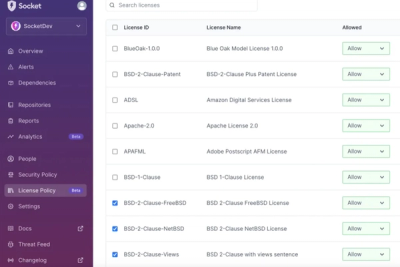
Product
Introducing License Enforcement in Socket
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
CRUD interface for mysql
When developing small CRUD apps it's useful to have a simple abstraction over database operations. This library provides a minimal viable interface over mysql, manages a connection pool, and transaction management.
import configureMysql from 'crud-mysql'
const mysqlConfig = {
user: 'test',
password: 'test',
database: 'test'
}
const database = configureMysql(mysqlConfig)
See mysql docs for config options
await database(async query => {
await query.create('users', { id: 1, username: 'possibilities' })
await query.create('users', { id: 2, username: 'thrivingkings' })
})
Read all rows
await database(async query => {
const users = await query.read('users')
const usernames = users.map(u => u.username)
console.info(usernames) //-> ['possibilities', 'thrivingkings']
})
Fetch certain rows
await database(async query => {
const users = await query.read('users', { id: 1 })
const { username } = users.pop()
console.info(username) //-> possibilities
})
await database(async query => {
const { country } = await query.update('users', { id: 1, country: 'denmark' })
console.info(country) //-> denmark
})
await database(async query => {
await query.delete('users', { id: 1 })
const users = query.read('users', { id: 1 })
console.info(users.length) //-> 0
})
await database(async query => {
const insertSql = "INSERT INTO users (`username`, `country`) VALUES ('possibilities', 'iceland')"
await query(insertSql)
const selectSql = 'SELECT username, country FROM users WHERE country = iceland'
const users = await query(selectSql)
const { country, username } = users.pop()
console.info(username) //-> possibilities
console.info(country) //-> iceland
})
Formating and escaping is provided by sqlstring
await database(async query => {
const insertSql = "INSERT INTO ?? (??) VALUES (?)"
await query(insertSql, ['users', ['username', 'country'], ['possibilities', 'iceland']])
const selectSql = 'SELECT ?? FROM ?? WHERE ?'
const users = await query(selectSql, [['username', 'country'], 'users', { country: 'iceland' }])
const { country, username } = users.pop()
console.info(username) //-> possibilities
console.info(country) //-> iceland
})
database.withTransaction(async query => {
await query("INSERT INTO users (`username`) VALUES ('possibilities')")
await query('INSERT INTO users (`nonexistent`) VALUES ('nonexistent')')
})
database(async query => {
const users = await query('SELECT * FROM users')
console.info(users.length) //-> 0
})
FAQs
CRUD interface for mysql data
The npm package crud-mysql receives a total of 0 weekly downloads. As such, crud-mysql popularity was classified as not popular.
We found that crud-mysql demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new set of license analysis and compliance features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.
Product
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.