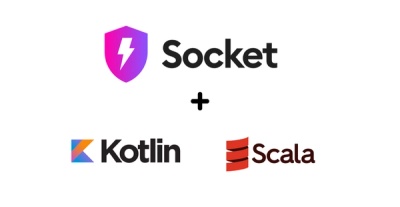
Product
Introducing Scala and Kotlin Support in Socket
Socket now supports Scala and Kotlin, bringing AI-powered threat detection to JVM projects with easy manifest generation and fast, accurate scans.
compress-commons
Advanced tools
a library that defines a common interface for working with archive formats within node
The compress-commons npm package is a library that provides a set of reusable classes and functions for creating and extracting archive files. It supports various archive formats, making it a versatile tool for handling compression and decompression tasks in Node.js applications.
Creating Archive Files
This feature allows you to create archive files. The code sample demonstrates how to create a TAR archive containing a single file with the content 'Hello World!'.
const fs = require('fs');
const tar = require('compress-commons').tar;
const output = fs.createWriteStream('archive.tar');
const archive = tar.createArchiveStream();
output.on('close', function() {
console.log('Archive has been finalized.');
});
archive.pipe(output);
archive.entry('file.txt', 'Hello World!', function(err) {
if (err) throw err;
archive.finish();
});
Extracting Archive Files
This feature enables the extraction of archive files. The provided code sample shows how to extract files from a TAR archive, handling each entry and completing the process.
const fs = require('fs');
const tar = require('compress-commons').tar;
const input = fs.createReadStream('archive.tar');
const archive = tar.extractArchiveStream();
input.pipe(archive);
archive.on('entry', function(header, stream, next) {
stream.on('end', next);
stream.resume();
});
archive.on('finish', function() {
console.log('Extraction complete.');
});
Archiver is a popular npm package for file archiving. It supports creating and streaming archives in formats like ZIP and TAR. Compared to compress-commons, Archiver offers a high-level API that might be easier to use for common archiving tasks.
extract-zip is focused solely on extracting ZIP files. It provides a simpler interface for this specific task, making it less versatile but potentially more straightforward to use for ZIP extraction compared to the broader scope of compress-commons.
yauzl is another npm package for reading and extracting ZIP files. It offers a low-level interface for ZIP file manipulation, providing more control but requiring more code for common tasks compared to compress-commons, which offers a more balanced approach between ease of use and control.
Compress Commons is a library that defines a common interface for working with archive formats within node.
npm install compress-commons --save
You can also use npm install https://github.com/archiverjs/node-compress-commons/archive/master.tar.gz
to test upcoming versions.
Concept inspired by Apache Commons Compress™.
Some logic derived from Apache Commons Compress™ and OpenJDK 7.
FAQs
a library that defines a common interface for working with archive formats within node
The npm package compress-commons receives a total of 7,579,372 weekly downloads. As such, compress-commons popularity was classified as popular.
We found that compress-commons demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports Scala and Kotlin, bringing AI-powered threat detection to JVM projects with easy manifest generation and fast, accurate scans.
Application Security
/Security News
Socket CEO Feross Aboukhadijeh and a16z partner Joel de la Garza discuss vibe coding, AI-driven software development, and how the rise of LLMs, despite their risks, still points toward a more secure and innovative future.
Research
/Security News
Threat actors hijacked Toptal’s GitHub org, publishing npm packages with malicious payloads that steal tokens and attempt to wipe victim systems.