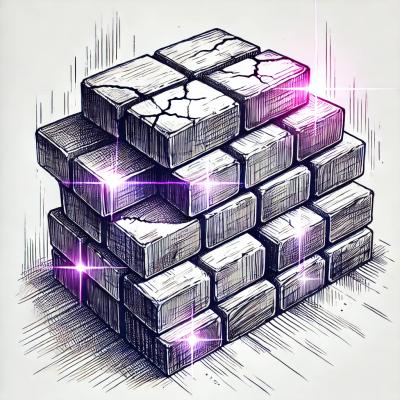
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
DateOnly
is a data type that will only store a date, without an associated time. This allows you to work with dates without having to worry about time zones shifting the date.
A simple example is a person's birthday - a birthday is not a single moment in time, but rather a full day. DateOnly
is the perfect data type to represent such data.
npm install dateonly
// CommonJS
var DateOnly = require('dateonly');
// AMD
require(['DateOnly'], function(DateOnly) { ... });
// Script Tag
var DateOnly = window.DateOnly;
var DateOnly = require('dateonly');
var myBirthday = new DateOnly('7/4/1980');
new DateOnly(defaultValue)
defaultValue: undefined
If nothing is passed into the DateOnly
constructor, it will default to today's date.
defaultValue: Date
If a Date
object is passed in, the DateOnly
instance will be set to the Date
's date.
defaultValue: String
If a String
is passed in, it will be passed to the Date
constructor, and that new Date
instance will then be used to set the DateOnly
's date.
defaultValue: Number
If a Number
is passed in, it will be passed to the Date
constructor, and that new Date
instance will then be used to set the DateOnly
's date.
new DateOnly().setDate(date)
Sets the date
portion of the DateOnly
instance.
date: Number
The number to set the date to. No validation is performed.
Example:
var date = new DateOnly('1/1/2000');
date.setDate(20);
console.log(date); // { date: 20, month: 0, year: 2000 }
date.setDate(80);
console.log(date); // { date: 80, month: 0, year: 2000 }
new DateOnly().getDate()
Gets the date
portion of the DateOnly
instance.
new DateOnly().setMonth(month)
Sets the month
portion of the DateOnly
instance. As with the Date
object, January === 0.
month: Number
The number to set the month to. No validation is performed.
new DateOnly().getMonth()
Gets the year
portion of the DateOnly
instance.
new DateOnly().setFullYear(year)
Sets the year
portion of the DateOnly
instance.
month: Number
The number to set the year to. No validation is performed.
new DateOnly().getFullYear()
Gets the year
portion of the DateOnly
instance.
new DateOnly().getDay()
Gets the day of the week of the DateOnly
instance. As with the Date
object, Sunday === 0.
new DateOnly().toDate()
Returns the Date
representation of the DateOnly
instance.
Example:
var dateOnly = new DateOnly('1/1/2000');
dateOnly.toDate() instanceof Date // true
dateOnly.toDate(); // Sat Jan 01 2000 00:00:00 GMT-0600 (CST)
new DateOnly().valueOf() | new DateOnly().toJSON()
Returns the numeric representation of the DateOnly
instance, of the form YYYYMMDD
.
Example:
var dateOnly = new DateOnly('7/15/2000');
dateOnly.valueOf() // 20000615
dateOnly.toJSON() // 20000615
new DateOnly().toString()
Returns the date string of the DateOnly
instance. This is equivalent to calling new DateOnly().toDate().toDateString()
.
Example:
var dateOnly = new DateOnly('7/4/2000');
dateOnly.toString() // 'Tue Jul 04 2000'
If you are using Mongo and Mongoose, there is a package that will allow you to store and query DateOnly
properties that can be found here.
##Test npm test
FAQs
A JavaScript class that stores a date without a time.
The npm package dateonly receives a total of 818 weekly downloads. As such, dateonly popularity was classified as not popular.
We found that dateonly demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.