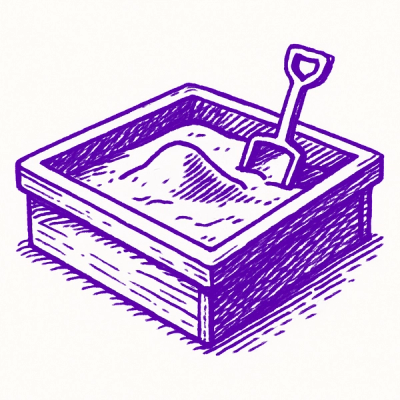
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
countries-and-timezones
Advanced tools
Minimalistic library to work with countries and timezones data.
The 'countries-and-timezones' npm package provides utilities for working with country and timezone data. It allows you to retrieve information about countries, their timezones, and perform various operations related to timezones.
Get Country Information
This feature allows you to retrieve information about a specific country using its ISO 3166-1 alpha-2 code. The returned object includes details such as the country's name, timezones, and other relevant data.
const ctz = require('countries-and-timezones');
const country = ctz.getCountry('US');
console.log(country);
Get Timezone Information
This feature allows you to retrieve information about a specific timezone using its IANA timezone identifier. The returned object includes details such as the timezone's name, offset, and other relevant data.
const ctz = require('countries-and-timezones');
const timezone = ctz.getTimezone('America/New_York');
console.log(timezone);
Get All Countries
This feature allows you to retrieve a list of all countries supported by the package. The returned object includes details for each country.
const ctz = require('countries-and-timezones');
const countries = ctz.getAllCountries();
console.log(countries);
Get All Timezones
This feature allows you to retrieve a list of all timezones supported by the package. The returned object includes details for each timezone.
const ctz = require('countries-and-timezones');
const timezones = ctz.getAllTimezones();
console.log(timezones);
Get Timezones for a Country
This feature allows you to retrieve a list of all timezones for a specific country using its ISO 3166-1 alpha-2 code. The returned array includes details for each timezone.
const ctz = require('countries-and-timezones');
const timezones = ctz.getTimezonesForCountry('US');
console.log(timezones);
The 'moment-timezone' package is an extension for the 'moment' library to handle timezones. It provides utilities for parsing, manipulating, and displaying dates and times in various timezones. Compared to 'countries-and-timezones', 'moment-timezone' focuses more on date and time manipulation rather than country-specific data.
The 'timezone-support' package provides utilities for working with timezones and converting dates between different timezones. It includes features for parsing and formatting dates, as well as handling daylight saving time. Compared to 'countries-and-timezones', 'timezone-support' is more focused on date and time operations rather than providing country-specific information.
The 'world-countries' package provides a comprehensive list of countries with various details such as names, codes, and regions. It is similar to 'countries-and-timezones' in terms of providing country data, but it does not include timezone information.
Minimalistic library to work with countries and timezones data. Updated with the IANA timezones database.
Install with npm or yarn:
npm install --save countries-and-timezones
Add the following script to your project (only ~9kb):
<!-- Latest version -->
<script
src="https://cdn.jsdelivr.net/gh/manuelmhtr/countries-and-timezones@latest/dist/index.min.js"
type="text/javascript"
></script>
<!-- Or specify a version -->
<script
src="https://cdn.jsdelivr.net/gh/manuelmhtr/countries-and-timezones@v3.8.0/dist/index.min.js"
type="text/javascript"
></script>
<!-- This will export a variable named "ct": -->
<script type="text/javascript">
var data = ct.getCountry("MX");
console.log(data);
</script>
.getCountry(id, options = {})
Returns a country referenced by its id
.
Accepts a parameter with options
.
const ct = require("countries-and-timezones");
const country = ct.getCountry("DE");
console.log(country);
/*
Prints:
{
id: 'DE',
name: 'Germany',
timezones: [ 'Europe/Berlin', 'Europe/Zurich' ]
}
*/
.getTimezone(name)
Returns a timezone referenced by its name
.
const ct = require("countries-and-timezones");
const timezone = ct.getTimezone("America/Los_Angeles");
console.log(timezone);
/*
Prints:
{
name: 'America/Los_Angeles',
countries: [ 'US' ],
utcOffset: -480,
utcOffsetStr: '-08:00',
dstOffset: -420,
dstOffsetStr: '-07:00',
aliasOf: null
}
*/
.getAllCountries(options = {})
Returns an object with the data of all countries.
Accepts a parameter with options
.
const ct = require("countries-and-timezones");
const countries = ct.getAllCountries();
console.log(countries);
/*
Prints:
{
AD: {
id: 'AD',
name: 'Andorra',
timezones: [ 'Europe/Andorra' ]
},
AE: {
id: 'AE',
name: 'United Arab Emirates',
timezones: [ 'Asia/Dubai' ]
},
AF: {
id: 'AF',
name: 'Afghanistan',
timezones: [ 'Asia/Kabul' ]
},
AG: {
id: 'AG',
name: 'Antigua and Barbuda',
timezones: [ 'America/Puerto_Rico' ]
},
...
}
*/
.getAllTimezones(options = {})
Returns an object with the data of all timezones.
Accepts a parameter with options
.
const ct = require("countries-and-timezones");
const timezones = ct.getAllTimezones();
console.log(timezones);
/*
Prints:
{
"Africa/Abidjan": {
"name": "Africa/Abidjan",
"countries": [
"CI", "BF", "GH",
"GM", "GN", "ML",
"MR", "SH", "SL",
"SN", "TG"
],
"utcOffset": 0,
"utcOffsetStr": "+00:00",
"dstOffset": 0,
"dstOffsetStr": "+00:00",
"aliasOf": null
},
"Africa/Algiers": {
"name": "Africa/Algiers",
"countries": [
"DZ"
],
"utcOffset": 60,
"utcOffsetStr": "+01:00",
"dstOffset": 60,
"dstOffsetStr": "+01:00",
"aliasOf": null
},
"Africa/Bissau": {
"name": "Africa/Bissau",
"countries": [
"GW"
],
"utcOffset": 0,
"utcOffsetStr": "+00:00",
"dstOffset": 0,
"dstOffsetStr": "+00:00",
"aliasOf": null
},
...
}
*/
.getTimezonesForCountry(id, options = {})
Returns an array with all the timezones of a country given its id
.
Accepts a parameter with options
.
const ct = require("countries-and-timezones");
const timezones = ct.getTimezonesForCountry("MX");
console.log(timezones);
/*
Prints:
[
{
"name": "America/Bahia_Banderas",
"countries": [ "MX" ],
"utcOffset": -360,
"utcOffsetStr": "-06:00",
"dstOffset": -300,
"dstOffsetStr": "-05:00",
"aliasOf": null
},
{
"name": "America/Cancun",
"countries": [ "MX" ],
"utcOffset": -300,
"utcOffsetStr": "-05:00",
"dstOffset": -300,
"dstOffsetStr": "-05:00",
"aliasOf": null
},
{
"name": "America/Chihuahua",
"countries": [ "MX" ],
"utcOffset": -420,
"utcOffsetStr": "-07:00",
"dstOffset": -360,
"dstOffsetStr": "-06:00",
"aliasOf": null
},
...
}
*/
.getCountriesForTimezone(name, options = {})
Returns a list of the countries that uses a timezone given its name
. When a timezone has multiple countries the first element is more relevant due to its geographical location.
Accepts a parameter with options
.
const ct = require("countries-and-timezones");
const timezone = ct.getCountriesForTimezone("Europe/Zurich");
console.log(timezone);
/*
Prints:
[
{
"id": "CH",
"name": "Switzerland",
"timezones": [
"Europe/Zurich"
]
},
{
"id": "DE",
"name": "Germany",
"timezones": [
"Europe/Berlin",
"Europe/Zurich"
]
},
{
"id": "LI",
"name": "Liechtenstein",
"timezones": [
"Europe/Zurich"
]
}
]
*/
.getCountryForTimezone(name, options = {})
Returns a the most relevant country (due to its geographical location) that uses a timezone given its name
.
Accepts a parameter with options
.
const ct = require("countries-and-timezones");
const timezone = ct.getCountryForTimezone("Europe/Zurich");
console.log(timezone);
/*
Prints:
{
"id": "CH",
"name": "Switzerland",
"timezones": [
"Europe/Zurich"
]
}
*/
options
Available options for functions are:
Parameter | Type | Description |
---|---|---|
deprecated | Boolean | Indicates if the result should include deprecated timezones or not. By default no deprecated timezones are included. |
A country is defined by the following parameters:
Parameter | Type | Description |
---|---|---|
id | String | The country ISO 3166-1 code. |
name | String | Preferred name of the country. |
timezones | Array[String] | The list of timezones used in the country. |
{
id: 'MX',
name: 'Mexico',
timezones: [
'America/Bahia_Banderas',
'America/Cancun',
'America/Chihuahua',
'America/Hermosillo',
'America/Matamoros',
'America/Mazatlan',
'America/Merida',
'America/Mexico_City',
'America/Monterrey',
'America/Ojinaga',
'America/Tijuana'
]
}
A timezone is defined by the following parameters:
Parameter | Type | Description |
---|---|---|
name | String | The name of the timezone, from tz database. |
countries | [String] | A list of ISO 3166-1 codes of the countries where it's used. Etc/* , GMT and UTC timezones don't have associated countries. |
utcOffset | Number | The difference in minutes between the timezone and UTC. |
utcOffsetStr | String | The difference in hours and minutes between the timezone and UTC, expressed as string with format: ±[hh]:[mm] . |
dstOffset | Number | The difference in minutes between the timezone and UTC during daylight saving time (DST). When utcOffset and dstOffset are the same, means that the timezone does not observe a daylight saving time. |
dstOffsetStr | String | The difference in hours and minutes between the timezone and UTC during daylight saving time (DST, expressed as string with format: ±[hh]:[mm] . |
aliasOf | String | The name of a primary timezone in case this is an alias. null means this is a primary timezone. |
deprecated | Boolean | true when the timezone has been deprecated, otherwise this property is not returned. |
{
name: 'Asia/Tel_Aviv',
countries: [ 'IL' ],
utcOffset: 120,
utcOffsetStr: '+02:00',
dstOffset: 180,
dstOffsetStr: '+03:00',
aliasOf: 'Asia/Jerusalem',
deprecated: true
}
Consider sponsoring this project.
Meet Spott:
MIT
[3.8.0] - 2025-04-12
FAQs
Minimalistic library to work with countries and timezones data.
The npm package countries-and-timezones receives a total of 277,061 weekly downloads. As such, countries-and-timezones popularity was classified as popular.
We found that countries-and-timezones demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.