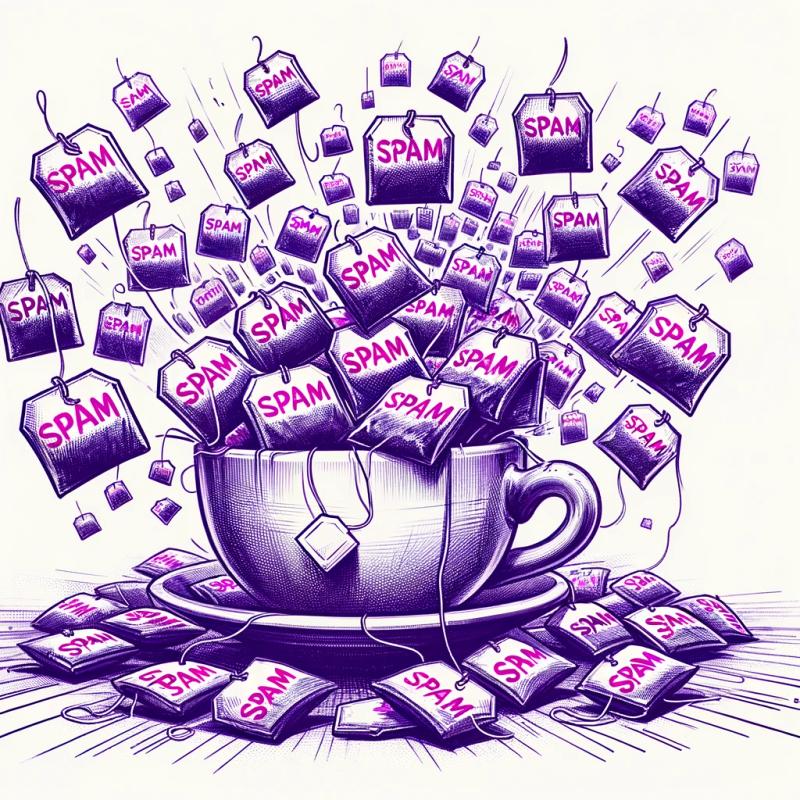
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
easings-css
Advanced tools
Changelog
Readme
Provides easing functions for CSS.
Available as CSS Custom Properties, JavaScript modules, JSON object and Less and Sass variables.
@import 'easings-css';
:warning: If you're not using postcss-import or similar to automatically resolve
node_modules
, you'll need to manually resolve to the path:node_modules/easings-css/index.css
.
.example {
transition-timing-function: var(--ease-out-quad);
}
@import 'easings-css';
.example {
transition-timing-function: @ease-out-quad;
}
For Sass v3.6.0 and newer:
@import 'node_modules/easings-css';
For all other versions:
@import 'node_modules/easings-css/easings';
.example {
transition-timing-function: $ease-out-quad;
}
const easings = require('easings-css');
const Example = styled.div`
transition-timing-function: ${easings.easeOutQuad};
`;
import * as easings from 'easings-css';
You can also import individual easings for better tree-shaking:
import { easeOutQuad } from 'easings-css';
const Example = styled.div`
transition-timing-function: ${easeOutQuad};
`;
cubic-bezier(0.47, 0, 0.745, 0.715)
CSS | LESS | SASS | JS |
---|---|---|---|
var(--easeInSine) orvar(--ease-in-sine) | @easeInSine or@ease-in-sine | $easeInSine or$ease-in-sine | easeInSine |
cubic-bezier(0.39, 0.575, 0.565, 1)
CSS | LESS | SASS | JS |
---|---|---|---|
var(--easeOutSine) orvar(--ease-out-sine) | @easeOutSine or@ease-out-sine | $easeOutSine or$ease-out-sine | easeOutSine |
cubic-bezier(0.445, 0.05, 0.55, 0.95)
CSS | LESS | SASS | JS |
---|---|---|---|
var(--easeInOutSine) orvar(--ease-in-out-sine) | @easeInOutSine or@ease-in-out-sine | $easeInOutSine or$ease-in-out-sine | easeInOutSine |
cubic-bezier(0.55, 0.085, 0.68, 0.53)
CSS | LESS | SASS | JS |
---|---|---|---|
var(--easeInQuad) orvar(--ease-in-quad) | @easeInQuad or@ease-in-quad | $easeInQuad or$ease-in-quad | easeInQuad |
cubic-bezier(0.25, 0.46, 0.45, 0.94)
CSS | LESS | SASS | JS |
---|---|---|---|
var(--easeOutQuad) orvar(--ease-out-quad) | @easeOutQuad or@ease-out-quad | $easeOutQuad or$ease-out-quad | easeOutQuad |
cubic-bezier(0.455, 0.03, 0.515, 0.955)
CSS | LESS | SASS | JS |
---|---|---|---|
var(--easeInOutQuad) orvar(--ease-in-out-quad) | @easeInOutQuad or@ease-in-out-quad | $easeInOutQuad or$ease-in-out-quad | easeInOutQuad |
cubic-bezier(0.55, 0.055, 0.675, 0.19)
CSS | LESS | SASS | JS |
---|---|---|---|
var(--easeInCubic) orvar(--ease-in-cubic) | @easeInCubic or@ease-in-cubic | $easeInCubic or$ease-in-cubic | easeInCubic |
cubic-bezier(0.215, 0.61, 0.355, 1)
CSS | LESS | SASS | JS |
---|---|---|---|
var(--easeOutCubic) orvar(--ease-out-cubic) | @easeOutCubic or@ease-out-cubic | $easeOutCubic or$ease-out-cubic | easeOutCubic |
cubic-bezier(0.645, 0.045, 0.355, 1)
CSS | LESS | SASS | JS |
---|---|---|---|
var(--easeInOutCubic) orvar(--ease-in-out-cubic) | @easeInOutCubic or@ease-in-out-cubic | $easeInOutCubic or$ease-in-out-cubic | easeInOutCubic |
cubic-bezier(0.895, 0.03, 0.685, 0.22)
CSS | LESS | SASS | JS |
---|---|---|---|
var(--easeInQuart) orvar(--ease-in-quart) | @easeInQuart or@ease-in-quart | $easeInQuart or$ease-in-quart | easeInQuart |
cubic-bezier(0.165, 0.84, 0.44, 1)
CSS | LESS | SASS | JS |
---|---|---|---|
var(--easeOutQuart) orvar(--ease-out-quart) | @easeOutQuart or@ease-out-quart | $easeOutQuart or$ease-out-quart | easeOutQuart |
cubic-bezier(0.77, 0, 0.175, 1)
CSS | LESS | SASS | JS |
---|---|---|---|
var(--easeInOutQuart) orvar(--ease-in-out-quart) | @easeInOutQuart or@ease-in-out-quart | $easeInOutQuart or$ease-in-out-quart | easeInOutQuart |
cubic-bezier(0.755, 0.05, 0.855, 0.06)
CSS | LESS | SASS | JS |
---|---|---|---|
var(--easeInQuint) orvar(--ease-in-quint) | @easeInQuint or@ease-in-quint | $easeInQuint or$ease-in-quint | easeInQuint |
cubic-bezier(0.23, 1, 0.32, 1)
CSS | LESS | SASS | JS |
---|---|---|---|
var(--easeOutQuint) orvar(--ease-out-quint) | @easeOutQuint or@ease-out-quint | $easeOutQuint or$ease-out-quint | easeOutQuint |
cubic-bezier(0.86, 0, 0.07, 1)
CSS | LESS | SASS | JS |
---|---|---|---|
var(--easeInOutQuint) orvar(--ease-in-out-quint) | @easeInOutQuint or@ease-in-out-quint | $easeInOutQuint or$ease-in-out-quint | easeInOutQuint |
cubic-bezier(0.95, 0.05, 0.795, 0.035)
CSS | LESS | SASS | JS |
---|---|---|---|
var(--easeInExpo) orvar(--ease-in-expo) | @easeInExpo or@ease-in-expo | $easeInExpo or$ease-in-expo | easeInExpo |
cubic-bezier(0.19, 1, 0.22, 1)
CSS | LESS | SASS | JS |
---|---|---|---|
var(--easeOutExpo) orvar(--ease-out-expo) | @easeOutExpo or@ease-out-expo | $easeOutExpo or$ease-out-expo | easeOutExpo |
CSS | LESS | SASS | JS |
---|---|---|---|
var(--easeInOutExpo) orvar(--ease-in-out-expo) | @easeInOutExpo or@ease-in-out-expo | $easeInOutExpo or$ease-in-out-expo | easeInOutExpo |
cubic-bezier(0.6, 0.04, 0.98, 0.335)
CSS | LESS | SASS | JS |
---|---|---|---|
var(--easeInCirc) orvar(--ease-in-circ) | @easeInCirc or@ease-in-circ | $easeInCirc or$ease-in-circ | easeInCirc |
cubic-bezier(0.075, 0.82, 0.165, 1)
CSS | LESS | SASS | JS |
---|---|---|---|
var(--easeOutCirc) orvar(--ease-out-circ) | @easeOutCirc or@ease-out-circ | $easeOutCirc or$ease-out-circ | easeOutCirc |
cubic-bezier(0.785, 0.135, 0.15, 0.86)
CSS | LESS | SASS | JS |
---|---|---|---|
var(--easeInOutCirc) orvar(--ease-in-out-circ) | @easeInOutCirc or@ease-in-out-circ | $easeInOutCirc or$ease-in-out-circ | easeInOutCirc |
cubic-bezier(0.6, -0.28, 0.735, 0.045)
CSS | LESS | SASS | JS |
---|---|---|---|
var(--easeInBack) orvar(--ease-in-back) | @easeInBack or@ease-in-back | $easeInBack or$ease-in-back | easeInBack |
cubic-bezier(0.175, 0.885, 0.32, 1.275)
CSS | LESS | SASS | JS |
---|---|---|---|
var(--easeOutBack) orvar(--ease-out-back) | @easeOutBack or@ease-out-back | $easeOutBack or$ease-out-back | easeOutBack |
cubic-bezier(0.68, -0.55, 0.265, 1.55)
CSS | LESS | SASS | JS |
---|---|---|---|
var(--easeInOutBack) orvar(--ease-in-out-back) | @easeInOutBack or@ease-in-out-back | $easeInOutBack or$ease-in-out-back | easeInOutBack |
FAQs
Easing functions for CSS, provided as CSS Custom Properties, JavaScript modules, JSON object and Less and Sass variables.
The npm package easings-css receives a total of 37,641 weekly downloads. As such, easings-css popularity was classified as popular.
We found that easings-css demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.