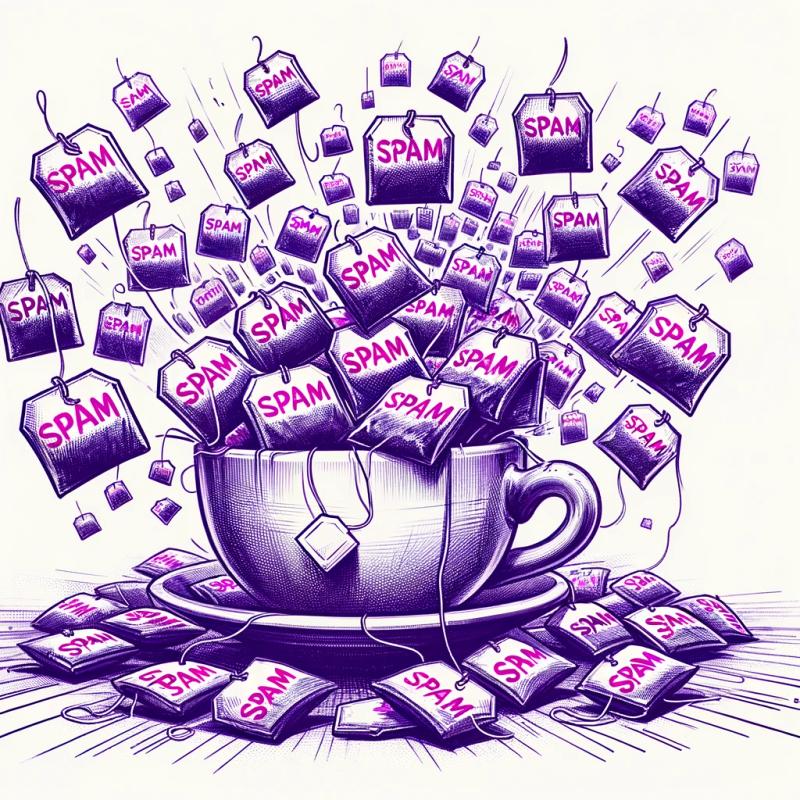
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
easy-ini
Advanced tools
Readme
why should you use this package?
const INI = require('easy-ini')
const myINI = new INI('cool=awesome')
myINI.putStringInSection('; so important','[WOW]')
const giveItBack = myINI.createINIString()
constructor(,{}) Create ini object
str
,defSec
= 'DEFAULT_SECTION!',eol
= require('os').EOL)autoTrim
= true// You can start with an empty INI
const myINI = new INI('')
// By default the EOL is the taken from the OS, set it if needed
const myINI = new INI('',{eol: '\r\n'})
// DebSec is needed to represent the first lines that may not be under a section
// By default it's: [DEFAULT_SECTION!] , if for some reason you actually use this name for a section, provide another to avoid unwanted behavior
const myINI = new INI('',{defSec: 'NEXT_LEVEL_DEFAULT_SECTION'})
createINIString
({})
Make an ini string from the object, every param is optional and do what it says
// To get true representation use without any parameter
const newINIString = myINI.createINIString()
createSimpleObject
()
Make an Object including only they types that are specified
// by passing [3,4] , will also create a level for sections
const newINIString = myINI.createSimpleObject()
getKeyIfExists
()
Get line object by referance {type, key, val}
// Changing the returned object will change the original
myINI.getKeyIfExists('cool').val = 'ouch'
// Returnes null if failed to find
findAndChangeKeyIfExists
()
finds a pair by key and replace value
// Same as using getKeyIfExists but without returning referance
myINI.findAndChangeKeyIfExists('cool','OUCH')
// Returnes true for success, otherwize false
findAndRemoveKeyIfExists
()
remove key value pair from object
// Remove a pair (key=value) by matching the key with input string
myINI.findAndRemoveKeyIfExists('cool')
// Returnes true for success, otherwize false
removeEverythingButSections
()
remove all other sections
// wanting to remove everything else
myINI.removeEverythingButSections(['[GLOBALS]'])
// can also be ussed for partial mataches
myINI.removeEverythingButSections(['GLOB'], true)
findAndRemoveSectionIfExists
()
remove entire section from object
// Remove an entire section
myINI.findAndRemoveSectionIfExists('[DO_NOT_REMOVE]')
// Returnes true for success, otherwize false
putStringInSection
()
adds a line to the end of a section
// If section does not exist, will create it at the end
myINI.putStringInSection('#comment','[BLAHBLAH]')
// If the input is a key=value pair, and key exists in section , will change its value
// Also true when no section is provided (will use default one)
getLinesByMatch
()
find all lines containing a string
// Will return an array with with all matches across all sections
myINI.getLinesByMatch('#INCLUDE=')
removeLineByMatch
()
matches keys, values or comments
// Will return true if at least one line was removed, else false
myINI.removeLineByMatch(';DUAH', true)
findAndReplace
()
searches for the token and replaces with the value if found
// if global is false will change only the first occurrence
myINI.findAndReplace('<<BASE_DOMAIN>>', 'mashu-mashu-mashu.com', true)
// Will return true if at least one line was removed, else false
solveDuplicates
()
fixes ini object so and removes duplicate keys leaving first or last occurence
// Will remove duplicate keys across the entire ini object
myINI.solveDuplicates()
// Returnes true when finished
solveSelfReferences
()
use values to replace matching content wrapped by a given prefix and suffix
/* super_secret_config.ini:
something=whatever
say=ha
dude=%say%%say%%say% %relax%
relax=%something% */
myINI.solveSelfReferences('%', '%')
/* ==>
something=whatever
say=ha
dude=hahaha whatever
relax=whatever */
mergeWith
()
merges with another ini object
// If before is true will place new values at the beginning of each section
myINI.mergeWith(notMyINI)
myINI.iniData
directlyconst fs = require('fs')
const INI = require('easy-ini')
const productConfig = new INI(fs.readFileSync('./amazing_app_info.ini',{encoding: 'utf8'}))
let includes
while (includes = productConfig.getLinesByMatch("#INCLUDE")){
if (includes.length == 0) {break}
productConfig.removeLineByMatch('#INCLUDE', true)
for (const include of includes.reverse()) {
const includePath = include.split('=')[1]
const tempINI = new INI(fs.readFileSync(includePath, {encoding: 'utf8'}))
productConfig.mergeWith(tempINI, true)
}
}
productConfig.solveDuplicates()
const finalConfig = productConfig.createINIString()
fs.writeFileSync("./final.ini", finalConfig)
const fs = require('fs')
const INI = require('easy-ini')
const webCon = new INI(fs.readFileSync('./website_config.ini',{encoding: 'utf8'}))
// latest=GGEZ.v69.zip
// download-url=<<SubDomain>>.<<BaseDomain>>/download/%latest%
webCon.findAndReplace('<<BaseDomain>>', 'easy-ini.com', true)
webCon.findAndReplace('<<SubDomain>>', 'download', true)
webCon.findAndReplace('<<Author>>', 'Gal Angel', true)
webCon.solveSelfReferences('%', '%')
const upCon = webCon.createINIString()
fs.writeFileSync("./to_upload.ini", upCon)
GNU General Public License v3.0
FAQs
manage ini files content
The npm package easy-ini receives a total of 17 weekly downloads. As such, easy-ini popularity was classified as not popular.
We found that easy-ini demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.