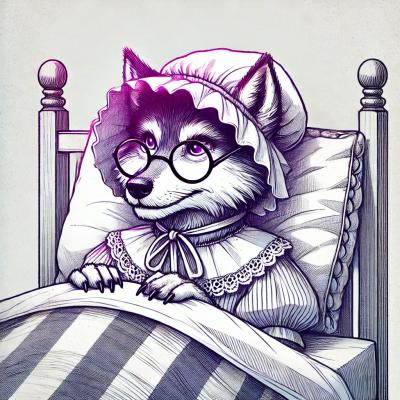
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
domhandler
Advanced tools
The domhandler npm package is a backend module used to handle and manipulate HTML and XML documents. It provides a way to build a DOM (Document Object Model) from HTML/XML strings, which can then be manipulated or queried programmatically. This is particularly useful for server-side applications where you need to parse and interact with HTML/XML content.
Building DOM from HTML
This code demonstrates how to use domhandler to parse an HTML string into a DOM structure. The `DomHandler` is used in conjunction with `htmlparser2` to parse the HTML and build the DOM.
const { parseDocument } = require('htmlparser2');
const { DomHandler } = require('domhandler');
const html = '<div><p>Hello World</p></div>';
const handler = new DomHandler((error, dom) => {
if (error) {
console.error(error);
} else {
console.log(dom);
}
});
const parser = new parseDocument(handler);
parser.write(html);
parser.end();
Manipulating DOM
This example shows how to manipulate the DOM after parsing. It changes the text inside a <p> tag from 'Hello World' to 'Hello DOMHandler'.
const { DomHandler } = require('domhandler');
const { parseDocument } = require('htmlparser2');
const html = '<div><p>Hello World</p></div>';
const handler = new DomHandler((error, dom) => {
if (!error) {
const pElement = dom[0].children[0];
pElement.firstChild.data = 'Hello DOMHandler';
console.log(pElement);
}
});
const parser = new parseDocument(handler);
parser.write(html);
parser.end();
Cheerio is a fast, flexible, and lean implementation of core jQuery designed specifically for the server. It uses a very similar approach to domhandler but provides a jQuery-like API for manipulating the DOM, making it more familiar to those who have used jQuery. Unlike domhandler, which is more low-level, cheerio abstracts many of the complexities involved in DOM manipulation.
jsdom is another popular npm package that allows you to create a web browser environment from Node.js. It simulates a web page by creating a realistic document structure. While domhandler is primarily used for handling and manipulating DOM elements, jsdom provides a more comprehensive simulation of a web environment, including scripting and event capabilities.
The DOM handler creates a tree containing all nodes of a page. The tree can be manipulated using the domutils or cheerio libraries and rendered using dom-serializer .
const handler = new DomHandler([ <func> callback(err, dom), ] [ <obj> options ]);
// const parser = new Parser(handler[, options]);
Available options are described below.
const { Parser } = require("htmlparser2");
const { DomHandler } = require("domhandler");
const rawHtml =
"Xyz <script language= javascript>var foo = '<<bar>>';</script><!--<!-- Waah! -- -->";
const handler = new DomHandler((error, dom) => {
if (error) {
// Handle error
} else {
// Parsing completed, do something
console.log(dom);
}
});
const parser = new Parser(handler);
parser.write(rawHtml);
parser.end();
Output:
[
{
data: "Xyz ",
type: "text",
},
{
type: "script",
name: "script",
attribs: {
language: "javascript",
},
children: [
{
data: "var foo = '<bar>';<",
type: "text",
},
],
},
{
data: "<!-- Waah! -- ",
type: "comment",
},
];
withStartIndices
Add a startIndex
property to nodes.
When the parser is used in a non-streaming fashion, startIndex
is an integer
indicating the position of the start of the node in the document.
The default value is false
.
withEndIndices
Add an endIndex
property to nodes.
When the parser is used in a non-streaming fashion, endIndex
is an integer
indicating the position of the end of the node in the document.
The default value is false
.
normalizeWhitespace
(deprecated)Replace all whitespace with single spaces.
The default value is false
.
Note: Enabling this might break your markup.
For the following examples, this HTML will be used:
<font> <br />this is the text <font></font></font>
normalizeWhitespace: true
[
{
type: "tag",
name: "font",
children: [
{
data: " ",
type: "text",
},
{
type: "tag",
name: "br",
},
{
data: "this is the text ",
type: "text",
},
{
type: "tag",
name: "font",
},
],
},
];
normalizeWhitespace: false
[
{
type: "tag",
name: "font",
children: [
{
data: "\n\t",
type: "text",
},
{
type: "tag",
name: "br",
},
{
data: "this is the text\n",
type: "text",
},
{
type: "tag",
name: "font",
},
],
},
];
License: BSD-2-Clause
To report a security vulnerability, please use the Tidelift security contact. Tidelift will coordinate the fix and disclosure.
domhandler
for enterpriseAvailable as part of the Tidelift Subscription
The maintainers of domhandler
and thousands of other packages are working with Tidelift to deliver commercial support and maintenance for the open source dependencies you use to build your applications. Save time, reduce risk, and improve code health, while paying the maintainers of the exact dependencies you use. Learn more.
FAQs
Handler for htmlparser2 that turns pages into a dom
The npm package domhandler receives a total of 7,504,587 weekly downloads. As such, domhandler popularity was classified as popular.
We found that domhandler demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.