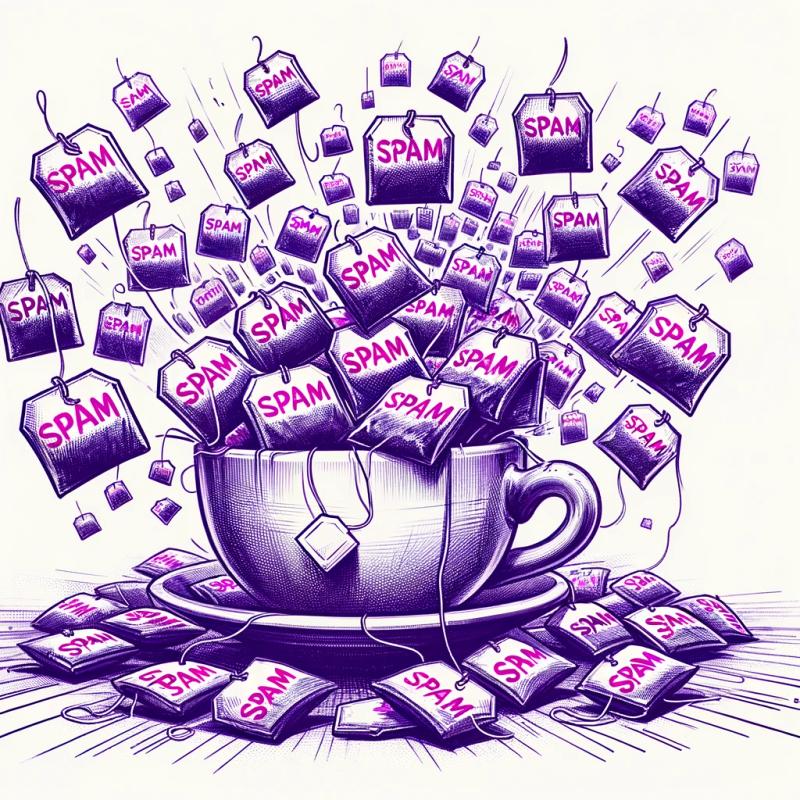
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
dijkstrajs
Advanced tools
Readme
dijkstrajs is a simple JavaScript implementation of Dijkstra's single-source shortest-paths algorithm.
The code was originally written by Wyatt Baldwin and turned into a node module by Thomas Cort.
npm install dijkstrajs
See test/dijkstra.test.js
in the sources for some example code.
FAQs
A simple JavaScript implementation of Dijkstra's single-source shortest-paths algorithm.
The npm package dijkstrajs receives a total of 1,984,321 weekly downloads. As such, dijkstrajs popularity was classified as popular.
We found that dijkstrajs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.