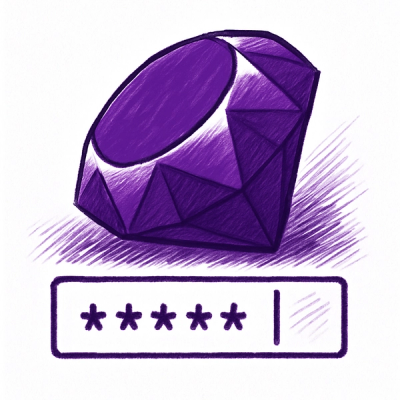
Research
/Security News
60 Malicious Ruby Gems Used in Targeted Credential Theft Campaign
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
eventemitter-callback
Advanced tools
Emit an event and waiting for a result. Based on eventemitter3.
By package manager:
npm install eventemitter-callback --save
# For Yarn, use the command below.
yarn add eventemitter-callback
Installation from CDN:
<!-- For UNPKG use the code below. -->
<script src="https://unpkg.com/eventemitter-callback"></script>
<!-- For JSDelivr use the code below. -->
<script src="https://cdn.jsdelivr.net/npm/eventemitter-callback"></script>
<script>
// UMD module is exposed through the "EventEmitterCB" global variable.
console.log(EventEmitterCB);
</script>
import emitter from 'eventemitter-callback'
emitter.on('event-name', (arg) => {
console.log(arg) // prints "ping"
return 'pong'
})
// emit an event and waiting for its result
emitter.emit('event-name', 'ping', (arg) => {
console.log(arg) // prints "pong"
})
By default, the following works normally:
emitter.emit('msg', 1)
emitter.on('msg', (arg) => {
console.log(arg) // prints 1
})
If this doesn't meet your expectations, you can disable it:
emitter.disablePreEmitter();
emitter.emit('msg', 1)
emitter.on('msg', (arg) => {
console.log(arg) // will not be called!
})
Adds the listener
function to the end of the listeners array for the event named eventName
.
string
the name of the eventfunction
the callback functionThe listener
function support AsyncFunction
or returns a Promise
emitter.on('event-name', (arg) => {
console.log(arg) // prints "ping"
return new Promise((resolve) => {
resolve('pong')
})
})
// emitter.on('event-name', async (arg) => {
// console.log(arg) // prints "ping"
// const res = await queryRemote() // suppose res returns 'pong'
// return res
// })
emitter.emit('event-name', 'ping', (arg) => {
console.log(arg) // prints "pong"
})
string
the name of the eventfunction
the callback functionAdds a one-time listener
function for the event named eventName
.
string
the name of the eventany
any
the callback functionReturns true
if the event had listeners, false
otherwise.
string
the name of the eventany
Trigger all the listeners at once. Returns a result array by Promise.all
.
string
the name of the eventany
Trigger all the listeners at once and check the values returned.
Returns true
if all listeners returns true, false
otherwise.
string
the name of the eventany
Trigger all the listeners at once and check the values returned.
Returns true
if either listener returns true, false
otherwise.
string
the name of the eventfunction
the callback functionRemoves the specified listener
from the listener array for the event named eventName
.
Removes all the listeners for the event:
emitter.off('event-name')
Reference to current instance of EventEmitter3.
Reference to constructor of EventEmitter3.
MIT, see the LICENSE file for detail.
FAQs
Emit an event and waiting for a result.
The npm package eventemitter-callback receives a total of 0 weekly downloads. As such, eventemitter-callback popularity was classified as not popular.
We found that eventemitter-callback demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
Security News
The CNA Scorecard ranks CVE issuers by data completeness, revealing major gaps in patch info and software identifiers across thousands of vulnerabilities.
Research
/Security News
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.