coolshapes-react
Advanced tools
Comparing version 0.0.5-alpha.1 to 0.0.6-alpha.0
@@ -19,2 +19,3 @@ import React, { ForwardRefExoticComponent, ComponentPropsWithRef } from 'react'; | ||
rectangle: ShapeType[]; | ||
number: ShapeType[]; | ||
}; | ||
@@ -39,3 +40,4 @@ type shapeTypes = keyof typeof shapes; | ||
declare const Polygon: React.ForwardRefExoticComponent<BaseShapeOptions>; | ||
declare const Number: React.ForwardRefExoticComponent<BaseShapeOptions>; | ||
export { Coolshape, Ellipse, Flower, Misc, Moon, Polygon, Rectangle, Star, Triangle, type shapeTypes, shapes }; | ||
export { Coolshape, Ellipse, Flower, Misc, Moon, Number, Polygon, Rectangle, Star, Triangle, type shapeTypes, shapes }; |
{ | ||
"name": "coolshapes-react", | ||
"version": "0.0.5-alpha.1", | ||
"version": "0.0.6-alpha.0", | ||
"description": "A react component library for coolshapes", | ||
@@ -18,3 +18,2 @@ "keywords": [ | ||
"./dist", | ||
"!dist/**/*.map", | ||
"LICENSE", | ||
@@ -45,3 +44,3 @@ "README.md" | ||
"type": "git", | ||
"url": "https://github.com/realvjy/coolshapes-react" | ||
"url": "git+https://github.com/realvjy/coolshapes-react.git" | ||
}, | ||
@@ -53,3 +52,3 @@ "bugs": { | ||
"engines": { | ||
"node": ">=10" | ||
"node": ">=14.0.0" | ||
}, | ||
@@ -82,2 +81,2 @@ "peerDependencies": { | ||
} | ||
} | ||
} |
206
README.md
@@ -0,1 +1,2 @@ | ||
[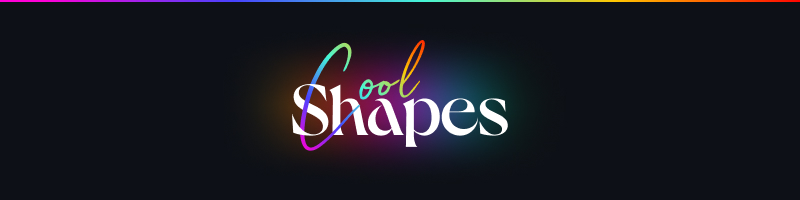](https://coolshap.es) | ||
# <p align=center>Coolshapes</p> | ||
@@ -8,38 +9,24 @@ <p align="center"> | ||
### What & Why? | ||
A simple, fun project for the sake of creating some cool-looking abstract shapes with little grainy gradients crafted by [@realvjy](https://x.com/realvjy). Coolshapes is a completely open-source set of 100+ abstract shapes crafted for any design and development projects. Free for both commercial and personal use. Licensed under MIT. | ||
## Table of Contents | ||
- [Usage](#usage) | ||
- [React](#react) | ||
### Table of Contents | ||
- [Installation](#installation) | ||
- [How to use](#how-to-use) | ||
- [Global Component](#how-to-use) | ||
- [Using Shape Category](#component-with-shape-category-example) | ||
- [Random functions](#using-random-shape-function) | ||
- [Other Methods](#other-method) | ||
- [Props](#props) | ||
- [Categories](#categories) | ||
- [Figma File](#figma-file) | ||
- [Contributing](#contributing) | ||
- [Community](#community) | ||
- [License](#license) | ||
- [License](https://github.com/realvjy/coolshapes-react?tab=MIT-1-ov-file) | ||
- [Credits](#credits) | ||
- [Sponsors](#sponsors) | ||
## Usage | ||
At its core, Coolshapes is a collection of [SVG](https://coolshap.es) files. This means that you can use this in all the same ways you can use SVGs (e.g. `img`, `background-image`, `inline`, `object`, `embed`, `iframe`). | ||
## Installation | ||
The following are ways you can use Coolshapes. | ||
# Coolshapes React | ||
Implementation of the coolshapes icon library for react applications. | ||
## How to use | ||
It's built with ES modules so it's completely tree-shakable. | ||
Each icon can be imported as a react component. | ||
Implementation of the coolshapes icon library for web applications. | ||
```sh | ||
@@ -54,6 +41,10 @@ npm install coolshapes-react | ||
``` | ||
### Example | ||
You can pass additional props to adjust the icon. | ||
## How to use | ||
There are two types of components available in **Coolshapes**, which you can use: **Global component** and **Component with a category of shapes**. Then, you can pass additional [props](#props) to adjust the shapes and their properties available on Coolshapes SVG elements. | ||
#### Global component example | ||
Just import the Global component `Coolshape` and it will work. | ||
```js | ||
@@ -63,3 +54,3 @@ import { Coolshape } from 'coolshapes-react'; | ||
const App = () => { | ||
return <Coolshape type="star" size={48} noise={true} />; | ||
return <Coolshape type="star" index={0} size={48} noise={true} />; | ||
}; | ||
@@ -70,7 +61,9 @@ | ||
#### Component with shape category example | ||
You can import the component for specific category - `Star`,`Ellipse`... etc - and simply pass the index of the shape. | ||
```js | ||
import { Star, Ellipse } from 'coolshapes-react'; | ||
import { Star } from 'coolshapes-react'; | ||
const App = () => { | ||
return <Star index="1" size={48} noise={true} />; | ||
return <Star index={0} size={48} />; | ||
}; | ||
@@ -80,23 +73,148 @@ | ||
``` | ||
#### Generating random shapes | ||
setting the `random` [prop](#props) to true or leaving the `index` or `type` prop empty would replace the shape with a random shape every time it renders. | ||
```js | ||
// renders a random shape from any category | ||
const Component = () => { | ||
return <Coolshape random={true}/>; | ||
}; | ||
// renders a shape from the category star | ||
const Component2 = () => { | ||
return <Coolshape type="star" random={true} />; | ||
}; | ||
``` | ||
#### Using random shape function | ||
```js | ||
import { getRandomShape } from 'coolshapes-react'; | ||
``` | ||
```js | ||
getRandomShape() // returns a random shape component | ||
``` | ||
```js | ||
getRandomShape({type:"ellipse"}) // returns a random shape component from the category ellipse | ||
``` | ||
```js | ||
getRandomShape({onlyId: true}) // returns shape identifier that can passed as props to the shape component | ||
// {shapeType, index} | ||
``` | ||
```js | ||
getRandomShape({onlyId: true, type:"star"}) // returns shape identifier that can passed as props to the shape component | ||
// {shapeType: "star", index} | ||
``` | ||
#### Other Methods | ||
All the components are mapped from object that we have given you access to | ||
```js | ||
const shapes = { | ||
star: [Star1, Star2, ...], | ||
ellipse: [Ellipse1, Ellipse2, ...], | ||
... | ||
} | ||
``` | ||
#### Renders the shapes from all catagories | ||
```jsx | ||
import { shapes } from 'coolshapes-react' | ||
const ShapeGrid = () => { | ||
return ( | ||
<> | ||
{ | ||
Object.keys(shapes).map((shapeType, _) =>{ | ||
return shapes[shapeType].map((Shape, index)=>{ | ||
return <Shape size={48}/> | ||
}) | ||
})} | ||
</> | ||
) | ||
}; | ||
``` | ||
###### syntax | ||
```js | ||
shapes[type][index] | ||
``` | ||
```js | ||
const starComponents = shapes['star'] | ||
const StarComponent1 = starComponents[0] | ||
``` | ||
### Props | ||
| name | data type | default | | ||
| ------------- | -------- | ------------- | | ||
| `size` | _Number_ | 200 | | ||
| `type` | _String_ | currentColor | | ||
| `noise` | _Boolean_ | true | | ||
| `index` | _Number_ | random | | ||
| name | data type | default | description | | ||
| ------------- | -------- | ------------- | ------------- | | ||
| `size` | _Number_ | 200 | The dimension of shape | | ||
| [`type`](#categories) | _String_ | random | The category of shapes, if left empty it would randomly select a category. | | ||
| `noise` | _Boolean_ | true | Whether to add noise to the shape or not. | | ||
| `index` | _Number_ | random | The index of shape within the shape [category](#categories), it will randomly select a shape from the category if type prop given. Start from 0. | | ||
| `random` | _Boolean_ | false | If set true it would select a random component | | ||
**Notes:** | ||
Index starts from number 0, so if you want to retrive the first shape of any category, you would use the index number 0. | ||
### Props Value | ||
### Categories | ||
There are a total of **115** shapes available in Coolshapes under the following categories. | ||
| name | count | | ||
| ------------- | -------- | | ||
| `star` | 13 | | ||
| `triangle` | 14 | | ||
| `moon` | 15 | | ||
| `polygon` | 8 | | ||
| `flower` | 16 | | ||
| `rectangle` | 9 | | ||
| `ellipse` | 12 | | ||
| `wheel` | 7 | | ||
| `misc` | 11 | | ||
| `number` | 10 | | ||
If using | ||
**Note:** If you're importing directly as component, the name must be in Title case. And the category type it will be lowercase. | ||
### Others | ||
There are `cjs`, `umd` and `es` included in bundled versions of the module, | ||
#### cjs | ||
```js | ||
const Coolshapes = require("coolshapes-react") | ||
``` | ||
using with react in the browser | ||
```html | ||
<Coolshape type={star} index={1} size={48} noise={true} /> | ||
<!DOCTYPE html> | ||
<html> | ||
<head> | ||
<meta charset="UTF-8" /> | ||
<script src="https://unpkg.com/react@18/umd/react.development.js"></script> | ||
<script src="https://unpkg.com/react-dom@18/umd/react-dom.development.js"></script> | ||
<script src="https://unpkg.com/@babel/standalone/babel.min.js"></script> | ||
<script src="https://unpkg.com/coolshapes-react/dist/umd/coolshapes.js"></script> | ||
</head> | ||
<body> | ||
<div id="root"></div> | ||
<script type="text/babel"> | ||
const domContainer = document.querySelector('#root'); | ||
const root = ReactDOM.createRoot(domContainer); | ||
const coolshapes = window.coolshapes; | ||
const Coolshape = coolshapes.Coolshape; | ||
root.render(<Coolshape/>); | ||
</script> | ||
</body> | ||
</html> | ||
``` | ||
| type | index | default | | ||
| ------------- | -------- | ------------- | | ||
| `star` | 1,2,..4 | random | | ||
| `ellipse` | 1,2,..12 | random | | ||
## Figma File | ||
Figma file coming soon on community | ||
## Contributing | ||
Created by realvjy. You are always welcome to share your feedback on twitter or any social media platform. | ||
If you want to contribute. Just create a [pull request](https://github.com/realvjy/coolshapes-react/pulls). | ||
## Support & Donation | ||
**Coffee fuels coding ☕️** | ||
<a href="https://www.buymeacoffee.com/realvjy" target="_blank"><img src="https://cdn.buymeacoffee.com/buttons/v2/default-yellow.png" alt="Buy Me A Coffee" style="height: 60px !important;width: 217px !important;" ></a> |
Sorry, the diff of this file is too big to display
Sorry, the diff of this file is too big to display
Sorry, the diff of this file is too big to display
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
2116397
10
2538
215