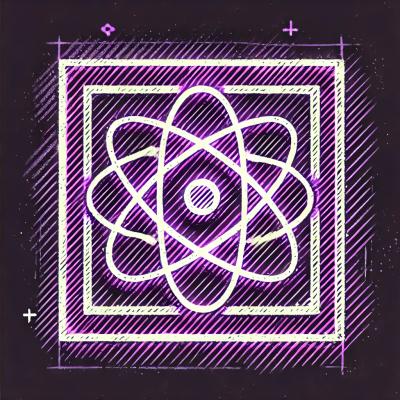
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
custom-jquery-methods
Advanced tools
:us: English | :ru: Русский язык
Custom jQuery methods ($.fn)
Basically, methods are intended for use with the webpack
bundler system, there is also the ability to connect methods via direct links in browser or download them (see description of each method)
npm i --save custom-jquery-methods
# or using yarn cli
yarn add custom-jquery-methods
methods list
$.fn.nodeName()
$.fn.addClassSiblingsRemove()
$.fn.changeMyClass()
$.fn.getMyElements()
$.fn.hasInitedKey()
$.fn.removeInitedKey()
You can include all methods by one file
// es6
import 'custom-jquery-methods';
// or minimised version
import 'custom-jquery-methods/fn/index.min';
// es5
require('custom-jquery-methods');
// or minimised version
require('custom-jquery-methods/dist/index.min');
Returns nodeName
property of DOM element in lowercase.
Useful when you can get unknown elements.
function myFunction ($el) {
switch ($el.nodeName()) {
case 'img':
// ...
break;
case 'div':
// ...
break;
case 'input':
// ...
break;
default:
// ...
}
}
// es6
import 'custom-jquery-methods/fn/node-name';
// or minimised version
import 'custom-jquery-methods/fn/node-name.min';
// es5
require('custom-jquery-methods/dist/node-name');
// or minimised version
require('custom-jquery-methods/dist/node-name.min');
Adding a class to the current element and deleting from adjacent elements
Name | Type | Attributes | Description |
---|---|---|---|
cssClass | string | The class to be added | |
customPath | string[] | <optional> | Custom path to adjacent elements |
example 1. On the same level
$('.item').addClassSiblingsRemove('is-active');
// it will reproduce
$('.item').addClass('is-active').siblings().removeClass('is-active');
example 2. At the level of the parent elements with customPath
parameter
$('.item').addClassSiblingsRemove('is-active', ['parent', 'siblings', 'children']);
// it will reproduce
$('.item').addClass('is-active').parent().siblings().children().removeClass('is-active');
example 3. Also, customPath
allows you to use a dynamically calculated path depending on the conditions you need
let customPath = ['parent', 'siblings', 'children'];
if (condition1) {
customPath.unshift('parent');
} else if (condition2) {
customPath = ['next'];
} else if (condition3) {
customPath = ['prev'];
}
$('.item').addClassSiblingsRemove('is-active', customPath);
// it will reproduce
if (condition1) {
$('.item').addClass('is-active').parent().parent().siblings().children().removeClass('is-active');
} else if (condition2) {
$('.item').addClass('is-active').next().removeClass('is-active');
} else if (condition3) {
$('.item').addClass('is-active').prev().removeClass('is-active');
} else {
$('.item').addClass('is-active').parent().siblings().children().removeClass('is-active');
}
// es6
import 'custom-jquery-methods/fn/add-class-siblings-remove';
// or minimised version
import 'custom-jquery-methods/fn/add-class-siblings-remove.min';
// es5
require('custom-jquery-methods/dist/add-class-siblings-remove');
// or minimised version
require('custom-jquery-methods/dist/add-class-siblings-remove.min');
Add className
if previously was removed.
Remove className
if previously was added.
Returns boolean
- className was added or not!
Name | Type | Attributes | Description |
---|---|---|---|
needToAdd | boolean | add className ? | |
previouslyAdded | boolean | was className previously added or not | |
className | string / string[] / function | see api.jquery.com/addClass and api.jquery.com/removeClass | |
onChange | function | <optional> |
/**
* @param {jQuery} $section
* @param {jQuery} $frontier
*/
function showSection ($section, $frontier) {
const $window = $(window);
let previouslyAdded = $section.hasClass('show'); // <--
$window.on('scroll', () => {
const top = $window.scrollTop();
const height = $frontier.offset().top + $frontier.outerHeight();
previouslyAdded = $section.changeMyClass(top > height, previouslyAdded, 'show'); // <--
});
}
// es6
import 'custom-jquery-methods/fn/change-my-class';
// or minimised version
import 'custom-jquery-methods/fn/change-my-class.min';
// es5
require('custom-jquery-methods/dist/change-my-class');
// or minimised version
require('custom-jquery-methods/dist/change-my-class.min');
Search on the page or retrieve from the data of the desired item.
First, look at the data object on a certain property.
If it is empty - look for the element on the specified selector in the given direction.
When found, write it in the data object to
at subsequent calls - we get from the data, faster and without searching.
!!! If called element more then one,
then the method is performed only for the first
Name | Type | Attributes | Default | Description |
---|---|---|---|---|
dataKey | string | the property key from the data object of the element | ||
selector | JQuery.Selector | search selector | ||
direction | string/JQuery | <optional> | "document" | direction where to look for - [closest, parent, children, find, prev, next, siblings] , or can be jQuery element for find selector inside |
notSelf | boolean | <optional> | ignore the current element, when searching for elements, for example in document using the same selector as the current element |
jQuery.<Element> | undefined
example 1. Find / retrieve nested items
let $els = $('.wrapper').getMyElements('$myEls', '.els-selector', 'find');
example 2. Find / retrieve nested items only in context block
let $context = $('.demo');
let $els = $('.wrapper').getMyElements('$myEls', '.els-selector', $context);
example 3. Finding / getting the parent element
let $wrapper = $('.els').getMyElements('$myWrapper', '.wrapper-selector', 'closest');
example 4. Search / retrieve similar items except for the current one
let $sameEls = $('.els').getMyElements('$mySameEls', '.els', 'document', true);
// es6
import 'custom-jquery-methods/fn/get-my-elements';
// or minimised version
import 'custom-jquery-methods/fn/get-my-elements.min';
// es5
require('custom-jquery-methods/dist/get-my-elements');
// or minimised version
require('custom-jquery-methods/dist/get-my-elements.min');
Check for the existence of an initialization key in .data()
.
Name | Type | Attributes | Default | Description |
---|---|---|---|---|
key | string | key name | ||
setKey | boolean | <optional> | true | set the key, if not exist |
boolean
example 1. Check in cycle .each()
let initKey = 'my-key';
$('.my-elements').each((i, el) => {
let $element = $(el);
if ($element.hasInitedKey(initKey)) {
return true;
}
// process current element
});
example 2. Check for single element
let initKey = 'my-key';
let $myEl = $('.my-elements');
if (!$myEl.hasInitedKey(initKey)) {
// process current element
}
// es6
import 'custom-jquery-methods/fn/has-inited-key';
// or minimised version
import 'custom-jquery-methods/fn/has-inited-key.min';
// es5
require('custom-jquery-methods/dist/has-inited-key');
// or minimised version
require('custom-jquery-methods/dist/has-inited-key.min');
Removing an initialization key in .data()
.
The method is the inverse action for $.fn.hasInitedKey
Name | Type | Attributes | Default | Description |
---|---|---|---|---|
key | string | имя ключа |
jQuery.<Element>
- this
let initKey = 'my-key';
let $myEl = $('.my-elements');
$myEl.removeInitedKey(initKey);
The method is described in the same file as $.fn.hasInitedKey, accordingly, you get both methods.
FAQs
Custom jQuery methods ($.fn)
The npm package custom-jquery-methods receives a total of 10 weekly downloads. As such, custom-jquery-methods popularity was classified as not popular.
We found that custom-jquery-methods demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.