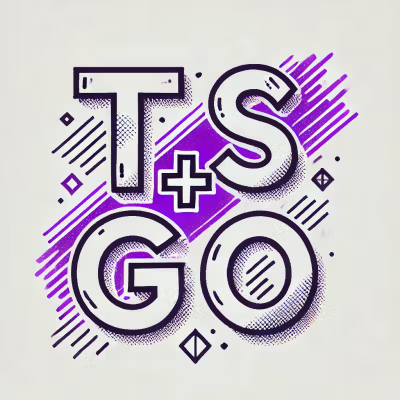
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
expect-more
Advanced tools
Curried JavaScript Type Testing Library with Zero Dependencies
npm install expect-more --save-dev
import { endsWith, isWithinRange } from 'expect-more';
const result: boolean = endsWith('Script', 'JavaScript');
// => true
const endsWithScript = endsWith('Script');
endsWithScript('JavaScript');
// => true
isWithinRange(10, 20, 21);
// => false
[0, 1, 1, 2, 3, 5, 8, 13, 21, 34].filter(isWithinRange(5, 15));
// => [5, 8, 13]
expect-more
declare const isBoolean: (value: unknown) => value is boolean;
declare const isFalse: (value: unknown) => value is false;
declare const isNull: (value: unknown) => value is null;
declare const isRegExp: (value: unknown) => value is RegExp;
declare const isTrue: (value: unknown) => value is true;
declare const isUndefined: (value: unknown) => value is undefined;
declare const isAsyncFunction: <T = (...args: any[]) => Promise<any>>(
value: unknown,
) => value is T;
declare const isFunction: <T = (...args: any[]) => any>(value: unknown) => value is T;
declare const isGeneratorFunction: <T = Generator>(value: unknown) => value is T;
declare const throwsAnyError: <T = (...args: any[]) => any>(value: unknown) => value is T;
declare const throwsErrorOfType: <T = (...args: any[]) => any>(
typeName: string,
value: unknown,
) => value is T;
declare const hasMember: <T = any>(memberName: string, value: unknown) => value is T;
declare const isEmptyObject: <T = any>(value: unknown) => value is T;
declare const isNil: (value: unknown) => value is undefined | null;
declare const isNonEmptyObject: <T = any>(value: unknown) => value is T;
declare const isObject: <T = any>(value: unknown) => value is T;
declare const isWalkable: <T = any>(value: unknown) => value is T;
declare const isArray: <T extends any[] = any[]>(value: unknown) => value is T;
declare const isArrayIncludingAllOf: <T extends any[] = any[]>(needed: any[], value: unknown) => value is T;
declare const isArrayIncludingAnyOf: <T extends any[] = any[]>(needed: any[], value: unknown) => value is T;
declare const isArrayIncludingOnly: <T extends any[] = any[]>(needed: any[], value: unknown) => value is T;
declare const isArrayOfBooleans: (value: unknown) => value is boolean[];
declare const isArrayOfNumbers: (value: unknown) => value is number[];
declare const isArrayOfObjects: <T extends any[] = any[]>(value: unknown) => value is T;
declare const isArrayOfSize: <T extends any[] = any[]>(
size: number,
value: unknown,
) => value is T;
declare const isArrayOfStrings: (value: unknown) => value is string[];
declare const isEmptyArray: <T extends [] = []>(any) => value is T;
declare const isNonEmptyArray: <T extends any[] = any[]>(value: unknown) => value is T;
declare const isAfter: (other: Date, value: unknown) => value is Date;
declare const isBefore: (other: Date, value: unknown) => value is Date;
declare const isDate: (value: unknown) => value is Date;
declare const isDateBetween: (floor: Date, ceiling: Date, value: unknown) => value is Date;
declare const isDateInMonth: (index: number, value: unknown) => value is Date;
declare const isDateInYear: (year: number, value: unknown) => value is Date;
declare const isDateOnDayOfMonth: (day: number, value: unknown) => value is Date;
declare const isDateOnDayOfWeek: (index: number, value: unknown) => value is Date;
declare const isDateOnOrAfter: (other: Date, value: unknown) => value is Date;
declare const isDateOnOrBefore: (other: Date, value: unknown) => value is Date;
declare const isValidDate: (value: unknown) => value is Date;
declare const isCalculable: (value: unknown) => value is number;
declare const isDecimalNumber: (value: unknown) => value is number;
declare const isDivisibleBy: (other: number, value: unknown) => value is number;
declare const isEvenNumber: (value: unknown) => value is number;
declare const isGreaterThanOrEqualTo: (other: number, value: unknown) => value is number;
declare const isLessThanOrEqualTo: (other: number, value: unknown) => value is number;
declare const isNear: (other: number, epsilon: number, value: unknown) => value is number;
declare const isNegativeNumber: (value: unknown) => value is number;
declare const isNumber: (value: unknown) => value is number;
declare const isOddNumber: (value: unknown) => value is number;
declare const isPositiveNumber: (value: unknown) => value is number;
declare const isWholeNumber: (value: unknown) => value is number;
declare const isWithinRange: (
floor: number,
ceiling: number,
value: unknown,
) => value is number;
declare const endsWith: (other: string, value: unknown) => value is string;
declare const isEmptyString: (value: unknown) => value is string;
declare const isIso8601: (value: unknown) => value is string;
declare const isJsonString: (value: unknown) => value is string;
declare const isLongerThan: (other: string, value: unknown) => value is string;
declare const isNonEmptyString: (value: unknown) => value is string;
declare const isSameLengthAs: (other: string, value: unknown) => value is string;
declare const isShorterThan: (other: string, value: unknown) => value is string;
declare const isString: (value: unknown) => value is string;
declare const isVisibleString: (value: unknown) => value is string;
declare const isWhitespace: (value: unknown) => value is string;
declare const startsWith: (other: string, value: unknown) => value is string;
expect-more/gen
declare const withMissingBranches: (value: object | any[]) => Generator;
declare const withMissingLeaves: (value: object | any[]) => Generator;
declare const withMissingNodes: (value: object | any[]) => Generator;
declare const withNullBranches: (value: object | any[]) => Generator;
declare const withNullLeaves: (value: object | any[]) => Generator;
declare const withNullNodes: (value: object | any[]) => Generator;
FAQs
Curried JavaScript Type Testing Library with Zero Dependencies
The npm package expect-more receives a total of 45,701 weekly downloads. As such, expect-more popularity was classified as popular.
We found that expect-more demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.