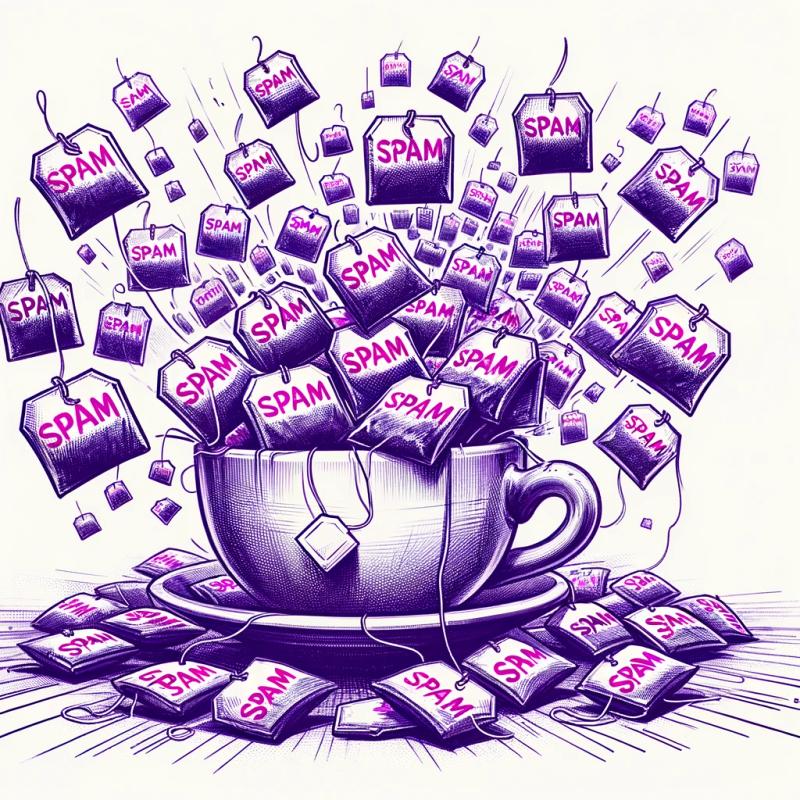
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
decompress
Advanced tools
Package description
The decompress npm package is a module that allows for decompressing archive files such as zip, tar, tar.gz, and many others. It provides a simple API to easily extract files from these archives.
Extracting archive files
This feature allows you to extract files from an archive like a zip file into a specified directory. The 'decompress' function returns a promise that resolves with an array of file objects when the extraction is complete.
const decompress = require('decompress');
decompress('unicorn.zip', 'dist').then(files => {
console.log('Files decompressed successfully');
});
Extracting specific files
This feature allows you to extract only specific files from an archive by using a filter function. The filter function is passed each file object, and you return a boolean to indicate whether the file should be extracted.
const decompress = require('decompress');
const filesToExtract = ['unicorn.png', 'rainbow.jpg'];
decompress('archive.zip', 'dist', {
filter: file => filesToExtract.includes(file.path)
}).then(files => {
console.log('Selected files decompressed successfully');
});
Using plugins to handle different archive types
The decompress package can be extended with plugins to support more archive types. In this example, the 'decompress-targz' plugin is used to decompress a '.tar.gz' file.
const decompress = require('decompress');
const decompressTargz = require('decompress-targz');
decompress('unicorn.tar.gz', 'dist', {
plugins: [
decompressTargz()
]
}).then(files => {
console.log('tar.gz file decompressed successfully');
});
adm-zip is a JavaScript implementation for zip data compression for NodeJS. It provides functionalities to read and write zip files, much like decompress, but it is focused solely on zip format.
yauzl is another npm package for reading and extracting zip files. It aims to be a low-level and high-performance library for zip file I/O, and unlike decompress, it does not support multiple file formats.
The 'tar' package provides the ability to create, extract, and list tar archives. It is similar to decompress in handling tar files but does not support other types of archives.
unzipper is a fully streaming unzip library for NodeJS, which provides a simple API for parsing and extracting zip files. It is similar to decompress but focuses on the zip format and offers a streaming interface.
Readme
Extracting archives made easy
See decompress-cli for the command-line version.
$ npm install decompress
const decompress = require('decompress');
decompress('unicorn.zip', 'dist').then(files => {
console.log('done!');
});
Returns a Promise for an array of files in the following format:
{
data: Buffer,
mode: Number,
mtime: String,
path: String,
type: String
}
Type: string
Buffer
File to decompress.
Type: string
Output directory.
Type: Function
Filter out files before extracting. E.g:
decompress('unicorn.zip', 'dist', {
filter: file => path.extname(file.path) !== '.exe'
}).then(files => {
console.log('done!');
});
Note that in the current implementation, filter
is only applied after fully reading all files from the archive in memory. Do not rely on this option to limit the amount of memory used by decompress
to the size of the files included by filter
. decompress
will read the entire compressed file into memory regardless.
Type: Function
Map files before extracting: E.g:
decompress('unicorn.zip', 'dist', {
map: file => {
file.path = `unicorn-${file.path}`;
return file;
}
}).then(files => {
console.log('done!');
});
Type: Array
Default: [decompressTar(), decompressTarbz2(), decompressTargz(), decompressUnzip()]
Array of plugins to use.
Type: number
Default: 0
Remove leading directory components from extracted files.
MIT © Kevin Mårtensson
FAQs
Extracting archives made easy
The npm package decompress receives a total of 2,254,485 weekly downloads. As such, decompress popularity was classified as popular.
We found that decompress demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.