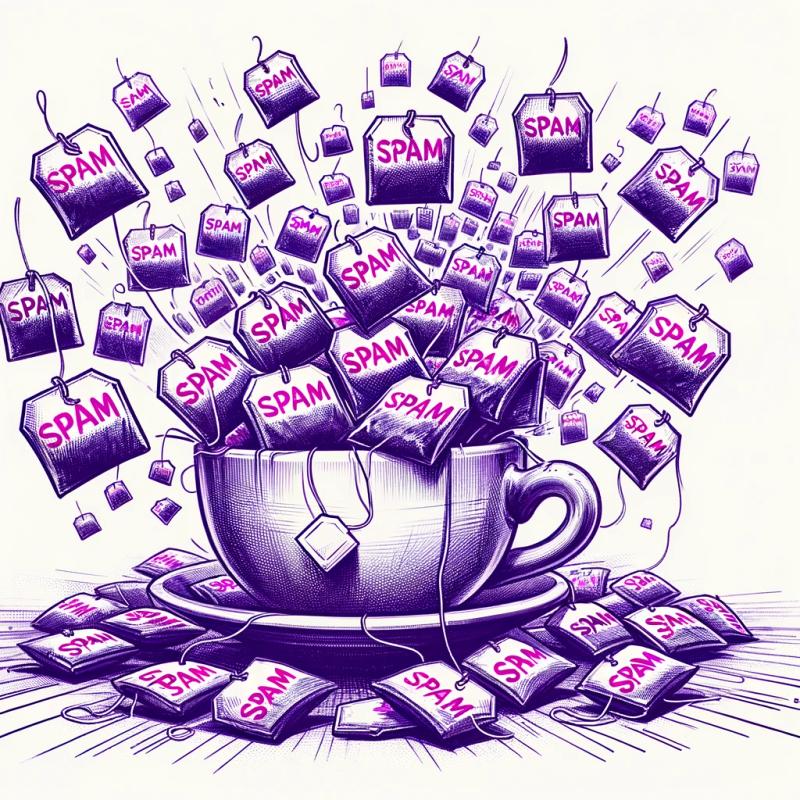
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
ee-first
Advanced tools
Package description
The ee-first package is a utility for Node.js that allows you to wait for multiple EventEmitter or Stream instances to emit an event or error and then execute a callback. It is particularly useful when you need to listen for an event from multiple sources and want to take action only after the first one occurs.
Listening for the first event or error from multiple emitters
This feature allows you to pass an array of arrays, where each sub-array contains an EventEmitter instance followed by one or more event names. The callback function is then executed when the first event from any of the listed emitters is emitted.
const first = require('ee-first');
const stream1 = getSomeReadableStream();
const stream2 = getAnotherReadableStream();
const thunk = first([
[stream1, 'end', 'error'],
[stream2, 'end', 'error']
], function (err, ee, event, args) {
// This function will be called when one of the streams emits 'end' or 'error'
// `ee` is the event emitter that fired
// `event` is the string name of the event that fired
// `args` is an array of the arguments that were passed to the event
});
The event-promise package provides a way to wait for an event to be emitted using promises. It is similar to ee-first in that it deals with events, but it works with individual emitters and events rather than a collection of them.
Await-event is another package that allows you to wait for an event to be emitted, but it is designed to be used with async/await syntax for a single EventEmitter. Unlike ee-first, it does not handle multiple emitters.
Event-to-promise converts events to promises, similar to event-promise. It allows you to wait for an event on an EventEmitter, but it does not provide the functionality to listen to multiple emitters and act on the first event like ee-first does.
Readme
Get the first event in a set of event emitters and event pairs, then clean up after itself.
$ npm install ee-first
var first = require('ee-first')
Invoke listener
on the first event from the list specified in arr
. arr
is
an array of arrays, with each array in the format [ee, ...event]
. listener
will be called only once, the first time any of the given events are emitted. If
error
is one of the listened events, then if that fires first, the listener
will be given the err
argument.
The listener
is invoked as listener(err, ee, event, args)
, where err
is the
first argument emitted from an error
event, if applicable; ee
is the event
emitter that fired; event
is the string event name that fired; and args
is an
array of the arguments that were emitted on the event.
var ee1 = new EventEmitter()
var ee2 = new EventEmitter()
first([
[ee1, 'close', 'end', 'error'],
[ee2, 'error']
], function (err, ee, event, args) {
// listener invoked
})
FAQs
return the first event in a set of ee/event pairs
The npm package ee-first receives a total of 22,367,630 weekly downloads. As such, ee-first popularity was classified as popular.
We found that ee-first demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.