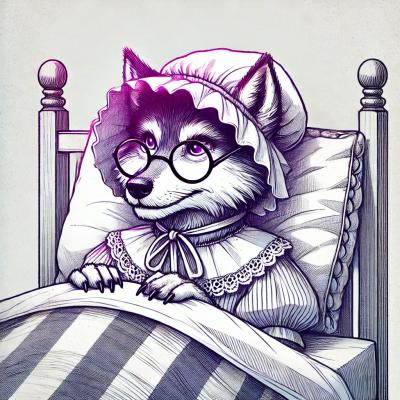
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The duplexer2 npm package is a utility that allows you to create a duplex stream from two separate streams, one readable and one writable. This is particularly useful in scenarios where you need to treat two separate streams as a single duplex (both readable and writable) stream, enabling more flexible and efficient data handling in Node.js applications.
Creating a duplex stream from a readable and writable stream
This code demonstrates how to create a duplex stream using duplexer2 by combining a readable and a writable stream. The resulting `duplexStream` can be both read from and written to, acting as a bridge between the two original streams.
const { Duplex } = require('stream');
const duplexer2 = require('duplexer2');
const readableStream = new Duplex();
const writableStream = new Duplex();
const duplexStream = duplexer2(writableStream, readableStream);
Duplexify is similar to duplexer2 in that it allows for the creation of a duplex stream from a writable and readable stream. However, duplexify provides additional features such as automatic detection of finish and end events, and it can also turn a writable and readable stream into a duplex stream even if one of them is not yet available.
Through2 is a tiny wrapper around Node.js streams.Transform (a standard duplex stream) to make defining custom transform streams easier. While it doesn't directly offer the same functionality as duplexer2, it simplifies the process of creating transform streams, which can be used in combination with other streams to achieve similar duplex stream setups.
Pumpify combines an array of streams into a single duplex stream, handling errors and cleanup automatically. It's similar to duplexer2 in that it creates a duplex stream, but it focuses on combining multiple streams into one, which can be particularly useful for setting up pipelines of transform streams.
Like duplexer (http://npm.im/duplexer) but using streams2.
duplexer2 is a reimplementation of duplexer using the readable-stream API which is standard in node as of v0.10. Everything largely works the same.
Available via npm:
$ npm install duplexer2
Or via git:
$ git clone git://github.com/deoxxa/duplexer2.git node_modules/duplexer2
duplexer2
Creates a new DuplexWrapper
object, which is the actual class that implements
most of the fun stuff. All that fun stuff is hidden. DON'T LOOK.
duplexer2([options], writable, readable)
var duplex = duplexer2(new stream.Writable(), new stream.Readable());
Arguments
stream.Duplex
options, as
well as the properties described below.Options
true
.Also see example.js.
Code:
var stream = require("stream");
var duplexer2 = require("duplexer2");
var writable = new stream.Writable({objectMode: true}),
readable = new stream.Readable({objectMode: true});
writable._write = function _write(input, encoding, done) {
if (readable.push(input)) {
return done();
} else {
readable.once("drain", done);
}
};
readable._read = function _read(n) {
// no-op
};
// simulate the readable thing closing after a bit
writable.once("finish", function() {
setTimeout(function() {
readable.push(null);
}, 500);
});
var duplex = duplexer2(writable, readable);
duplex.on("data", function(e) {
console.log("got data", JSON.stringify(e));
});
duplex.on("finish", function() {
console.log("got finish event");
});
duplex.on("end", function() {
console.log("got end event");
});
duplex.write("oh, hi there", function() {
console.log("finished writing");
});
duplex.end(function() {
console.log("finished ending");
});
Output:
got data "oh, hi there"
finished writing
got finish event
finished ending
got end event
3-clause BSD. A copy is included with the source.
FAQs
Like duplexer but using streams3
The npm package duplexer2 receives a total of 4,713,853 weekly downloads. As such, duplexer2 popularity was classified as popular.
We found that duplexer2 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.