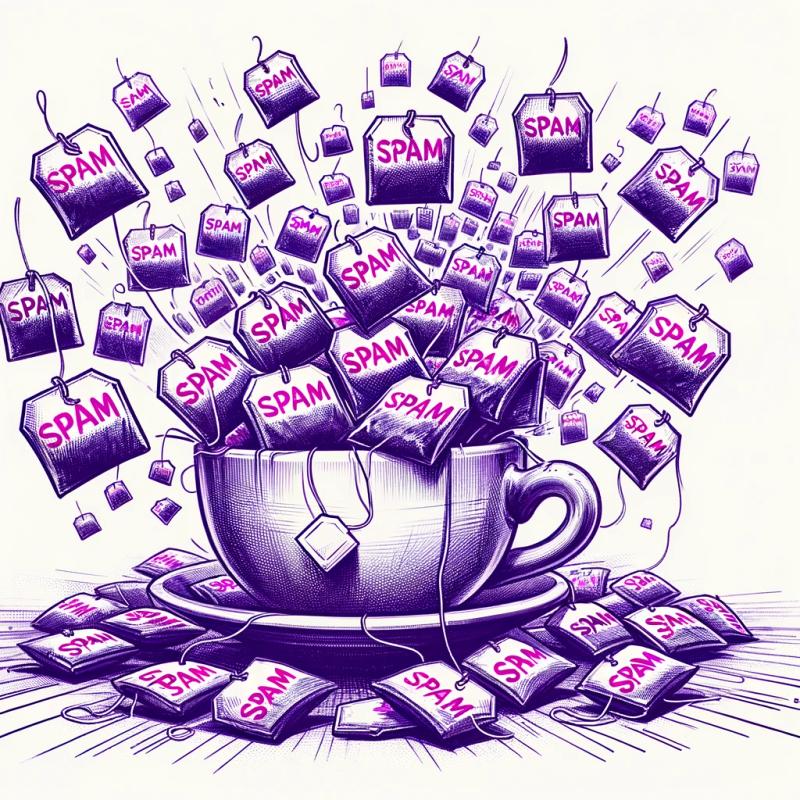
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
compressorjs
Advanced tools
Changelog
1.2.1 (Feb 28, 2023)
Readme
JavaScript image compressor. Uses the Browser's native canvas.toBlob API to do the compression work, which means it is lossy compression, asynchronous, and has different compression effects in different browsers. Generally use this to precompress a image on the client side before uploading it.
dist/
├── compressor.js (UMD)
├── compressor.min.js (UMD, compressed)
├── compressor.common.js (CommonJS, default)
└── compressor.esm.js (ES Module)
npm install compressorjs
new Compressor(file[, options])
file
The target image file for compressing.
options
Object
The options for compressing. Check out the available options.
<input type="file" id="file" accept="image/*">
import axios from 'axios';
import Compressor from 'compressorjs';
document.getElementById('file').addEventListener('change', (e) => {
const file = e.target.files[0];
if (!file) {
return;
}
new Compressor(file, {
quality: 0.6,
// The compression process is asynchronous,
// which means you have to access the `result` in the `success` hook function.
success(result) {
const formData = new FormData();
// The third parameter is required for server
formData.append('file', result, result.name);
// Send the compressed image file to server with XMLHttpRequest.
axios.post('/path/to/upload', formData).then(() => {
console.log('Upload success');
});
},
error(err) {
console.log(err.message);
},
});
});
You may set compressor options with new Compressor(file, options)
.
If you want to change the global default options, You may use Compressor.setDefaults(options)
.
boolean
true
Indicates whether to output the original image instead of the compressed one when the size of the compressed image is greater than the original one's, except the following cases:
retainExif
option is set to true
.mimeType
option is set and its value is different from the mime type of the image.width
option is set and its value is greater than the natural width of the image.height
option is set and its value is greater than the natural height of the image.minWidth
option is set and its value is greater than the natural width of the image.minHeight
option is set and its value is greater than the natural height of the image.maxWidth
option is set and its value is less than the natural width of the image.maxHeight
option is set and its value is less than the natural height of the image.boolean
true
Indicates whether to read the image's Exif Orientation value (JPEG image only), and then rotate or flip the image automatically with the value.
Notes:
boolean
false
Indicates whether to retain the image's Exif information after compressed.
number
Infinity
The max-width of the output image. The value should be greater than 0
.
Avoid getting a blank output image, you might need to set the
maxWidth
andmaxHeight
options to limited numbers, because of the size limits of a canvas element, recommend to use4096
or lesser.
number
Infinity
The max height of the output image. The value should be greater than 0
.
number
0
The min-width of the output image. The value should be greater than 0
and should not be greater than the maxWidth
.
number
0
The min-height of the output image. The value should be greater than 0
and should not be greater than the maxHeight
.
number
undefined
The width of the output image. If not specified, the natural width of the original image will be used, or if the height
option is set, the width will be computed automatically by the natural aspect ratio.
number
undefined
The height of the output image. If not specified, the natural height of the original image will be used, or if the width
option is set, the height will be computed automatically by the natural aspect ratio.
string
"none"
"none"
, "contain"
, and "cover"
.Sets how the size of the image should be resized to the container specified by the width
and height
options.
Note: This option only available when both the width
and height
options are specified.
number
0.8
The quality of the output image. It must be a number between 0
and 1
. If this argument is anything else, the default values 0.92
and 0.80
are used for image/jpeg
and image/webp
respectively. Other arguments are ignored. Be careful to use 1
as it may make the size of the output image become larger.
Note: This option only available for image/jpeg
and image/webp
images.
Check out canvas.toBlob for more detail.
Examples:
Quality | Input size | Output size | Compression ratio | Description |
---|---|---|---|---|
0 | 2.12 MB | 114.61 KB | 94.72% | - |
0.2 | 2.12 MB | 349.57 KB | 83.90% | - |
0.4 | 2.12 MB | 517.10 KB | 76.18% | - |
0.6 | 2.12 MB | 694.99 KB | 67.99% | Recommend |
0.8 | 2.12 MB | 1.14 MB | 46.41% | Recommend |
1 | 2.12 MB | 2.12 MB | 0% | Not recommend |
NaN | 2.12 MB | 2.01 MB | 5.02% | - |
string
'auto'
The mime type of the output image. By default, the original mime type of the source image file will be used.
Array
or string
(multiple types should be separated by commas)['image/png']
['image/png', 'image/webp']
'image/png,image/webp'
Files whose file type is included in this list, and whose file size exceeds the convertSize
value will be converted to JPEGs.
number
5000000
(5 MB)Files whose file type is included in the convertTypes
list, and whose file size exceeds this value will be converted to JPEGs. To disable this, just set the value to Infinity
.
Examples:
convertSize | Input size (type) | Output size (type) | Compression ratio |
---|---|---|---|
5 MB | 1.87 MB (PNG) | 1.87 MB (PNG) | 0% |
5 MB | 5.66 MB (PNG) | 450.24 KB (JPEG) | 92.23% |
5 MB | 9.74 MB (PNG) | 883.89 KB (JPEG) | 91.14% |
Function
null
context
: The 2d rendering context of the canvas.canvas
: The canvas for compression.The hook function to execute before drawing the image into the canvas for compression.
new Compressor(file, {
beforeDraw(context, canvas) {
context.fillStyle = '#fff';
context.fillRect(0, 0, canvas.width, canvas.height);
context.filter = 'grayscale(100%)';
},
});
Function
null
context
: The 2d rendering context of the canvas.canvas
: The canvas for compression.The hook function to execute after drawing the image into the canvas for compression.
new Compressor(file, {
drew(context, canvas) {
context.fillStyle = '#fff';
context.font = '2rem serif';
context.fillText('watermark', 20, canvas.height - 20);
},
});
Function
null
result
: The compressed image (a File
(read only) or Blob
object).The hook function to execute when successful to compress the image.
Function
null
err
: The compression error (an Error
object).The hook function executes when fails to compress the image.
Abort the compression process.
const compressor = new Compressor(file);
// Do something...
compressor.abort();
If you have to use another compressor with the same namespace, just call the Compressor.noConflict
static method to revert to it.
<script src="other-compressor.js"></script>
<script src="compressor.js"></script>
<script>
Compressor.noConflict();
// Code that uses other `Compressor` can follow here.
</script>
Please read through our contributing guidelines.
Maintained under the Semantic Versioning guidelines.
FAQs
JavaScript image compressor.
The npm package compressorjs receives a total of 106,444 weekly downloads. As such, compressorjs popularity was classified as popular.
We found that compressorjs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.