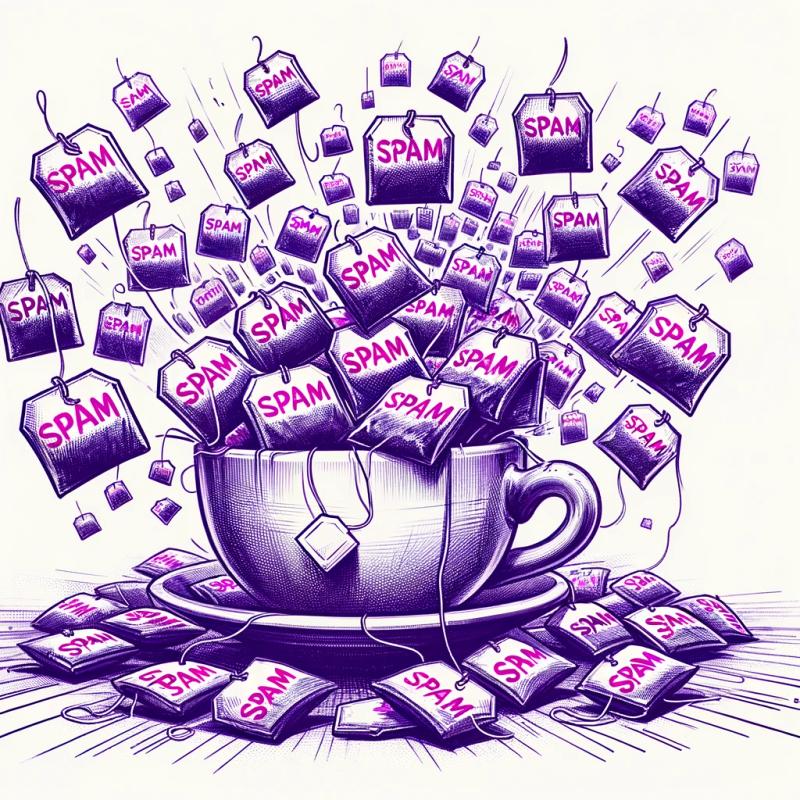
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
css-grid-template-parser
Advanced tools
Readme
A simple CSS Grid template parser
npm install --save css-grid-template-parser
import { grid } from 'css-grid-template-parser'
const areas = grid(`
"a a a b b"
"a a a b b"
". . c c c"
"d d d d d"
`)
// → {
// width: 5,
// height: 4,
// areas: {
// a: {
// column: {start: 1, end: 4, span: 3},
// row: {start: 1, end: 3, span: 2},
// },
// b: {
// column: {start: 4, end: 6, span: 2},
// row: {start: 1, end: 3, span: 2},
// },
// c: {
// column: {start: 3, end: 6, span: 3},
// row: {start: 3, end: 4, span: 1},
// },
// d: {
// column: {start: 1, end: 6, span: 5},
// row: {start: 4, end: 5, span: 1},
// },
// },
// }
import { template } from 'css-grid-template-parser'
const areas = template({
width: 5,
height: 4,
areas: {
a: {
column: { start: 1, end: 4, span: 3 },
row: { start: 1, end: 3, span: 2 },
},
b: {
column: { start: 3, end: 6, span: 3 },
row: { start: 3, end: 5, span: 2 },
},
},
})
// → `"a a a . ."
// "a a a . ."
// ". . b b b"
// ". . b b b"`
An helper is provided to declare areas more intuitively. The following example is equivalent to the previous:
import { template, area } from 'css-grid-template-parser'
const a = area({
x: 0,
y: 0,
width: 3,
height: 2,
})
const b = area({
x: 2,
y: 2,
width: 3,
height: 2,
})
const areas = template({
width: 5,
height: 4,
areas: { a, b },
})
// → `"a a a . ."
// "a a a . ."
// ". . b b b"
// ". . b b b"`
grid(template)
Parses a grid template and returns an object representation.
template
string The grid template to parse.Grid An object representation of the grid template.
import { grid } from 'css-grid-template-parser'
const areas = grid(`
"a a a b b"
"a a a b b"
". . c c c"
"d d d d d"
`)
// → {
// width: 5,
// height: 4,
// areas: {
// a: {
// column: {start: 1, end: 4, span: 3},
// row: {start: 1, end: 3, span: 2},
// },
// b: {
// column: {start: 4, end: 6, span: 2},
// row: {start: 1, end: 3, span: 2},
// },
// c: {
// column: {start: 3, end: 6, span: 3},
// row: {start: 3, end: 4, span: 1},
// },
// d: {
// column: {start: 1, end: 6, span: 5},
// row: {start: 4, end: 5, span: 1},
// },
// },
// }
template(grid)
Builds a grid template from an object representation.
grid
Grid The grid to build.string The equivalent grid template.
import { template } from 'css-grid-template-parser'
const areas = template({
width: 5,
height: 4,
areas: {
a: {
column: { start: 1, end: 4, span: 3 },
row: { start: 1, end: 3, span: 2 },
},
b: {
column: { start: 3, end: 6, span: 3 },
row: { start: 3, end: 5, span: 2 },
},
},
})
// → `"a a a . ."
// "a a a . ."
// ". . b b b"
// ". . b b b"`
rect(area)
Converts an area into a rect.
area
Area The area to convert.Rect The equivalent rect.
import { rect } from 'css-grid-template-parser'
const r = rect({
column: { start: 1, end: 4, span: 3 },
row: { start: 1, end: 3, span: 2 },
})
// → {
// x: 0,
// y: 0,
// width: 3,
// height: 2,
// }
area(rect)
Converts a rect into an area.
rect
Rect The rect to convert.Area The equivalent area.
import { area } from 'css-grid-template-parser'
const a = area({
x: 0,
y: 0,
width: 3,
height: 2,
})
// → {
// column: {start: 1, end: 4, span: 3},
// row: {start: 1, end: 3, span: 2},
// }
minColumnStart(grid)
Finds the min column start of all grid areas.
grid
Grid The grid to analyze.number The min column start.
import { grid, minColumnStart } from 'css-grid-template-parser'
const min = minColumnStart(
grid(`
". . a a a"
". b b b b"
". . . c c"
`)
)
// → 2
maxColumnStart(grid)
Finds the max column start of all grid areas.
grid
Grid The grid to analyze.number The max column start.
import { grid, maxColumnStart } from 'css-grid-template-parser'
const max = maxColumnStart(
grid(`
". . a a a"
". b b b b"
". . . c c"
`)
)
// → 4
minColumnEnd(grid)
Finds the min column end of all grid areas.
grid
Grid The grid to analyze.number The min column end.
import { grid, minColumnEnd } from 'css-grid-template-parser'
const min = minColumnEnd(
grid(`
"a a . . ."
"b b b b ."
"c c c . ."
`)
)
// → 3
maxColumnEnd(grid)
Finds the max column end of all grid areas.
grid
Grid The grid to analyze.number The max column end.
import { grid, maxColumnEnd } from 'css-grid-template-parser'
const max = maxColumnEnd(
grid(`
"a a . . ."
"b b b b ."
"c c c . ."
`)
)
// → 5
minRowStart(grid)
Finds the min row start of all grid areas.
grid
Grid The grid to analyze.number The min row start.
import { grid, minRowStart } from 'css-grid-template-parser'
const min = minRowStart(
grid(`
". . . ."
"a a . ."
"a a b b"
"a a b b"
`)
)
// → 2
maxRowStart(grid)
Finds the max row start of all grid areas.
grid
Grid The grid to analyze.number The max row start.
import { grid, maxRowStart } from 'css-grid-template-parser'
const max = maxRowStart(
grid(`
". . . ."
"a a . ."
"a a b b"
"a a b b"
`)
)
// → 3
minRowEnd(grid)
Finds the min row end of all grid areas.
grid
Grid The grid to analyze.number The min row end.
import { grid, minRowEnd } from 'css-grid-template-parser'
const min = minRowEnd(
grid(`
"a a b b"
"a a b b"
". . b b"
". . . ."
`)
)
// → 3
maxRowEnd(grid)
Finds the max row end of all grid areas.
grid
Grid The grid to analyze.number The max row end.
import { grid, maxRowEnd } from 'css-grid-template-parser'
const max = maxRowEnd(
grid(`
"a a b b"
"a a b b"
". . b b"
". . . ."
`)
)
// → 4
Track
export interface Track {
start: number
end: number
span: number
}
Area
type Area = {
row: Track
column: Track
}
Rect
export interface Rect {
x: number
y: number
width: number
height: number
}
Grid
export interface Grid {
width: number
height: number
areas: Record<string, Area>
}
MIT
FAQs
A simple CSS Grid template parser
The npm package css-grid-template-parser receives a total of 458 weekly downloads. As such, css-grid-template-parser popularity was classified as not popular.
We found that css-grid-template-parser demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.