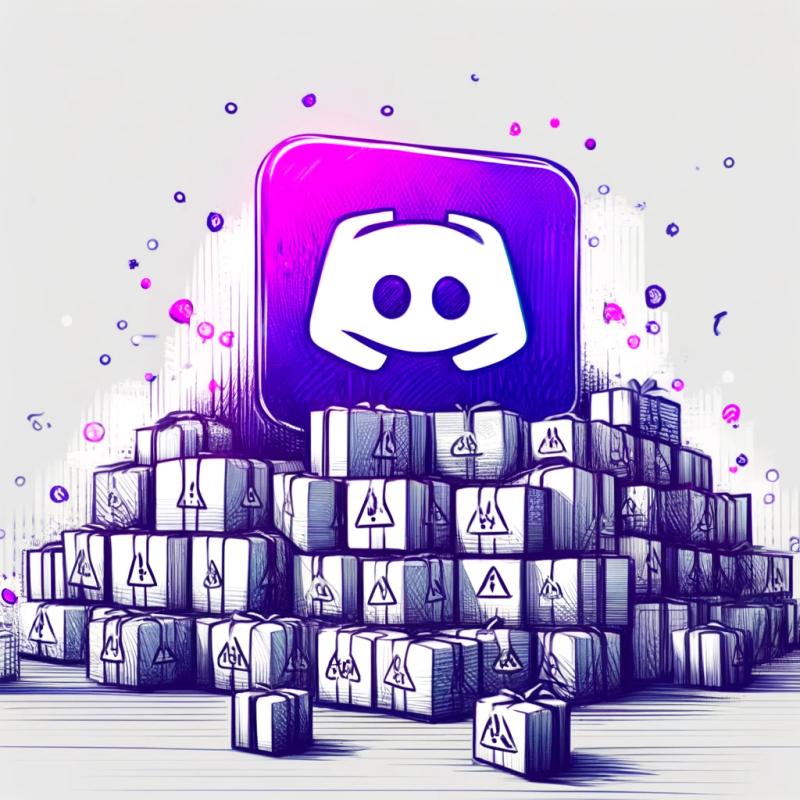
Research
Recent Trends in Malicious Packages Targeting Discord
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
compile-mime-match
Advanced tools
Readme
Compiles a function that matches a given MIME type. A faster alternative to type-is
for when the MIME type is known ahead of time.
// npm
npm install compile-mime-match --save
// yarn
yarn add compile-mime-match
const compileMimeMatch = require('compile-mime-match');
const mimeMatch = compileMimeMatch('application/json');
mimeMatch('application/json'); // true
mimeMatch('application/json; charset=utf-8'); // true
mimeMatch('text/plain'); // false
type/subtype
Matches exactly the given MIME type (see the example above).
type/*
Matches any MIME type will the given type
.
const compileMimeMatch = require('compile-mime-match');
const mimeMatch = compileMimeMatch('text/*');
mimeMatch('text/plain'); // true
mimeMatch('text/html'); // true
mimeMatch('image/png'); // false
*/subtype
Matches any MIME type will the given subtype
.
const compileMimeMatch = require('compile-mime-match');
const mimeMatch = compileMimeMatch('*/xml');
mimeMatch('application/xml'); // true
mimeMatch('text/xml'); // true
mimeMatch('image/svg+xml'); // false
*/*
Matches any valid MIME type.
const compileMimeMatch = require('compile-mime-match');
const mimeMatch = compileMimeMatch('*/*');
mimeMatch('image/png'); // true
mimeMatch('application/x-www-form-urlencoded'); // true
mimeMatch('invalid'); // false
mimeMatch('/'); // false
+suffix
Matches any valid MIME type that ends with +suffix
.
const compileMimeMatch = require('compile-mime-match');
const mimeMatch = compileMimeMatch('+json');
mimeMatch('application/calendar+json'); // true
mimeMatch('application/geo+json'); // true
mimeMatch('application/json'); // false
mimeMatch('invalid+json'); // false
compile-mime-match
also accepts an array of strings to match multiple types at the same time:
const compileMimeMatch = require('compile-mime-match');
const mimeMatch = compileMimeMatch(['application/json', 'text/*']);
mimeMatch('application/json'); // true
mimeMatch('application/json; charset=utf-8'); // true
mimeMatch('text/plain'); // true
mimeMatch('text/html'); // true
mimeMatch('image/png'); // false
FAQs
Compiles a function that matches a given MIME type.
We found that compile-mime-match demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
Security News
Socket CEO Feross Aboukhadijeh joins a16z partners to discuss how modern, sophisticated supply chain attacks require AI-driven defenses and explore the challenges and solutions in leveraging AI for threat detection early in the development life cycle.
Security News
NIST's new AI Risk Management Framework aims to enhance the security and reliability of generative AI systems and address the unique challenges of malicious AI exploits.