (!(this instanceof FileDetails)) return new FileDetails(filename) | ||
this.filename = filename | ||
this.exists = fs.existsSync(filename) | ||
} | ||
const cli = commandLineArgs([ | ||
{ name: 'file', type: FileDetails }, | ||
{ name: 'depth', type: Number } | ||
]) | ||
``` | ||
| # | Command line args| .parse() output | | ||
| --- | ----------------- | ------------ | | ||
| 1 | `--file asdf.txt` | `{ file: { filename: 'asdf.txt', exists: false } }` | | ||
The `--depth` option expects a `Number`. If no value was set, you will receive `null`. | ||
| # | Command line args | .parse() output | | ||
| --- | ----------------- | ------------ | | ||
| 2 | `--depth` | `{ depth: null }` | | ||
| 3 | `--depth 2` | `{ depth: 2 }` | | ||
**Kind**: instance property of <code>[OptionDefinition](#exp_module_definition--OptionDefinition)</code> | ||
**Default**: <code>String</code> | ||
<a name="module_definition--OptionDefinition.OptionDefinition+alias"></a> | ||
### option.alias : <code>string</code> | ||
getopt-style short option names. Can be any single character (unicode included) except a digit or hypen. | ||
```js | ||
[ | ||
{ name: "hot", alias: "h", type: Boolean }, | ||
{ name: "discount", alias: "d", type: Boolean }, | ||
{ name: "courses", alias: "c" , type: Number } | ||
] | ||
``` | ||
| # | Command line | .parse() output | | ||
| --- | ------------ | ------------ | | ||
| 1 | `-hcd` | `{ hot: true, courses: null, discount: true }` | | ||
| 2 | `-hdc 3` | `{ hot: true, discount: true, courses: 3 }` | | ||
**Kind**: instance property of <code>[OptionDefinition](#exp_module_definition--OptionDefinition)</code> | ||
<a name="module_definition--OptionDefinition.OptionDefinition+multiple"></a> | ||
### option.multiple : <code>boolean</code> | ||
Set this flag if the option takes a list of values. You will receive an array of values, each passed through the `type` function (if specified). | ||
```js | ||
[ | ||
{ name: "files", type: String, multiple: true } | ||
] | ||
``` | ||
| # | Command line | .parse() output | | ||
| --- | ------------ | ------------ | | ||
| 1 | `--files one.js two.js` | `{ files: [ 'one.js', 'two.js' ] }` | | ||
| 2 | `--files one.js --files two.js` | `{ files: [ 'one.js', 'two.js' ] }` | | ||
| 3 | `--files *` | `{ files: [ 'one.js', 'two.js' ] }` | | ||
**Kind**: instance property of <code>[OptionDefinition](#exp_module_definition--OptionDefinition)</code> | ||
<a name="module_definition--OptionDefinition.OptionDefinition+defaultOption"></a> | ||
### option.defaultOption : <code>boolean</code> | ||
Any unclaimed command-line args will be set on this option. This flag is typically set on the most commonly-used option to make for more concise usage (i.e. `$ myapp *.js` instead of `$ myapp --files *.js`). | ||
```js | ||
[ | ||
{ name: "files", type: String, multiple: true, defaultOption: true } | ||
] | ||
``` | ||
| # | Command line | .parse() output | | ||
| --- | ------------ | ------------ | | ||
| 1 | `--files one.js two.js` | `{ files: [ 'one.js', 'two.js' ] }` | | ||
| 2 | `one.js two.js` | `{ files: [ 'one.js', 'two.js' ] }` | | ||
| 3 | `*` | `{ files: [ 'one.js', 'two.js' ] }` | | ||
**Kind**: instance property of <code>[OptionDefinition](#exp_module_definition--OptionDefinition)</code> | ||
<a name="module_definition--OptionDefinition.OptionDefinition+defaultValue"></a> | ||
### option.defaultValue : <code>\*</code> | ||
An initial value for the option. | ||
```js | ||
[ | ||
{ name: "files", type: String, multiple: true, defaultValue: [ "one.js" ] }, | ||
{ name: "max", type: Number, defaultValue: 3 } | ||
] | ||
``` | ||
| # | Command line | .parse() output | | ||
| --- | ------------ | ------------ | | ||
| 1 | | `{ files: [ 'one.js' ], max: 3 }` | | ||
| 2 | `--files two.js` | `{ files: [ 'two.js' ], max: 3 }` | | ||
| 3 | `--max 4` | `{ files: [ 'one.js' ], max: 4 }` | | ||
**Kind**: instance property of <code>[OptionDefinition](#exp_module_definition--OptionDefinition)</code> | ||
<a name="module_definition--OptionDefinition.OptionDefinition+group"></a> | ||
### option.group : <code>string</code> \| <code>Array.<string></code> | ||
When your app has a large amount of options it makes sense to organise them in groups. | ||
There are two automatic groups: `_all` (contains all options) and `_none` (contains options without a `group` specified in their definition). | ||
```js | ||
[ | ||
{ name: "verbose", group: "standard" }, | ||
{ name: "help", group: [ "standard", "main" ] }, | ||
{ name: "compress", group: [ "server", "main" ] }, | ||
{ name: "static", group: "server" }, | ||
{ name: "debug" } | ||
] | ||
``` | ||
<table> | ||
<tr> | ||
<th>#</th><th>Command Line</th><th>.parse() output</th> | ||
</tr> | ||
<tr> | ||
<td>1</td><td><code>--verbose</code></td><td><pre><code> | ||
{ | ||
_all: { verbose: true }, | ||
standard: { verbose: true } | ||
} | ||
</code></pre></td> | ||
</tr> | ||
<tr> | ||
<td>2</td><td><code>--debug</code></td><td><pre><code> | ||
{ | ||
_all: { debug: true }, | ||
_none: { debug: true } | ||
} | ||
</code></pre></td> | ||
</tr> | ||
<tr> | ||
<td>3</td><td><code>--verbose --debug --compress</code></td><td><pre><code> | ||
{ | ||
_all: { | ||
verbose: true, | ||
debug: true, | ||
compress: true | ||
}, | ||
standard: { verbose: true }, | ||
server: { compress: true }, | ||
main: { compress: true }, | ||
_none: { debug: true } | ||
} | ||
</code></pre></td> | ||
</tr> | ||
<tr> | ||
<td>4</td><td><code>--compress</code></td><td><pre><code> | ||
{ | ||
_all: { compress: true }, | ||
server: { compress: true }, | ||
main: { compress: true } | ||
} | ||
</code></pre></td> | ||
</tr> | ||
</table> | ||
**Kind**: instance property of <code>[OptionDefinition](#exp_module_definition--OptionDefinition)</code> | ||
* * * | ||
© 2014-17 Lloyd Brookes \<75pound@gmail.com\>. Documented by [jsdoc-to-markdown](https://github.com/75lb/jsdoc-to-markdown). | ||
© 2014-18 Lloyd Brookes \<75pound@gmail.com\>. Documented by [jsdoc-to-markdown](https://github.com/75lb/jsdoc-to-markdown). |
@@ -8,10 +8,6 @@ 'use strict' | ||
const optionDefinitions = [ | ||
{ name: 'verbose', alias: 'v' }, | ||
{ name: 'colour', alias: 'c' }, | ||
{ name: 'number', alias: 'n' }, | ||
{ name: 'dry-run', alias: 'd' } | ||
] | ||
runner.test('alias: one boolean', function () { | ||
runner.test('alias: one string alias', function () { | ||
const optionDefinitions = [ | ||
{ name: 'verbose', alias: 'v' } | ||
] | ||
const argv = [ '-v' ] | ||
@@ -23,6 +19,9 @@ a.deepStrictEqual(commandLineArgs(optionDefinitions, { argv }), { | ||
runner.test('alias: one --this-type boolean', function () { | ||
runner.test('alias: one boolean alias', function () { | ||
const optionDefinitions = [ | ||
{ name: 'dry-run', alias: 'd', type: Boolean } | ||
] | ||
const argv = [ '-d' ] | ||
a.deepStrictEqual(commandLineArgs(optionDefinitions, { argv }), { | ||
'dry-run': null | ||
'dry-run': true | ||
}) | ||
@@ -32,7 +31,11 @@ }) | ||
runner.test('alias: one boolean, one string', function () { | ||
const optionDefinitions = [ | ||
{ name: 'verbose', alias: 'v', type: Boolean }, | ||
{ name: 'colour', alias: 'c' } | ||
] | ||
const argv = [ '-v', '-c' ] | ||
a.deepStrictEqual(commandLineArgs(optionDefinitions, { argv }), { | ||
verbose: null, | ||
verbose: true, | ||
colour: null | ||
}) | ||
}) |
@@ -8,3 +8,3 @@ 'use strict' | ||
runner.test('ambiguous input: value looks like option', function () { | ||
runner.test('ambiguous input: value looks like an option 1', function () { | ||
const optionDefinitions = [ | ||
@@ -16,8 +16,28 @@ { name: 'colour', type: String, alias: 'c' } | ||
}) | ||
a.throws(function () { | ||
commandLineArgs(optionDefinitions, { argv: [ '--colour', '--red' ] }) | ||
}) | ||
}) | ||
runner.test('ambiguous input: value looks like an option 2', function () { | ||
const optionDefinitions = [ | ||
{ name: 'colour', type: String, alias: 'c' } | ||
] | ||
const argv = [ '--colour', '--red' ] | ||
a.throws( | ||
() => commandLineArgs(optionDefinitions, { argv }), | ||
err => err.name === 'UNKNOWN_OPTION' | ||
) | ||
}) | ||
runner.test('ambiguous input: value looks like an option 3', function () { | ||
const optionDefinitions = [ | ||
{ name: 'colour', type: String, alias: 'c' } | ||
] | ||
a.doesNotThrow(function () { | ||
commandLineArgs(optionDefinitions, { argv: [ '--colour=--red' ] }) | ||
}) | ||
}) | ||
runner.test('ambiguous input: value looks like an option 4', function () { | ||
const optionDefinitions = [ | ||
{ name: 'colour', type: String, alias: 'c' } | ||
] | ||
a.deepStrictEqual(commandLineArgs(optionDefinitions, { argv: [ '--colour=--red' ] }), { | ||
@@ -24,0 +44,0 @@ colour: '--red' |
@@ -8,11 +8,11 @@ 'use strict' | ||
runner.test('bad-input: handles missing option value', function () { | ||
const definitions = [ | ||
runner.test('bad-input: missing option value should be null', function () { | ||
const optionDefinitions = [ | ||
{ name: 'colour', type: String }, | ||
{ name: 'files' } | ||
] | ||
a.deepStrictEqual(commandLineArgs(definitions, { argv: [ '--colour' ] }), { | ||
a.deepStrictEqual(commandLineArgs(optionDefinitions, { argv: [ '--colour' ] }), { | ||
colour: null | ||
}) | ||
a.deepStrictEqual(commandLineArgs(definitions, { argv: [ '--colour', '--files', 'yeah' ] }), { | ||
a.deepStrictEqual(commandLineArgs(optionDefinitions, { argv: [ '--colour', '--files', 'yeah' ] }), { | ||
colour: null, | ||
@@ -24,7 +24,7 @@ files: 'yeah' | ||
runner.test('bad-input: handles arrays with relative paths', function () { | ||
const definitions = [ | ||
const optionDefinitions = [ | ||
{ name: 'colours', type: String, multiple: true } | ||
] | ||
const argv = [ '--colours', '../what', '../ever' ] | ||
a.deepStrictEqual(commandLineArgs(definitions, { argv }), { | ||
a.deepStrictEqual(commandLineArgs(optionDefinitions, { argv }), { | ||
colours: [ '../what', '../ever' ] | ||
@@ -34,4 +34,4 @@ }) | ||
runner.test('bad-input: empty string', function () { | ||
const definitions = [ | ||
runner.test('bad-input: empty string added to unknown values', function () { | ||
const optionDefinitions = [ | ||
{ name: 'one', type: String }, | ||
@@ -44,3 +44,6 @@ { name: 'two', type: Number }, | ||
const argv = [ '--one', '', '', '--two', '0', '--three=', '', '--four=', '--five=' ] | ||
a.deepStrictEqual(commandLineArgs(definitions, { argv }), { | ||
a.throws(() => { | ||
commandLineArgs(optionDefinitions, { argv }) | ||
}) | ||
a.deepStrictEqual(commandLineArgs(optionDefinitions, { argv, partial: true }), { | ||
one: '', | ||
@@ -50,3 +53,4 @@ two: 0, | ||
four: '', | ||
five: true | ||
five: true, | ||
_unknown: [ '', '--five=' ] | ||
}) | ||
@@ -56,8 +60,8 @@ }) | ||
runner.test('bad-input: non-strings in argv', function () { | ||
const definitions = [ | ||
const optionDefinitions = [ | ||
{ name: 'one', type: Number } | ||
] | ||
const argv = [ '--one', 1 ] | ||
const result = commandLineArgs(definitions, { argv }) | ||
const result = commandLineArgs(optionDefinitions, { argv }) | ||
a.deepStrictEqual(result, { one: 1 }) | ||
}) |
@@ -8,12 +8,2 @@ 'use strict' | ||
runner.test('defaultOption: string', function () { | ||
const optionDefinitions = [ | ||
{ name: 'files', defaultOption: true } | ||
] | ||
const argv = [ 'file1', 'file2' ] | ||
a.deepStrictEqual(commandLineArgs(optionDefinitions, { argv }), { | ||
files: 'file1' | ||
}) | ||
}) | ||
runner.test('defaultOption: multiple string', function () { | ||
@@ -40,3 +30,3 @@ const optionDefinitions = [ | ||
runner.test('defaultOption: multiple defaultOption values between other arg/value pairs', function () { | ||
runner.test('defaultOption: multiple-defaultOption values spread out', function () { | ||
const optionDefinitions = [ | ||
@@ -55,3 +45,3 @@ { name: 'one' }, | ||
runner.test('defaultOption: multiple defaultOption values between other arg/value pairs 2', function () { | ||
runner.test('defaultOption: multiple-defaultOption values spread out 2', function () { | ||
const optionDefinitions = [ | ||
@@ -69,35 +59,1 @@ { name: 'one', type: Boolean }, | ||
}) | ||
runner.test('defaultOption: floating args present but no defaultOption', function () { | ||
const definitions = [ | ||
{ name: 'one', type: Boolean } | ||
] | ||
a.deepStrictEqual( | ||
commandLineArgs(definitions, { argv: [ 'aaa', '--one', 'aaa', 'aaa' ] }), | ||
{ one: true } | ||
) | ||
}) | ||
runner.test('defaultOption: non-multiple should take first value', function () { | ||
const optionDefinitions = [ | ||
{ name: 'file', defaultOption: true } | ||
] | ||
const argv = [ 'file1', 'file2' ] | ||
a.deepStrictEqual(commandLineArgs(optionDefinitions, { argv }), { | ||
file: 'file1' | ||
}) | ||
}) | ||
runner.test('defaultOption: non-multiple should take first value 2', function () { | ||
const optionDefinitions = [ | ||
{ name: 'file', defaultOption: true }, | ||
{ name: 'one', type: Boolean }, | ||
{ name: 'two', type: Boolean } | ||
] | ||
const argv = [ '--two', 'file1', '--one', 'file2' ] | ||
a.deepStrictEqual(commandLineArgs(optionDefinitions, { argv }), { | ||
file: 'file1', | ||
two: true, | ||
one: true | ||
}) | ||
}) |
@@ -9,7 +9,7 @@ 'use strict' | ||
runner.test('default value', function () { | ||
let defs = [ | ||
const defs = [ | ||
{ name: 'one' }, | ||
{ name: 'two', defaultValue: 'two' } | ||
] | ||
let argv = [ '--one', '1' ] | ||
const argv = [ '--one', '1' ] | ||
a.deepStrictEqual(commandLineArgs(defs, { argv }), { | ||
@@ -19,19 +19,28 @@ one: '1', | ||
}) | ||
}) | ||
defs = [ { name: 'two', defaultValue: 'two' } ] | ||
argv = [] | ||
runner.test('default value 2', function () { | ||
const defs = [ { name: 'two', defaultValue: 'two' } ] | ||
const argv = [] | ||
a.deepStrictEqual(commandLineArgs(defs, { argv }), { two: 'two' }) | ||
}) | ||
defs = [ { name: 'two', defaultValue: 'two' } ] | ||
argv = [ '--two', 'zwei' ] | ||
runner.test('default value 3', function () { | ||
const defs = [ { name: 'two', defaultValue: 'two' } ] | ||
const argv = [ '--two', 'zwei' ] | ||
a.deepStrictEqual(commandLineArgs(defs, { argv }), { two: 'zwei' }) | ||
}) | ||
defs = [ { name: 'two', multiple: true, defaultValue: [ 'two', 'zwei' ] } ] | ||
argv = [ '--two', 'duo' ] | ||
runner.test('default value 4', function () { | ||
const defs = [ { name: 'two', multiple: true, defaultValue: [ 'two', 'zwei' ] } ] | ||
const argv = [ '--two', 'duo' ] | ||
a.deepStrictEqual(commandLineArgs(defs, { argv }), { two: [ 'duo' ] }) | ||
}) | ||
runner.test('default value 2', function () { | ||
const defs = [{ name: 'two', multiple: true, defaultValue: ['two', 'zwei'] }] | ||
const result = commandLineArgs(defs, []) | ||
runner.test('default value 5', function () { | ||
const defs = [ | ||
{ name: 'two', multiple: true, defaultValue: ['two', 'zwei'] } | ||
] | ||
const argv = [] | ||
const result = commandLineArgs(defs, { argv }) | ||
a.deepStrictEqual(result, { two: [ 'two', 'zwei' ] }) | ||
@@ -38,0 +47,0 @@ }) |
@@ -10,2 +10,9 @@ 'use strict' | ||
process.argv = [ 'node', 'filename', '--one', 'eins' ] | ||
a.deepStrictEqual(commandLineArgs({ name: 'one' }), { | ||
one: 'eins' | ||
}) | ||
}) | ||
runner.test('detect process.argv: should automatically remove first two argv items 2', function () { | ||
process.argv = [ 'node', 'filename', '--one', 'eins' ] | ||
a.deepStrictEqual(commandLineArgs({ name: 'one' }, { argv: process.argv }), { | ||
@@ -12,0 +19,0 @@ one: 'eins' |
@@ -8,8 +8,17 @@ 'use strict' | ||
runner.test('multiple: string unset', function () { | ||
const argv = [] | ||
runner.test('multiple: empty argv', function () { | ||
const optionDefinitions = [ | ||
{ name: 'one', multiple: true } | ||
] | ||
const argv = [] | ||
const result = commandLineArgs(optionDefinitions, { argv }) | ||
a.deepStrictEqual(result, {}) | ||
}) | ||
runner.test('multiple: boolean, empty argv', function () { | ||
const optionDefinitions = [ | ||
{ name: 'one', type: Boolean, multiple: true } | ||
] | ||
const argv = [] | ||
const result = commandLineArgs(optionDefinitions, { argv }) | ||
a.deepStrictEqual(result, { }) | ||
@@ -19,17 +28,52 @@ }) | ||
runner.test('multiple: string unset with defaultValue', function () { | ||
const argv = [] | ||
const optionDefinitions = [ | ||
{ name: 'one', multiple: true, defaultValue: 1 } | ||
] | ||
const argv = [] | ||
const result = commandLineArgs(optionDefinitions, { argv }) | ||
a.deepStrictEqual(result, { one: [ 1 ]}) | ||
a.deepStrictEqual(result, { one: [ 1 ] }) | ||
}) | ||
runner.test('multiple: boolean unset', function () { | ||
const argv = [] | ||
runner.test('multiple: string', function () { | ||
const optionDefinitions = [ | ||
{ name: 'one', type: Boolean, multiple: true } | ||
{ name: 'one', multiple: true } | ||
] | ||
const argv = [ '--one', '1', '2' ] | ||
const result = commandLineArgs(optionDefinitions, { argv }) | ||
a.deepStrictEqual(result, { }) | ||
a.deepStrictEqual(result, { | ||
one: [ '1', '2' ] | ||
}) | ||
}) | ||
runner.test('multiple: string, --option=value', function () { | ||
const optionDefinitions = [ | ||
{ name: 'one', multiple: true } | ||
] | ||
const argv = [ '--one=1', '--one=2' ] | ||
const result = commandLineArgs(optionDefinitions, { argv }) | ||
a.deepStrictEqual(result, { | ||
one: [ '1', '2' ] | ||
}) | ||
}) | ||
runner.test('multiple: string, --option=value mix', function () { | ||
const optionDefinitions = [ | ||
{ name: 'one', multiple: true } | ||
] | ||
const argv = [ '--one=1', '--one=2', '3' ] | ||
const result = commandLineArgs(optionDefinitions, { argv }) | ||
a.deepStrictEqual(result, { | ||
one: [ '1', '2', '3' ] | ||
}) | ||
}) | ||
runner.test('multiple: string, defaultOption', function () { | ||
const optionDefinitions = [ | ||
{ name: 'one', multiple: true, defaultOption: true } | ||
] | ||
const argv = [ '1', '2' ] | ||
const result = commandLineArgs(optionDefinitions, { argv }) | ||
a.deepStrictEqual(result, { | ||
one: [ '1', '2' ] | ||
}) | ||
}) |
@@ -8,10 +8,9 @@ 'use strict' | ||
const optionDefinitions = [ | ||
{ name: 'one', alias: 'o' }, | ||
{ name: 'two', alias: 't' }, | ||
{ name: 'three', alias: 'h' }, | ||
{ name: 'four', alias: 'f' } | ||
] | ||
runner.test('name-alias-mix: one of each', function () { | ||
const optionDefinitions = [ | ||
{ name: 'one', alias: 'o' }, | ||
{ name: 'two', alias: 't' }, | ||
{ name: 'three', alias: 'h' }, | ||
{ name: 'four', alias: 'f' } | ||
] | ||
const argv = [ '--one', '-t', '--three' ] | ||
@@ -18,0 +17,0 @@ const result = commandLineArgs(optionDefinitions, { argv }) |
@@ -8,9 +8,8 @@ 'use strict' | ||
const optionDefinitions = [ | ||
{ name: 'один' }, | ||
{ name: '两' }, | ||
{ name: 'три', alias: 'т' } | ||
] | ||
runner.test('name-unicode: unicode names and aliases are permitted', function () { | ||
const optionDefinitions = [ | ||
{ name: 'один' }, | ||
{ name: '两' }, | ||
{ name: 'три', alias: 'т' } | ||
] | ||
const argv = [ '--один', '1', '--两', '2', '-т', '3' ] | ||
@@ -17,0 +16,0 @@ const result = commandLineArgs(optionDefinitions, { argv }) |
@@ -32,4 +32,42 @@ 'use strict' | ||
runner.test('partial: defaultOption 2', function () { | ||
runner.test('defaultOption: floating args present but no defaultOption', function () { | ||
const definitions = [ | ||
{ name: 'one', type: Boolean } | ||
] | ||
a.deepStrictEqual( | ||
commandLineArgs(definitions, { argv: [ 'aaa', '--one', 'aaa', 'aaa' ], partial: true }), | ||
{ | ||
one: true, | ||
_unknown: [ 'aaa', 'aaa', 'aaa' ] | ||
} | ||
) | ||
}) | ||
runner.test('partial: combined short option, both unknown', function () { | ||
const definitions = [ | ||
{ name: 'one', alias: 'o' }, | ||
{ name: 'two', alias: 't' } | ||
] | ||
const argv = [ '-ab' ] | ||
const options = commandLineArgs(definitions, { argv, partial: true }) | ||
a.deepStrictEqual(options, { | ||
_unknown: [ '-a', '-b' ] | ||
}) | ||
}) | ||
runner.test('partial: combined short option, one known, one unknown', function () { | ||
const definitions = [ | ||
{ name: 'one', alias: 'o' }, | ||
{ name: 'two', alias: 't' } | ||
] | ||
const argv = [ '-ob' ] | ||
const options = commandLineArgs(definitions, { argv, partial: true }) | ||
a.deepStrictEqual(options, { | ||
one: null, | ||
_unknown: [ '-b' ] | ||
}) | ||
}) | ||
runner.test('partial: defaultOption with --option=value and combined short options', function () { | ||
const definitions = [ | ||
{ name: 'files', type: String, defaultOption: true, multiple: true }, | ||
@@ -57,15 +95,39 @@ { name: 'one', type: Boolean }, | ||
file: 'file1', | ||
_unknown: [ '--two', '3', '--four', '5' ] | ||
_unknown: [ '--two=3', '--four', '5' ] | ||
}) | ||
}) | ||
runner.test('partial: defaultOption with value equal to defaultValue 2', function () { | ||
runner.test('partial: string defaultOption can be set by argv once', function () { | ||
const definitions = [ | ||
{ name: 'file', type: String, defaultOption: true, defaultValue: 'file1' } | ||
] | ||
const argv = [ '--file', '--file=file1', '--two=3', '--four', '5' ] | ||
const argv = [ '--file', '--file=file2', '--two=3', '--four', '5' ] | ||
const options = commandLineArgs(definitions, { argv, partial: true }) | ||
a.deepStrictEqual(options, { | ||
file: 'file2', | ||
_unknown: [ '--two=3', '--four', '5' ] | ||
}) | ||
}) | ||
runner.test('partial: string defaultOption can not be set by argv twice', function () { | ||
const definitions = [ | ||
{ name: 'file', type: String, defaultOption: true, defaultValue: 'file1' } | ||
] | ||
const argv = [ '--file', '--file=file2', '--two=3', '--four', '5', 'file3' ] | ||
const options = commandLineArgs(definitions, { argv, partial: true }) | ||
a.deepStrictEqual(options, { | ||
file: 'file2', | ||
_unknown: [ '--two=3', '--four', '5', 'file3' ] | ||
}) | ||
}) | ||
runner.test('partial: defaultOption with value equal to defaultValue 3', function () { | ||
const definitions = [ | ||
{ name: 'file', type: String, defaultOption: true, defaultValue: 'file1' } | ||
] | ||
const argv = [ 'file1', 'file2', '--two=3', '--four', '5' ] | ||
const options = commandLineArgs(definitions, { argv, partial: true }) | ||
a.deepStrictEqual(options, { | ||
file: 'file1', | ||
_unknown: [ '--two', '3', '--four', '5' ] | ||
_unknown: [ 'file2', '--two=3', '--four', '5' ] | ||
}) | ||
@@ -203,1 +265,12 @@ }) | ||
}) | ||
runner.test('defaultOption: single string', function () { | ||
const optionDefinitions = [ | ||
{ name: 'files', defaultOption: true } | ||
] | ||
const argv = [ 'file1', 'file2' ] | ||
a.deepStrictEqual(commandLineArgs(optionDefinitions, { argv, partial: true }), { | ||
files: 'file1', | ||
_unknown: [ 'file2' ] | ||
}) | ||
}) |
@@ -8,4 +8,4 @@ 'use strict' | ||
runner.test('type-boolean: different values', function () { | ||
const definitions = [ | ||
runner.test('type-boolean: simple', function () { | ||
const optionDefinitions = [ | ||
{ name: 'one', type: Boolean } | ||
@@ -15,17 +15,5 @@ ] | ||
a.deepStrictEqual( | ||
commandLineArgs(definitions, { argv: [ '--one' ] }), | ||
commandLineArgs(optionDefinitions, { argv: [ '--one' ] }), | ||
{ one: true } | ||
) | ||
a.deepStrictEqual( | ||
commandLineArgs(definitions, { argv: [ '--one', 'true' ] }), | ||
{ one: true } | ||
) | ||
a.deepStrictEqual( | ||
commandLineArgs(definitions, { argv: [ '--one', 'false' ] }), | ||
{ one: true } | ||
) | ||
a.deepStrictEqual( | ||
commandLineArgs(definitions, { argv: [ '--one', 'sfsgf' ] }), | ||
{ one: true } | ||
) | ||
}) | ||
@@ -41,3 +29,3 @@ | ||
const definitions = [ | ||
const optionDefinitions = [ | ||
{ name: 'one', type: Boolean } | ||
@@ -47,25 +35,13 @@ ] | ||
a.deepStrictEqual( | ||
commandLineArgs(definitions, { argv: [ '--one', 'true' ] }), | ||
commandLineArgs(optionDefinitions, { argv: [ '--one' ] }), | ||
{ one: true } | ||
) | ||
a.deepStrictEqual( | ||
commandLineArgs(definitions, { argv: [ '--one', 'false' ] }), | ||
{ one: true } | ||
) | ||
a.deepStrictEqual( | ||
commandLineArgs(definitions, { argv: [ '--one', 'sfsgf' ] }), | ||
{ one: true } | ||
) | ||
a.deepStrictEqual( | ||
commandLineArgs(definitions, { argv: [ '--one' ] }), | ||
{ one: true } | ||
) | ||
}) | ||
runner.test('type-boolean-multiple: 1', function () { | ||
const definitions = [ | ||
const optionDefinitions = [ | ||
{ name: 'array', type: Boolean, multiple: true } | ||
] | ||
const argv = [ '--array', '--array', '--array' ] | ||
const result = commandLineArgs(definitions, { argv }) | ||
const result = commandLineArgs(optionDefinitions, { argv }) | ||
a.deepStrictEqual(result, { | ||
@@ -72,0 +48,0 @@ array: [ true, true, true ] |
@@ -6,21 +6,20 @@ 'use strict' | ||
const definitions = [ | ||
{ name: 'one', type: String } | ||
] | ||
const runner = new TestRunner() | ||
runner.test('type-string: different values', function () { | ||
const optionDefinitions = [ | ||
{ name: 'one', type: String } | ||
] | ||
a.deepStrictEqual( | ||
commandLineArgs(definitions, { argv: [ '--one', 'yeah' ] }), | ||
commandLineArgs(optionDefinitions, { argv: [ '--one', 'yeah' ] }), | ||
{ one: 'yeah' } | ||
) | ||
a.deepStrictEqual( | ||
commandLineArgs(definitions, { argv: [ '--one' ] }), | ||
commandLineArgs(optionDefinitions, { argv: [ '--one' ] }), | ||
{ one: null } | ||
) | ||
a.deepStrictEqual( | ||
commandLineArgs(definitions, { argv: [ '--one', '3' ] }), | ||
commandLineArgs(optionDefinitions, { argv: [ '--one', '3' ] }), | ||
{ one: '3' } | ||
) | ||
}) |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
No v1
QualityPackage is not semver >=1. This means it is not stable and does not support ^ ranges.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Dynamic require
Supply chain riskDynamic require can indicate the package is performing dangerous or unsafe dynamic code execution.
Found 1 instance in 1 package
Filesystem access
Supply chain riskAccesses the file system, and could potentially read sensitive data.
Found 1 instance in 1 package
106764
46
2788
1
4
2
76
1
+ Addedlodash.camelcase@^4.3.0
+ Addedfind-replace@2.0.1(transitive)
+ Addedlodash.camelcase@4.3.0(transitive)
+ Addedtest-value@3.0.0(transitive)
- Removedarray-back@1.0.4(transitive)
- Removedfind-replace@1.0.3(transitive)
- Removedtest-value@2.1.0(transitive)
Updatedfind-replace@^2.0.1