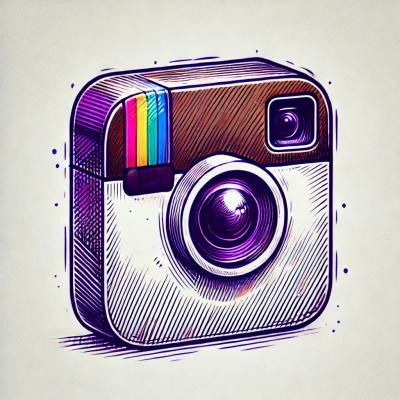
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
dropbox-fs
Advanced tools
Node fs
wrapper for Dropbox. Wraps the Dropbox javascript module with an async fs
-like API so it can be used where a fileSystem API is expected.
To use this module you'll need a Dropbox Access Token.
$ npm install --save dropbox-fs
const dfs = require('dropbox-fs')({
apiKey: 'DROPBOX_API_KEY_HERE'
});
dfs.readdir('/Public', (err, result) => {
console.log(result); // Array of files and folders
});
Promises are also supported.
const dfs = require('dropbox-fs/promises')({
apiKey: 'DROPBOX_API_KEY_HERE'
});
You can also pass in a client
option if you’re using your own dropbox
module instead of the apiKey
.
If you'd like some peace of mind then there's a read-only option too:
const dfs = require('dropbox-fs/readonly')({
apiKey: 'DROPBOX_API_KEY_HERE'
});
// Methods that might change data now return an error without performing any action:
// - mkdir
// - rename
// - rmdir
// - unlink
// - writeFile
dfs.unlink('/Public', (err) => {
console.log(err); // Message saying `unlink` is not supported in read-only
});
This module exposes the following methods:
Reads a directory and returns a list of files and folders inside.
dfs.readdir('/', (err, result) => {
console.log(result);
});
path
: String|Buffer
callback
: Function
Creates a directory.
dfs.mkdir('/Public/Resume', (err, stat) => {
console.log(stat.name); // Resume
console.log(stat.isDirectory()); // true
});
path
: String|Buffer
callback
: Function
Deletes a directory.
dfs.rmdir('/Public/Resume', err => {
if (!err) {
console.log('Deleted.');
}
});
path
: String|Buffer
callback
: Function
Reads a file and returns it’s contents.
// Buffer:
dfs.readFile('/Work/doc.txt', (err, result) => {
console.log(result.toString('utf8'));
});
// String
dfs.readFile('/Work/doc.txt', {encoding: 'utf8'}, (err, result) => {
console.log(result);
});
path
: String|Buffer
options
: Optional String|Object
encoding
: String
callback
: Function
If you pass a string as the options
parameter, it will be used as options.encoding
. If you don’t provide an encoding, a raw Buffer
will be passed to the callback.
Writes a file to the remote API and returns it’s stat
.
const content = fs.readFileSync('./localfile.md');
dfs.writeFile('/Public/doc.md', content, {encoding: 'utf8'}, (err, stat) => {
console.log(stat.name); // doc.md
});
path
: String|Buffer
data
: String|Buffer
options
: Optional String|Object
encoding
: String
overwrite
: Boolean
Default: true
.callback
: Function
Renames a file (moves it to a new location).
dfs.rename('/Public/doc.md', '/Backups/doc-backup.md', err => {
if err {
console.error('Failed!');
} else {
console.log('Moved!');
}
});
path
: String|Buffer
data
: String|Buffer
callback
: Function
Returns the file/folder information.
dfs.stat('/Some/Remote/Folder/', (err, stat) => {
console.log(stat.isDirectory()); // true
console.log(stat.name); // Folder
});
path
: String|Buffer
callback
: Function
err
: null|Error
stat
: Stat ObjectDeletes a file.
dfs.unlink('/Path/To/file.txt', err => {
if (!err) {
console.log('Deleted!');
}
});
path
: String|Buffer
callback
: Function
Creates a readable stream.
const stream = fs.createReadStream('/test/test1.txt');
stream.on('data', data => {
console.log('data', data);
});
stream.on('end', () => {
console.log('stream finished');
});
path
: String|Buffer
Creates a writable stream.
const stream = fs.createWriteStream('/test/test1.txt');
someStream().pipe(stream).on('finish', () => {
console.log('write stream finished');
});
path
: String|Buffer
The stat object that is returned from writeFile()
and stat()
contains two methods in addition to some standard information like name
, etc:
stat.isDirectory()
Returns true
if the target is a directorystat.isFile()
Returns true
if the target is a fileThis software is released under the MIT License.
FAQs
Node FS wrapper for Dropbox API
The npm package dropbox-fs receives a total of 14 weekly downloads. As such, dropbox-fs popularity was classified as not popular.
We found that dropbox-fs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.