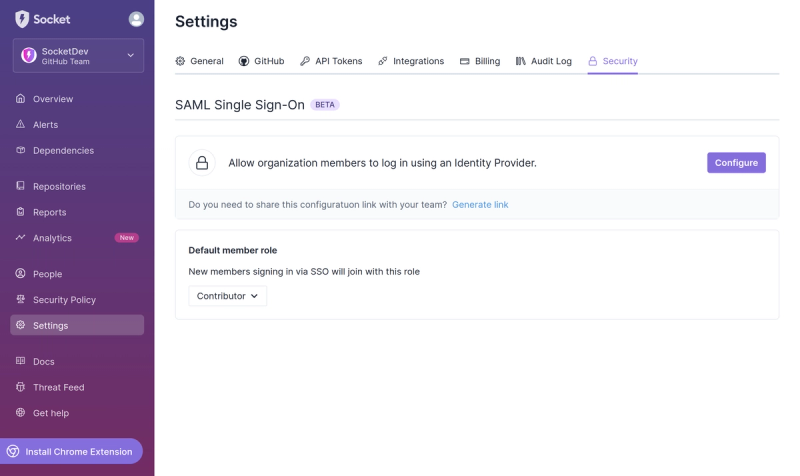
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
cordova-plugin-voiceit-api-2
Advanced tools
This plugin is a Cordova VoiceIt plugin that encapsulates VoiceIt's Face and Voice Verification API
Readme
An Apache Cordova plugin that lets you easily integrate VoiceIt's Face and Voice Verification API into your Cordova Based iOS and Android apps.
For more information on VoiceIt API 2 and its features, see the website and documentation for expected JSON responses.
To use the VoiceIt Cordova Plugin in your Cordova Project, if you haven't already, please Sign Up for a free Developer Id at voiceit.io/signup. Then in the settings page, you should now be able view the API Key and Token (as shown below).
First please cd into your Cordova App's root directory via the terminal/command line and then run the following command (feel free to change the microphone and camera usage description below before running the command):
$ cordova plugin add cordova-plugin-voiceit-api-2 --variable MICROPHONE_USAGE_DESCRIPTION="This app needs to access to your microphone for voice biometrics" --variable CAMERA_USAGE_DESCRIPTION="This app needs to access to your camera for face biometrics"
Here are code snippets that show you how you can call the Various VoiceIt API 2 API calls inside of your Cordova Project JavaScript Files.
Initialize a variable as the reference to VoiceIt API 2, passing in the API Key and Token from the settings page, and optionally a hexadecimal theme color to match your app.
var myVoiceIt = new VoiceItAPITwo({ apiKey : 'API_KEY_HERE', apiToken: 'API_TOKEN_HERE', themeColor: '#FBC132'});
To get all users for the apiKey call the getAllUsers function like this with no parameters:
myVoiceIt.getAllUsers(function(jsonResponse) {
console.log("All users for developer :");
jsonResponse.users.forEach(function(user){
console.log(user.userId + ',');
});
});
To get phrases for the apiKey call getPhrases, padding the contentLanguage
myVoiceIt.getPhrases({
contentLanguage: "CONTENT_LANGUAGE_HERE"
},function(jsonResponse) {
console.log("All phrases for developer :");
jsonResponse.phrases.forEach(function(phrase){
console.log(phrase.text + ',');
});
});
To create a new user call the createUser function like this with no parameters:
myVoiceIt.createUser(function(jsonResponse) {
console.log("User created with userId : " + jsonResponse.userId);
});
To check if a user exists call checkUserExists, passing the userId:
myVoiceIt.checkUserExists({
userId: "USER_ID_HERE"
}, function(jsonResponse) {
console.log("User exists : " + jsonResponse.exists);
});
To get groups that the user belongs to call getGroupsForUser, passing the userId:
myVoiceIt.getGroupsForUser({
userId: "USER_ID_HERE"
}, function(jsonResponse) {
console.log("Groups for user :");
jsonResponse.groups.forEach(function(groupId){
console.log(groupId + ',');
});
});
To delete a user call deleteUser, passing the userId:
myVoiceIt.deleteUser({
userId: "USER_ID_HERE"
}, function(jsonResponse) {
console.log(jsonResponse);
});
To get all groups for the apiKey call the getAllGroups function like this with no parameters:
myVoiceIt.getAllGroups(function(jsonResponse) {
console.log("All groups for developer :");
jsonResponse.groups.forEach(function(group){
console.log(group.groupId + ',');
});
});
To get a group's details call getGroup, passing the groupId:
myVoiceIt.getGroup({
groupId: "GROUP_ID_HERE"
}, function(jsonResponse) {
console.log(jsonResponse);
});
To check if a group exists call groupExists, passing the groupId:
myVoiceIt.groupExists({
groupId: "GROUP_ID_HERE"
}, function(jsonResponse) {
console.log("Group exists : ",jsonResponse.exist);
});
To create a new group call the createGroup function like this with no parameters:
myVoiceIt.createGroup(function(jsonResponse) {
console.log("Group created with groupId : " + jsonResponse.groupId);
});
To add a user to a group call the addUserToGroup function, passing the groupId and userId:
myVoiceIt.addUserToGroup({
userId:"USER_ID_HERE", groupId: "GROUP_ID_HERE"
}, function(jsonResponse) {
console.log("User added to group: " + jsonResponse);
});
To remove a user from a group call the removeUserFromGroup function, passing the groupId and userId:
myVoiceIt.removeUserFromGroup({
userId:"USER_ID_HERE", groupId: "GROUP_ID_HERE"
}, function(jsonResponse) {
console.log("User removed from group: " + jsonResponse);
});
To delete a group call deleteGroup, passing the groupId:
myVoiceIt.deleteGroup({
groupId: "GROUP_ID_HERE"
}, function(jsonResponse) {
console.log(jsonResponse);
});
To get all voice enrollments for a user call getAllVoiceEnrollments, passing the userId:
myVoiceIt.getAllVoiceEnrollments({
userId: "USER_ID_HERE"
}, function(jsonResponse) {
console.log("All voice enrollments for user :");
jsonResponse.voiceEnrollments.forEach(function(enrollment){
console.log(enrollment.voiceEnrollmentId + ',');
});
});
To get all face enrollments for a user call getAllFaceEnrollments, passing the userId:
myVoiceIt.getAllFaceEnrollments({
userId: "USER_ID_HERE"
}, function(jsonResponse) {
console.log("All face enrollments for user :");
jsonResponse.faceEnrollments.forEach(function(enrollment){
console.log(enrollment.faceEnrollmentId + ',');
});
});
To get all video enrollments for a user call getAllVideoEnrollments, passing the userId:
myVoiceIt.getAllVideoEnrollments({
userId: "USER_ID_HERE"
}, function(jsonResponse) {
console.log("All video enrollments for user :");
jsonResponse.videoEnrollments.forEach(function(enrollment){
console.log(enrollment.videoEnrollmentId + ',');
});
});
To delete a voice enrollment call deleteVoiceEnrollment, passing the userId and voiceEnrollmentId parameters:
myVoiceIt.deleteEnrollment({
userId: "USER_ID_HERE",
voiceEnrollmentId:"VOICE_ENROLLMENT_ID_HERE"
}, function(jsonResponse) {
console.log(jsonResponse);
});
To delete a face enrollment call deleteFaceEnrollment, passing the userId and faceEnrollmentId parameters:
myVoiceIt.deleteFaceEnrollment({
userId: "USER_ID_HERE",
faceEnrollmentId:"FACE_ENROLLMENT_ID_HERE"
}, function(jsonResponse) {
console.log(jsonResponse);
});
To delete a video enrollment call deleteVideoEnrollment, passing the userId and videoEnrollmentId parameters:
myVoiceIt.deleteVideoEnrollment({
userId: "USER_ID_HERE",
videoEnrollmentId:"VIDEO_ENROLLMENT_ID_HERE"
}, function(jsonResponse) {
console.log(jsonResponse);
});
To delete all voice enrollments call deleteAllVoiceEnrollments, passing the userId:
myVoiceIt.deleteAllVoiceEnrollments({
userId: "USER_ID_HERE"
}, function(jsonResponse) {
console.log(jsonResponse);
});
To delete all face enrollments call deleteAllFaceEnrollments, passing the userId:
myVoiceIt.deleteAllFaceEnrollments({
userId: "USER_ID_HERE"
}, function(jsonResponse) {
console.log(jsonResponse);
});
To delete all video enrollments call deleteAllVideoEnrollments, passing the userId:
myVoiceIt.deleteAllVideoEnrollments({
userId: "USER_ID_HERE"
}, function(jsonResponse) {
console.log(jsonResponse);
});
To delete all voice,face and video enrollments for a user call deleteAllEnrollments, passing the userId:
myVoiceIt.deleteAllEnrollments({
userId: "USER_ID_HERE"
}, function(jsonResponse) {
console.log(jsonResponse);
});
To enroll a user's voice, call the encapsulatedVoiceEnrollUser function, passing the userId, contentLanguage, and phrase ( make sure you use an approved phrase for your developer account at https://voiceit.io/phraseManagement) parameters. This method includes the UI and walks the user through three successful voice enrollments before returning.
myVoiceIt.encapsulatedVoiceEnrollUser({
userId: "USER_ID_HERE",
contentLanguage:"en-US",
phrase:"my face and voice identify me"
}, function() {
console.log("User cancelled enrollments");
}, function() {
console.log("User successfully completed enrolling");
});
To enroll a user's face, call the encapsulatedFaceEnrollUser function passing the userId to enroll. This method includes the UI and walks the user through a successful face enrollment before returning;
myVoiceIt.encapsulatedFaceEnrollUser({
userId: "USER_ID_HERE"
}, function() {
console.log("User cancelled face enrollment");
}, function() {
console.log("User successfully completed enrolling");
});
To enroll a user's face and voice, call the encapsulatedVideoEnrollUser function, passing the userId, contentLanguage, and phrase ( make sure you use an approved phrase for your developer account at https://voiceit.io/phraseManagement) parameters. This method includes the UI and walks the user through three successful enrollments before returning.
myVoiceIt.encapsulatedVideoEnrollUser({
userId: "USER_ID_HERE",
contentLanguage:"en-US",
phrase:"my face and voice identify me"
}, function() {
console.log("User cancelled enrollments");
}, function() {
console.log("User successfully completed enrolling");
});
To verify a user's voice, call the encapsulatedVoiceVerification function, passing the userId, contentLanguage, and phrase (make sure you use an approved phrase for your developer account at https://voiceit.io/phraseManagement) parameters. This method includes the UI for voice verification and returns the voice confidence.
myVoiceIt.encapsulatedVoiceVerification({
userId: "USER_ID_HERE",
contentLanguage:"en-US",
phrase:"my face and voice identify me"
}, function() {
console.log("User cancelled verifications");
}, function(jsonResponse) {
console.log("User successfully completed verification with voiceConfidence : " + jsonResponse.voiceConfidence);
});
To verify a user's face call the encapsulatedFaceVerification function, passing the userId and doLivenessDetection parameters. doLivenessDetection is a boolean that makes the user attempt liveness challenges, such as smiling, blinking, moving their face left/right for enhanced security. This method includes the UI for face verification and returns the face confidence.
myVoiceIt.encapsulatedFaceVerification({
userId: "USER_ID_HERE",
doLivenessDetection: true
}, function() {
console.log("User cancelled verifications");
}, function(jsonResponse) {
console.log("User successfully completed verification with faceConfidence : " + jsonResponse.faceConfidence);
});
To verify a user's face and voice, call the encapsulatedVideoVerification function, passing the userId, contentLanguage, phrase( make sure you use an approved phrase for your developer account at https://voiceit.io/phraseManagement) and doLivenessDetection parameters. doLivenessDetection is a boolean that makes the user attempt liveness challenges, such as smiling, blinking, moving their face left/right for enhanced security. This method includes the UI for face and voice verification and returns the face and voice confidence.
myVoiceIt.encapsulatedVideoVerification({
userId: "USER_ID_HERE",
contentLanguage:"en-US",
phrase:"my face and voice identify me",
doLivenessDetection: true
}, function() {
console.log("User cancelled verifications");
}, function(jsonResponse) {
console.log("User successfully completed verification with faceConfidence : " + jsonResponse.faceConfidence + " and voiceConfidence : " + jsonResponse.voiceConfidence);
});
To identify a user's voice, call the encapsulatedVoiceIdentification function, passing the groupId, contentLanguage, and phrase (make sure you use an approved phrase for your developer account at https://voiceit.io/phraseManagement) parameters. This method includes the UI for voice identification and returns the voice confidence.
myVoiceIt.encapsulatedVoiceIdentification({
groupId: "GROUP_ID_HERE",
contentLanguage:"en-US",
phrase:"my face and voice identify me"
}, function() {
console.log("User cancelled identification");
}, function(jsonResponse) {
console.log("User successfully completed identification with voiceConfidence : " + jsonResponse.voiceConfidence);
});
To identify a user's face call the encapsulatedFaceIdentification function, passing the groupId and doLivenessDetection parameters. doLivenessDetection is a boolean that makes the user attempt liveness challenges, such as smiling, blinking, moving their face left/right for enhanced security. This method includes the UI for face identification and returns the face confidence.
myVoiceIt.encapsulatedFaceIdentification({
groupId: "GROUP_ID_HERE",
doLivenessDetection: true
}, function() {
console.log("User cancelled identification");
}, function(jsonResponse) {
console.log("User successfully completed identification with faceConfidence : " + jsonResponse.faceConfidence);
});
To identify a user's face and voice out of a group, call the encapsulatedVideoIdentification function, passing the groupId, contentLanguage, phrase( make sure you use an approved phrase for your developer account at https://voiceit.io/phraseManagement) and doLivenessDetection parameters. doLivenessDetection is a boolean that makes the user attempt liveness challenges, such as smiling, blinking, moving their face left/right for enhanced security. This method includes the UI for face and voice identification and returns the face and voice confidence.
myVoiceIt.encapsulatedVideoIdentification({
groupId: "USER_ID_HERE",
contentLanguage:"en-US",
phrase:"my face and voice identify me",
doLivenessDetection: true
}, function() {
console.log("User cancelled identification");
}, function(jsonResponse) {
console.log("User " + jsonResponse.userId + " was successfully identified with faceConfidence : " + jsonResponse.faceConfidence + " and voiceConfidence : " + jsonResponse.voiceConfidence);
});
armaanbindra, armaan@voiceit.io
armaanbindra, armaan@voiceit.io
stephenakers, stephen@voiceit.io
Cordova Plugin VoiceIt API 2 is available under the GNU GENERAL PUBLIC LICENSE. See the LICENSE file for more info.
FAQs
This plugin is a Cordova VoiceIt plugin that encapsulates VoiceIt's Face and Voice Verification API
The npm package cordova-plugin-voiceit-api-2 receives a total of 60 weekly downloads. As such, cordova-plugin-voiceit-api-2 popularity was classified as not popular.
We found that cordova-plugin-voiceit-api-2 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.